Understanding Dependency Injection Challenges in Spring Framework
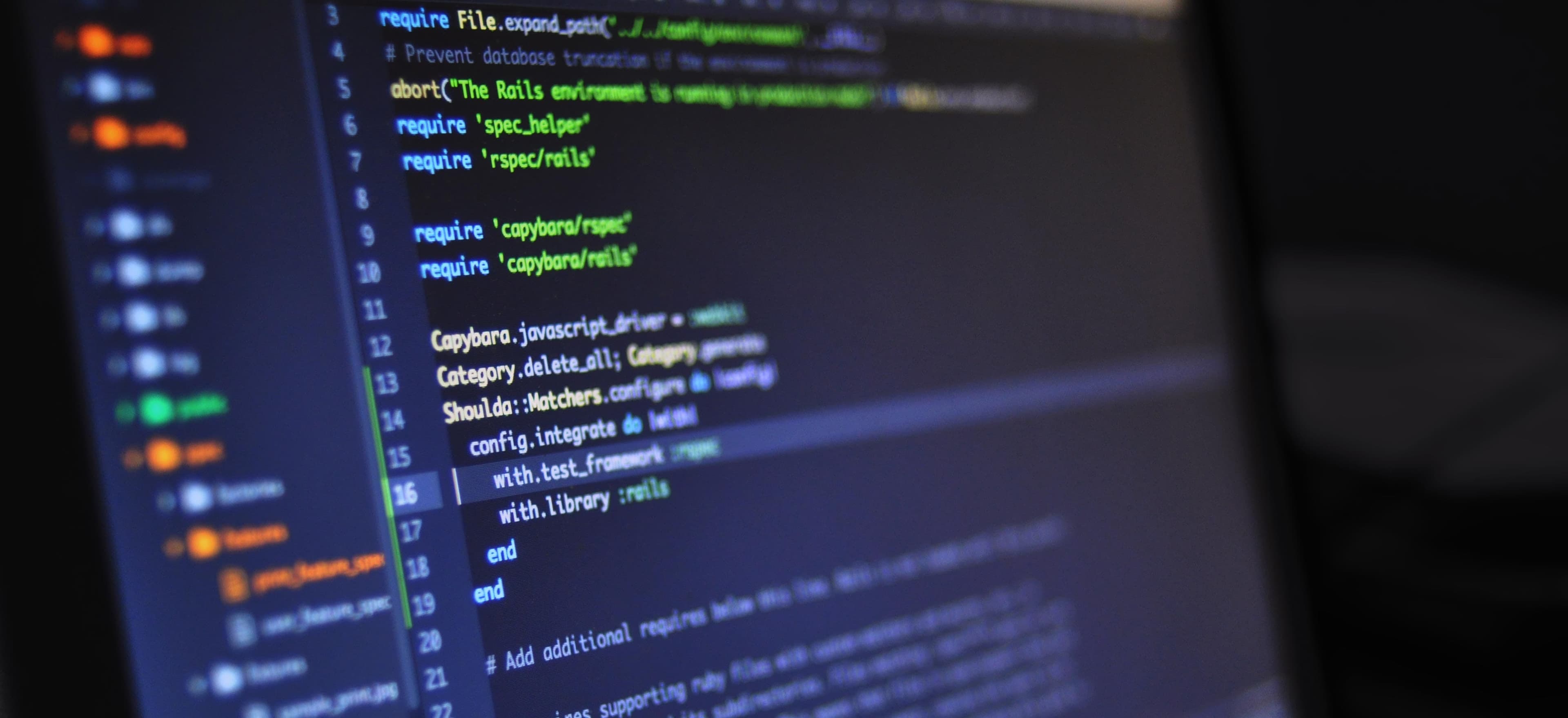
- Published on
Understanding Dependency Injection Challenges in Spring Framework
Dependency Injection (DI) is a core principle of the Spring Framework, which simplifies the process of wiring application components. While Spring is powerful and flexible, it is not without its challenges. This blog post delves into the intricacies of Dependency Injection in Spring, highlighting common challenges developers face and ways to troubleshoot them.
What is Dependency Injection?
Dependency Injection is a design pattern that allows the removal of hard-coded dependencies, making the code more modular and testable. In essence, it involves providing an object (or dependency) to another object in a way that promotes loose coupling.
public class Car {
private Engine engine;
public Car(Engine engine) {
this.engine = engine;
}
public void start() {
engine.start();
}
}
In the example above, Car
class depends on Engine
. Instead of Car
creating an instance of Engine
, we inject Engine
into the Car
constructor. This promotes better code organization and easier unit testing.
Core Principles of Dependency Injection
-
Inversion of Control (IoC): The control of dependency management is inverted from the object itself to a container or framework, where Spring takes the wheel.
-
Loose Coupling: Components interact through interfaces rather than concrete implementations, allowing changes without affecting the system.
-
Separation of Concerns: Each class handles its own responsibility, leading to a clear structure, easier management, and a cleaner codebase.
Spring Dependency Injection Challenges
While the advantages of DI are noteworthy, several challenges arise in its implementation within the Spring Framework.
1. Circular Dependencies
One of the more common pitfalls in Spring's DI is circular dependencies. This situation occurs when two or more beans reference each other.
@Component
public class A {
@Autowired
B b;
}
@Component
public class B {
@Autowired
A a;
}
In this scenario, the Spring container cannot instantiate A
because it requires B
, while B
needs A
to be fully constructed.
Solution: Use Setter Injection
To break the cycle, you can use setter injection, which allows each bean to be created without meeting its dependency head-on.
@Component
public class A {
private B b;
@Autowired
public void setB(B b) {
this.b = b;
}
}
@Component
public class B {
private A a;
@Autowired
public void setA(A a) {
this.a = a;
}
}
By deferring the wiring until after both objects are constructed, you alleviate the circular contradiction.
2. Over-Autowiring
Spring’s @Autowired
annotation makes injecting dependencies straightforward. However, over-autowiring can lead to unexpected behaviors, particularly when multiple beans of the same type exist.
@Component
public class Driver {
@Autowired
private Vehicle vehicle; // Ambiguity if there are multiple Vehicle beans
}
Solution: Use @Qualifier
Using the @Qualifier
annotation can resolve ambiguity by explicitly defining which bean to inject.
@Autowired
@Qualifier("car")
private Vehicle vehicle;
This ensures that Spring will always inject the correct instance.
3. Scope Mismatches
Spring beans can have different scopes: singleton, prototype, request, session, etc. Mixing up these scopes can lead to unintentional behavior and memory leaks, particularly when a singleton bean is used with prototype-scoped dependencies.
@Component
@Scope("singleton")
public class SingletonBean {
@Autowired
private PrototypeBean prototypeBean; // Memory leak potential
}
Solution: Use Lookup Method Injection
This method allows you to dynamically fetch a prototype bean by defining an abstract method in the singleton bean.
@Component
@Scope("singleton")
public abstract class SingletonBean {
public PrototypeBean getPrototypeBean() {
return ApplicationContextProvider.getApplicationContext().getBean(PrototypeBean.class);
}
}
This provides fresh instances each time while maintaining overall application integrity.
4. Testing and Mocking
Dependency injection brings about an automated way to handle dependencies, but it can complicate unit testing. Mocking dependencies in tests requires an understanding of the Spring context.
Solution: Use Spring Test Context Framework
By utilizing Spring’s test context management, one can easily create a context for testing.
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = {TestConfig.class})
public class ServiceTest {
@Autowired
private MyService myService;
@MockBean
private MyDependency myDependency;
@Test
public void testMyService() {
// Logic to test myService
}
}
This approach allows for controlling the environment, ensuring that tests are both predictable and reproducible.
5. Configuration Complexity
As applications grow, managing @Configuration
classes or XML files can get cumbersome. Too many configurations can lead to confusion and errors in dependency management.
Solution: Utilize Profiles
Spring Profiles allow you to group different configurations for various environments.
@Configuration
@Profile("dev")
public class DevDatabaseConfig {
// Dev environment beans
}
@Configuration
@Profile("prod")
public class ProdDatabaseConfig {
// Production environment beans
}
This lets you switch configurations easily without altering the core application code.
To Wrap Things Up
Dependency Injection in Spring Framework is essential but comes with its own set of challenges. Understanding and troubleshooting these issues leads to robust application design. From avoiding circular dependencies to managing scope mismatches, being equipped with knowledge helps developers navigate complexities better.
For further reading, consider taking a look at Spring's Official Documentation and The Dependency Injection Pattern. These resources deepen understanding and enhance skills in crafting clean, maintainable code within the Spring ecosystem.
By acknowledging the challenges that arise in Dependency Injection and implementing effective strategies to overcome them, developers can leverage Spring Framework to build powerful and scalable applications. Embrace these insights to enhance your coding practice and streamline your development process. Happy coding!
Checkout our other articles