Unlocking Java 15's Hidden Classes: A Practical Guide
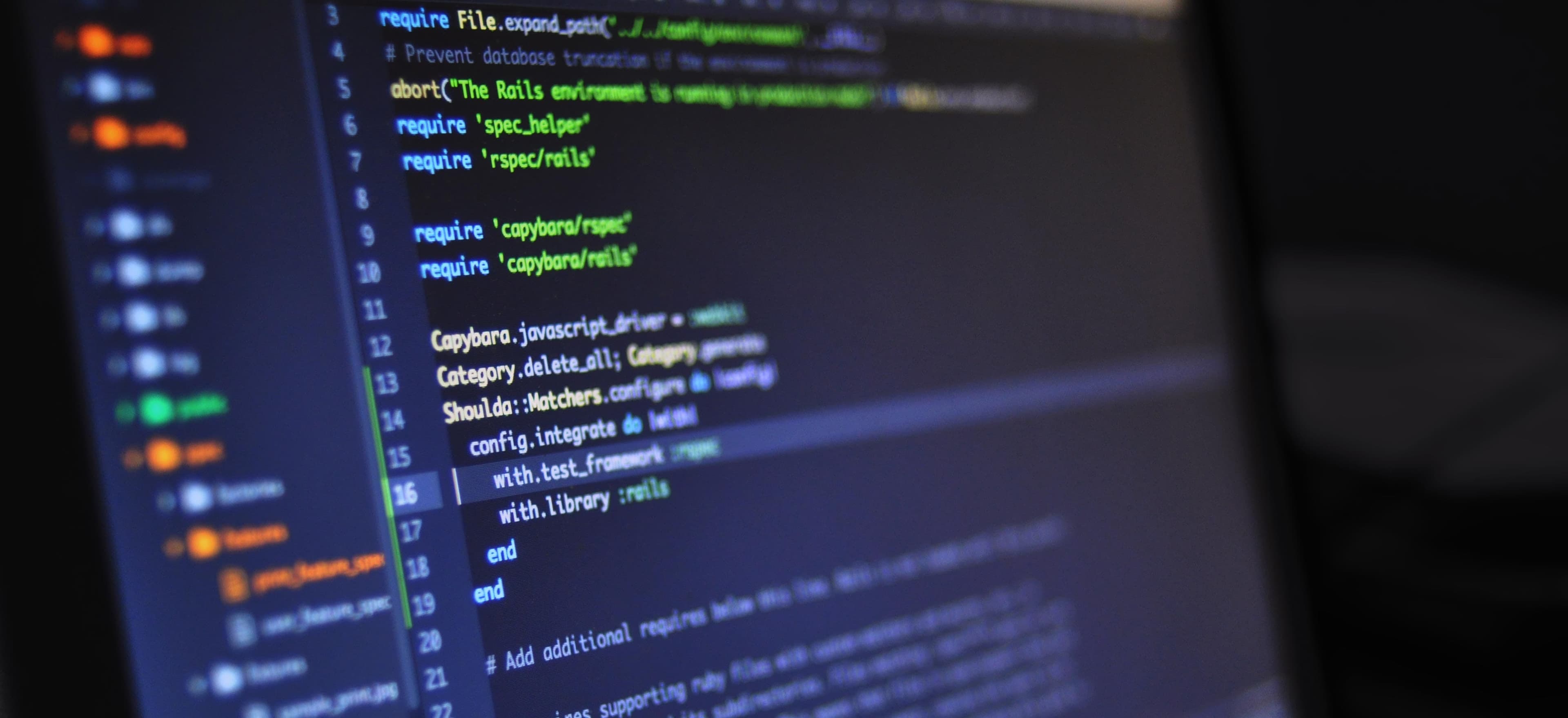
- Published on
Unlocking Java 15's Hidden Classes: A Practical Guide
Java has always been a powerful language for building robust and scalable applications. With each iteration, Java brings exciting features that enhance its functionality and usability. One of the underrated but impactful features introduced in Java 15 is hidden classes. This feature is particularly beneficial for frameworks that generate classes at runtime, such as those used in serialization and proxies. In this blog post, we will explore hidden classes, their use cases, and provide practical examples.
What are Hidden Classes?
Hidden classes in Java are classes that are not discoverable by the application classloader but are necessary for framework functionalities. This additional level of encapsulation ensures that the classes can only be accessed by their companion classes, thus avoiding naming conflicts and improving security.
Key Characteristics of Hidden Classes:
- Non-accessible: Hidden classes are not visible to the normal class lookup procedures.
- Restricted Instantiate: They can only be instantiated by the classes that have been defined to access them.
- Reduced Clutter: Using hidden classes helps keep the runtime environment clean by limiting the visibility of dynamically generated classes.
Why Use Hidden Classes?
Framework developers can greatly benefit from hidden classes. Here are several reasons why:
- Encapsulation: They reduce the risk of conflicts between classes with similar names in different libraries.
- Security: By limiting the access to these classes, frameworks can prevent unintended or malicious use.
- Improved Performance: Concealment of unnecessary classes from user code can result in better performance, especially in large applications.
How to Create Hidden Classes
Creating hidden classes in Java is straightforward using the java.lang.invoke
package. Now let’s delve into some code snippets to illustrate how to create and use hidden classes effectively.
Step 1: Create a Hidden Class
To create a hidden class, you will use the MethodHandles.Lookup
class. Here is how you can do it:
import java.lang.invoke.MethodHandles;
import java.lang.invoke.MethodType;
public class HiddenClassExample {
public static void main(String[] args) throws Throwable {
// Get the lookup for the current class
MethodHandles.Lookup lookup = MethodHandles.lookup();
// Define a hidden class
Class<?> hiddenClass = lookup.defineClass(MethodHandles.Lookup.class.getResourceAsStream("/path/to/MyHiddenClass.class"));
// Instantiate the hidden class
Object hiddenInstance = hiddenClass.getDeclaredConstructor().newInstance();
// Confirm the instance is created
System.out.println("A hidden class instance was created: " + hiddenInstance);
}
}
Explanation of the Code:
- Get the Lookup: The
Lookup
object is obtained usingMethodHandles.lookup()
. This provides the necessary access to create the hidden class. - Define the Class: The
defineClass
method takes anInputStream
of the class file. This can be created dynamically or be sourced from anywhere. - Instantiate the Class: Finally, we create an instance of the hidden class using reflection.
Important Note on Class Loading
Hidden classes are tied to the lookup object that created them. This ensures that only that particular lookup can instantiate or access the hidden classes. This mechanism helps in maintaining tighter control over the infinite scope of classes in large systems.
Use Case: Dynamic Proxy Creation
Apart from everyday application development, hidden classes shine in the realm of dynamic proxies. Let’s build a simple example to illustrate this.
Step 2: Creating a Dynamic Proxy
You can use hidden classes to create dynamic proxies that are hidden to the outside world.
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
public class DynamicProxyExample {
interface HelloWorld {
void greet(String name);
}
static class HiddenInvocationHandler implements InvocationHandler {
private final HelloWorld original;
public HiddenInvocationHandler(HelloWorld original) {
this.original = original;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
System.out.println("Before method: " + method.getName());
return method.invoke(original, args);
}
}
public static void main(String[] args) {
HelloWorld realHello = new HelloWorld() {
@Override
public void greet(String name) {
System.out.println("Hello, " + name);
}
};
HelloWorld proxyInstance = (HelloWorld) Proxy.newProxyInstance(
DynamicProxyExample.class.getClassLoader(),
new Class<?>[]{HelloWorld.class},
new HiddenInvocationHandler(realHello)
);
proxyInstance.greet("Java Developer");
}
}
Explanation of the Proxy Code
- HelloWorld Interface: This defines a simple interface with a
greet
method. - Invocation Handler: The
HiddenInvocationHandler
manages the calls to the actual implementation. - Creating the Proxy: The
Proxy.newProxyInstance
method creates a proxy instance of theHelloWorld
interface, wrapped around the real implementation, capturing invocations seamlessly.
Final Considerations
Java 15’s hidden classes provide significant advantages for advanced Java programming, especially for frameworks and libraries that thrive on runtime class generation. The combination of encapsulation, security, and performance is enticing for developers who want to enhance modularity and maintainability.
Resources like the Java Documentation and Baeldung can provide further insights into advanced Java concepts. Engage with the community, practice these patterns, and keep an eye on future Java updates as they continue to evolve and enhance our programming experience.
Embrace the power of hidden classes and elevate your Java application development to new heights!
Checkout our other articles