Mastering MongoDB Configuration in Spring Boot
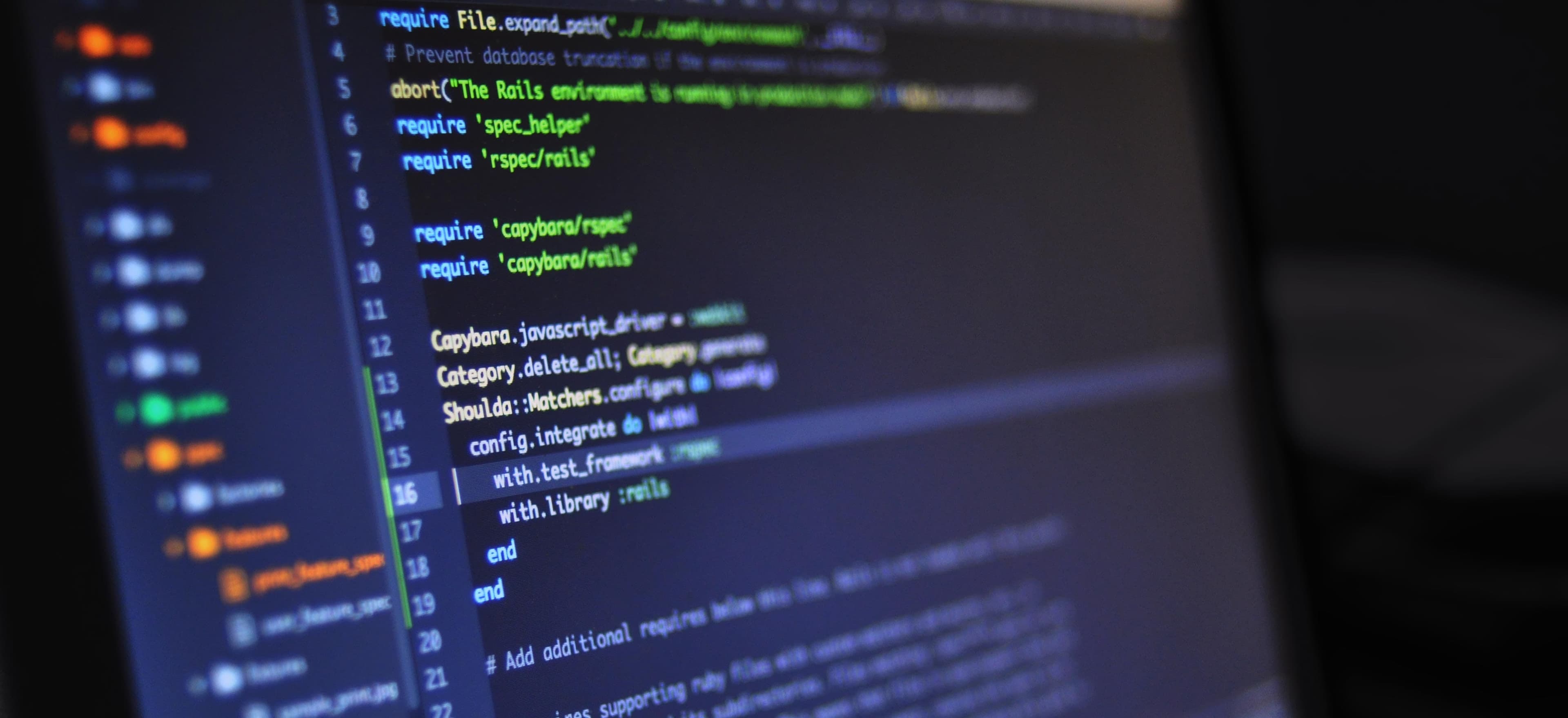
- Published on
Mastering MongoDB Configuration in Spring Boot
As the reliance on NoSQL databases like MongoDB increases, developers are gravitating towards frameworks that simplify integration and configuration processes. Spring Boot is an excellent choice due to its rapid application development capabilities. In this blog post, we’ll explore how to configure MongoDB in a Spring Boot application effectively.
Why Choose MongoDB?
MongoDB is a document-oriented NoSQL database that excels in situations where data can have complex structures. Here are several reasons why you might want to use MongoDB in your Spring Boot applications:
-
Flexible Schema: Unlike traditional relational databases, MongoDB allows for a variable schema design. This flexibility can speed up development, especially in agile environments.
-
Scalability: MongoDB is designed to scale out easily, allowing you to manage large amounts of data effortlessly.
-
Rich Queries: The database supports a rich query language that can handle complex queries and aggregations.
Setting Up MongoDB with Spring Boot
Step 1: Adding Dependencies
To use MongoDB in a Spring Boot application, you'll first need to add the necessary dependencies. In your pom.xml
, include:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
This dependency pulls in the necessary components for MongoDB support, including Spring Data MongoDB.
Step 2: Configuring MongoDB Properties
Next, you need to provide your MongoDB connection details. Open your application.properties
or application.yml
file and add the following configurations:
spring.data.mongodb.uri=mongodb://localhost:27017/mydatabase
In this configuration, replace localhost
with your MongoDB server address, 27017
with your MongoDB port (default is 27017), and mydatabase
with the name of your database.
Step 3: Creating a Domain Model
Now, let's create a domain model to represent the data stored in MongoDB. For instance, consider a simple Book
class:
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.mapping.Document;
@Document(collection = "books")
public class Book {
@Id
private String id;
private String title;
private String author;
private Double price;
// Constructors, Getters, and Setters
public Book(String title, String author, Double price) {
this.title = title;
this.author = author;
this.price = price;
}
// Getters and setters…
}
Here, the @Document
annotation specifies that this class is a MongoDB document and maps it to a collection called "books". The @Id
annotation indicates the primary key for the document.
Step 4: Creating a Repository Interface
With the domain model in place, the next step is to create a repository interface to interact with the MongoDB database. This is where Spring Data makes things remarkably simple.
import org.springframework.data.mongodb.repository.MongoRepository;
public interface BookRepository extends MongoRepository<Book, String> {
// Custom query methods can be defined here
List<Book> findByAuthor(String author);
}
Here, MongoRepository
provides basic CRUD operations and some additional query methods based on method names.
Step 5: Applying a Service Layer
Adding a service layer can help in managing business logic and ensure clean code practices.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class BookService {
@Autowired
private BookRepository bookRepository;
public List<Book> getAllBooks() {
return bookRepository.findAll();
}
public void addBook(Book book) {
bookRepository.save(book);
}
}
The @Service
annotation marks this class as a service component, allowing Spring to manage it. @Autowired
injects the BookRepository
, giving you access to all its methods.
Step 6: Creating a REST Controller
Finally, let's create a REST controller to allow for interaction with the service layer. This will expose endpoints to manage books.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/api/books")
public class BookController {
@Autowired
private BookService bookService;
@GetMapping
public List<Book> getAllBooks() {
return bookService.getAllBooks();
}
@PostMapping
public void addBook(@RequestBody Book book) {
bookService.addBook(book);
}
}
In this snippet, the controller defines an endpoint /api/books
to retrieve and add books to the MongoDB collection.
Step 7: Testing Your Application
To ensure that everything is working correctly, it’s essential to test your application. You can use tools like Postman to send HTTP requests to your API.
-
Retrieve all books: Send a GET request to http://localhost:8080/api/books.
-
Add a new book: Send a POST request to the same URL with the JSON body:
{ "title": "Effective Java", "author": "Joshua Bloch", "price": 45.0 }
The Last Word
In summary, configuring MongoDB in a Spring Boot application isn't just straightforward; it encapsulates a variety of built-in features that strengthen your application without unnecessary complexity. Throughout this guide, we've explored each crucial step—from dependencies and properties to creating repositories, services, and controllers.
Further Resources
By embracing these principles, you’re well on your way to mastering MongoDB configuration in your Spring Boot applications. Happy coding!