Common Java Mistakes New Developers Make and How to Avoid Them
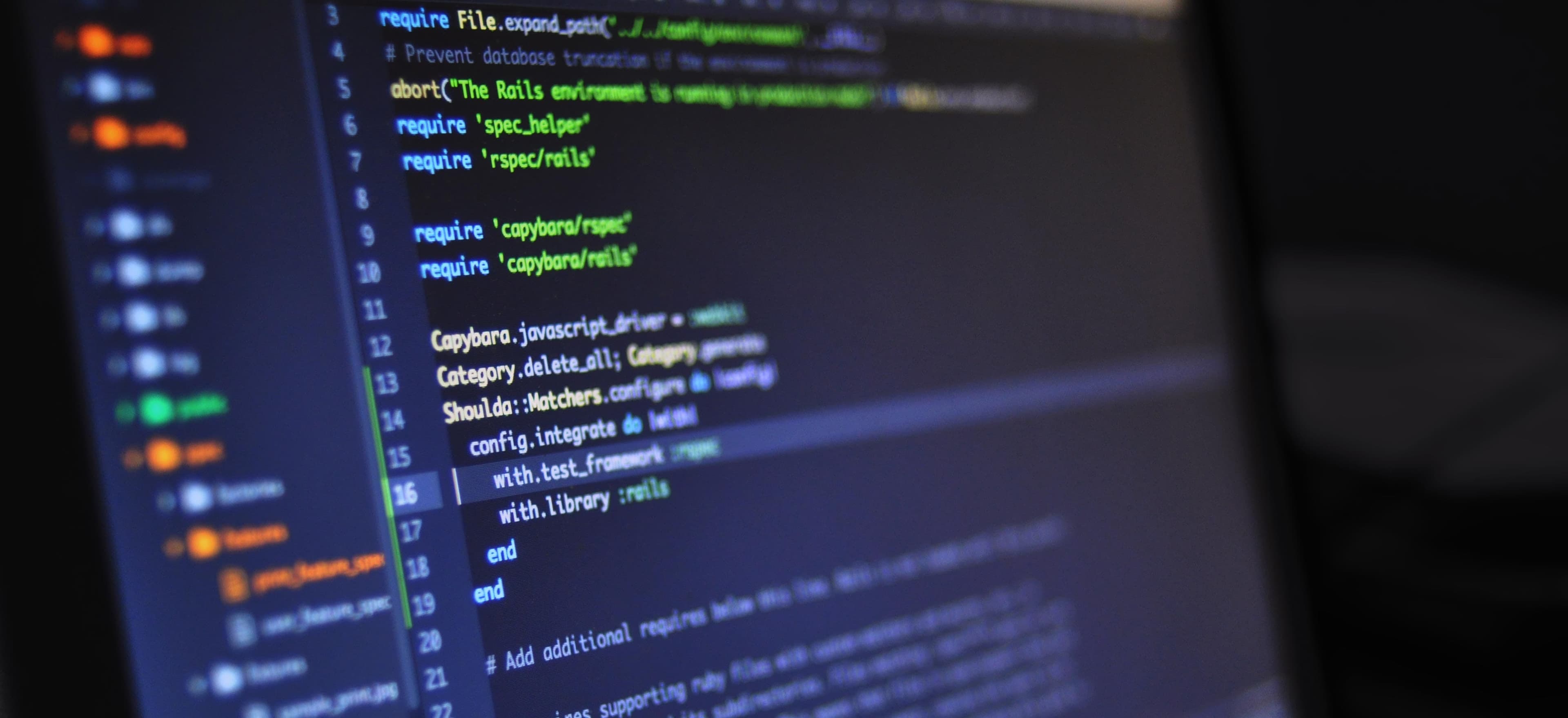
- Published on
Common Java Mistakes New Developers Make and How to Avoid Them
Java is one of the most popular programming languages in the world, cherished for its simplicity, object-oriented approach, and robustness. However, as new developers embark on their Java programming journey, they often stumble upon certain pitfalls. In this blog post, we will explore common mistakes made by beginners and provide solutions to avoid them. By understanding these common mistakes, you will improve your coding skills significantly and build a strong foundation for your Java development career.
1. Ignoring Best Practices for Naming Conventions
In Java, naming conventions are critical for readability and maintainability. Beginners often struggle with naming variables, classes, and methods.
Example of Poor Naming
int x; // What does x mean?
String d; // Unclear what d stands for
Solution: Follow Naming Conventions
- Use descriptive names:
int numberOfStudents;
- Employ camelCase for variables and methods:
calculateTotalSalary();
- Use PascalCase for classes:
StudentDetails;
These naming conventions not only clarify your intent but also make it easier for others to understand your code.
Why This Matters
Adhering to naming conventions brings consistency across the codebase. Once you and other developers recognize naming patterns, navigating and maintaining the code becomes much simpler.
2. Not Utilizing the Java Collection Framework
Beginners often reinvent the wheel when it comes to data storage by creating custom data structures rather than using existing, robust ones offered by Java.
Example of a Custom Implementation
class CustomList {
private int[] items = new int[100];
private int size = 0;
public void add(int item) {
if (size < items.length) {
items[size++] = item;
}
}
}
Solution: Use Java Collections
Java provides a collection framework that includes classes like ArrayList
, HashMap
, and HashSet
. Utilizing these standard implementations reduces development time and increases efficiency.
Example Using ArrayList
import java.util.ArrayList;
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(10);
numbers.add(20);
System.out.println(numbers); // Prints: [10, 20]
Why This Matters
Using Java Collections saves time and effort because they come with built-in methods and functionalities that are optimized for performance. Moreover, they are well-documented and frequently updated.
3. Failing to Handle Exceptions Properly
New developers often neglect the importance of exception handling, leading to software that crashes unexpectedly.
Example of Ignored Exception Handling
public void divide(int a, int b) {
System.out.println(a / b); // What if b is zero?
}
Solution: Implement Try-Catch Blocks
Always anticipate exceptions, especially those that can occur during runtime. Use try-catch blocks effectively.
Example with Exception Handling
public void divide(int a, int b) {
try {
System.out.println(a / b);
} catch (ArithmeticException e) {
System.out.println("Error: Division by zero is not allowed.");
}
}
Why This Matters
Proper exception handling ensures that your application remains stable and provides meaningful feedback to the user, which enhances the overall user experience.
4. Overusing Static Methods and Variables
New developers sometimes fall into the trap of using static methods and variables excessively, assuming they simplify their code.
Example of Overuse
public class Utility {
public static void performTask() {
// Task implementation
}
}
Solution: Use Static Judiciously
Static should be used when a method or variable does not depend on the instance of a class. For most cases, instance methods are preferred.
Example with Instance Variables
public class Task {
private int taskId;
public Task(int taskId) {
this.taskId = taskId;
}
public void performTask() {
// Task implementation
}
}
Why This Matters
Excessive use of static can lead to a rigid design that lacks flexibility. Emphasizing instance methods allows for more dynamic and reusable code.
5. null Pointer Exceptions
The null pointer exception is a common error that catches many beginners off guard.
Example of a Null Pointer
String str = null;
System.out.println(str.length()); // Throws NullPointerException
Solution: Null Checks
Perform null checks before accessing methods or properties. Additionally, consider using Optional in Java 8 and higher.
Example with Null Check
String str = null;
if (str != null) {
System.out.println(str.length());
} else {
System.out.println("String is null.");
}
Using Optional
import java.util.Optional;
Optional<String> optionalStr = Optional.ofNullable(null);
optionalStr.ifPresent(s -> System.out.println(s.length()));
Why This Matters
Null pointer exceptions can lead to application crashes. Implementing null checks and using Optional improves code robustness, making your applications more reliable.
6. Not Understanding the Object-Oriented Programming Principles
Java is an object-oriented programming (OOP) language, and new developers often overlook OOP principles like inheritance, encapsulation, and polymorphism.
Example Without OOP Principles
public class Vehicle {
void start() {
System.out.println("Vehicle started");
}
}
Solution: Apply OOP Principles
Structure your code using encapsulation, inherit from classes properly, and utilize polymorphism effectively.
Example with OOP
public abstract class Vehicle {
abstract void start();
}
public class Car extends Vehicle {
@Override
void start() {
System.out.println("Car started");
}
}
public class Bike extends Vehicle {
@Override
void start() {
System.out.println("Bike started");
}
}
Why This Matters
Understanding and implementing OOP principles lead to cleaner, more organized, and reusable code. This is crucial when working on larger applications where code maintainability becomes a priority.
7. Neglecting Code Comments and Documentation
New developers sometimes forget to comment on their code, thinking that the code should be self-explanatory.
Example of No Comments
public void calculateTotal(int[] items) {
int total = 0;
for (int item : items) {
total += item;
}
System.out.println(total);
}
Solution: Add Meaningful Comments
Comment your code effectively. Explain why certain decisions were made and what specific blocks of code accomplish.
Example with Comments
/**
* Calculates the total price from an array of item prices.
* @param items Array of item prices.
*/
public void calculateTotal(int[] items) {
int total = 0; // Initialize total to accumulate prices
for (int item : items) {
total += item; // Adding each item price
}
System.out.println(total); // Print the total
}
Why This Matters
Proper code comments enhance code readability, making it easier for you and others to understand the logic behind your code. This is especially helpful when debugging or enhancing features later.
Final Thoughts
Java is a powerful language, but common pitfalls can hinder your progress as a developer. Avoiding these mistakes can significantly enhance your coding skills and streamline the development process. Always apply best practices, leverage built-in libraries, handle errors efficiently, and embrace OOP principles. With continued learning and experience, you will become a proficient Java developer, paving the way for a successful career.
For further reading, check out resources like Oracle's Java Documentation and Java Collections Tutorial from Oracle. Happy coding!