Troubleshooting Arquillian Tests on OpenShift's WildFly
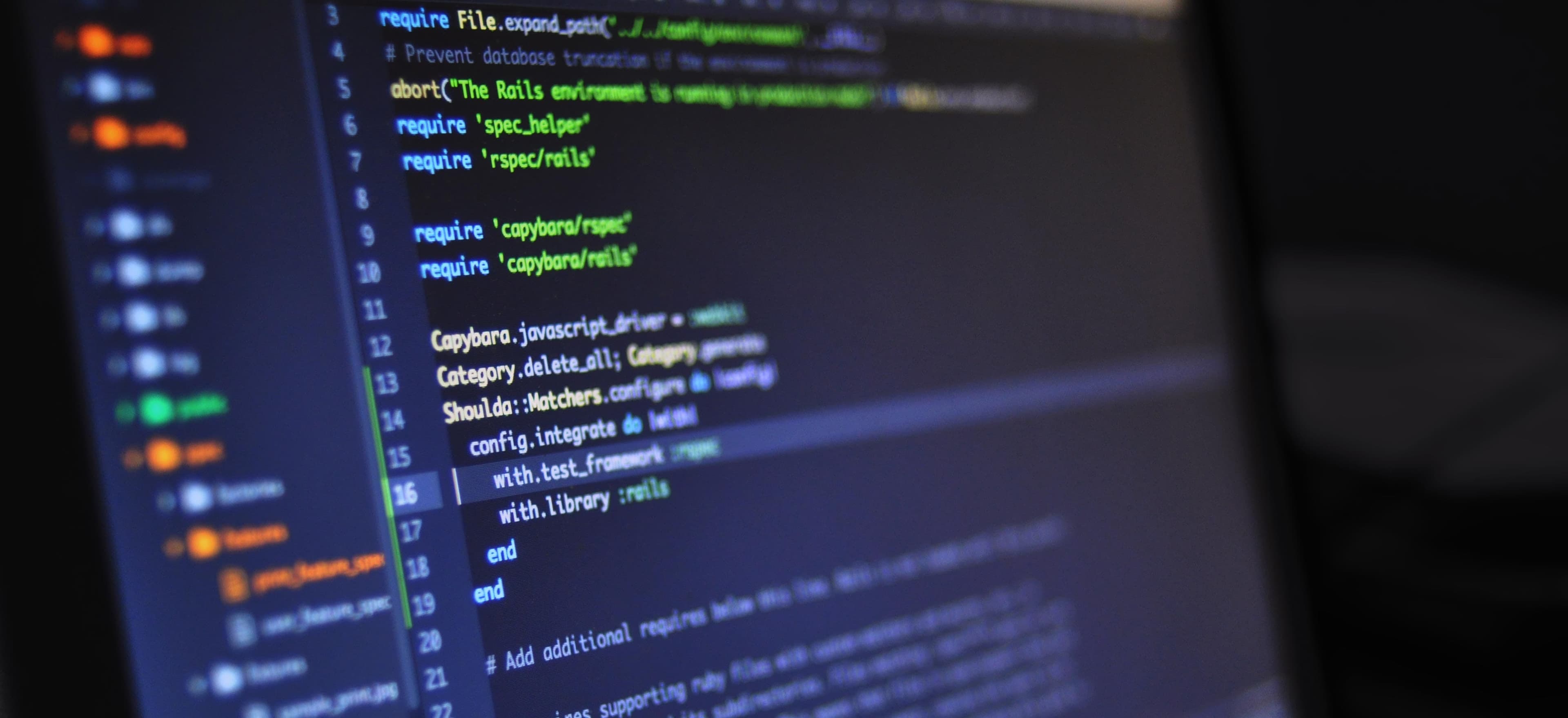
- Published on
Troubleshooting Arquillian Tests on OpenShift's WildFly
When grappling with containerized applications, especially Java applications running on WildFly with Arquillian testing, developers can encounter a myriad of issues that can seem overwhelming at first glance. However, with the right strategies and insight into each component, diagnosing problems becomes a less daunting task. This post aims to arm you with the tools and knowledge needed to troubleshoot Arquillian tests within the OpenShift ecosystem effectively.
What is Arquillian?
Before we dive into troubleshooting, let’s briefly discuss what Arquillian is. Arquillian is a powerful testing platform for Java that allows developers to execute tests inside different containers. This is particularly useful for integration tests where the interaction between multiple components is crucial. In the case of OpenShift, you are working with containerized applications, making Arquillian even more essential.
Why Use OpenShift with WildFly?
OpenShift is a robust platform for developing, deploying, and managing applications in a cloud environment. Paired with WildFly, an application server known for its lightweight and modular architecture, the combination offers:
- Scalability
- High availability
- A microservices-friendly environment
However, integrating Arquillian tests within this setup can introduce its own challenges.
Common Issues and Solutions
1. Connection Problems
Symptom: Your Arquillian tests are failing with connection refused errors.
Why it Happens: This often indicates that the application isn't available on the expected port or that the test configuration is incorrect.
Solution: Verify that your WildFly instance is running and check the environment configuration. The persistence.xml
file may need adjustments to connect to the correct database.
<properties>
<property name="javax.persistence.jdbc.url" value="jdbc:postgresql://<YOUR_DB_HOST>:<YOUR_DB_PORT>/<YOUR_DB_NAME>"/>
<property name="javax.persistence.jdbc.user" value="<YOUR_DB_USER>"/>
<property name="javax.persistence.jdbc.password" value="<YOUR_DB_PASSWORD>"/>
</properties>
2. Environment Configuration
Symptom: Tests pass locally but fail on OpenShift.
Why it Happens: This may be due to differences in environment variables or configurations between local and OpenShift deployments.
Solution: Ensure that the same configurations are applied in both environments. Use ConfigMaps
in OpenShift to manage these configurations.
apiVersion: v1
kind: ConfigMap
metadata:
name: app-config
data:
DB_URL: jdbc:postgresql://<YOUR_DB_HOST>:<YOUR_DB_PORT>/<YOUR_DB_NAME>
3. Dependency Issues
Symptom: Errors related to missing classes or libraries.
Why it Happens: Arquillian might be unable to detect or pull in certain dependencies necessary to run the tests.
Solution: Ensure that your pom.xml
contains all required dependencies. For example, if using JPA and Hibernate, ensure that they are specified:
<dependency>
<groupId>org.hibernate.orm</groupId>
<artifactId>hibernate-core</artifactId>
<version>${hibernate.version}</version>
<scope>provided</scope>
</dependency>
4. Arquillian Deployment Issues
Symptom: Deployment issues reported by Arquillian.
Why it Happens: If your application is not deployed correctly in the WildFly container, Arquillian tests will fail.
Solution: Review the deployment archive. Use an @Deployment
method to package the application correctly.
@Deployment
public static Archive<?> createTestArchive() {
return ShrinkWrap.create(WebArchive.class, "test.war")
.addClasses(MyBean.class, MyService.class)
.addAsResource("META-INF/persistence.xml");
}
5. Logging
Symptom: Unclear error messages and exceptions.
Why it Happens: Logs are essential for deciphering what goes wrong during tests.
Solution: Configure better logging to capture detailed reports.
<logger category="org.jboss.as">
<level name="DEBUG"/>
</logger>
Increase the verbosity of logs by adjusting WildFly’s configuration settings to output more detailed information. Having detailed logs can drastically simplify the troubleshooting process.
Additional Tips for Troubleshooting
- Utilize the OpenShift Tools: OpenShift CLI (
oc
) can be an invaluable tool for debugging. Use it to get logs, shell into containers, and check the status of pods. - Handle Networking Carefully: OpenShift uses different networking models. Make sure you have appropriate routes and services set up.
- Debug Locally First: Ensure the tests run locally before running them in the container environment to isolate issues.
Resources
For further reading, consider checking the following resources:
- Arquillian Documentation
- OpenShift Documentation
Final Thoughts
Troubleshooting Arquillian tests on OpenShift’s WildFly may pose some challenges, but understanding the common issues and their solutions can empower developers to navigate these waters confidently. From connection problems to managing dependencies, each aspect requires careful consideration and testing.
With these tools and insights, you are well on your way to mastering your Arquillian tests and ensuring your applications run smoothly on OpenShift. Remember, every issue is an opportunity to learn and grow as a developer. Happy testing!