Common Maven Cargo Plugin Issues and How to Fix Them
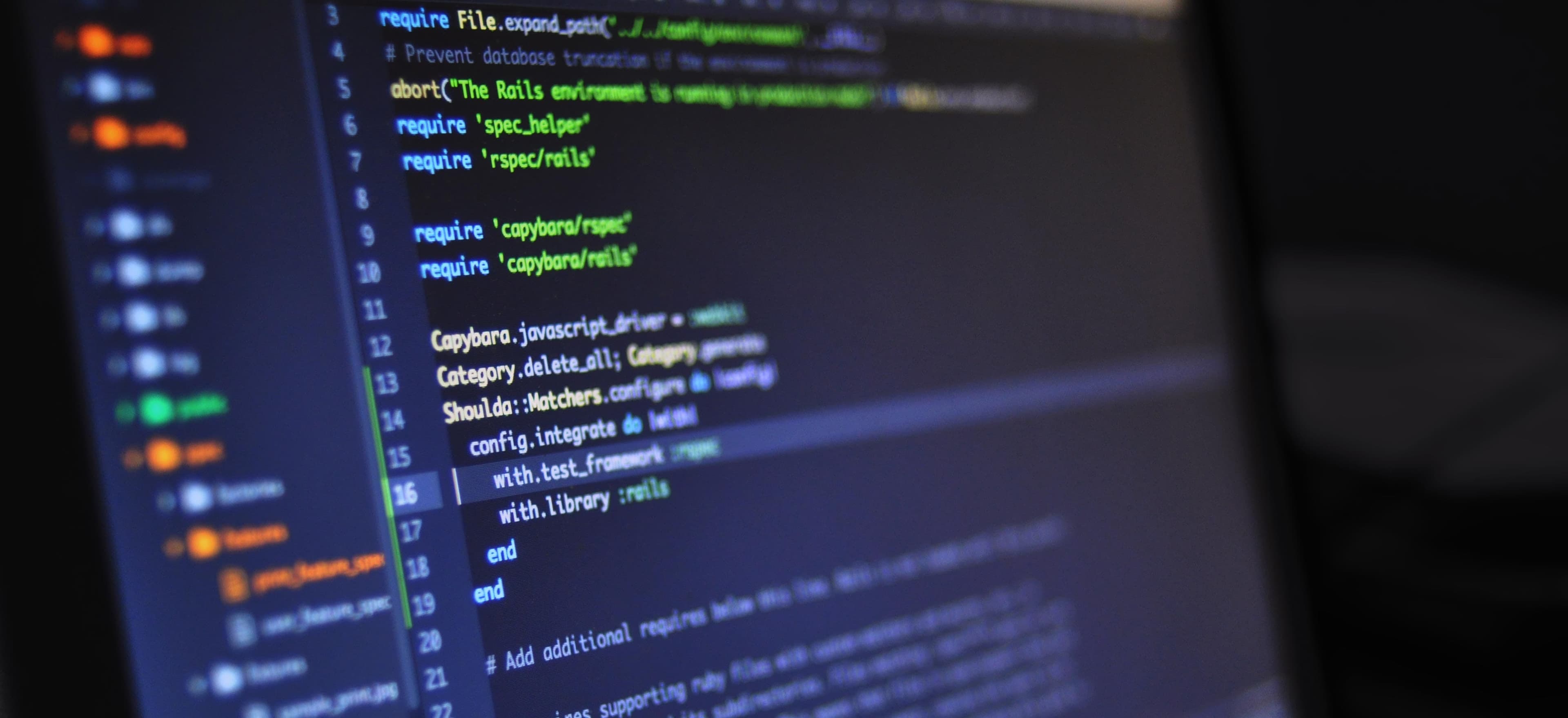
- Published on
Common Maven Cargo Plugin Issues and How to Fix Them
The Maven Cargo Plugin is a powerful tool that helps developers deploy their Java applications to various containers easily. Although it simplifies deployment, you may encounter some challenges. In this blog post, we will dive deep into the common issues associated with the Maven Cargo Plugin and, more importantly, how to fix them.
What is Maven Cargo Plugin?
The Maven Cargo Plugin enables you to deploy Java web applications to various application servers like Apache Tomcat, JBoss, GlassFish, etc. It allows you to manage your deployment lifecycle through Maven build commands. For a comprehensive overview, visit Cargo’s official documentation.
Setting Up Your Maven Cargo Plugin
Before we address common issues, let’s quickly review how to set up the Maven Cargo Plugin in your pom.xml
.
<build>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>cargo-maven2-plugin</artifactId>
<version>1.9.5</version>
<configuration>
<container>
<containerId>jboss70x</containerId>
</container>
<deployer>
<archive>
<groupId>com.example</groupId>
<artifactId>myapp</artifactId>
<version>1.0-SNAPSHOT</version>
</archive>
</deployer>
</configuration>
</plugin>
</plugins>
</build>
Why Use the Maven Cargo Plugin?
- Consistency: It offers a consistent deployment mechanism for different environments.
- Integration: It fits seamlessly into your existing Maven build process.
- Flexibility: Supports various configuration options for different containers.
Common Issues and Their Solutions
Issue 1: Unable to find the specified container
Problem:
If you run the deploy command and receive an error like:
Unable to find container with id: jboss70x
Fix:
-
Check if the container exists: Make sure that you've specified a valid container ID in your
pom.xml
. -
Update the container ID: If you're using an older version, you might want to switch to a more recent one. You can find the available container IDs here.
Example:
<containerId>jboss7x</containerId>
Issue 2: Deployment fails due to port conflicts
Problem:
Deployment fails because the server cannot start due to port conflicts. You might see an error like:
Port 8080 is already in use
Fix:
-
Change Port Configurations: You can specify alternative ports by configuring the container configuration in the
pom.xml
.Example:
<configuration> <container> <containerId>tomcat7x</containerId> <configuration> <configuration> <property> <name>cargo.container.port</name> <value>8081</value> </property> </configuration> </configuration> </container> </configuration>
-
Kill the Process: If you are aware of any other application using the port, terminate that process.
Issue 3: Missing Dependencies
Problem:
You find that your application does not deploy correctly because of missing dependencies like:
NoClassDefFoundError: org/springframework/...
Fix:
-
Add Missing Dependencies: Ensure that your
pom.xml
includes all necessary dependencies for your project.Example:
<dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>5.3.10</version> </dependency>
-
Run Maven Clean: This clears the
target
directory and helps ensure that dependencies are not stale.Command:
mvn clean install
Issue 4: Container Not Starting
Problem:
The container starts but crashes immediately after. You may see logs indicating the issue.
Fix:
-
Check Logs: The most efficient way to find out what went wrong is by checking the logs generated by the container. You can configure your plugin to specify a log file:
<configuration> <logFile>${project.build.directory}/container.log</logFile> </configuration>
-
Configuration Errors: Look for incorrect configurations in your application, such as malformed XML in
web.xml
or missing resource files.
Issue 5: Deployment Delays
Problem:
Sometimes, deploying with Maven Vault can take longer than expected.
Fix:
-
Increase Deployment Timeout: You can extend the deployment timeout setting, allowing more time for the deployment process to complete.
Example:
<configuration> <deployTimeout>600</deployTimeout> <!-- 600 seconds --> </configuration>
-
Optimize Application Start: Check the startup processes of your application. For instance, reducing the startup time of services can significantly improve deployment performance.
Issue 6: Intermittent Network Issues During Remote Deployments
Problem:
When deploying applications to remote servers, you may experience intermittent failures due to network issues.
Fix:
-
Network Stability: Ensure that your network connection to the remote host is stable. This may involve checking your local network or the server’s network health.
-
Increase Connection Timeout: To allow more time for remote deployments, increase the connection timeout in your configuration.
Example:
<configuration> <configuration> <property> <name>cargo.connection.timeout</name> <value>120</value> <!-- timeout in seconds --> </property> </configuration> </configuration>
Best Practices for Using Maven Cargo Plugin
-
Use the Right Version: Keep your dependencies and the Maven Cargo Plugin up to date to benefit from fixes and enhancements.
-
Automate with CI/CD: Integrate your deployment steps into a CI/CD pipeline to minimize human errors and streamline your workflow.
-
Testing: Always test your app locally before deploying to a remote server. Use
mvn install
to validate your build. -
Rollback Strategy: Have a rollback strategy in case of failed deployments, ensuring minimal downtime for your application.
Final Thoughts
Deploying Java applications using the Maven Cargo Plugin can significantly streamline your workflow. However, as with any tool, you may encounter challenges. This guide provided insights into common issues and their fixes, enabling you to deploy your applications effectively.
For more advanced configurations and options, refer to the official Cargo documentation. By remaining proactive and informed about potential pitfalls, you can ensure that your deployments are smooth and efficient.
Feel free to share your experiences with the Maven Cargo Plugin or any other challenges you have faced in the comments below! Happy deploying!