Navigating I/O vs NIO: Managing Interruptions and Timeouts
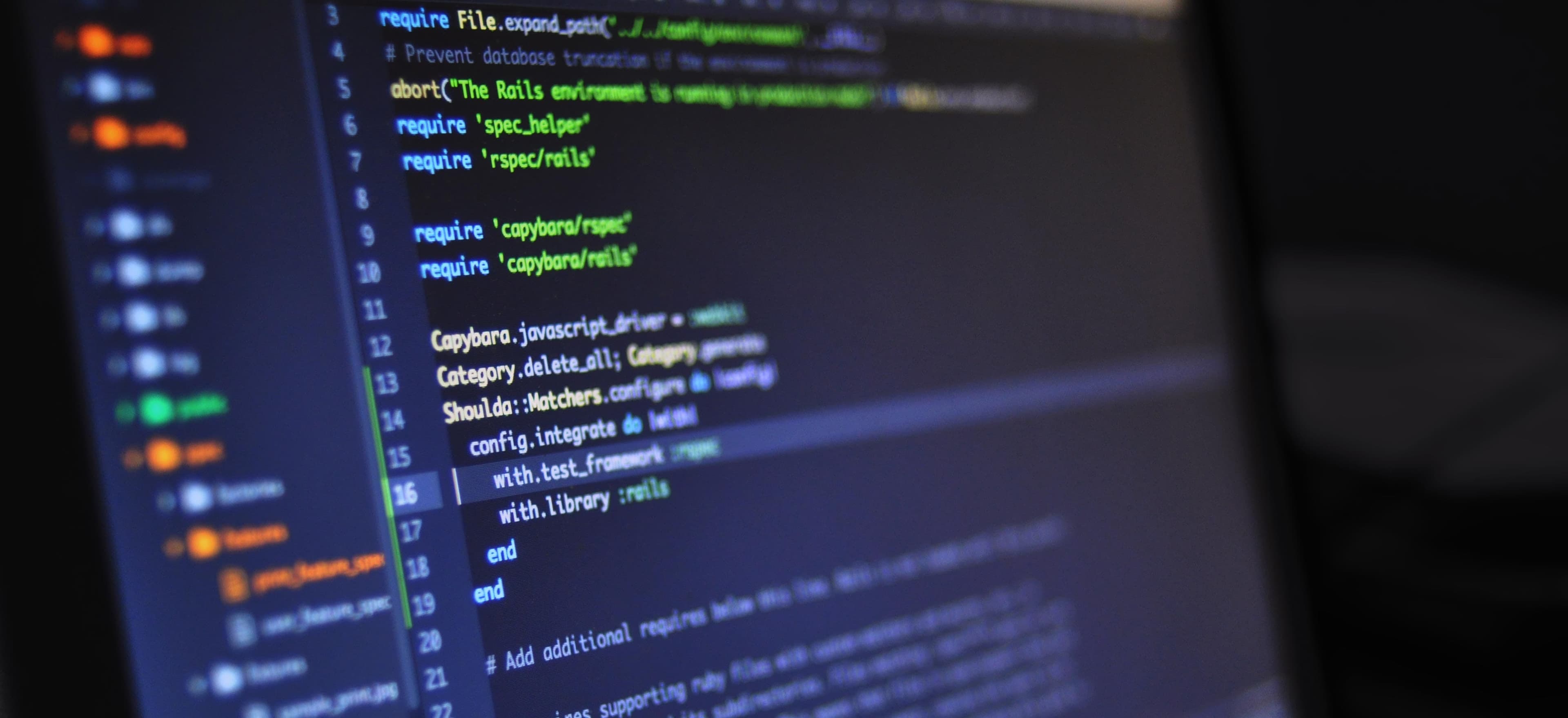
- Published on
Navigating I/O vs NIO: Managing Interruptions and Timeouts
In the world of Java, input/output (I/O) operations are crucial for interaction with users and systems. However, handling these I/O operations can be challenging, especially when dealing with interruptions and timeouts. This blog post will explore the concepts of traditional I/O versus Non-blocking I/O (NIO) in Java, emphasizing how to effectively manage interruptions and timeouts.
Understanding I/O versus NIO
Traditional I/O
Java's traditional I/O API has been a staple for many years. It is straightforward and easy to use, making it suitable for many applications. However, it has inherent limitations when it comes to performance, particularly in multi-threaded environments.
Key Characteristics of I/O:
- Blocking Calls: I/O operations block the executing thread until completion, which can lead to unresponsive applications.
- Lower Throughput: Handling multiple simultaneous connections can be cumbersome, as each connection often requires a dedicated thread.
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class TraditionalIOExample {
public static void main(String[] args) {
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
}
}
}
In this example, the method blocks until the file has been fully read.
Non-blocking I/O (NIO)
NIO, introduced in Java 1.4, provides a more efficient way to handle I/O. It allows for non-blocking operations, enabling applications to manage multiple connections without relying heavily on threads.
Key Characteristics of NIO:
- Non-blocking Calls: Threads can continue executing while waiting for I/O operations to complete.
- Higher Throughput: Better suited for high-load applications as it can handle multiple connections with fewer resources.
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.AsynchronousSocketChannel;
import java.nio.channels.AsynchronousChannelGroup;
import java.util.concurrent.Future;
public class NIOExample {
public static void main(String[] args) {
try (AsynchronousChannelGroup group = AsynchronousChannelGroup.withCachedThreadPool()) {
AsynchronousSocketChannel socketChannel = AsynchronousSocketChannel.open(group);
socketChannel.connect(new InetSocketAddress("localhost", 8080)).get();
ByteBuffer buffer = ByteBuffer.allocate(1024);
Future<Integer> result = socketChannel.read(buffer);
while (!result.isDone()) {
// Application can do other work here while waiting for the I/O
}
System.out.println("Read bytes: " + result.get());
} catch (IOException | InterruptedException | ExecutionException e) {
System.err.println("Error: " + e.getMessage());
}
}
}
In this example, the thread is free to perform other tasks while reading data from the socket.
Managing Interruptions
I/O Interruptions
Interruptions in I/O operations often occur when a thread is blocked. This can significantly affect user experience. In traditional I/O, if a thread is blocked while reading a file, other operations cannot proceed.
To handle interruptions in traditional I/O, it is vital to implement checks that gracefully handle such scenarios.
Here is a simple strategy using flags for interruption handling:
public class TraditionalIOWithInterrupt {
private static volatile boolean interrupted = false;
public static void main(String[] args) {
Thread thread = new Thread(() -> {
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) {
String line;
while (!interrupted && (line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
if (!interrupted) {
System.err.println("Error reading file: " + e.getMessage());
}
}
});
thread.start();
// Simulate interruption after some time
try {
Thread.sleep(1000); // Let it read for a while
interrupted = true; // Set the flag to true to stop reading
} catch (InterruptedException e) {
System.err.println("Interrupted: " + e.getMessage());
}
// Wait for the thread to terminate
try {
thread.join();
} catch (InterruptedException e) {
System.err.println("Thread join interrupted: " + e.getMessage());
}
}
}
This implementation allows the reading thread to check for interruptions without leaving resources hanging.
NIO Interruptions
With NIO, managing interruptions is more fluid. Asynchronous operations naturally lend themselves to a responsive design. You can use a combination of futures and callbacks to handle interruptions smoothly.
In NIO, handling interruptions can also be achieved via Futures:
import java.nio.channels.AsynchronousSocketChannel;
public class NIOWithInterrupt {
public static void main(String[] args) {
AsynchronousSocketChannel channel = AsynchronousSocketChannel.open();
Future<Integer> op = channel.read(buffer);
// Wait for completion or timeout
if (op.isDone()) {
// Process completion
} else {
// You can interrupt the operation gracefully
channel.close(); // Interrupts the read operation
System.err.println("Read operation interrupted.");
}
}
}
Here, closing the channel serves as an effective interruption strategy, as it terminates blocking reads or writes.
Timeout Handling
While handling I/O operations, timeouts are another crucial aspect. Timeouts prevent operations from hanging indefinitely.
I/O Timeout Handling
In traditional I/O, timeouts must be managed manually. One can implement background threads or checks at intervals to see if an operation is taking too long.
public class TraditionalIOWithTimeout {
public static void main(String[] args) {
Thread readThread = new Thread(() -> {
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
System.err.println("Error: " + e.getMessage());
}
});
readThread.start();
// Implement timeout logic
try {
readThread.join(5000); // wait for 5 seconds
if (readThread.isAlive()) {
readThread.interrupt(); // Terminate thread if still running
System.err.println("Read operation timed out.");
}
} catch (InterruptedException e) {
System.err.println("Thread join interrupted: " + e.getMessage());
}
}
}
This method allows you to set a maximum wait time for the operation, ensuring your application remains responsive.
NIO Timeout Handling
NIO provides built-in timeout management via the use of Selector
. You can set a timeout for selecting keys, allowing you to implement responsive, time-aware processing.
import java.nio.channels.Selector;
import java.nio.channels.SocketChannel;
public class NIOWithTimeout {
public static void main(String[] args) {
Selector selector = Selector.open();
// Setting up channels...
while (true) {
int readyChannels = selector.select(5000); // 5 seconds timeout
if (readyChannels == 0) {
System.out.println("No channels ready. Timeout occurred, perform other tasks...");
continue;
}
// Handle ready channels...
}
}
}
In this setup, if no channels are ready within the specified timeout, the application can handle other tasks or processes, enhancing its efficiency.
Closing the Chapter
Java's traditional I/O and NIO both serve distinct purposes, each with its strengths and weaknesses. Understanding how to handle interruptions and timeouts is crucial to creating responsive applications. Traditional I/O may be simpler for small applications, but as scalability and performance become critical, NIO offers significant advantages.
To delve deeper into the differences between Java I/O and NIO, refer to Java I/O vs NIO.
In conclusion, pick the appropriate tool based on your application needs, and ensure you consider error, interruption, and timeout management for a more robust Java program. With the right approach, you can harness the full potential of Java's I/O capabilities.
Happy coding!
Checkout our other articles