Boosting Readability of Stream Operations with Local Classes
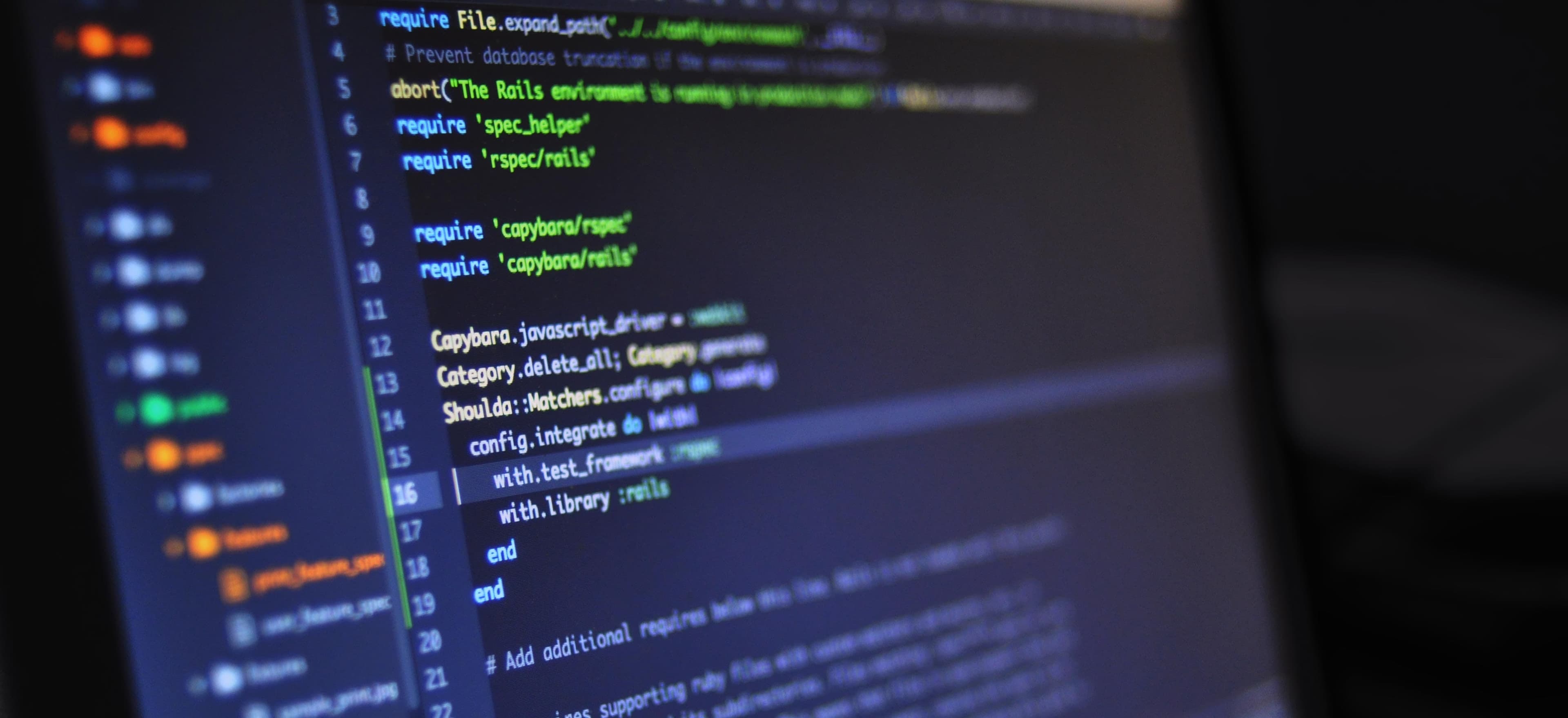
- Published on
Boosting Readability of Stream Operations with Local Classes in Java
In the modern era of Java programming, Stream API has revolutionized the way we process collections of data. However, the eloquence of stream operations can sometimes be overshadowed by lengthy lambda expressions, especially in intricate operations. One effective approach to enhance clarity and maintainability in such situations is through the utilization of local classes. In this blog post, we will explore how local classes can streamline stream operations and ultimately boost code readability.
Understanding Local Classes
A local class is defined within a method, constructor, or block and can access local variables from the enclosing scope. The core advantages of using local classes include encapsulation of logic, enhanced readability, and improved separation of concerns. This allows you to create a more maintainable approach to coding, especially when dealing with complex stream operations.
Why Use Local Classes?
- Encapsulation: Local classes can encapsulate specific logic related to a single method, making it easier to manage and understand.
- Clarity: Using named classes instead of anonymous inner classes often increases your code's clarity, especially for more complex operations.
- Reusability: Although they are scoped to a single method, they can reduce duplication of effort within that method.
Example of Stream Operations Without Local Classes
To illustrate the initial challenges, let's take a look at a simple use case where we have a list of integers and we want to filter and collect even numbers.
Without Local Classes
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println(evenNumbers);
}
}
While this code functions correctly, it lacks structure and may be difficult to decipher for someone unfamiliar with stream operations or lambda expressions.
Refactoring with Local Classes
Let's refactor the above code using a local class to enhance its readability. This will also help in segregating different operations for clarity.
With Local Classes
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamWithLocalClass {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
class EvenFilter {
boolean isEven(int number) {
return number % 2 == 0;
}
}
EvenFilter evenFilter = new EvenFilter();
List<Integer> evenNumbers = numbers.stream()
.filter(evenFilter::isEven)
.collect(Collectors.toList());
System.out.println(evenNumbers);
}
}
Explanation
- Local Class Creation: We defined a local class
EvenFilter
within themain
method. This class contains the methodisEven
, which encapsulates the logic for determining whether a number is even. - Using Method Reference: By using
evenFilter::isEven
, we directly reference the method of our local class, thus keeping the intention clear and understandable. This makes it easier for the reader to grasp what the code aims to achieve. - Improved Readability: With the added layer of local class, the code is much clearer as it indicates the function of the filtering logic without detracting from the overall stream operation.
Advantages of Using Local Classes with Streams
- Readability: The use of local classes breaks down complex operations into more manageable pieces.
- Easier Debugging: When an issue arises, local classes help isolate functionality and simplify debugging.
- Refactoring: When the need arises to change logic, local classes allow for localized adjustments without impacting other areas.
Considerations When Using Local Classes
While local classes provide numerous advantages in terms of readability and organization, they also come with considerations that should be kept in mind:
- Overhead: If local classes are too simple, they may add unnecessary complexity. Keep a balance between readability and simplicity.
- Access to Variables: Local classes can only access final or effectively final variables from the enclosing scope. This can be both a limitation and a protection against unintentional modifications.
Key Takeaways
Stream operations in Java can lead to highly compact and expressive code. However, when dealing with more complex transformations, it's vital to maintain clarity. By utilizing local classes, developers can not only enhance the readability of their code but also create more maintainable and encapsulated components for processing data.
Embracing local classes for stream operations is just one tactic in your programming toolbox. As Java evolves and new features emerge, always consider the balance between performance, readability, and maintainability in your code. For further reading and understanding about Java’s Stream API, you might want to check out the Java Documentation on Stream API.
Incorporating these practices will not only improve your coding experience but will also make collaboration with other developers smoother and more productive. Happy coding!
Checkout our other articles