Common Pitfalls in Java Observer Pattern Implementation
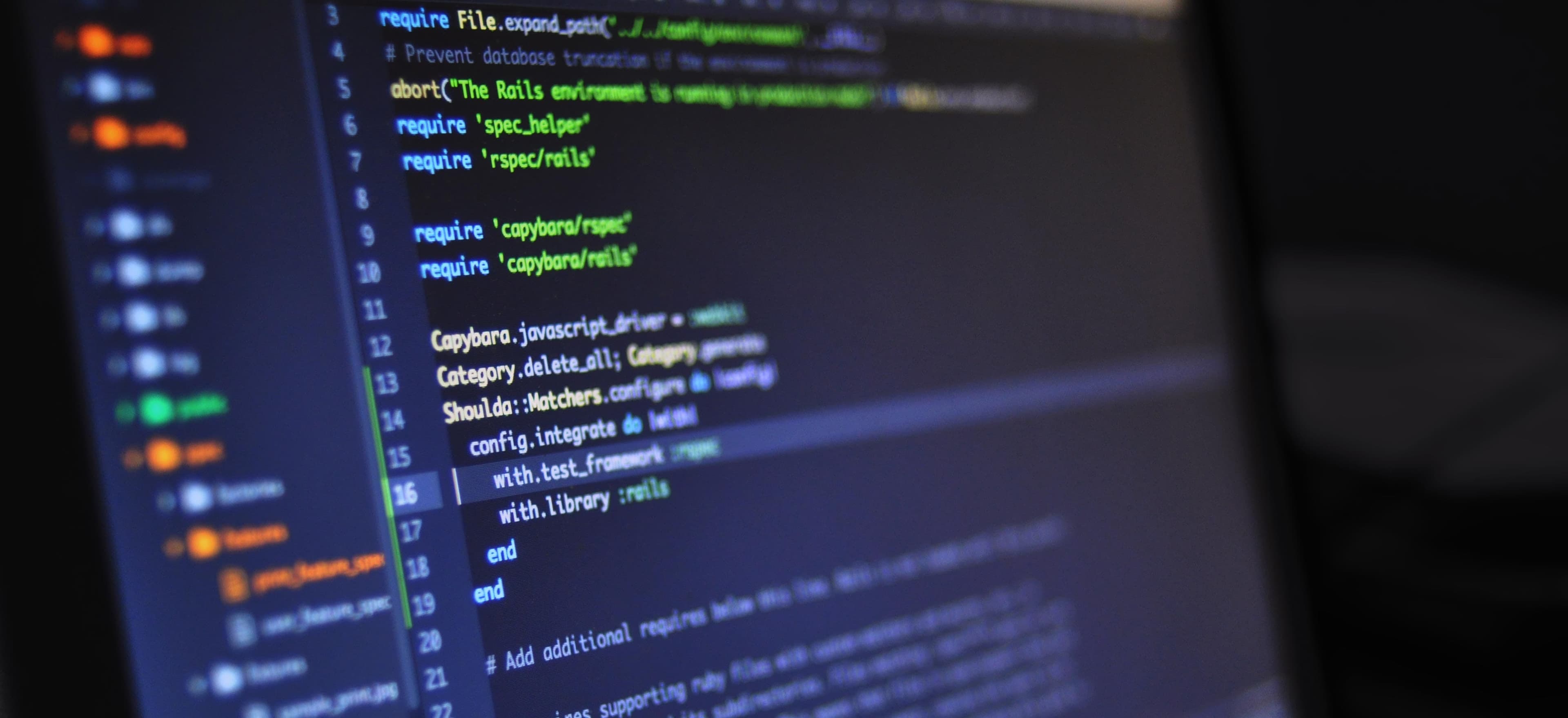
- Published on
Common Pitfalls in Java Observer Pattern Implementation
The Observer Pattern is a core design pattern in software engineering. It allows a subject (or publisher) to notify multiple observers (or subscribers) about changes in its state, creating a loose coupling between components. This pattern is widely used in event handling systems, including user interface frameworks. However, its implementation can be riddled with challenges. In this blog post, we will explore some common pitfalls when implementing the Observer Pattern in Java and provide strategies to avoid them.
What Is the Observer Pattern?
Before delving into the pitfalls, let's briefly review the Observer Pattern. In essence, it involves three main components:
- Subject (Observable): Maintains a list of observers and provides methods to attach and detach them.
- Observer: Defines an updating interface for objects that should be notified of changes to the subject.
- Concrete Subject and Concrete Observer: Implements the behavior of both subject and observer, respectively.
Here's a basic example of how the Observer Pattern can be structured in Java:
import java.util.ArrayList;
import java.util.List;
// Observer Interface
interface Observer {
void update(String message);
}
// Subject Class
class Subject {
private List<Observer> observers = new ArrayList<>();
public void attach(Observer observer) {
observers.add(observer);
}
public void detach(Observer observer) {
observers.remove(observer);
}
public void notifyObservers(String message) {
for (Observer observer : observers) {
observer.update(message);
}
}
}
// Concrete Observer
class ConcreteObserver implements Observer {
private String name;
public ConcreteObserver(String name) {
this.name = name;
}
@Override
public void update(String message) {
System.out.println(name + " received: " + message);
}
}
Scenario
In this example, when the Subject
changes its state (or some relevant condition triggers), it calls the notifyObservers
method. The attached observers are then informed via their update
method. This simple approach can lead us to the first common pitfall.
Pitfall 1: Not Handling Observer Lifecycle
A critical aspect often overlooked is managing the lifecycle of observers. If observers are not removed properly, it can lead to memory leaks or unwanted notifications when they no longer need to observe a subject.
Solution: Use a dedicated detach mechanism and maintain clean references. Always ensure that when an observer is no longer needed, it is removed properly from the list.
public void detach(Observer observer) {
observers.remove(observer);
// Consider using a weak reference if necessary
}
Why This Matters
Failure to detach observers can lead to stale data being sent to observers, resulting in an application that behaves unpredictably or grows increasingly inefficient over time.
Pitfall 2: Lack of Thread Safety
When implementing the Observer Pattern, concurrency issues can arise, especially in multi-threaded applications. If an observer is modified or detached during notification, it can result in a ConcurrentModificationException
or an inconsistent state.
Solution: Utilize synchronization mechanisms or thread-safe collections (such as CopyOnWriteArrayList
) for managing observers.
import java.util.concurrent.CopyOnWriteArrayList;
class ThreadSafeSubject {
private List<Observer> observers = new CopyOnWriteArrayList<>();
public void attach(Observer observer) {
observers.add(observer);
}
public void detach(Observer observer) {
observers.remove(observer);
}
public void notifyObservers(String message) {
for (Observer observer : observers) {
observer.update(message);
}
}
}
Why Thread Safety is Important
Java applications today often run in multi-threaded environments. Ensuring that your Observer implementation is thread-safe prevents unpredictable behavior, such as lost notifications or exceptions during concurrent modifications.
Pitfall 3: Over-Notification
A common misstep is notifying observers unnecessarily. This often occurs when changes to the subject's state trigger a cascade of updates, overwhelming observers, especially if they perform lengthy operations.
Solution: Implement change flags or hashing techniques to ensure that observers only receive relevant notifications.
public void notifyObservers(String updateType, String message) {
if (!"NO_CHANGE".equals(updateType)) {
// Performance optimization to reduce notifications
for (Observer observer : observers) {
observer.update(message);
}
}
}
Why Manage Notifications?
Over-notifying can lead to performance bottlenecks and decreased responsiveness in applications. Employing an efficient notification strategy will improve user experience and application throughput.
Pitfall 4: Observer Update Method Complexity
When observers have complex update
methods, it's easy to introduce bugs or fail to handle certain cases. Ensuring that your observer's state remains consistent is vital.
Solution: Keep the update
method simple and delegate complex operations to other methods or classes.
@Override
public void update(String message) {
processMessage(message);
}
private void processMessage(String message) {
// Handle message processing, e.g., updating UI or database
}
Why Simplicity Matters
Simplicity facilitates easier maintenance and debugging, while complex methods can hide bugs and lead to unexpected states in your application.
Pitfall 5: Informational Overshadowing
Sometimes the subject might provide more information than what observers actually need. When this happens, observers can become overloaded and slow down their operations. It is crucial to strike a balance between providing necessary and excessive updates.
Solution: Design a well-structured notification system that filters out irrelevant details.
public void notifyObservers(Update update) {
for (Observer observer : observers) {
observer.update(update.getSummary());
// Use the update object shape to limit the details sent.
}
}
Why Filtering is Important
Providing only the necessary data ensures that observers remain focused on relevant updates, reducing clutter and improving overall system performance.
The Closing Argument
The Observer Pattern is a powerful construct that, if implemented correctly, can significantly enhance application architecture. However, if mishandled, it can lead to a range of problems from memory leaks to complex bugs.
By understanding these common pitfalls and their solutions, developers can create robust and maintainable observer implementations. Emphasizing lifecycle management, thread safety, efficient notifications, simplicity, and appropriate data sharing will ensure that your Observer Pattern implementation remains effective and scalable.
For further reading, check out Design Patterns: Elements of Reusable Object-Oriented Software and the Oracle Java Tutorials on Event Handling.
Embarking on your Observer Pattern journey can be daunting, but with these insights, you are better equipped to handle the obstacles that may arise.
This blog post seamlessly integrates code snippets, recommendations, and rationale, providing a holistic understanding of the Observer Pattern in Java. Aim for continual learning as you apply these best practices!