Common Hibernate Identifier Issues and How to Fix Them
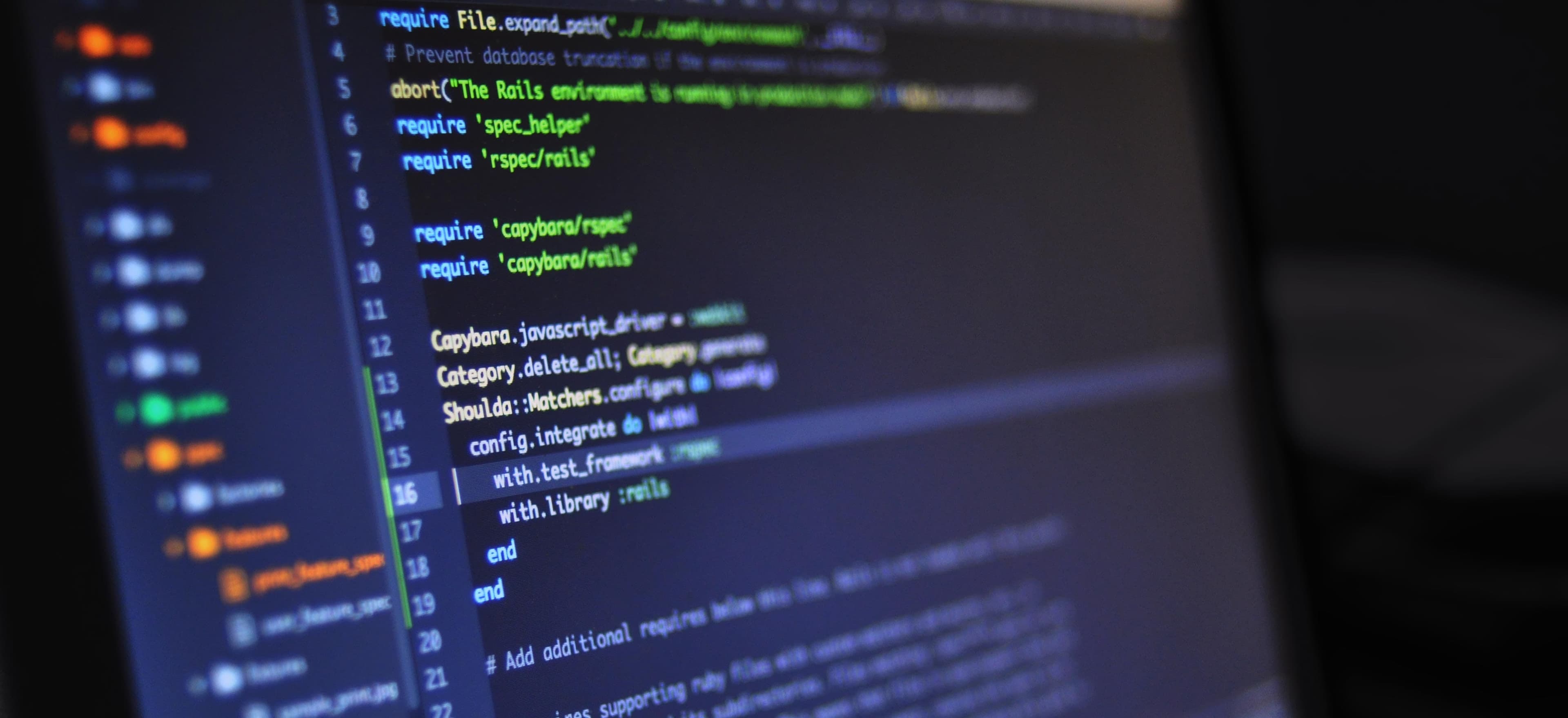
- Published on
Common Hibernate Identifier Issues and How to Fix Them
Hibernate is a powerful Object-Relational Mapping (ORM) framework that facilitates database interactions in Java applications. However, despite its comprehensive features, developers often encounter common issues related to identifiers, especially when dealing with primary keys and entity relationships. In this post, we will delve into these issues, explain their causes, and provide straightforward solutions.
Understanding Identifiers in Hibernate
Before we dive into specific issues, let's clarify what identifiers are in the context of Hibernate. An identifier is a unique key that is used to identify entities. Typically, the primary key in your database table serves as the identifier for Hibernate entities.
Common Issues with Identifiers
-
Duplicate Identifier Values
- Description: This occurs when two entities share the same identifier, leading to database integrity issues.
- Cause: This often results from improper configuration of the identifier generation strategy.
Solution: Ensure that you are using a proper identifier generation strategy. For example, use
@GeneratedValue
along with a strategy that fits your use case:@Entity public class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String name; // ... other fields }
Why: The
GenerationType.IDENTITY
strategy relies on the database to generate unique identifiers, which reduces the likelihood of duplicates. -
Null Identifiers
- Description: When an entity is persisted without an identifier, Hibernate raises an exception.
- Cause: This often results from forgetting to set the identifier before saving the entity.
Solution: Always ensure an identifier is set before persisting. If using a generated identifier strategy, simply clear the identifier field:
User user = new User(); user.setName("John Doe"); session.save(user);
Why: By not setting an identifier, we rely on Hibernate to generate it for us, preventing null exceptions.
-
Incorrect Identifier Type
- Description: Mismatched data types between the entity field and database key can lead to runtime errors.
- Cause: This often happens when you're using a wrapper class like
Integer
in your entity while the database column is defined asBIGINT
.
Solution: Align the data types in your Java class with those defined in the database schema:
@Entity public class Product { @Id @GeneratedValue(strategy = GenerationType.AUTO) private Long id; // Ensure this matches with the DB type private String productName; // ... other fields }
Why: Ensuring type consistency prevents runtime errors during database operations.
-
Entity State Confusion
- Description: Using the same entity instance in multiple sessions may lead to confusion over its state—transient, persistent, or detached.
- Cause: It often occurs when developers retrieve an entity, alter its state, and fail to understand how Hibernate tracks these states.
Solution: Always use the correct pattern for sharing entities between sessions. For example, use the session merge method to synchronize changes:
User user = session.get(User.class, userId); user.setName("New Name"); session.merge(user); // Synchronizes the detached instance
Why: Using
merge
allows for properly managing entity states, preventing unexpected behavior. -
Sharing Identifiers Across Sessions
- Description: This confusion arises when you retrieve an entity in one session, detach it, and attempt to save or update it in another session.
- Cause: This usually happens when developers are not aware of how Hibernate manages identity across different sessions.
Solution: Whenever you need to work with an entity across sessions, always consider the entity's current state:
// In session 1 User user = session.get(User.class, userId); session.close(); // Detaching the user // In session 2 User user2 = (User) session.merge(user); // Merging instead of saving directly user2.setName("Updated Name"); session.save(user2);
Why: This ensures that Hibernate understands the existing state and can manage it properly when persisting changes.
-
Improper Composite Key Mapping
- Description: When you need a composite key (a key made from more than one column), misconfiguring it leads to issues like loss of key integrity.
- Cause: Developers may mistakenly define composite keys without using
@EmbeddedId
or@IdClass
.
Solution: Use the correct annotations and create a Dedicated ID Class.
@Embeddable public class UserId implements Serializable { private Long userId; private String domain; // Implement equals and hashCode } @Entity public class User { @EmbeddedId private UserId id; // other fields }
Why: This setup maintains integrity for composite keys and ensures proper management of entities.
Additional Resources
For more insights on Hibernate and identifier handling, consider visiting these resources:
- Hibernate Official Documentation
- Baeldung's Guide to Spring Data JPA
- Java Persistence API (JPA) Specifications
The Last Word
Identifying and solving issues related to identifiers in Hibernate is crucial for the stability and reliability of your Java application. Understanding the causes and implementing the recommended solutions will help mitigate these common pitfalls. With proper configurations and best practices, you can harness the full power of Hibernate without being bogged down by identifier issues.
Navigating these challenges may seem daunting at first; however, with consistent practice and attention to detail, you can become proficient in using Hibernate effectively in your projects.
Checkout our other articles