Common Pitfalls in Custom DTO Deserialization and Validation
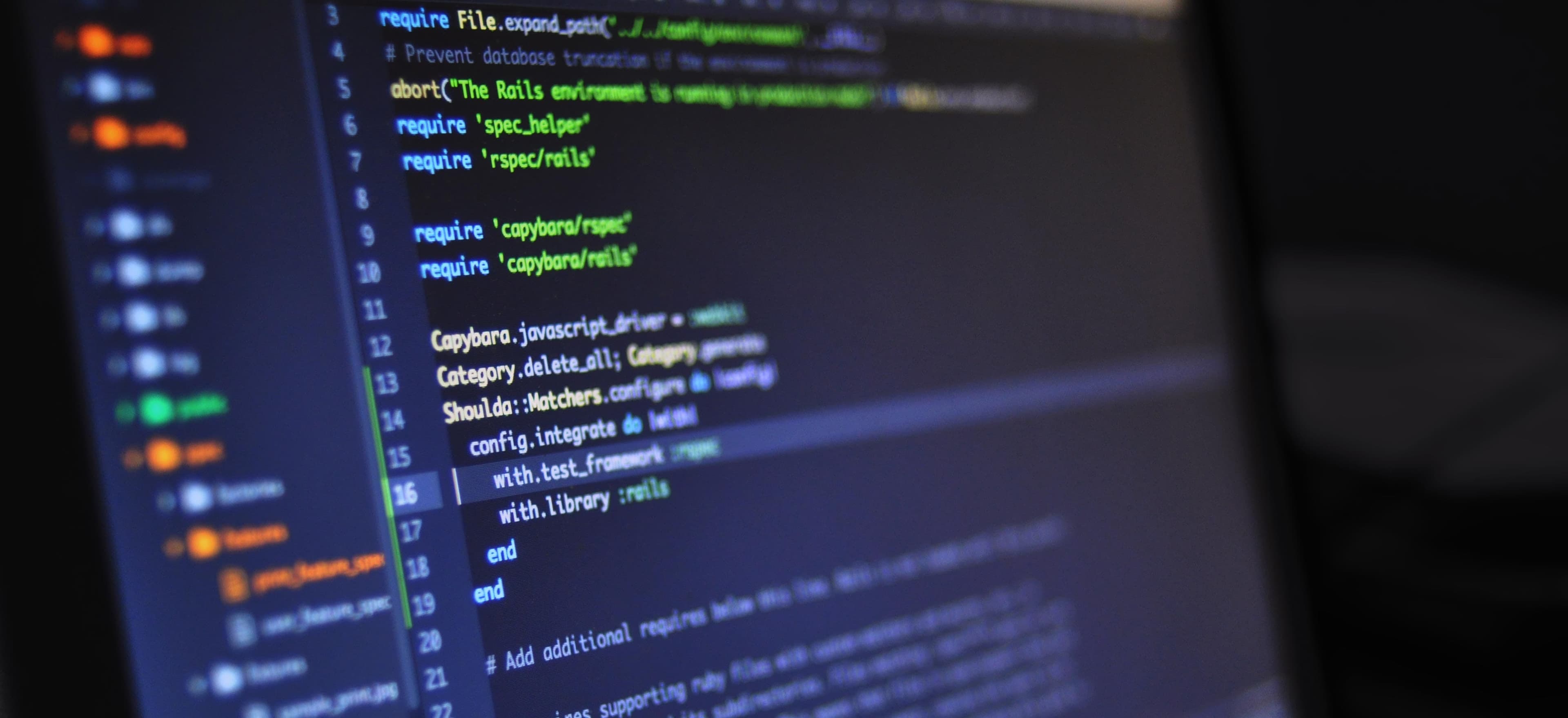
- Published on
Common Pitfalls in Custom DTO Deserialization and Validation
Data Transfer Objects (DTOs) are a staple in modern software development, especially when it comes to transferring data between systems. They encapsulate data and serve as a vehicle for data transfer. However, issues like improper deserialization and lack of validation can lead to critical failures in your application.
In this article, we will explore common pitfalls faced during custom DTO deserialization and validation, offer practical solutions, and back up our insights with code examples. This guide is designed for Java developers who want to enhance data integrity in their applications.
Table of Contents
- What are DTOs?
- The Importance of Proper Deserialization
- Common Pitfalls in DTO Deserialization
- Ignoring Field Type Mismatches
- Failing to Validate Input Data
- Overlooking Nested DTOs
- Implementing Effective Validation
- Code Examples
- Custom Deserialization
- Validation with Annotations
- Conclusion
What are DTOs?
Data Transfer Objects are simple objects that carry data between processes. DTOs are often used to encapsulate data for transportation, especially over the network when communicating with client-server architectures. They can carry data to and from services while minimizing the number of method calls.
Why Use DTOs?
- Simplicity: DTOs simplify the communication between different layers of your application.
- Data Encapsulation: They encapsulate all necessary attributes in a single object, making it easier to manage.
- Decoupling: Keeping your domain models separate from DTOs helps you decouple different layers of your application, making it easier to maintain.
The Importance of Proper Deserialization
Deserialization is the process of converting data from a format like JSON or XML back into a Java object. Proper deserialization is crucial because it ensures that the data you receive is correctly parsed into usable objects. Poor deserialization can introduce bugs and vulnerabilities into your application.
Common Pitfalls in DTO Deserialization
Ignoring Field Type Mismatches
One of the most common pitfalls is ignoring type mismatches between the JSON or XML data and the DTO fields. When you do not account for the expected data types, this can lead to runtime exceptions.
Example:
public class UserDTO {
private String name;
private int age;
// Getters and Setters
}
Suppose you receive a JSON response:
{
"name": "John Doe",
"age": "twenty"
}
If you fail to validate or convert the "age" field from String to int, your application will throw an exception at runtime.
Failing to Validate Input Data
Input validation is another critical area. If your DTOs lack comprehensive validation, your application could ingest invalid data, leading to unexpected behavior.
Best Practice:
Always validate user inputs. For instance, if age must be a positive integer, you should enforce this rule during deserialization.
Overlooking Nested DTOs
Complex applications often require nested DTOs. When these nested structures are not properly managed during deserialization, they can lead to NullPointerExceptions and other runtime errors.
Example:
public class OrderDTO {
private int orderId;
private UserDTO user;
// Getters and Setters
}
If the JSON represents an order without the associated user:
{
"orderId": 12345
}
You need to ensure that the user
field is properly initialized during deserialization.
Implementing Effective Validation
Validation helps ensure the data integrity of your application. Trivially validated data can cause security vulnerabilities, while starkly unvalidated data may cause unexpected behavior in your business logic.
Utilizing Annotations for Validation
Java's Bean Validation API (JSR 380) allows for convenient annotation-based validation. You can use annotations like @NotNull
, @Size
, and @Min
to enforce validation rules directly in your DTO.
Example:
import javax.validation.constraints.*;
public class UserDTO {
@NotBlank(message = "Name is required")
private String name;
@Min(value = 0, message = "Age must be positive")
private int age;
// Getters and Setters
}
Validating Nested DTOs
When you have nested DTOs, you can use the @Valid
annotation to ensure that validations are applied recursively.
public class OrderDTO {
private int orderId;
@Valid
private UserDTO user;
// Getters and Setters
}
Code Examples
Custom Deserialization
To illustrate our points, here’s an example of custom deserialization handling both type conversion and validation.
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.DeserializationFeature;
public class CustomDeserializer {
public static UserDTO deserializeUser(String json) throws Exception {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
UserDTO userDTO = objectMapper.readValue(json, UserDTO.class);
// Example validation
if (userDTO.getAge() < 0) {
throw new IllegalArgumentException("Age must be positive");
}
return userDTO;
}
}
In this example, we have configured the ObjectMapper with FAIL_ON_UNKNOWN_PROPERTIES
, which can help to avoid exceptions if the incoming data has additional fields unrelated to the DTO.
Validation with Annotations
Running validation can be accomplished easily with the help of the javax.validation
library:
import javax.validation.Validation;
import javax.validation.Validator;
import javax.validation.ValidatorFactory;
public class DTOValidator {
public static void validateUser(UserDTO userDTO) {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
Validator validator = factory.getValidator();
Set<ConstraintViolation<UserDTO>> violations = validator.validate(userDTO);
if (!violations.isEmpty()) {
StringBuilder sb = new StringBuilder("Validation errors occur");
for (ConstraintViolation<UserDTO> violation : violations) {
sb.append("\n").append(violation.getMessage());
}
throw new IllegalArgumentException(sb.toString());
}
}
}
In this example, after we deserialize the DTO, we immediately validate it. The validation logic is straightforward and readable, which maintains the simplicity of our DTO structure.
Closing Remarks
In conclusion, ensuring robust deserialization and validation of your DTOs is integral to maintaining the integrity of your applications. By addressing common pitfalls such as type mismatches, inadequate validation, and poorly managed nested objects, you can avoid critical runtime errors and potential vulnerabilities.
Always leverage available validation tools and libraries, such as Java annotations, to enhance data correctness. By following best practices in deserialization and validation, you create a resilient architecture capable of accurately handling data across multiple layers.
For more resources, feel free to check out:
Happy coding!