5 Common Code Review Pitfalls and How to Avoid Them
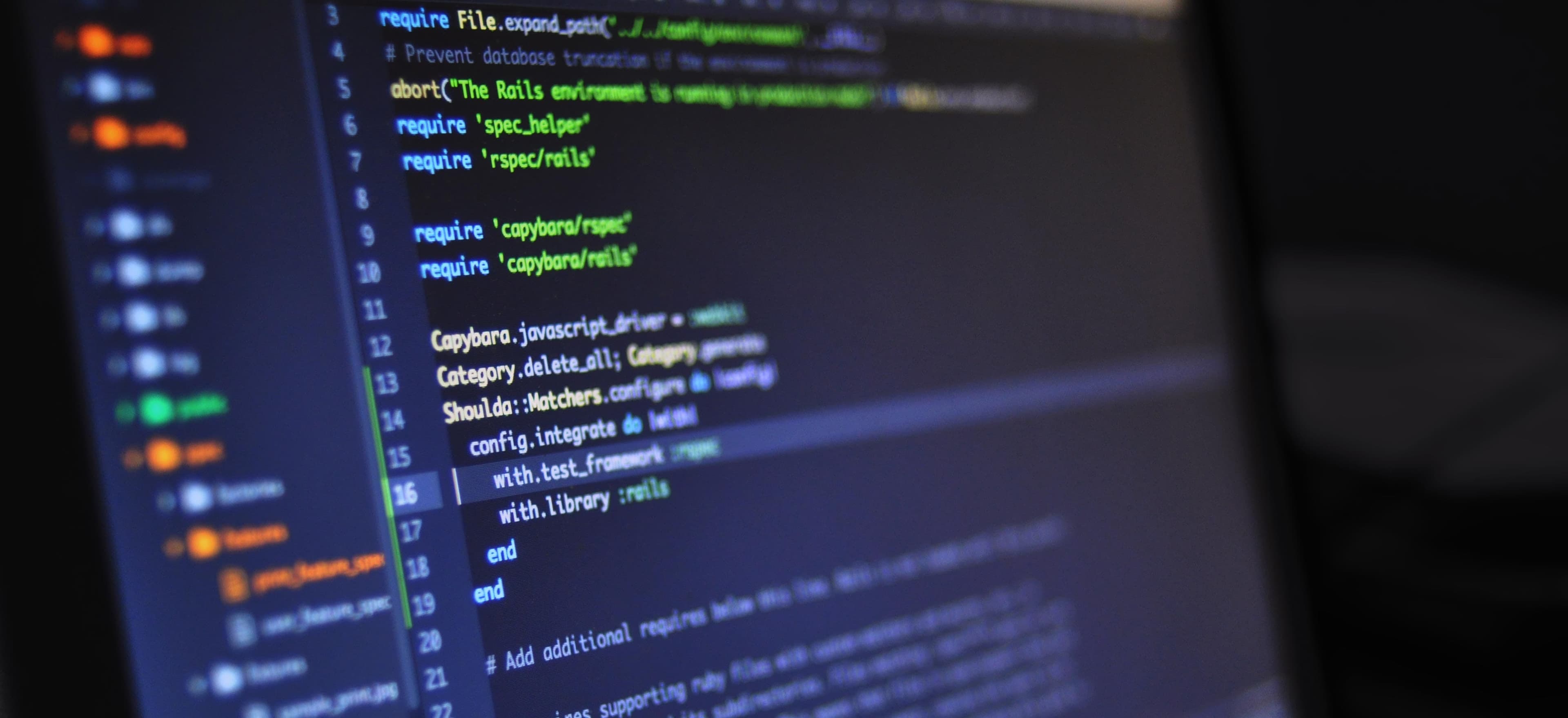
- Published on
5 Common Code Review Pitfalls and How to Avoid Them
In the world of software development, code reviews are an essential practice aimed at improving code quality, knowledge sharing, and team collaboration. However, code reviews can sometimes fall short of their intended goal. Here, we'll explore five common pitfalls in code review processes and propose effective strategies to avoid them.
1. Lack of Clarity in Code Comments
One of the most significant issues in code reviews occurs when code comments are unclear or entirely absent. Comments are not merely supplementary—they serve as documentation that enhances the understanding of complex logic and functionalities.
Why It Matters
Failure to provide clear comments can lead to misunderstandings, especially for new team members. Without context, reviewers may misinterpret the intent behind a piece of code.
Solution
Encourage developers to write meaningful comments. For example, instead of:
// This method does stuff
public void process() {
// logic here
}
Use more descriptive comments:
// Processes the user input and stores it in the database.
public void process() {
// Validate and store input in the database
}
Encouraging this practice can significantly enhance the code review process by ensuring everyone is on the same page.
2. Focusing Solely on Syntax
Another common pitfall is concentrating too much on syntax and style rather than the application logic and design. While maintaining coding standards is vital, reviewing for syntax alone can neglect deeper issues.
Why It Matters
Overemphasis on syntax can result in overlooking critical design flaws, performance issues, or potential security vulnerabilities which are far more consequential than minor stylistic inconsistencies.
Solution
Shift the review focus toward architecture, algorithms, and potential improvements. For instance, instead of merely checking the indentation:
void example(){
//some logic
}
Look for opportunities to enhance the algorithm's efficiency:
// Factorial calculation should prevent recursion depth issues using iteration.
public long factorial(int n) {
long result = 1;
for (int i = 2; i <= n; i++) {
result *= i;
}
return result;
}
This allows the reviewer to contribute to more impactful changes.
3. Skipping the Testing Phase
Code testing is often an afterthought in the code review process. In some cases, developers may submit code for review without adequate test cases, relying solely on the reviewer to check for potential bugs.
Why It Matters
Skipping tests can lead to issues in production and might undermine the whole code review process. Test cases validate functionality and enforce design contracts, making them crucial for securing code during reviews.
Solution
Develop a checklist that mandates test cases for all new code. For example:
// Unit test for factorial method
@Test
public void testFactorial() {
assertEquals(120, factorial(5));
assertEquals(1, factorial(0));
}
By incorporating testing as part of the review, the team can catch bugs early and improve reliability.
4. Rushing Through Reviews
It's not uncommon for reviewers to rush through the review process due to pressures of time. This hurried pace can lead to missing critical issues or undervaluing the review entirely.
Why It Matters
Rushed reviews can be counterproductive. It can breed frustration and lead to severe oversights that impact the quality of the codebase.
Solution
Allocate sufficient time for reviews and promote a culture where thorough checks are valued. Set realistic deadlines and encourage reviewers to be meticulous. For instance:
- Schedule regular code review sessions.
- Encourage developers to take breaks to return to code with fresh eyes.
A positive review environment leads to better code quality and team morale.
5. Avoiding Constructive Feedback
Finally, a culture that shies away from constructive criticism can stifle growth and learning. When feedback is vague or overly critical, it may affect the relationship between developers and lead to defensiveness.
Why It Matters
Constructive feedback encourages growth and promotes a better understanding of code. If developers dread receiving feedback, they may not put their best foot forward in their subsequent submissions.
Solution
Adopt a feedback model that emphasizes clear, constructive criticism. Consider the "sandwich" approach: positive feedback, then constructive points, and finish with encouraging remarks. For example:
- "I appreciate your use of clear naming conventions."
- "However, I see a potential issue with performance in this section."
- "Overall, this is a solid foundation; I look forward to seeing your next iteration!"
This approach fosters a positive learning environment that encourages improvement while highlighting strengths.
Key Takeaways
In summary, code reviews are an essential process in software development that can significantly influence the product quality and team dynamics. By recognizing these common pitfalls—lack of clarity in comments, overemphasis on syntax, neglecting tests, rushing through reviews, and avoiding constructive feedback—teams can implement strategies to mitigate them effectively.
By fostering a culture of clear communication, thorough examination, thoughtful criticism, and collaboration, teams can not only enhance their code quality but also their overall productivity.
For further insights into effective code reviews and best practices, refer to Atlassian's Guide to Code Reviews and GitHub’s documentation on Pull Requests.
With careful attention to these common pitfalls, your team can elevate its code review practices, ultimately leading to a more robust and reliable software product.