Common Pitfalls When Using Spring Data Cassandra 3
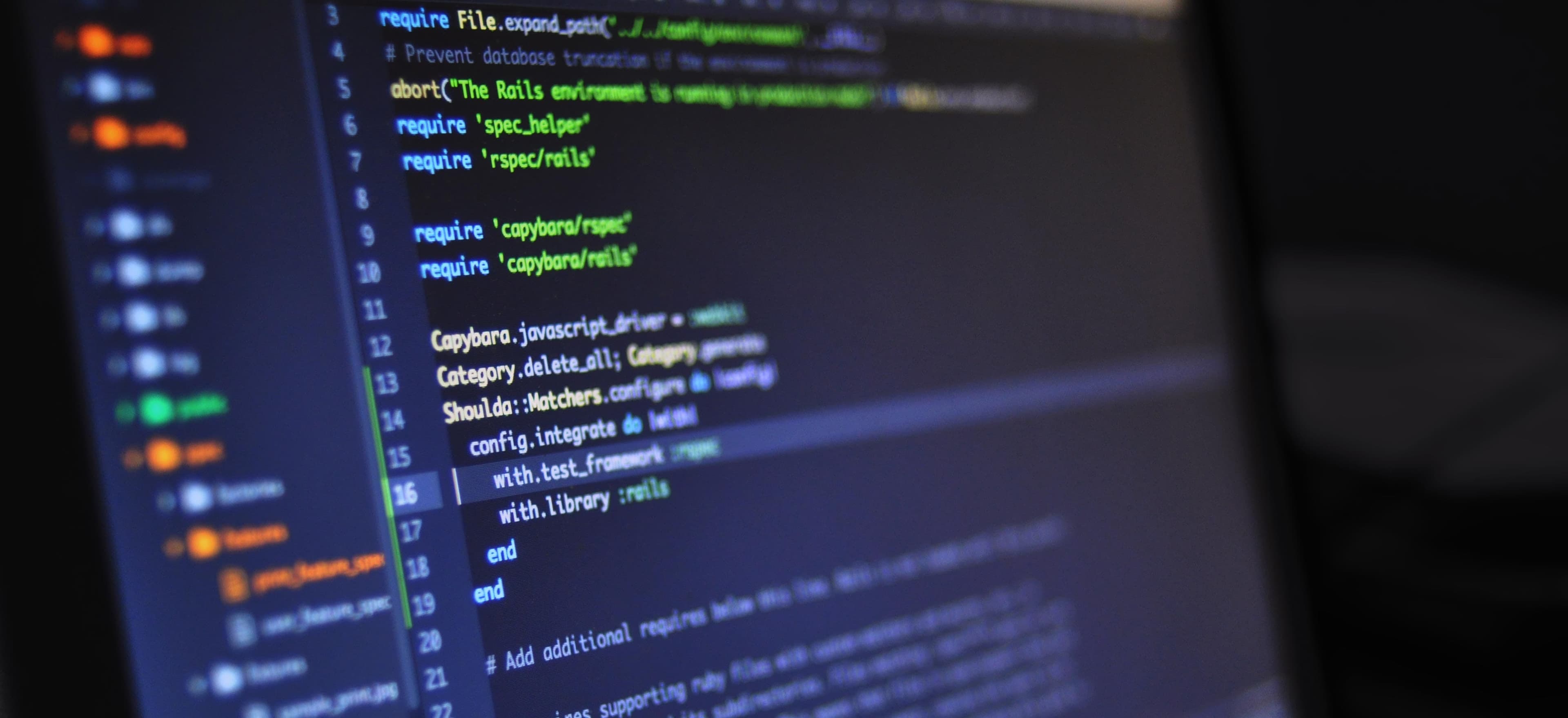
- Published on
Common Pitfalls When Using Spring Data Cassandra 3
Spring Data Cassandra is a powerful tool for developers looking to integrate Cassandra with their Spring applications. It offers a rich, high-level abstraction over Cassandra’s low-level APIs, but it is not without its challenges. This blog post will explore common pitfalls developers may face when using Spring Data Cassandra 3, as well as how to avoid or mitigate these issues.
Table of Contents
- Understanding Spring Data Cassandra
- Common Pitfalls
- Configuration Missteps
- Inefficient Query Patterns
- Lack of Exception Handling
- Ignoring Cassandra's Data Model
- Inadequate Testing
- Best Practices
- Conclusion
Understanding Spring Data Cassandra
Spring Data Cassandra provides a concise way to interact with Cassandra databases, leveraging Spring's own infrastructure, including dependency injection, transaction management, and more. This makes it easier to build robust applications without needing to write extensive boilerplate code.
To get started, you'd typically add the following dependencies:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-cassandra</artifactId>
</dependency>
This single line enables an entire suite of capabilities tailored for Cassandra.
Common Pitfalls
While Spring Data provides an easy path to using Cassandra, there are frequent pitfalls that many developers encounter. Understanding these can save you time and frustration.
Configuration Missteps
One common mistake is incorrect configuration of the application.properties
or application.yml
file. Setting improper contact points, ports, or timeouts can prevent your application from connecting to the Cassandra cluster.
Example Configuration:
spring:
data:
cassandra:
contact-points: localhost
port: 9042
keyspace-name: mykeyspace
Commentary:
Ensure that the contact-points
are accurately pointing to your Cassandra nodes. A misconfiguration here can make your application unable to reach your Cassandra cluster.
Inefficient Query Patterns
Cassandra is optimized for high throughput and low latency but without a proper understanding of its querying capabilities, one can easily fall into inefficient patterns.
Example of Inefficient Query:
public List<User> findUsersByAge(int age) {
return cassandraTemplate.select("SELECT * FROM users WHERE age = ?", User.class, age);
}
Commentary: Cassandra is not meant for queries involving inequality or aggregation such as ORDER BY or GROUP BY. Instead, design your tables for the specific access patterns you require.
Lack of Exception Handling
In a distributed environment like Cassandra, failures are inevitable. However, many developers forget to implement proper exception handling, leading to ungraceful application crashes.
Example of Handling Exceptions:
try {
cassandraTemplate.insert(user);
} catch (CassandraException e) {
// Logging the error for debugging purposes
logger.error("Error while inserting user: " + e.getMessage());
// Optionally, handle the fallback strategy
}
Commentary: Catch specific exceptions when performing operations that can fail, like network calls, and log these appropriately. This helps in diagnosing issues when they occur.
Ignoring Cassandra's Data Model
Cassandra's data model is unique and can be a hurdle if not properly understood. Users often model their data relationally, leading to poor performance. Familiarize yourself with concepts like partitioning and clustering.
Commentary: Instead of creating a new table for every query requirement, understand your access patterns, and design your models around that. For instance, if we need to store user activities, we can have:
CREATE TABLE user_activity (
user_id UUID,
activity_time TIMESTAMP,
activity_name TEXT,
PRIMARY KEY (user_id, activity_time)
);
Inadequate Testing
Testing is a critical aspect that is frequently overlooked. Unit tests for the data layer are important for ensuring data integrity. When not performed thoroughly, you may encounter data consistency issues.
Commentary: Utilize libraries that support in-memory testing of your Cassandra repository. Ensure that you cover a variety of cases, particularly edge cases such as timeouts or heavy load.
Best Practices
To alleviate the issues mentioned above, consider the following best practices:
-
Configuration Review: Regularly review your Cassandra configurations. Ensure that they comply with best practices and have been tunnel-visioned specifically for your use case.
-
Understand Your Data Access Patterns: Design your data model around the queries you will perform, not the other way around.
-
Implement Proper Exception Handling: Make exception handling a fundamental part of your code. Log exceptions and provide fallback strategies to improve user experience.
-
Perform Thorough Testing: Use integration testing to effectively test interactions with your Cassandra database.
-
Keep Up With Updates: Stay informed about updates to Spring Data Cassandra, as improvements and changes occur frequently. You can do this by checking out the official Spring Data documentation regularly.
A Final Look
Using Spring Data Cassandra 3 can be incredibly rewarding, yet it comes with its own set of challenges. By being aware of common pitfalls and following best practices, you can maximize the efficiency of your application and develop maintainable code.
Always remember to continuously evolve your practices as you learn from real-world applications. The community around Spring Data is vibrant, encouraging you to seek resources, ask questions, and share experiences.
For further reading on efficient Cassandra design practices, refer to Datastax's Cassandra Data Modeling Course. Happy coding!