Mastering Javadoc: Avoiding Inherited Comment Confusion
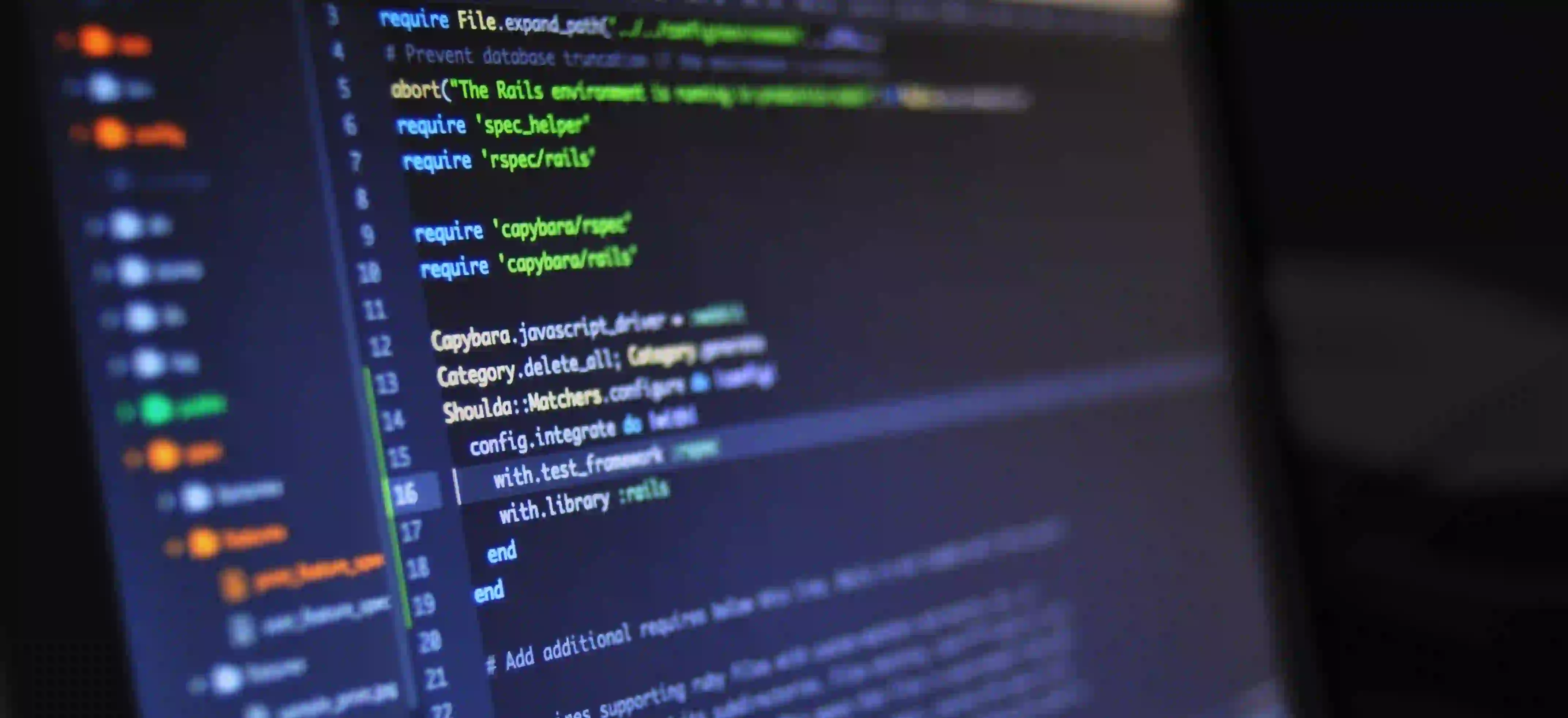
Mastering Javadoc: Avoiding Inherited Comment Confusion
When it comes to documenting Java code, mastering Javadoc is essential. Javadoc is not merely about comments; it is about creating a maintainable ecosystem of code that is easy to understand for developers, both current and future. One aspect of Javadoc that often leads to confusion is the inheritance of comments. This article provides an in-depth look at how to use Javadoc effectively while avoiding the pitfalls of inherited comments.
What is Javadoc?
Javadoc is a tool that comes bundled with the JDK (Java Development Kit). Its primary function is to create HTML documentation from the comments written in the Java source code. This utility simplifies the documentation process and provides a clear, structured way to present information about packages, classes, methods, and fields.
Using standardized comments allows Java developers to maintain clarity in their codebase, which is crucial in collaborative environments.
Understanding Inheritance in Java
Inheritance is one of the fundamental principles of object-oriented programming (OOP) in Java. It allows a class (subclass) to inherit fields and methods from another class (superclass), enabling code reuse and the creation of hierarchical classifications.
However, this feature can pose challenges when it comes to documentation. Specifically, when subclasses inherit Javadoc comments, confusion may arise regarding which class the comments pertain to.
Example of Inherited Comments
/**
* This is a base class representing a Vehicle.
*/
public class Vehicle {
/**
* A method that starts the vehicle.
*/
public void start() {
System.out.println("Vehicle starting...");
}
}
/**
* This is a subclass representing a Car.
* It inherits methods from Vehicle.
*/
public class Car extends Vehicle {
/**
* A method that starts the car.
* Inherits from Vehicle.
*/
@Override
public void start() {
// Calling the superclass start method
super.start();
System.out.println("Car starting with a roar!");
}
}
In the above code, note the comment in the Car
class that mentions the inheritance of the start
method from the Vehicle
class. While it does clarify that Car
uses the Vehicle
's method, this kind of comment can often lead to ambiguity. If someone is reading through the Javadoc generated for these classes, they might struggle to pinpoint which functionality belongs to which class.
Avoiding Inherited Comment Confusion
1. Write Original Comments for Each Class
One of the best strategies to avoid confusion is to write specific comments for each class, even for inherited methods. This practice ensures that each class has its own purpose clearly documented.
Example of Class-Specific Comments
/**
* This is a base class representing a Vehicle.
*/
public class Vehicle {
/**
* A method that starts the vehicle.
* Handles basic vehicle startup logic.
*/
public void start() {
System.out.println("Vehicle starting...");
}
}
/**
* This is a subclass representing a Car.
* It represents an abstraction of a specific type of vehicle.
*/
public class Car extends Vehicle {
/**
* A method that starts the car.
* Overrides the start method to add additional behavior exclusive to Car.
*/
@Override
public void start() {
super.start(); // Start the generic vehicle
System.out.println("Car starting with a roar!");
}
}
By rewriting the comments and specifying the role of the start
method in the subclass, we enhance the clarity of the documentation. Readers will know which part of the code relates specifically to Car
without misinterpretation.
2. Utilize @inheritDoc
Wisely
The @inheritDoc
tag in Javadoc allows subclasses to inherit the documentation from the superclass. While it can be convenient, it is essential to use this tag judiciously. If the inherited comment is not going to provide the necessary detail or will likely lead to confusion, it may be better to write new documentation.
/**
* This is a base class representing a Vehicle.
*/
public class Vehicle {
/**
* A method that starts the vehicle.
*/
public void start() {
System.out.println("Vehicle starting...");
}
}
/**
* This is a subclass representing a Car.
* It provides specific functionality for a car.
*/
public class Car extends Vehicle {
/**
* @inheritDoc
* This method starts the car and adds more functionality to it.
*/
@Override
public void start() {
super.start();
System.out.println("Car starting with a roar!");
}
}
In this example, you can see that the comment is still there for clarity, but the @inheritDoc
is handy as it can help minimize redundancy. Just be cautious—if the superclass's comment is not comprehensive enough for the subclass, consider writing an entirely new comment.
3. Use Consistent Terminology
Make sure to maintain consistent terminology throughout documentation. If you refer to an action as "initialize" in one place, do not call it "start" somewhere else. This consistency will help eliminate confusion when reading through the documentation.
4. Document Behavior Changes
Whenever a subclass alters the behavior of a method, document the change explicitly in the methods' comments. This change helps other developers recognize that although the method is inherited, its implementation differs.
/**
* This is a subclass representing a Car.
*/
public class Car extends Vehicle {
/**
* Starts the car, overriding the start method from Vehicle.
* <p>
* In addition to the behaviors defined in Vehicle, this
* method also prints a message specific to cars.
* </p>
*/
@Override
public void start() {
super.start();
System.out.println("Car starting with a roar!");
}
}
These additional details can vastly improve comprehension and ensure the purpose of every override is clear.
A Final Look
Mastering Javadoc is crucial for developers who want to create easily maintainable code. By being mindful of inherited comments, using @inheritDoc
wisely, writing specific comments, and maintaining terminology consistency, you can significantly improve the clarity and usefulness of your Javadoc.
Proper documentation fosters better collaboration among developers and contributes to building a robust software architecture.
For further reading on effective Java documentation practices, you can take a look at the Oracle Javadoc Guide and the Java Conventions.
Key Takeaways
- Write specific comments for each class.
- Use
@inheritDoc
cautiously. - Maintain consistent terminology.
- Document behavior changes explicitly.
By following these guidelines, you put yourself on the right path toward mastering Javadoc while avoiding common pitfalls related to inherited comments. Happy coding!