Overcoming Common STOMP WebSocket Issues in ActiveMQ
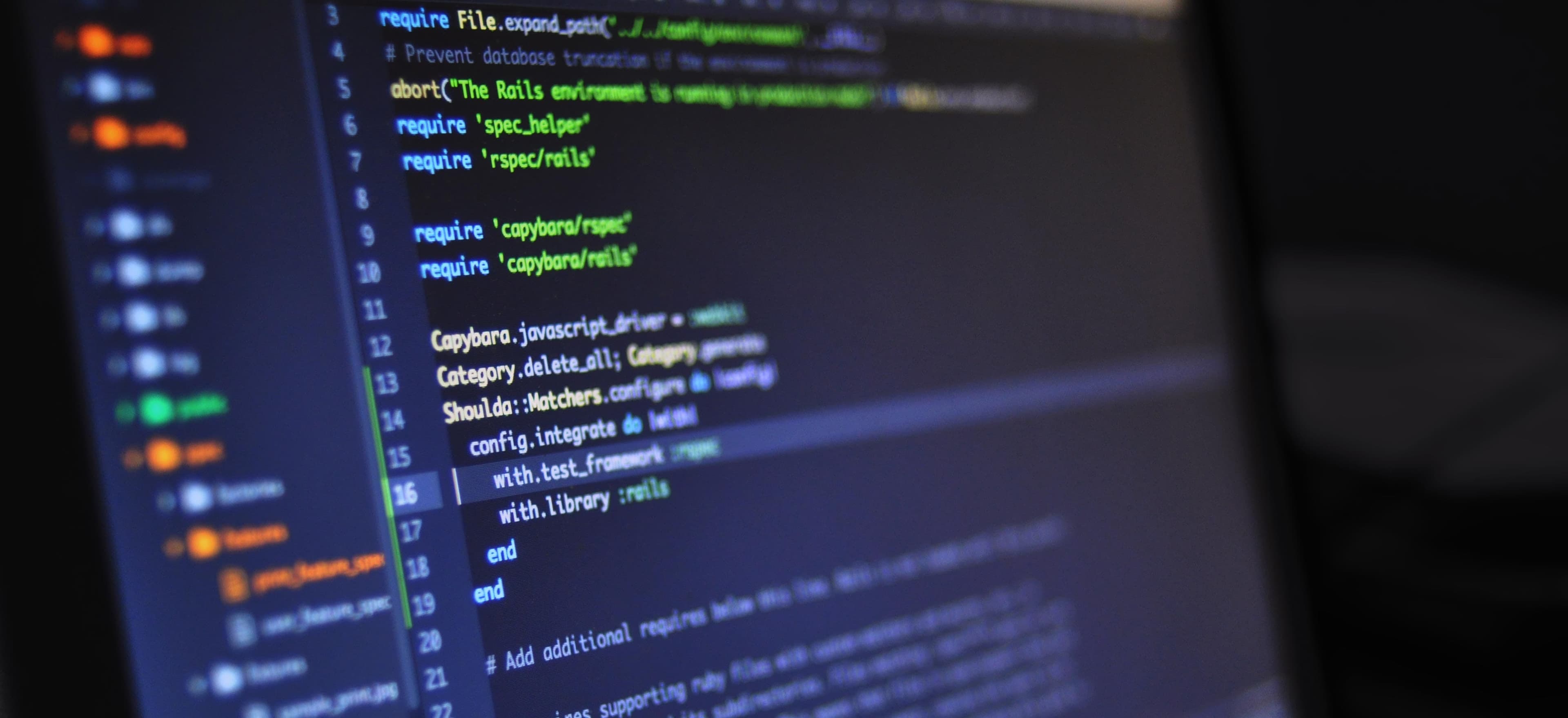
- Published on
Overcoming Common STOMP WebSocket Issues in ActiveMQ
ActiveMQ is a versatile message broker that supports various messaging protocols, including STOMP (Simple Text Oriented Messaging Protocol) over WebSocket. The combination of STOMP with WebSockets is powerful, as it allows for real-time communication in web applications. However, like any technology, it comes with its own set of challenges. This blog post aims to address common issues developers encounter when implementing STOMP over WebSocket in ActiveMQ, guiding you through solutions and best practices.
Understanding STOMP and WebSockets
Before delving into specific issues, let’s clarify what STOMP and WebSockets are:
-
STOMP: STOMP is a simple and lightweight messaging protocol that allows clients to interact with message brokers. It’s widely used due to its simplicity and ease of use, making integration into various applications straightforward.
-
WebSockets: WebSockets provide full-duplex communication channels over a single TCP connection. They are ideal for real-time applications where low latency is critical, such as chat applications or live data feeds.
Combining STOMP and WebSockets can allow for seamless real-time messaging solutions. However, you may encounter some common pitfalls.
Key Issues and Solutions
1. Connection Issues
Problem:
Often, the first hurdle when dealing with STOMP over WebSocket is establishing a proper connection. This can be influenced by network issues, broker configuration, or client settings.
Solution:
Ensure that the ActiveMQ server is properly configured to support WebSocket connections. Check the following settings in your activemq.xml
configuration file:
<transportConnector name="websocket" uri="ws://0.0.0.0:61614"/>
This line specifies the transport protocol and port. Make sure:
- The server is running and you can reach it via the specified port.
- Firewall settings are not blocking the WebSocket connection.
In your client code, you would connect to the WebSocket like this:
const client = Stomp.over(new SockJS('http://your-server:61614/stomp'));
client.connect({}, (frame) => {
console.log('Connected: ' + frame);
}, (error) => {
console.error('Connection error: ' + error);
});
Here, we use SockJS as a fallback for browsers that don't support WebSockets directly.
2. Handling Message Encoding
Problem:
Another frequent issue arises from character encoding. Different clients might send messages that are not properly encoded, leading to unreadable characters.
Solution:
Ensure that both the client and server are using the same encoding format. UTF-8 is widely supported and recommended.
In your ActiveMQ configuration, this is typically set by default, but always check:
<systemUsage>
<systemUsage>
<memoryUsage>
<memoryUsage limit="64mb"/>
</memoryUsage>
<storeUsage>
<storeUsage limit="2gb"/>
</storeUsage>
<tempUsage>
<tempUsage limit="1gb"/>
</tempUsage>
</systemUsage>
</systemUsage>
On the client side, ensure your messages are encoded properly:
const message = JSON.stringify({ text: "Hello World", encoding: "UTF-8" });
client.send("/topic/greetings", {}, message);
3. Subscription Management
Problem:
Managing subscriptions can lead to confusion, particularly if multiple subscribers are involved. You might encounter scenarios where subscribers do not receive messages they expect or unsubscribe unexpectedly.
Solution:
To effectively manage subscriptions, implement proper logging and track subscription IDs. Use a unique identifier for each subscription to differentiate them.
Here’s how you can manage subscriptions:
const subscriptionId = client.subscribe('/topic/greetings', (message) => {
console.log('Received: ' + message.body);
});
Additionally, always unsubscribe when you're done:
client.unsubscribe(subscriptionId);
This prevents memory leaks and unintended message handling.
4. Error Handling
Problem:
Errors can occur at various stages during messaging, leading to dropped messages or unexpected behavior. These can be network issues, message format errors, or broker-side failures.
Solution:
Implement robust error handling mechanisms in your application. STOMP provides a way to catch errors directly from the server. Here’s an example:
client.connect({}, (frame) => {
console.log('Connected: ' + frame);
}, (error) => {
console.error('Connection error: ' + error);
});
Also, consider adding event listeners for message errors:
client.onStompError = function(frame) {
console.error('Broker reported error: ' + frame.headers['message']);
console.log('Additional details: ' + frame.body);
};
5. Performance Tuning
Problem:
When scaling your application, performance can become a bottleneck. If message throughput is low, your application will lag.
Solution:
You can optimize performance by adjusting the following:
- Pre-fetching Messages: This is particularly important for applications that consume messages in bulk.
<destinationPolicy>
<policyEntry topic=">" producerPrefetch="10" />
</destinationPolicy>
-
Connection Pooling: Maintain a pool of connections to the broker instead of creating and destroying connections for each message.
-
Load Balancing: Consider deploying multiple instances of ActiveMQ and distributing the load among them.
The Bottom Line
By understanding the common challenges with STOMP over WebSocket in ActiveMQ, you can develop more robust and efficient real-time applications. Remember to regularly review both your client and server configurations and implement best practices to avoid these pitfalls.
Additional Resources
- ActiveMQ Documentation
- STOMP Protocol Specification
- WebSocket API Documentation
By following these guidelines, you will be equipped to handle the most common STOMP WebSocket issues effectively. Happy coding!
Checkout our other articles