Common Pitfalls in Securing Java Applications
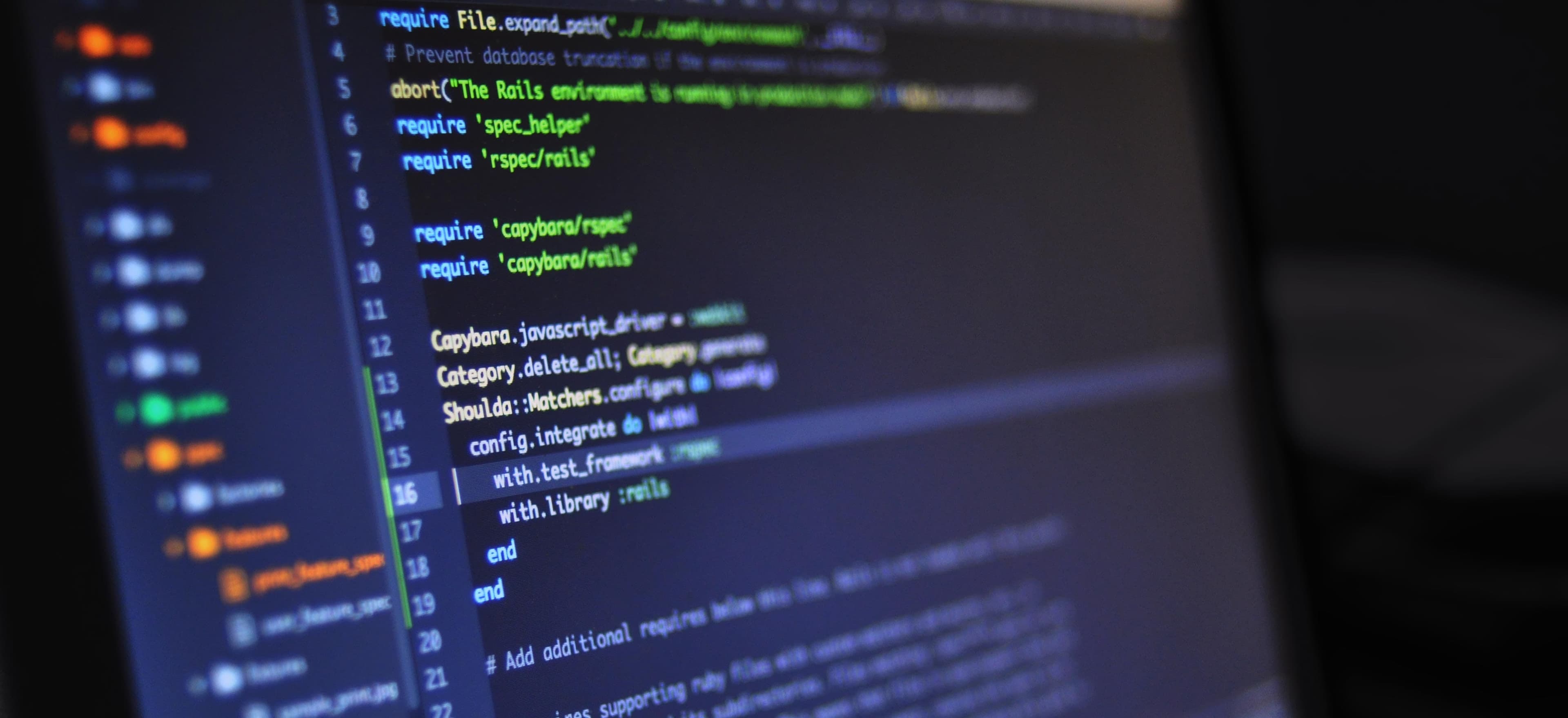
- Published on
Common Pitfalls in Securing Java Applications
In today's fast-paced digital world, security is paramount, especially for applications built using Java. Java, a robust and widely-used programming language, is utilized across various platforms and industries. However, despite its strengths, many developers encounter significant pitfalls when securing Java applications. This blog post will delve into these common pitfalls, providing insights and actionable recommendations to ensure your Java applications remain secure.
Understanding Java Security
Java was inherently designed with security in mind, utilizing a variety of features like the Java Security Manager and the Java Native Interface (JNI). However, building a secure application involves much more than just using the right tools. Developers must be cognizant of security best practices, and how their coding habits can inadvertently expose their applications to threats.
1. Improper Handling of User Input
Pitfall: Failing to validate user input can leave your application vulnerable to various forms of injection attacks, such as SQL injection and Cross-Site Scripting (XSS).
Solution: Always sanitize and validate input from users. For instance, using prepared statements for database queries is an excellent way to prevent SQL injection.
// Using prepared statements to prevent SQL Injection
String query = "SELECT * FROM users WHERE username = ? and password = ?";
try (PreparedStatement stmt = connection.prepareStatement(query)) {
stmt.setString(1, username);
stmt.setString(2, password);
ResultSet result = stmt.executeQuery();
}
In this code snippet, instead of building the SQL query as a string and risking injection, we use a PreparedStatement
which takes care of inserting user inputs safely.
2. Weak Authentication and Authorization
Pitfall: Many applications implement weak authentication mechanisms or neglect proper authorization checks.
Solution: Use established libraries and frameworks for authentication, like Spring Security or Apache Shiro. Always enforce strong password policies and consider multi-factor authentication (MFA) for added security.
// Example with Spring Security
public void configure(SecurityHttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
By leveraging Spring Security, we can ensure that only authenticated users can access certain parts of our application. This approach reduces the risk of unauthorized access.
3. Using Outdated Libraries and Dependencies
Pitfall: Every Java application relies on libraries and dependencies. Using outdated or vulnerable versions can put your application at risk.
Solution: Regularly update your dependencies and use tools like OWASP Dependency-Check to identify vulnerabilities in your libraries.
<!-- Maven Dependency Example -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
<version>2.5.6</version> <!-- Ensure this is the latest stable version -->
</dependency>
Staying up-to-date with libraries not only enhances functionality but also fortifies your application against known vulnerabilities.
4. Insufficient Logging and Monitoring
Pitfall: Without proper logging, it becomes impossible to trace security breaches or suspicious activities.
Solution: Implement robust logging and monitoring solutions like ELK Stack or Splunk. Ensure that sensitive actions, like login attempts and data access, are logged properly.
// Simple logging example using SLF4J
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class UserLogin {
private static final Logger logger = LoggerFactory.getLogger(UserLogin.class);
public void login(String username) {
logger.info("User {} attempting to log in", username);
// login logic
}
}
By logging user actions, developers can gain valuable insights that can be used to detect patterns that may indicate an attack.
5. Ignoring Secure Configuration
Pitfall: Many developers overlook configuring their applications securely. Default settings are often not sufficiently secure.
Solution: Always review and customize your application configurations. Use properties files or environment variables to hold sensitive information securely.
# application.properties
# Secure configuration example
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=${DB_USER} # Use environment variables for sensitive data
spring.datasource.password=${DB_PASSWORD}
Storing sensitive data like database credentials in environment variables instead of hardcoding them into your source code minimizes risks.
6. Insecure API Endpoints
Pitfall: Exposing your API endpoints without proper authentication can result in unauthorized access to sensitive functionalities.
Solution: Protect your APIs by validating user tokens and using HTTPS to encrypt data in transit.
@RequestMapping(value = "/secure-endpoint", method = RequestMethod.GET)
@PreAuthorize("hasRole('USER')")
public String secureEndpoint() {
return "This is a secure message!";
}
In this code, we secure an API endpoint using role-based access, ensuring that only users with the appropriate permissions can access it.
7. Failing to Implement Session Management
Pitfall: Poor session management can lead to session hijacking, where an attacker takes over a user session.
Solution: Enforce session timeouts and limit session lifetime. Utilize secure HTTP-only cookies to store session identifiers.
// Setting HTTP-only attribute in a session cookie
session.setMaxInactiveInterval(1800); // 30 minutes timeout
response.setHeader("Set-Cookie", "JSESSIONID=" + session.getId() + "; HttpOnly; Secure");
By configuring sessions correctly, we minimize the chance of session hijacking, enhancing the security of the user experience.
The Closing Argument
Securing Java applications requires a multifaceted approach. By recognizing and avoiding common pitfalls such as improper input handling, weak authentication, and overlooking dependency management, developers can significantly enhance their applications' security.
Stay vigilant, keep your libraries updated, and never underestimate the importance of coding with security in mind. For additional resources, consider visiting the OWASP Java Security guide for in-depth insights.
By embedding security into every phase of your development cycle, from design to deployment, you create a robust foundation for any Java application. As cyber threats continue to evolve, remaining informed and proactive is your best defense. Secure coding isn't just a practice—it's a necessity.