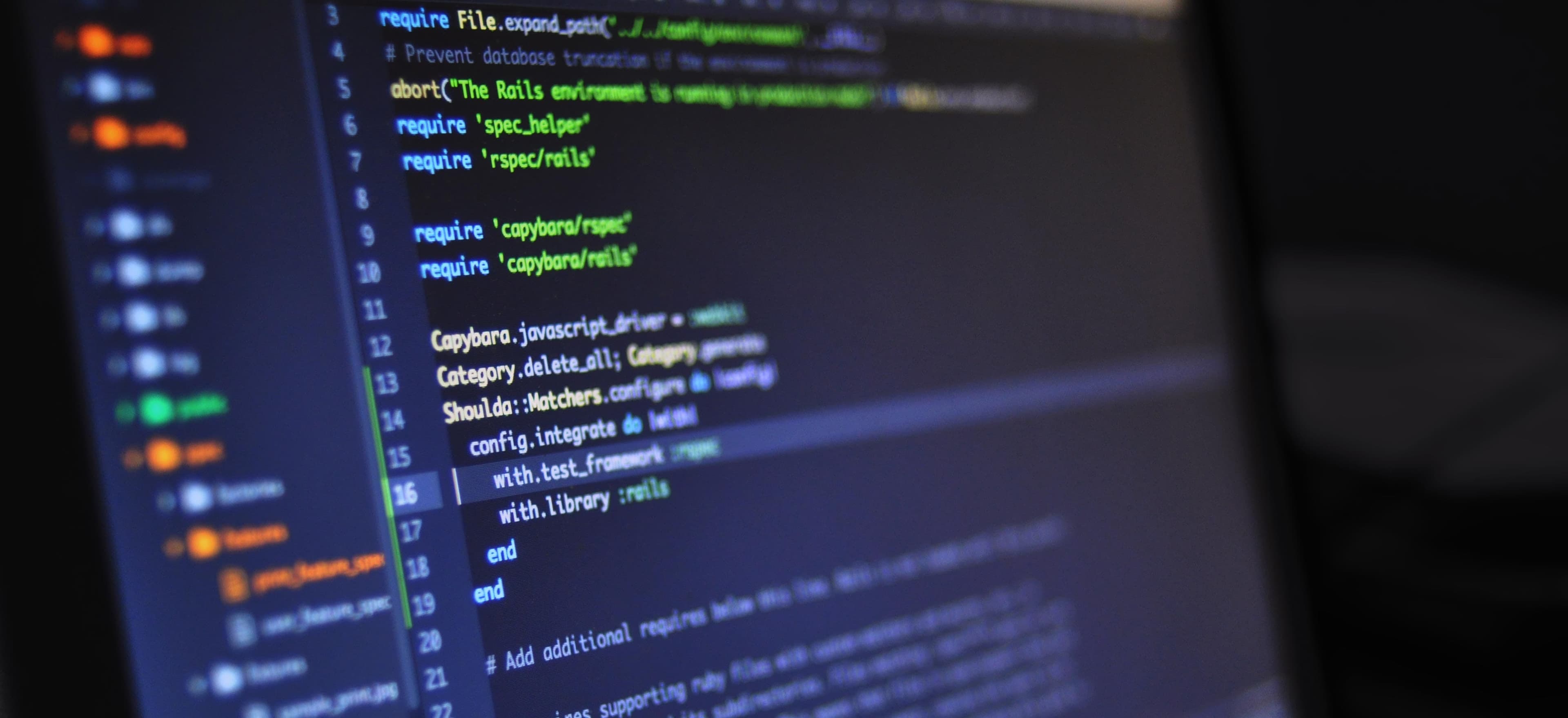
- Published on
Mastering Android Header/Footer Layouts: Avoiding Common Pitfalls
Creating a well-structured user interface is crucial in Android development. Among the various layout elements, headers and footers play a significant role in enhancing user experience. In this blog post, we'll explore the intricacies of implementing header and footer layouts in Android applications while avoiding common pitfalls.
Understanding Header and Footer Layouts
What are Header and Footer Layouts?
A header is the upper part of a page or section, often containing the title, navigation, or branding elements. A footer, on the other hand, appears at the bottom and frequently contains copyright information, additional navigation links, or important contact details.
Both are essential for giving a consistent look and feel throughout your application. Header and footer layouts allow your users to navigate easily and understand context better.
Why Use Header and Footer Layouts?
- Improved Navigation: Headers often contain navigation bars, enabling users to access different sections quickly.
- Consistency: Using the same header and footer across multiple activities/fragments maintains visual uniformity.
- User Engagement: Headers with dynamic content create a more engaging experience.
For more background on Android layouts, check out the official Android Developers documentation.
Common Pitfalls When Implementing Header/Footer Layouts
-
Overcomplicating Layouts
- Avoid using nested layouts excessively as they can slow down rendering and lead to a poor user experience.
-
Ignoring Screen Sizes and Orientation
- Design responsive layouts that adapt to different screen sizes and orientations.
-
Not Using Constraints Properly
- Constraints ensure that your layout behaves as expected across different devices and form factors.
Best Practices for Android Header/Footer Layouts
Let's go through some best practices for implementing header and footer layouts effectively.
1. Use ConstraintLayout
Using ConstraintLayout
is one of the best practices for organizing your layouts due to its efficiency and flexibility. Here’s how to create a header and footer using ConstraintLayout
.
Example Code Snippet
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/header"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="Header Title"
android:textSize="24sp"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent" />
<TextView
android:id="@+id/content"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginTop="16dp"
android:text="Main Content Here"
app:layout_constraintTop_toBottomOf="@+id/header"
app:layout_constraintBottom_toTopOf="@+id/footer"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent" />
<TextView
android:id="@+id/footer"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="Footer Information"
android:textSize="16sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Commentary
In the above code:
- We use
ConstraintLayout
to achieve a flexible and scalable design. - The header is anchored to the top of the layout, the content area fills the space between the header and footer, and the footer is anchored to the bottom.
This single layout eliminates unnecessary nesting.
2. Avoid Hardcoded Dimensions
Hardcoded dimensions can break your layout on different devices. Instead, opt for dimens.xml
for define consistent spacing and sizing across layouts.
Example Code Snippet
<dimen name="header_height">56dp</dimen>
<dimen name="footer_height">48dp</dimen>
<dimen name="content_margin">16dp</dimen>
In your layout:
<TextView
android:id="@+id/header"
android:layout_width="match_parent"
android:layout_height="@dimen/header_height"/>
Commentary
Using dimens.xml
allows you to maintain consistent sizes and margins, making it easier to adapt when creating different layouts for various screen sizes.
3. Use ViewGroups Efficiently
While ConstraintLayout
is versatile, sometimes it makes sense to use a combination of other layouts. However, avoid using deeply nested layouts.
Example Combination
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView android:id="@+id/header"... />
<FrameLayout
android:id="@+id/content"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1" />
<TextView android:id="@+id/footer"... />
</LinearLayout>
Commentary
In this example, a simple LinearLayout
is used, where the header and footer have their heights fixed, and the content area is flexible (using layout_weight
). This simplification can aid your understanding while preventing over-complication.
4. Optimize for Scrollable Content
If your main content area is scrollable, ensure your header and footer remain fixed using a CoordinatorLayout
.
Example Code Snippet
<androidx.coordinatorlayout.widget.CoordinatorLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/header"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:layout_gravity="top"/>
<ScrollView
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior">
<TextView
android:id="@+id/content"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</ScrollView>
<TextView
android:id="@+id/footer"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:layout_gravity="bottom"/>
</androidx.coordinatorlayout.widget.CoordinatorLayout>
Commentary
The CoordinatorLayout
allows the header and footer to remain visible while the content scrolls. This layout is essential for creating a modern application interface that lends to a better user experience.
Key Takeaways
Mastering header and footer layouts in Android not only separates your UI elements but also enhances usability and readability. By avoiding common pitfalls and applying the best practices we discussed, you can create cleaner, more efficient code. Understanding the specifics of how Android handles layout rendering will lead to better-performing applications.
Explore more about layouts and responsive design in the Android documentation here.
Implement the strategies discussed, and elevate your Android development skills. Happy coding!