Common Pitfalls When Using CapeDwarf on Java EE Apps
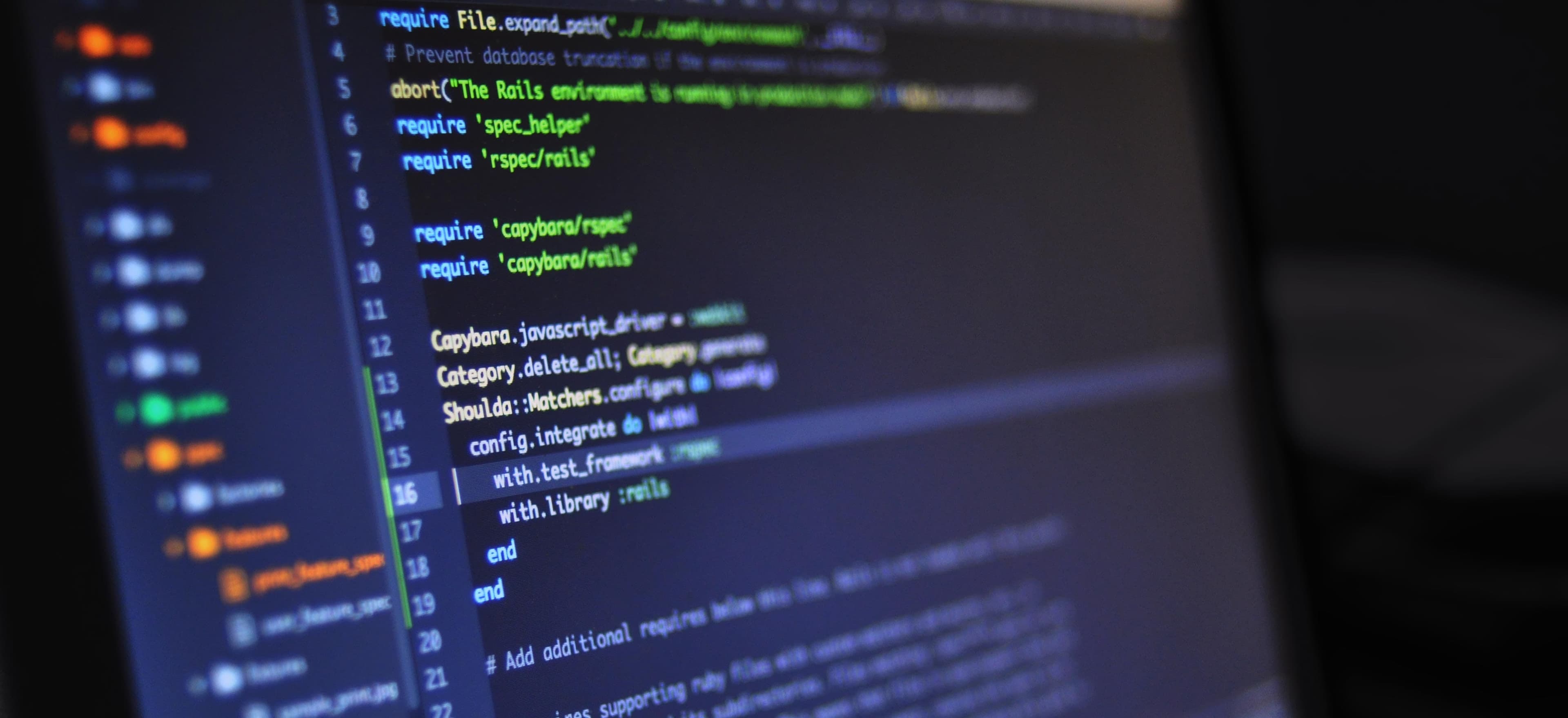
- Published on
Common Pitfalls When Using CapeDwarf on Java EE Apps
Java EE (Jakarta EE) applications benefit a great deal from their robust architecture, but when integrating with frameworks like CapeDwarf, developers often encounter challenges. CapeDwarf, an open-source framework for building applications on Google App Engine, presents unique opportunities alongside potential pitfalls. In this blog post, we’ll explore some common pitfalls when using CapeDwarf in Java EE apps and how to navigate them efficiently.
Understanding CapeDwarf
Before we dive into the pitfalls, it's crucial to understand what CapeDwarf is. Essentially, CapeDwarf acts as a bridge between the Java Standard Edition (SE) and the Google App Engine market. It allows developers to use the Java language seamlessly within the Google Cloud ecosystem while enjoying flexibility in application builds.
However, like all tools, improper usage can lead to complications. Here are some pitfalls to watch out for:
1. Improper Dependency Management
One of the most frequent issues encountered is improper management of dependencies in your project. In Java applications, dependency management is critical for maintaining compatibility and avoiding version conflicts.
Example of Dependency Configuration in Maven:
<dependencies>
<dependency>
<groupId>com.google.appengine</groupId>
<artifactId>appengine-api-1.0-sdk</artifactId>
<version>1.9.30</version>
</dependency>
<dependency>
<groupId>com.capedwarf</groupId>
<artifactId>capedwarf-core</artifactId>
<version>1.0.0</version>
</dependency>
</dependencies>
Why It Matters:
Using incompatible versions can lead to ClassNotFoundExceptions or runtime failures. To avoid such issues, ensure you lock compatible versions of all dependencies. Furthermore, regularly review updates to your dependencies while keeping their compatibility requirements in check.
2. Misconfiguring the Application Environment
It’s essential to configure the deployment environment accurately. Misconfigurations can take the form of incorrect settings in the appengine-web.xml
or faulty application properties.
Example Application Configuration:
<appengine-web-app xmlns="http://appengine.google.com/ns/1.0">
<application>your-app-id</application>
<version>1</version>
<threadsafe>true</threadsafe>
<runtime>java8</runtime>
</appengine-web-app>
Why It Matters:
Failing to configure the correct runtime or enabling threadsafety can lead to performance issues or even application crashes. Always ensure that your configurations align with the specifications mentioned in the CapeDwarf documentation.
3. Ignoring the Differences in Data Access Patterns
Google App Engine employs a non-relational data model through the Datastore, which behaves differently from traditional relational databases. Many developers new to this paradigm struggle with the fundamental difference in data access patterns.
Things to Consider:
- Datastore transactions: Understand that Datastore only supports transactions for entities with the same ancestor.
- Read and Write limits: App Engine has strict quotas and limits on reads and writes.
Code Snippet for a Transaction:
DatastoreService datastore = DatastoreServiceFactory.getDatastoreService();
Transaction txn = datastore.beginTransaction();
try {
Entity user = new Entity("User", userId);
user.setProperty("name", "John Doe");
datastore.put(user);
txn.commit();
} catch (Exception e) {
txn.rollback();
throw new RuntimeException("Error saving user", e);
}
Why It Matters:
Designing your data structure around these limitations is critical. If you ignore them, your application may perform poorly under load or fail to save data correctly.
4. Overlooking Logging and Debugging Capabilities
Logging is critical in any application development process, but in the context of Cloud-based apps, it gains even more significance. Developers may overlook the capabilities provided by CapeDwarf, resulting in challenges during the debugging process.
Setting Up Logging:
CapeDwarf integrates well with SLF4J, allowing for effective logging practices.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class UserService {
private static final Logger logger = LoggerFactory.getLogger(UserService.class);
public void createUser(String username) {
logger.info("Creating user: " + username);
// User creation code here
}
}
Why It Matters:
Effective logging not only assists in debugging but also helps in monitoring the application's health. Ignoring logging can lead to long troubleshooting sessions, making it difficult to pinpoint issues.
5. Not Leveraging Built-in Features
CapeDwarf comes with several built-in features designed to facilitate your development process. Not leveraging these features could lead to unnecessarily complicating your application’s architecture.
Built-in Features to Consider:
- User Authentication: CapeDwarf has integrated authentication mechanisms that allow you to quickly set up users within your app without building from scratch.
Example of Using the User API:
import com.capedwarf.users.UserApi;
import com.capedwarf.users.User;
public User fetchCurrentUser() {
User user = UserApi.getCurrentUser();
if (user != null) {
return user;
} else {
return null; // Handle unauthenticated user here
}
}
Why It Matters:
Instead of reinventing the wheel for tasks already covered by CapeDwarf, focus on utilizing these features. This not only speeds up development but also improves your application's robustness by relying on tested functionalities.
Closing Remarks
Developers using CapeDwarf in Java EE applications can maximize their productivity by avoiding the common pitfalls discussed in this post. Proper dependency management, accurate environment configuration, understanding data access paradigms, leveraging logging capabilities, and making full use of built-in features are all essential to achieving success.
By taking the time to set up your Java EE applications correctly and by referring to resources like the CapeDwarf Documentation, you can ensure a smoother development process. These preventative measures will allow you to harness the full potential of CapeDwarf, paving the way to building scalable and robust applications on Google Cloud Platform.
Now, go ahead and explore the fascinating world of CapeDwarf in your Java EE projects. Avoid these pitfalls, and you'll be on your way to becoming a proficient developer on the Google Cloud.
Checkout our other articles