Common Microservices Pitfalls and How to Avoid Them
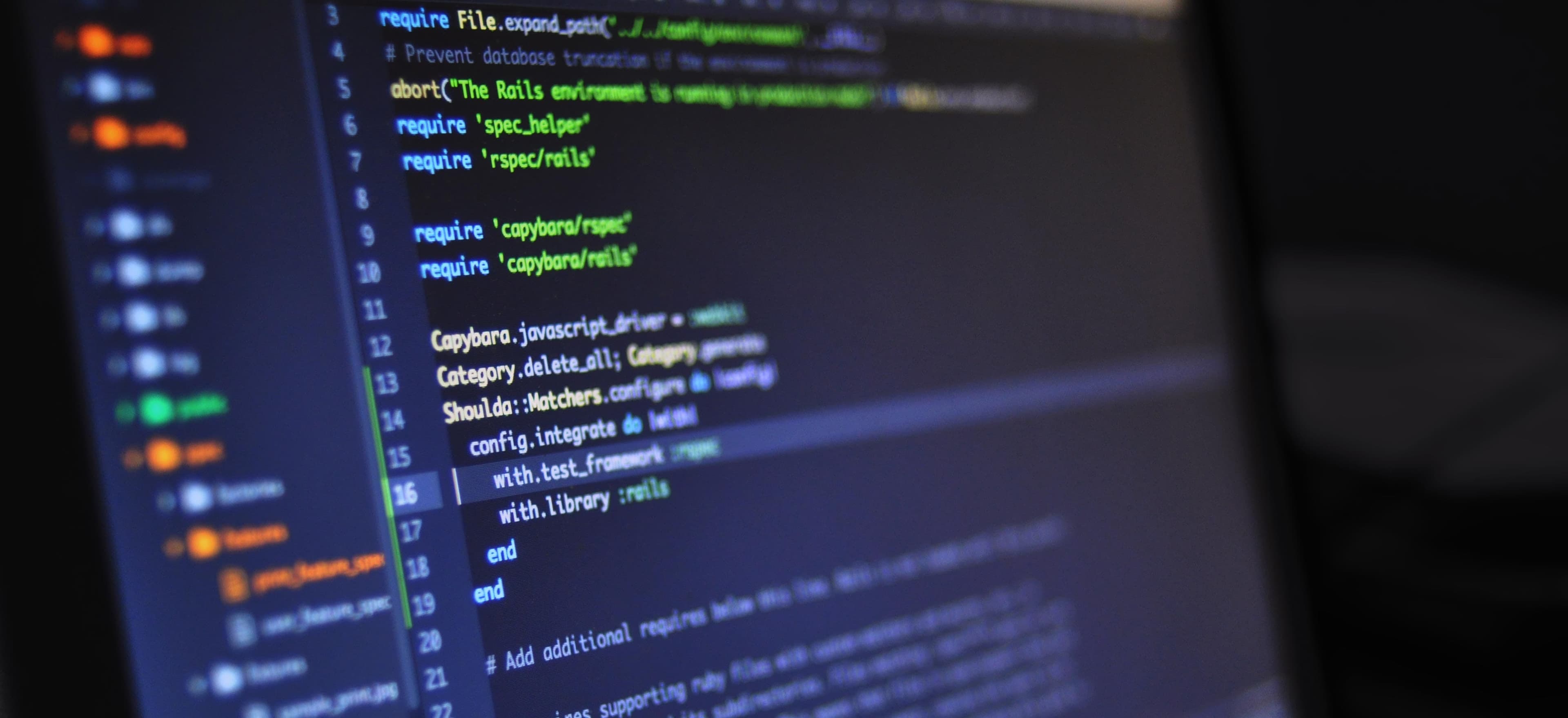
- Published on
Common Microservices Pitfalls and How to Avoid Them
Microservices architecture has gained immense popularity in recent years due to its scalability, flexibility, and ease of deployment. However, transitioning to microservices can introduce a variety of challenges and pitfalls. Understanding these potential issues and knowing how to avoid them is crucial for the success of any microservices initiative.
1. Lack of Service Boundaries
One of the most common pitfalls is the failure to define clear boundaries for each microservice. Without well-defined boundaries, you risk creating a monolith wrapped in microservices, which can defeat the purpose of adopting microservices architecture.
Avoiding This Pitfall: Define Bounded Contexts
A bounded context is critical for accurately defining service boundaries. Each microservice should represent a single business capability.
public class OrderService {
// Handles order placement
}
public class PaymentService {
// Handles payment processing
}
In the example above, the OrderService
and PaymentService
have distinct responsibilities. This separation simplifies development, testing, and scaling—allowing teams to work independently.
Additional Resource
- Exploring Bounded Contexts with Domain-Driven Design
2. Over-Engineering Services
Another common mistake is over-engineering microservices, where architects create overly complex systems with unnecessary features. This leads to increased maintenance burdens and performance issues.
Avoiding This Pitfall: Start Simple and Evolve
A practical approach is to start with a minimal viable product (MVP). You can always enhance your services as the system evolves. Here's an example:
public class UserService {
private List<User> users;
public UserService() {
users = new ArrayList<>();
}
public User createUser(String name) {
User user = new User(name);
users.add(user);
return user;
}
}
In this scenario, the UserService
starts simple, focusing solely on user creation. Additional features, such as user authentication and authorization, can be added later as needed.
3. Neglecting Data Management
When working with microservices, each service may manage its own database. A common pitfall is neglecting how services will communicate and manage data dependencies.
Avoiding This Pitfall: Use API Composition
One effective technique is to implement API composition for aggregating data from multiple services. By doing this, you prevent tight coupling and direct database access between services. Here’s a simple example using Spring Boot:
@RestController
@RequestMapping("/api")
public class UserController {
@Autowired
private OrderService orderService;
@GetMapping("/userOrders/{userId}")
public UserOrdersResponse getUserOrders(@PathVariable String userId) {
User user = userService.getUser(userId);
List<Order> orders = orderService.getUserOrders(userId);
return new UserOrdersResponse(user, orders);
}
}
In this example, UserController
aggregates user information alongside their respective orders without tightly coupling the services.
Additional Resource
- Data Management in Microservices
4. Mismanagement of Inter-Service Communication
Choosing the right communication protocol is essential. Using synchronous communication everywhere can lead to cascading failures and performance bottlenecks.
Avoiding This Pitfall: Mix Communication Patterns
Consider using a mix of synchronous and asynchronous communication patterns. While RESTful APIs are great for user-initiated requests, message brokers (like Apache Kafka or RabbitMQ) can facilitate decoupled communication:
public void publishOrderCreated(Order order) {
kafkaTemplate.send("order-created", order);
}
This example demonstrates how the publishOrderCreated
method uses Kafka to publish an event after an order is created. Asynchronous messaging allows for better resilience and scalability.
Additional Resource
- Microservices Communication Patterns
5. Ignoring Security Best Practices
Security can easily take a backseat in development priorities, especially with the rapid pace of development in microservices.
Avoiding This Pitfall: Implement Security from the Start
Utilizing APIs increases the attack surface; hence, security should be built into each service from the ground up. Consider implementing token-based authentication using JWT (JSON Web Tokens):
public String generateToken(String username) {
Map<String, Object> claims = new HashMap<>();
// additional claims can be added
return Jwts.builder()
.setClaims(claims)
.setSubject(username)
.setIssuedAt(new Date(System.currentTimeMillis()))
.setExpiration(new Date(System.currentTimeMillis() + JWT_EXPIRATION))
.signWith(SignatureAlgorithm.HS256, SECRET_KEY)
.compact();
}
In this code, we generate a JWT for a user after they authenticate. This ensures that only legitimate users can interact with each microservice, helping protect the overall system.
Additional Resource
6. Underestimating Operational Complexity
Microservices introduce significant operational complexity, which can overwhelm teams not prepared for it. This includes deployment, monitoring, and troubleshooting.
Avoiding This Pitfall: Embrace DevOps Practices
DevOps culture and practices can significantly mitigate these issues. Implement CI/CD pipelines for automated testing and deployment to ensure that services are deployed consistently:
# Jenkins Pipeline Script
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Deploy') {
steps {
sh 'docker-compose up -d'
}
}
}
}
By utilizing a CI/CD tool (like Jenkins), you automate the building and deploying of microservices. This reduces human error and speeds up release cycles.
Additional Resource
- DevOps Practices for Microservices
Closing Remarks
While microservices offer remarkable advantages, they are not without their challenges. By recognizing these common pitfalls and employing proactive strategies, your organization can successfully harness the power of microservices.
Remember, clarity in service boundaries, careful data management, pragmatic communication strategies, solid security measures, and a robust operational framework are essential for thriving in a microservices landscape.
Want to dive deeper into advanced microservices concepts? Check out this insightful site: Microservices.io.
Embrace these guidelines, and you will be on a principled journey toward a successful microservices architecture. Happy coding!
Checkout our other articles