Mastering Application Context: 3 Key Retrieval Methods
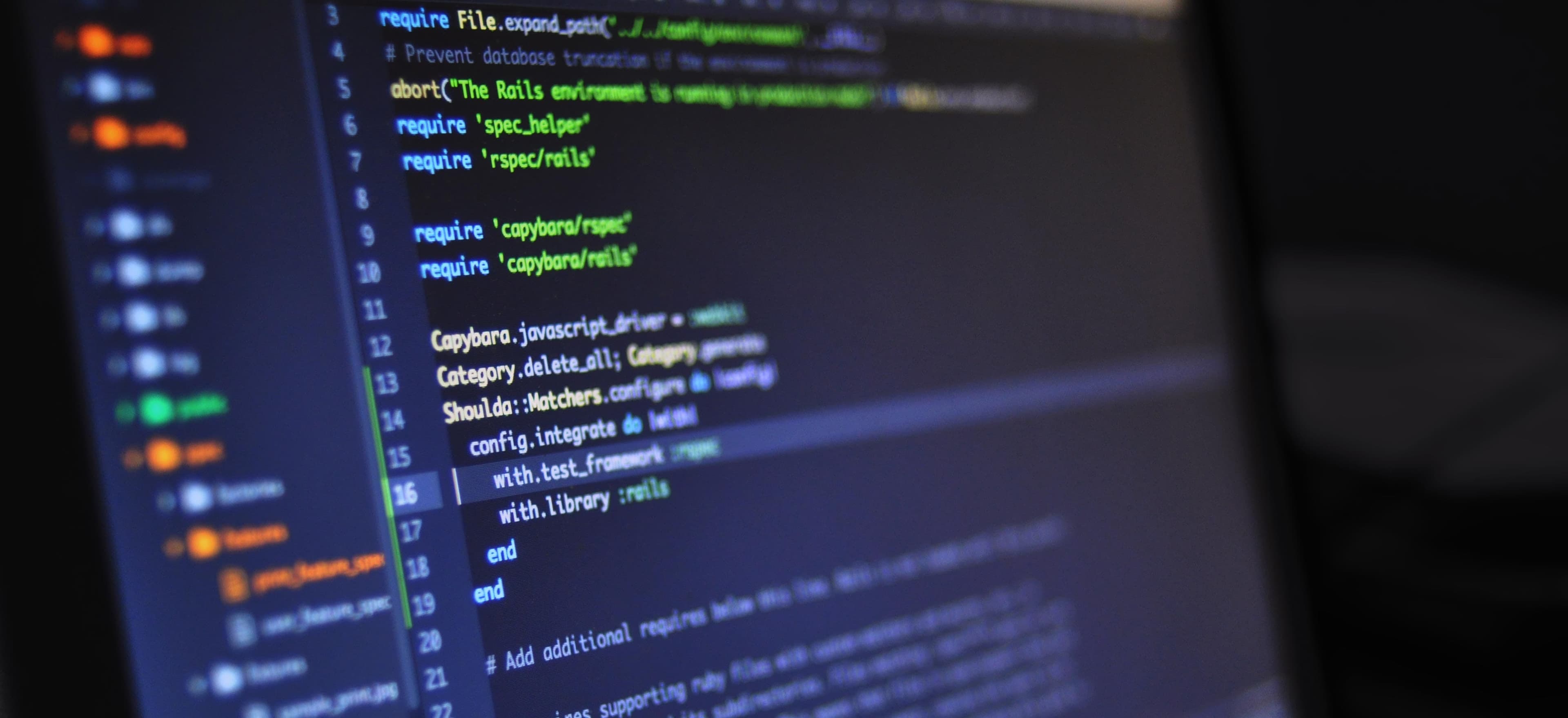
- Published on
Mastering Application Context: 3 Key Retrieval Methods
In the world of Java programming, especially within the realm of Android development, understanding the Application Context is crucial. The Application Context serves as a single global instance available throughout the whole application lifecycle. This post delves into the intricacies of the Application Context and provides three key methods for retrieving it, enhancing your capability to harness its functionality effectively.
What is Application Context?
In Android, there are two main types of context:
- Activity Context: This is tied to the lifecycle of an activity. It is destroyed once the activity is no longer needed.
- Application Context: This is created when the application is launched and remains in memory as long as the app is running.
Using Application Context comes in handy when you need a context that won't depend on a specific Activity's lifecycle, such as when you’re setting up services or accessibility that should outlive any individual Activity.
Why Application Context?
Using Application Context has several advantages:
- This context lasts throughout the entire application lifespan, which is ideal for singletons and other long-lived instances.
- It avoids memory leaks that can occur if an Activity context is referenced after the Activity has been destroyed.
Now, let's dive into the three key methods for retrieving the Application Context in Java.
1. Accessing Application Context from an Activity
One of the simplest and most common ways to obtain the Application Context is directly from an Activity. Since every Activity extends the Context class, you can use the getApplicationContext()
method.
Code Example
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Retrieve Application Context
Context appContext = getApplicationContext();
Log.i("ContextInfo", "Retrieved Application Context");
}
}
Commentary
In the above example, getApplicationContext()
provides a reference to the Application Context. This retrieval is convenient and straightforward. Since MainActivity
extends AppCompatActivity
, it naturally inherits methods from the Context class.
2. Using Application in a Custom Application Class
Another efficient way to retrieve Application Context is to create a custom Application
class. You can override the onCreate()
method to store the Application Context for later use.
Code Example
public class MyApplication extends Application {
private static MyApplication instance;
@Override
public void onCreate() {
super.onCreate();
instance = this;
}
public static Context getAppContext() {
return instance.getApplicationContext();
}
}
Commentary
In this example, we store the instance of the Application class in a static variable. The method getAppContext()
allows easy retrieval of the Application Context from anywhere within your application. Ensure that you declare your custom application class in the AndroidManifest.xml:
<application
android:name=".MyApplication"
...
>
This approach centralizes access to your Application Context and maintains a clear code structure.
3. Retrieving Application Context Through a Service
If you have a service running in the background, you can easily access the Application Context from there as well.
Code Example
public class MyService extends Service {
@Override
public IBinder onBind(Intent intent) {
// Binding logic
return null;
}
@Override
public void onCreate() {
super.onCreate();
// Retrieve Application Context
Context appContext = getApplicationContext();
Log.i("ContextInfo", "Retrieved Application Context from Service");
}
}
Commentary
In this example, we demonstrate how the getApplicationContext()
method can also be utilized within a Service. This method helps retrieve a stable reference to the Application Context, which is beneficial if your Service is long-lived or if it interacts with components that require context outside an Activity's lifecycle.
By leveraging the Application Context correctly, you will substantially reduce the risk of memory leaks that often plague Android applications.
Bringing It All Together
Understanding how to effectively retrieve Application Context is essential for any Java or Android developer. Applying these retrieval methods boosts your ability to manage resources efficiently while maintaining high-quality application performance.
Summary of Key Retrieval Methods
- From an Activity: Utilizing
getApplicationContext()
method for immediate access. - Custom Application Class: Creating a static method in a singleton Application class for global access.
- From Services: Retrieving directly from Services, offering stability during background operations.
Further Reading
To deepen your knowledge, consider exploring the following resources:
- Android Developers - Application Context
- Android Architecture Components
- Memory Leaks in Android
By mastering the Application Context and these retrieval techniques, you're on your way to building robust and efficient Android applications. Happy coding!
Checkout our other articles