Overcoming Common Pitfalls in Kafka REST Proxy Setup
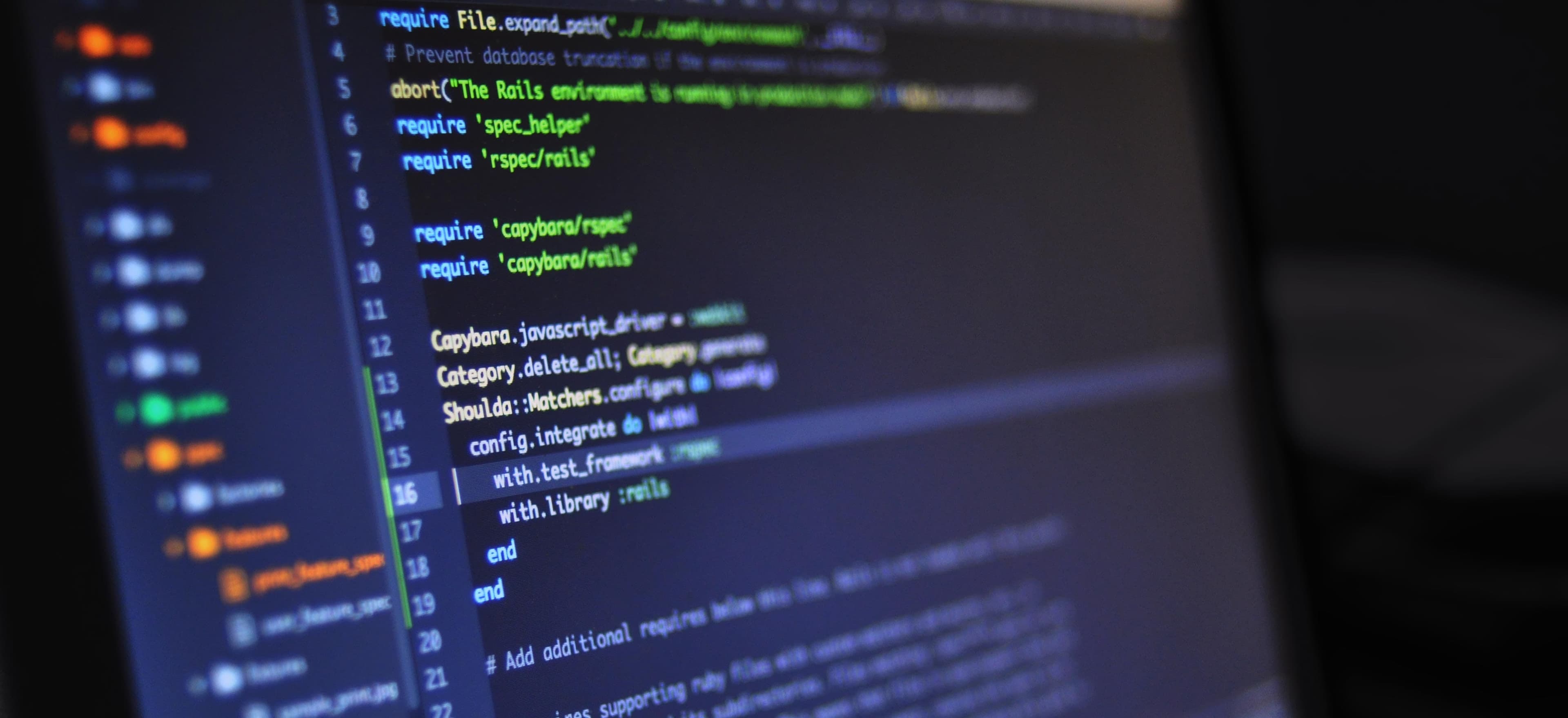
- Published on
Overcoming Common Pitfalls in Kafka REST Proxy Setup
Apache Kafka has become a de-facto standard for streaming data. While many users appreciate its efficiency, the learning curve can be steep, particularly when it comes to integrating Kafka with various systems using the Kafka REST Proxy. This blog post aims to guide you through the common pitfalls encountered during the setup of Kafka REST Proxy and how to overcome them effectively.
What is Kafka REST Proxy?
The Kafka REST Proxy offers a simple way to interact with Kafka without requiring the use of Kafka clients. By using HTTP REST API calls, applications can produce and consume messages easily and retrieve metadata. With the REST Proxy, developers can build applications that communicate with Kafka using familiar protocols without needing to implement or manage the complexities of the Kafka protocol directly.
However, setting up Kafka REST Proxy is not always straightforward. Let's explore the common pitfalls and their solutions.
Pitfall #1: Misconfigured Server Properties
One of the first hurdles during the setup phase of Kafka REST Proxy is misconfiguring server properties. These properties dictate how the server operates and connects to the Kafka broker.
Solution: Double-check Configuration Files
The configuration for the REST Proxy is mainly handled through the application.properties
file. Here's a basic configuration snippet:
bootstrap.servers=localhost:9092
port=8082
Why this matters: The bootstrap.servers
property needs to point to your active Kafka brokers. Ensure that the port number is correct or modify it according to your requirements. Here's a further breakdown:
- bootstrap.servers: List of Kafka brokers that the REST Proxy should connect to.
- port: The port on which the REST Proxy will expose the REST API.
Configuration Verification
After setting up, use the following command to verify the connection:
curl -X GET http://localhost:8082/v3/clusters
If configured correctly, you should receive a response containing cluster information. If not, examine your server logs for error messages indicating connectivity issues.
Pitfall #2: Inadequate Security Configurations
Security is a critical aspect often overlooked during the setup. Integrating Kafka REST Proxy into a secured Kafka cluster requires setting up SSL or SASL.
Solution: Implement Proper Security Configurations
For secured environments, you must provide SSL or SASL configurations. An example snippet can look like this:
security.protocol=SASL_SSL
sasl.mechanism=PLAIN
sasl.jaas.config=org.apache.kafka.common.security.plain.PlainLoginModule required
username="myuser"
password="mypassword";
Why Security Matters: Ensuring your data remains secure is non-negotiable, especially when traversing networks. Failure to configure security properly can expose sensitive data and lead to unauthorized access.
Make sure to also verify the configurations through REST API calls. If you encounter issues, reassess your credentials and ensure your Kafka brokers have the correct settings as well.
Pitfall #3: Lack of Response Handling in Client Applications
Once your REST Proxy is running, clients make HTTP requests to interact with it. A common mistake at this stage includes inadequate error handling.
Solution: Implement Robust Error Handling
Many developers focus solely on writing the code for successful requests and overlook handling errors. Here's an example of how to handle responses in Java:
import java.net.HttpURLConnection;
import java.net.URL;
public class KafkaRestClient {
public static void main(String[] args) {
try {
URL url = new URL("http://localhost:8082/topics/my-topic");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
int responseCode = conn.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
System.out.println("Success: " + responseCode);
} else {
System.out.println("Failed: " + responseCode);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why Robust Error Handling is Essential: Error handling is critical for diagnosing failures in production environments. This code checks the response code and provides clear feedback. You should expand this to manage specific HTTP error codes and provide corresponding messaging.
Pitfall #4: Incorrect Data Formats
When sending or consuming messages, organizations often use incompatible data formats between client applications and Kafka.
Solution: Standardize Data Formats
Using Avro, JSON, or Protobuf consistently across your applications helps prevent issues. Here’s how you might send JSON data to a Kafka topic using the POST method:
curl -X POST \
-H "Content-Type: application/vnd.kafka.json.v2+json" \
--data '{"value": {"field1": "value1", "field2": "value2"}}' \
http://localhost:8082/topics/my-topic
Why Standardization is Critical: Inconsistent data formats cause serialization issues, leading to unexpected errors during consumption. It’s essential that producers and consumers agree on message formats beforehand.
Pitfall #5: Missing Consumer Configuration
When consuming messages via the REST Proxy, developers may forget to set up the appropriate consumer configurations.
Solution: Specify All Required Consumer Options
Consumers through the REST Proxy should specify proper consumer configurations like group ID and session timeout. An example of the correct setup is:
curl -X POST \
-H "Content-Type: application/vnd.kafka.v2+json" \
--data '{"name": "my-consumer", "format": "json", "auto.offset.reset": "earliest"}' \
http://localhost:8082/consumers/my-group
Why Consumer Configuration is Important: Properly configuring the consumer ensures that you retrieve messages from Kafka accurately. Misconfigured settings can lead to missed messages or repeated consumption.
A Final Look
Setting up Kafka REST Proxy can be riddled with challenges, but recognizing common pitfalls allows you to grasp configurations better and integrate Kafka seamlessly within your applications.
Remember the importance of meticulous configuration, security protocol, error handling, data format consistency, and consumer setup. By following these guidelines, you significantly increase the chances of establishing a robust and functional Kafka REST Proxy environment.
For more details on Kafka REST Proxy, you can check the Apache Kafka Documentation.
Additional Resources
- Kafka REST Proxy: Getting Started
- Common Kafka REST Proxy Patterns
- Understanding Kafka Data Serialization
Now, as you embark on your Kafka REST Proxy journey, ensure you plan adequately and address these potential pitfalls to create a successful integration. Happy coding!
Checkout our other articles