Transforming Legacy Code: Overcoming Accessor Challenges
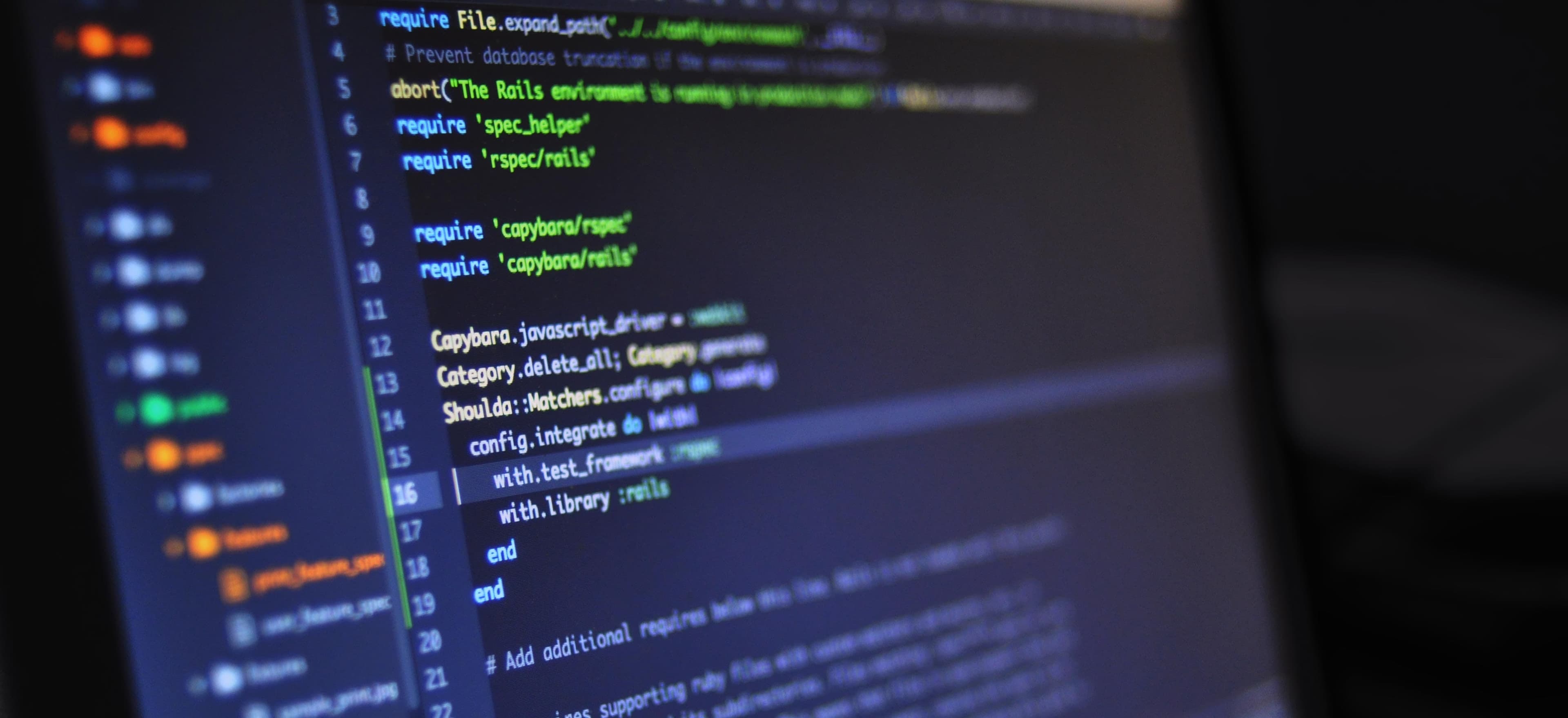
- Published on
Transforming Legacy Code: Overcoming Accessor Challenges
Legacy code can often be a maze of complex relationships, especially when it comes to accessor methods. Understanding how to effectively transform this old code into a manageable and scalable structure is essential for any developer. In this post, we'll tackle the common challenges associated with accessors in legacy code and present practical solutions that can help in transforming your codebase.
Understanding Legacy Code and Accessors
Legacy code is essentially code that is inherited from someone else or code that has been around for so long that it is difficult to change or improve. Often, this code lacks proper documentation and follows outdated practices that can make maintenance and feature additions problematic.
Accessors, or getter and setter methods, are integral parts of object-oriented programming as they provide a way to control access to an object's properties. However, poorly implemented accessors can lead to several issues:
- Encapsulation Violations: When accessors do not enforce encapsulation, they can expose sensitive internal states of an object.
- Unnecessary Complexity: Overusing accessors can lead to unnecessary complexity and hinder code readability.
- Difficulties in Testing: Legacy accessors might be tightly coupled with other parts of the system, making unit testing challenging.
Now, let’s explore how to strategically tackle these challenges while transforming legacy code.
Identifying Issues with Accessor Methods
The first step in overcoming accessor challenges is to identify where these issues occur. Here are some common signs:
- Excessive Boilerplate Code: If your class is merely consisting of getter and setter methods with no business logic, it may be a sign of high coupling.
Example:
public class User {
private String name;
private String email;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
In this code snippet, the User
class contains only accessors. There are no additional behaviors that define a user, which results in an anemic domain model.
Refactoring Accessors for Better Structure
After identifying the problems, the next step is to refactor accessors to provide better encapsulation and reduce complexity. Here are some strategies you can adopt:
1. Prioritize Domain Logic
Instead of adding only simple accessors, enhance your classes by embedding business logic directly into them. This can often eliminate the need for certain getters and setters.
Refactored Example:
public class User {
private String name;
private String email;
public User(String name, String email) {
setName(name);
setEmail(email);
}
public String getName() {
return name;
}
public void setName(String name) {
if (name != null && !name.trim().isEmpty()) {
this.name = name;
} else {
throw new IllegalArgumentException("Name cannot be null or empty");
}
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
if (email != null && email.contains("@")) {
this.email = email;
} else {
throw new IllegalArgumentException("Invalid email address");
}
}
public String displayInfo() {
return "User: " + name + ", Email: " + email;
}
}
In this revised code, validation logic is included in the setter methods, which not only simplifies the accessors but also enhances data integrity.
2. Use Immutable Objects
In many cases, immutable objects can eliminate the need for setters altogether, simplifying the state management.
Example of Immutable Class:
public final class ImmutableUser {
private final String name;
private final String email;
public ImmutableUser(String name, String email) {
this.name = name;
this.email = email;
}
public String getName() {
return name;
}
public String getEmail() {
return email;
}
}
By making the properties final
and providing only getters, we ensure that the state of the object cannot change after instantiation, decreasing the likelihood of unintended side effects.
3. Avoid Direct Access to Properties
Instead of exposing properties directly, use functional methods that represent actions. This is particularly useful when modifying properties should trigger additional behaviors.
Example:
public class User {
private String name;
private String email;
public void updateName(String name) {
// some complex logic might follow
setName(name);
}
public void updateEmail(String email) {
// some complex logic might follow
setEmail(email);
}
}
By encapsulating property changes in methods that reflect the intended action, you keep the user’s intent clear and manage side effects more effectively.
Testing Refactored Accessors
When refactoring accessors, it’s vital to ensure that the behavior of your classes remains intact. Employ unit tests to validate that no unintended consequences arise from your changes.
Example of a Simple JUnit Test:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
class UserTest {
@Test
void testSetName() {
User user = new User("Alice", "alice@example.com");
assertEquals("Alice", user.getName());
user.setName("Bob");
assertEquals("Bob", user.getName());
}
@Test
void testSetNameInvalid() {
User user = new User("Alice", "alice@example.com");
assertThrows(IllegalArgumentException.class, () -> user.setName(""));
}
}
This unit test ensures our methods are adhering to the expected behavior, preventing future changes from inadvertently breaking existing functionality.
Key Takeaways
Transforming legacy code often poses significant challenges, particularly regarding accessor methods. By prioritizing domain logic, using immutable objects, and avoiding direct property access, you can create a cleaner and more maintainable structure while ensuring the integrity of your application.
Remember to validate your changes through thorough testing. Embracing these practices will not only improve your codebase but also enhance the overall software development process.
If you're navigating the world of legacy code, tools like Refactoring.Guru can provide valuable insights into common refactoring techniques and principles to further aid in your journey.
Keep iterating on your codebase, and welcome the continuous improvements that come with a clear structure. Happy coding!