Top 5 Hibernate Questions That Trip Up Developers
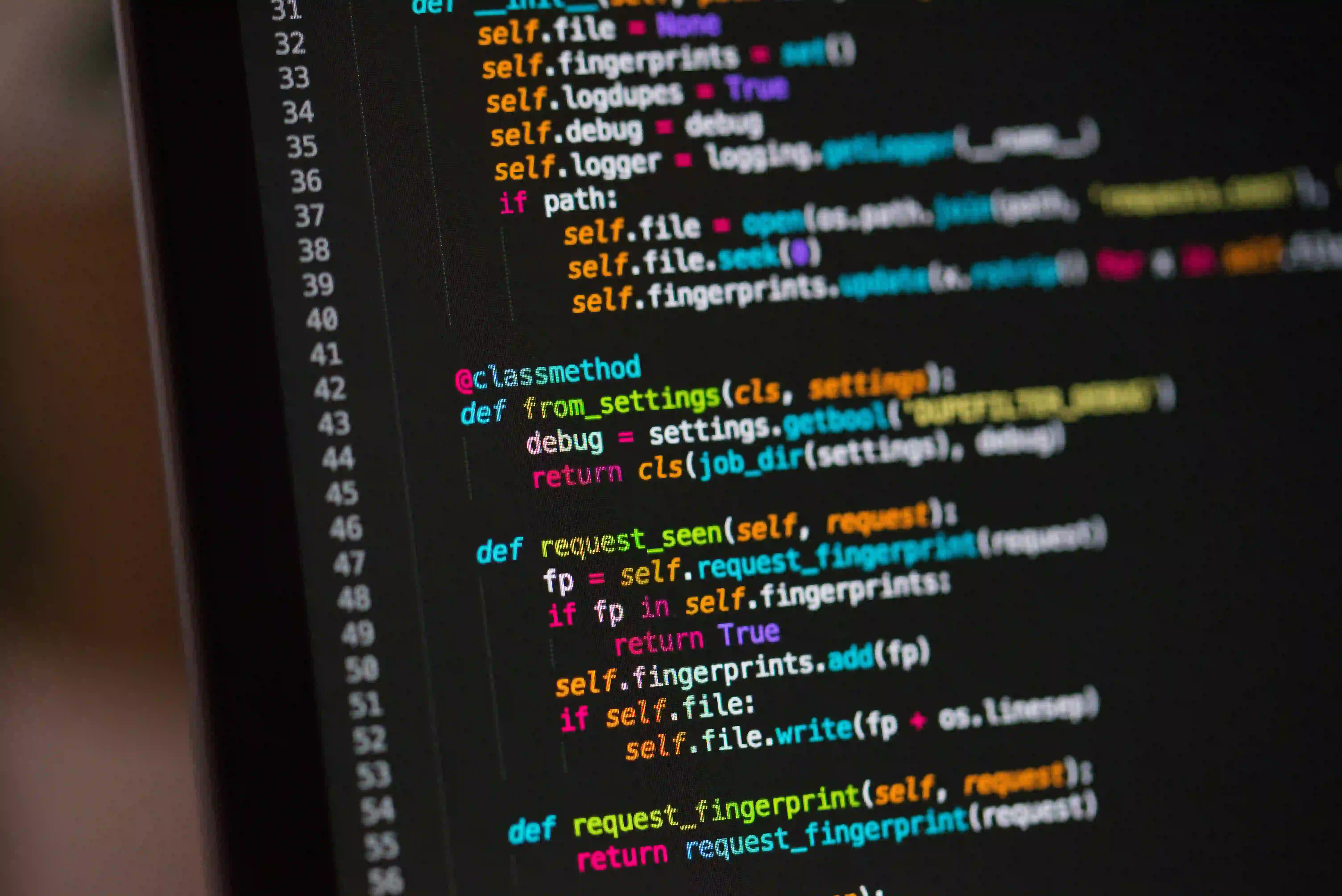
Top 5 Hibernate Questions That Trip Up Developers
Hibernate is an object-relational mapping (ORM) framework for Java, used to simplify database interactions by allowing developers to work with objects instead of traditional SQL queries. Despite its powerful features and flexibility, many developers encounter difficulties when working with Hibernate. This blog post dives into five common Hibernate questions that trip up developers, providing insights and clarification to help you navigate these challenges.
1. What is the N+1 Select Problem, and How Can It Be Avoided?
The N+1 Select Problem occurs when an application issues one query to fetch a list of entities and then an additional query for each entity to fetch associated entities. This can lead to performance inefficiencies, especially when dealing with larger datasets.
Example Scenario
Suppose you have an Order
entity that has a one-to-many relationship with an OrderItem
entity. If you retrieve a list of Order
objects but trigger a fetch for OrderItem
for each retrieved Order
, you experience an N+1 Select Problem.
List<Order> orders = session.createQuery("FROM Order", Order.class).list();
for (Order order : orders) {
List<OrderItem> items = order.getOrderItems(); // This causes an additional query for each order
}
Solution
To mitigate this problem, use Hibernate's JOIN FETCH
to fetch associated collections in the same query.
List<Order> orders = session.createQuery("SELECT DISTINCT o FROM Order o JOIN FETCH o.orderItems", Order.class).list();
This efficiently retrieves the Order
entities along with their associated OrderItem
entities in one go.
2. How Does Lazy Loading Work in Hibernate?
Lazy loading is a design pattern used to optimize performance by postponing initialization of an entity until it is needed. This means that instead of loading all associated data upfront, Hibernate loads it on demand.
Eager vs Lazy Fetching
Hibernate supports both eager and lazy fetching strategies. Eager fetching loads all associated data when the parent entity is fetched, while lazy fetching keeps related fields uninitialized until accessed.
To illustrate, consider the following mapping:
@Entity
public class Customer {
@OneToMany(fetch = FetchType.LAZY)
private Set<Order> orders;
}
Pros and Cons
- Pros: With lazy loading, the initial load time decreases. You only retrieve the data you need, which improves performance.
- Cons: Lazy loading can lead to
LazyInitializationException
if the session is closed before access to a lazily initialized object.
To avoid these exceptions, consider using OPEN_IN_VIEW
or explicitly initializing collections with Hibernate.initialize()
.
3. What’s the Difference Between merge()
and saveOrUpdate()
?
Both merge()
and saveOrUpdate()
are methods used for synchronizing objects with the Hibernate session. Yet, they behave differently in terms of object states.
saveOrUpdate()
- Use Case:
saveOrUpdate()
works with transient instances and can be used to either create new records or update existing ones based on whether the entity is already in the session or database. - Behavior: It may trigger a new insert or an update operation.
Example
session.saveOrUpdate(existingOrder); // Updates if exists, else saves new
merge()
- Use Case: The
merge()
method is primarily used for detached instances. When callingmerge()
, it copies the state of the given object onto the persistent object with the same identifier. - Behavior: It returns a new instance that is managed by the session.
Example
Order managedOrder = (Order) session.merge(detachedOrder);
In brief, use saveOrUpdate()
for transient objects and merge()
for detached objects that may have come from a different session.
4. How to Handle Transactions in Hibernate?
Transactions form the backbone of data integrity in applications. Handling transactions correctly in Hibernate requires understanding the differences between manual and automatic transaction management.
Manual Transaction Management
In manual management, you manage the transaction lifecycle yourself:
Session session = sessionFactory.openSession();
Transaction tx = null;
try {
tx = session.beginTransaction();
// Your database operation here
tx.commit(); // Must commit the transaction explicitly
} catch (Exception e) {
if (tx != null) {
tx.rollback(); // Always rollback on failure
}
} finally {
session.close(); // Ensure resources are released
}
Automatic Transaction Management
With Spring, for example, you can simplify transaction management with annotations:
@Service
public class OrderService {
@Transactional
public void createOrder(Order order) {
// Hibernate will manage the transaction automatically
session.save(order);
}
}
This automatic management reduces boilerplate code and makes the code cleaner and more maintainable.
5. What Are Hibernate Caches and How Do They Work?
Caching is crucial for optimizing performance by minimizing database access. Hibernate incorporates both first-level (session cache) and second-level cache.
First-Level Cache
The first-level cache is automatically associated with the Hibernate session. It acts as a temporary storage that exists while the session is active, strictly managing entity instances by their identifiers.
Session session = sessionFactory.openSession();
Order order1 = session.get(Order.class, 1L);
Order order2 = session.get(Order.class, 1L); // order2 is retrieved from the first-level cache
Second-Level Cache
The second-level cache is optional and shared among sessions. It can store data across different sessions and is designed to optimize read-heavy operations.
To enable second-level caching, configure your hibernate.cfg.xml
file:
<property name="hibernate.cache.use_second_level_cache">true</property>
<property name="hibernate.cache.region.factory_class">org.hibernate.cache.ehcache.EhCacheRegionFactory</property>
Key Takeaways
- Efficiency: Caching reduces the number of database accesses, boosting performance.
- Configuration: Customize which entities and collections are cached based on your application's needs.
The Bottom Line
Hibernate, while powerful, presents various challenges for developers, especially if you are new to ORM concepts. Understanding the N+1 Select Problem, lazy loading, transaction management, and the nuances of caching are essential to effective Hibernate usage. By keeping these common pitfalls in mind, you can avoid common mistakes and write better, more efficient Java applications.
If you would like to learn more about Hibernate in depth, consider checking out the official Hibernate documentation for more resources and examples.
Mastering these concepts not only enhances your skills as a Java developer but also significantly improves application performance. Happy coding!