Mastering Exception Handling in Spring 3.2: Common Pitfalls
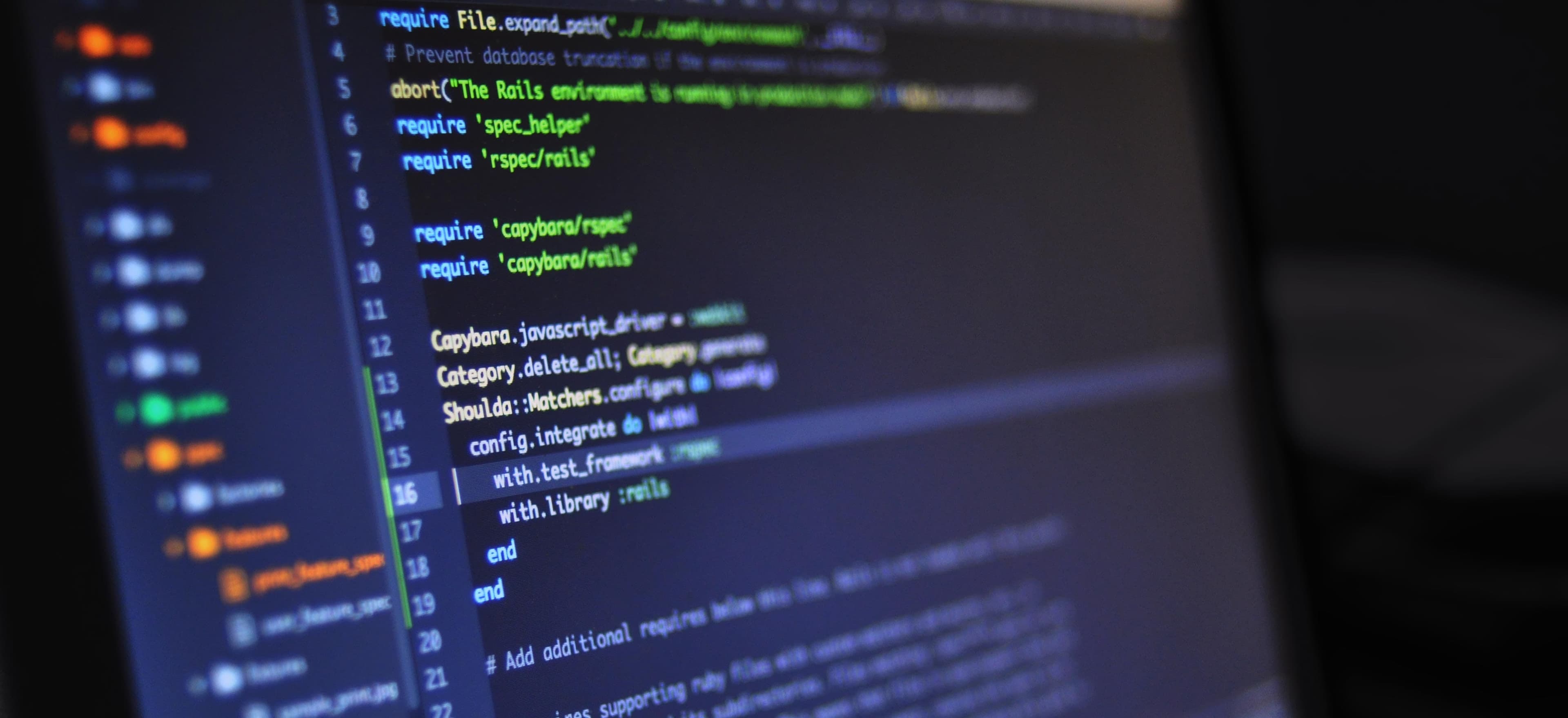
- Published on
Mastering Exception Handling in Spring 3.2: Common Pitfalls
Exception handling is a critical aspect of developing robust applications, especially in complex frameworks like Spring. Spring 3.2 introduced various features aimed at simplifying error handling. However, many developers still fall into common pitfalls. This blog post will explore these pitfalls, helping you avoid them and master exception handling in Spring 3.2.
Understanding the Basics of Exception Handling in Spring
Before diving into the common pitfalls, it is important to understand how exception handling works in Spring. In Spring, exceptions can be caught and handled using the following mechanisms:
- @ControllerAdvice: A specialization of the @Component annotation, allowing global handling of exceptions.
- @ExceptionHandler: This annotation specifies which exception to handle in a specific controller.
- ResponseEntityExceptionHandler: A base class for handling exceptions, providing more control over the HTTP response.
By correctly implementing these mechanisms, you can ensure that your application responds gracefully to errors.
Common Pitfalls in Exception Handling
1. Not Using @ControllerAdvice
One common mistake is neglecting the use of @ControllerAdvice. Without it, error handling is confined to the specific controller where an exception occurs. This can lead to duplicated error-handling logic across multiple controllers. Using @ControllerAdvice centralizes your error handling, making it easier to maintain. Here's how to use it:
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleAllExceptions(Exception ex) {
return new ResponseEntity<>("Error occurred: " + ex.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
In this code snippet, we define a global exception handler. The handleAllExceptions method will be triggered for any uncaught exception across the application. This reduces redundancy and enhances maintainability.
2. Ignoring Specific Exception Types
Another frequent pitfall is catching generic exceptions instead of specific ones. This practice leads to the loss of precise error information. For example, handling Exception
might obscure subclass exceptions like NullPointerException
or DataAccessException
, which could require different handling strategies.
@ExceptionHandler(NullPointerException.class)
public ResponseEntity<String> handleNullPointerException(NullPointerException ex) {
return new ResponseEntity<>("NullPointerException occurred: " + ex.getMessage(), HttpStatus.BAD_REQUEST);
}
By catching specific exceptions, you can provide more informative responses and implement tailored recovery strategies.
3. Improper Use of ResponseEntity
When returning a ResponseEntity, it is crucial to provide a meaningful status code and body. Many developers overlook this aspect, and simply returning HttpStatus.INTERNAL_SERVER_ERROR
for all exceptions can lead to confusion.
Instead, you should reflect the nature of the exception in the HTTP response.
@ExceptionHandler(DataAccessException.class)
public ResponseEntity<String> handleDataAccessException(DataAccessException ex) {
return new ResponseEntity<>("Database error: " + ex.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
This method clarifies to the client that the issue is database-related, rather than being a more generic server error.
4. Lack of Logging
Proper logging is often an afterthought in error handling. However, logging exceptions is crucial for diagnosing and fixing issues. If you don't log exceptions, you might never realize that an error occurred.
Make use of a logging framework like SLF4J with Logback or Log4j.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@ControllerAdvice
public class GlobalExceptionHandler {
private static final Logger logger = LoggerFactory.getLogger(GlobalExceptionHandler.class);
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleAllExceptions(Exception ex) {
logger.error("An error occurred: {}", ex.getMessage(), ex);
return new ResponseEntity<>("Error occurred: " + ex.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
With this implementation, not only do we respond to the client, but we also log the error, providing valuable information for debugging.
5. Failing to Handle Asynchronous Exceptions
In modern applications, asynchronous processing is prevalent. However, one pitfall is failing to account for exception handling in asynchronous processing. Exceptions in @Async methods might not propagate to the caller as expected, thus leading to suppressed errors.
For asynchronous methods, ensure to handle exceptions within the method itself or utilize a custom asynchronous exception handler:
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Service;
@Service
public class MyService {
@Async
public void asyncMethod() {
try {
// some code...
} catch (Exception ex) {
logger.error("Asynchronous error: {}", ex.getMessage(), ex);
// handle or rethrow as required
}
}
}
Here, exceptions are caught within the asynchronous method, allowing for appropriate handling or rethrowing.
6. Ignoring Client-Side Handling
Comparing server-side error handling to client-side handling is essential. Client applications should respond differently based on the type of error returned by the server. Failing to provide error codes or messages can hinder user experience.
For instance, a client application could take specific actions based on HTTP status codes. Here's an outline of common codes:
- 400 – Bad Request: client-side error
- 401 – Unauthorized: re-authentication required
- 500 – Internal Server Error: server-side issue
By providing precise status codes in your responses, clients can better handle and display error messages.
7. Not Testing Exception Scenarios
Finally, many developers neglect to write tests for exception scenarios. Not all errors are easy to reproduce, but it is still imperative to ensure your exception handling works as intended.
Utilize integration tests to simulate exceptions and verify that your application responds appropriately. Tools like Spring Boot Test can assist:
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
@RunWith(SpringJUnit4ClassRunner.class)
@SpringBootTest
@AutoConfigureMockMvc
public class MyControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void testHandleNullPointerException() throws Exception {
mockMvc.perform(get("/api/resource/null"))
.andExpect(status().isBadRequest())
.andExpect(content().string(containsString("NullPointerException occurred")));
}
}
This test simulates a call that would throw a NullPointerException, ensuring that your handler provides the expected response.
Closing Remarks
Mastering exception handling in Spring 3.2 requires awareness of common pitfalls and careful implementation of best practices. By utilizing @ControllerAdvice and @ExceptionHandler, distinguishing between exception types, logging exceptions, handling asynchronous problems, and writing thorough tests, you can create a robust error-handling strategy.
The efficient management of exceptions not only improves the maintainability of your code but also enhances the user experience by providing clear guidance when things go wrong. Remember, well-handled exceptions can turn potential failures into opportunities for better user engagement.
For further reading on Spring’s exception handling mechanisms, consider the official Spring documentation on exception handling.
By integrating these insights, you'll be well on your way to mastering exception handling in your Spring applications. Happy coding!