Common Spring Security Misconfigurations and How to Fix Them
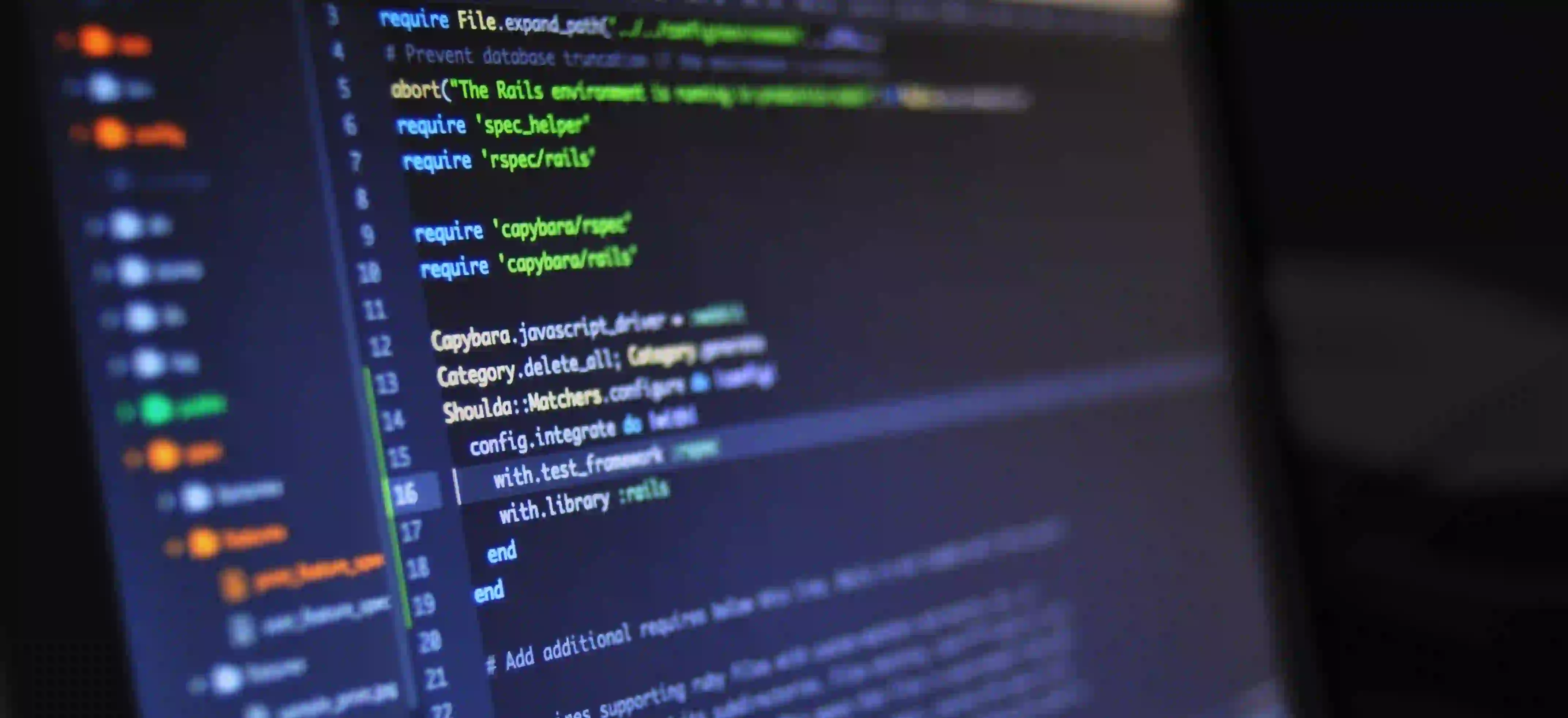
Common Spring Security Misconfigurations and How to Fix Them
Spring Security is a powerful and customizable authentication and access control framework that is widely used in Java applications. However, its config complexities can lead to common misconfigurations, making applications vulnerable to attacks. In this post, we'll explore some common Spring Security misconfigurations and provide solutions to mitigate them.
Table of Contents
- Introduction to Spring Security
- Common Misconfigurations
- Weak Password Policies
- Incorrectly Configured CSRF Protection
- Unsecured Endpoints
- Missing Logout Functionality
- Best Practices for Configuration
- Conclusion
Setting the Stage to Spring Security
Spring Security is not just about authentication; it encompasses various aspects of securing your applications. It offers comprehensive security services for Java EE-based enterprise software applications. Configuring it correctly is crucial for protecting sensitive data.
The Importance of Proper Configuration
Misconfigurations can lead to a range of vulnerabilities, including unauthorized access, data leaks, and diminished application integrity. Thus, understanding common pitfalls is imperative for any Software Engineer.
Common Misconfigurations
1. Weak Password Policies
Issue
A common misconfiguration in Spring Security is the failure to enforce strong password policies. Default settings might allow simple, weak passwords.
Solution
You can introduce a custom password validator to ensure passwords meet your security requirements.
Here’s a sample implementation:
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.stereotype.Component;
@Component
public class CustomPasswordEncoder implements PasswordEncoder {
@Override
public String encode(CharSequence rawPassword) {
// Use a strong hashing algorithm
return BCrypt.hashpw(rawPassword.toString(), BCrypt.gensalt());
}
@Override
public boolean matches(CharSequence rawPassword, String encodedPassword) {
// Password match verification
return BCrypt.checkpw(rawPassword.toString(), encodedPassword);
}
}
Why This Matters
Using hashed passwords prevents attackers from being able to read the plaintext passwords, providing a layer of security for user data.
2. Incorrectly Configured CSRF Protection
Issue
Cross-Site Request Forgery (CSRF) is an attack that tricks the user into submitting a malicious request. If CSRF protection is disabled or misconfigured, your application may become susceptible.
Solution
Ensure that CSRF protection is enabled in your Spring Security configuration:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf()
.csrfTokenRepository(CookieCsrfTokenRepository.withHttpOnlyFalse())
.and()
.authorizeRequests()
.anyRequest().authenticated();
}
Why This Matters
Enabling CSRF protection makes it difficult for attackers to execute unauthorized commands on behalf of authenticated users by requiring a unique token for each request.
3. Unsecured Endpoints
Issue
Often, developers forget to secure certain endpoints, leaving them accessible to everyone. Public endpoints can easily be targeted by attackers.
Solution
Specify authorization on your endpoints:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll() // Public endpoints
.antMatchers("/admin/**").hasRole("ADMIN") // Secured admin endpoints
.anyRequest().authenticated(); // Protect other requests
}
Why This Matters
Controlling access to endpoints prevents unauthorized users from accessing sensitive information. In a real-world scenario, for instance, admin resources should never be available to general users.
4. Missing Logout Functionality
Issue
It's easy to overlook proper logout functionality. If not implemented, users may remain authenticated even after they think they have "logged out."
Solution
Add proper logout configuration to your HttpSecurity
settings:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.logout()
.logoutUrl("/logout")
.logoutSuccessUrl("/login?logout")
.invalidateHttpSession(true);
}
Why This Matters
Implementing logout functionality helps invalidate the session securely, ensuring that the user cannot perform actions post-logout. It's critical for maintaining session integrity.
Best Practices for Configuration
-
Keep Dependencies Updated: Use the latest stable version of Spring Security to leverage built-in security updates.
-
Use HTTPS: Always serve your application over HTTPS to prevent man-in-the-middle attacks.
-
Configure Secured Headers: Use HTTP security headers to mitigate risks (like X-Content-Type-Options, X-Frame-Options, etc.).
-
Limit Session Duration: Implement session management to control user session lifetimes effectively.
-
Regular Security Audits: Conduct regular code reviews and penetration testing to identify and fix potential vulnerabilities.
Key Takeaways
Configuring Spring Security correctly is essential for building secure Java applications. This post highlighted some common misconfigurations and provided actionable solutions.
By following best practices, you can safeguard your applications against vulnerabilities and ensure a robust security posture.
For further reading, check out the Spring Security reference documentation or explore additional security features through the Spring Boot Security Guide.
Remember, security is an ongoing process, not a set-it-and-forget-it approach. Regular updates and audits are key to maintaining a secure application ecosystem.