Common Pitfalls When Using Google Gson: Avoid These Mistakes!
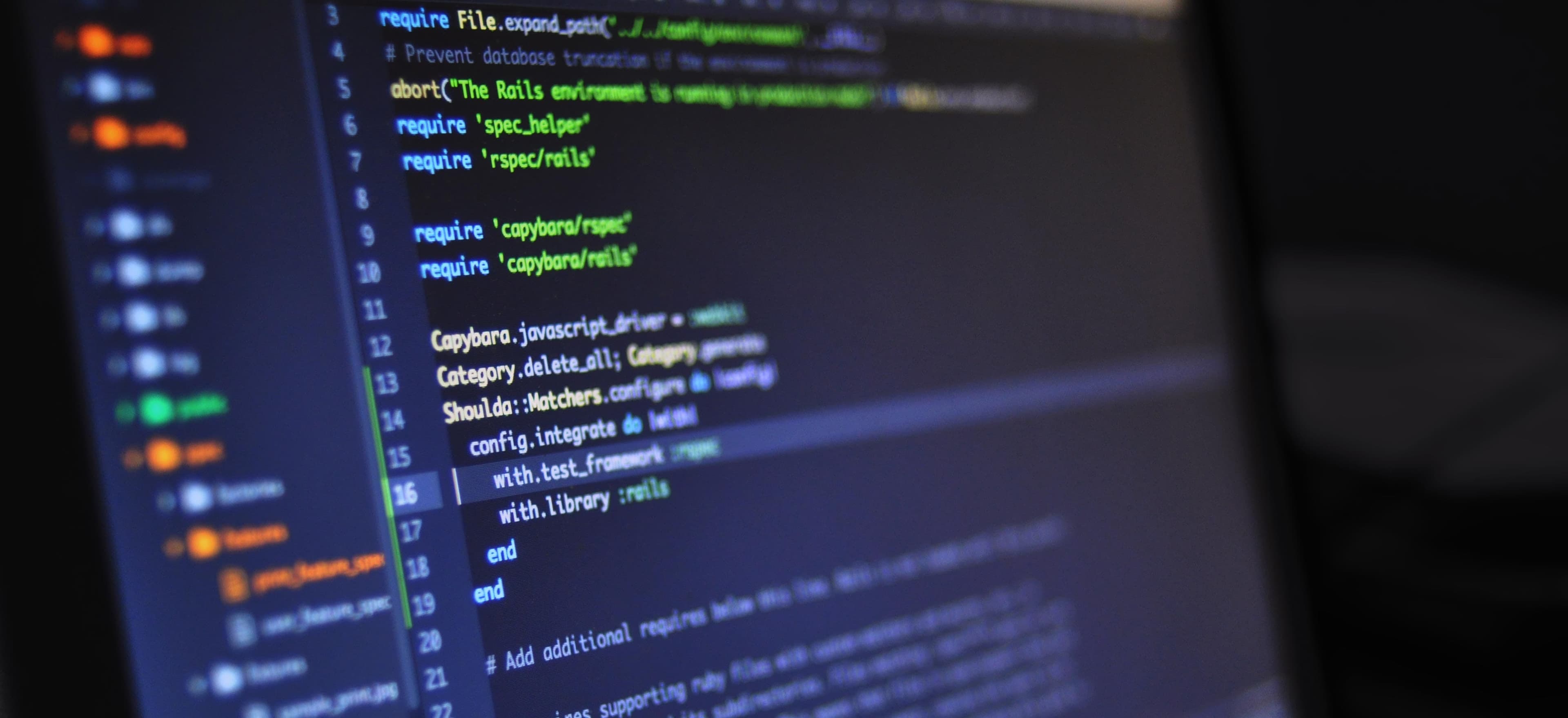
- Published on
Common Pitfalls When Using Google Gson: Avoid These Mistakes!
Google Gson is a powerful library that offers a simple way to convert Java objects to JSON and vice versa. However, even experienced developers can stumble upon certain pitfalls while using Gson. In this blog post, we will explore some common mistakes, providing illustrative examples and actionable solutions to help you avoid them.
Table of Contents
- Understanding Gson Basics
- Pitfall 1: Ignoring Nulls
- Pitfall 2: Incorrect Type Handling
- Pitfall 3: Using Raw Types
- Pitfall 4: Missing Default Constructor
- Pitfall 5: Complex Nested Objects
- Conclusion
Understanding Gson Basics
Before we dive into the pitfalls, let’s establish a foundational understanding of how Gson works. Gson allows Java developers to serialize (convert) and deserialize (parse) Java objects. Here’s how you can initiate Gson in your project.
import com.google.gson.Gson;
public class JsonExample {
public static void main(String[] args) {
Gson gson = new Gson();
// Java object
User user = new User("John Doe", 30);
// Serialize to JSON
String json = gson.toJson(user);
System.out.println(json);
}
}
class User {
String name;
int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
}
In the example above, we define a simple User
class and use Gson to convert it to JSON. Keep these basics in mind as we investigate specific pitfalls.
Pitfall 1: Ignoring Nulls
One common mistake developers make is misunderstanding how Gson treats null values. By default, Gson omits null fields from the output JSON. While this may seem convenient, it can lead to unexpected behavior in applications that rely on consistent JSON structure.
class User {
String name;
Integer age;
public User(String name, Integer age) {
this.name = name;
this.age = age;
}
}
// Serialization with null values
User user = new User(null, null);
String json = gson.toJson(user); // Outputs: {}
Solution
To ensure null values are included, you can configure Gson like this:
Gson gson = new GsonBuilder().serializeNulls().create();
This will change the output to:
{"name":null,"age":null}
Pitfall 2: Incorrect Type Handling
Gson performs type conversion based on the declared data type. Mixing up primitive types and their wrapper classes can lead to undesired outcomes. Consider this example:
class User {
String name;
int age; // Primitive type
public User(String name, int age) {
this.name = name;
this.age = age;
}
}
User user = new User("John", 30);
String json = gson.toJson(user); // Works fine
But if you switch the age
to Integer:
class User {
String name;
Integer age; // Wrapper class
public User(String name, Integer age) {
this.name = name;
this.age = age;
}
}
Deserialization might become problematic:
String json = "{\"name\":\"John\", \"age\":30}";
User user = gson.fromJson(json, User.class); // Works, but what if age is null?
Solution
Be careful with your data types. Ensure that the input JSON and the Java object structure match correctly. Utilize the Optional
class where applicable.
Pitfall 3: Using Raw Types
Another frequent mistake is using raw types when dealing with generic collections. This can lead to runtime exceptions. For example:
class User {
String name;
List<String> friends;
public User(String name, List<String> friends) {
this.name = name;
this.friends = friends;
}
}
String json = "{\"name\":\"Jane\", \"friends\":[\"Alice\", \"Bob\"]}";
User user = gson.fromJson(json, User.class); // This works fine
// But what if we were using raw types?
List rawList = new ArrayList();
rawList.add("Friend");
User userWithRawList = new User("Alice", rawList); // This can be problematic
Solution
Always specify the generic type when using collections. This prevents the potential for type mismatch, ensuring that Gson can properly serialize and deserialize your data.
List<String> friends = new ArrayList<>();
Pitfall 4: Missing Default Constructor
Gson requires a no-argument constructor for deserialization. If you forget to create one, you will encounter exceptions.
class User {
String name;
int age;
// Missing default constructor
public User(String name, int age) {
this.name = name;
this.age = age;
}
}
Solution
Always add a no-argument constructor to your classes, especially if you plan to use Gson for deserialization.
class User {
String name;
int age;
public User() {} // Default constructor
public User(String name, int age) {
this.name = name;
this.age = age;
}
}
Pitfall 5: Complex Nested Objects
Complex nested objects can easily trip up developers, especially when they're not adhering to consistent structure. If you have nested objects, you might end up with broken JSON if you're not careful.
class Address {
String city;
String country;
// Constructor and Getters ommitted for brevity
}
class User {
String name;
Address address;
// Constructor and Getters ommitted for brevity
}
// Serialization
Address address = new Address("New York", "USA");
User john = new User("John Doe", address);
String json = gson.toJson(john); // Works fine
// But deserialization can fail if the JSON structure is inconsistent
Solution
Make sure your JSON structure matches the expected structure of your Java objects. Using tools like jsonschema2pojo can help create Java classes from JSON schema.
Lessons Learned
Gson is a fantastic tool that simplifies JSON handling within Java applications. However, being mindful of common pitfalls can save you time and frustration. Whether handling nulls, type issues, or nested objects, ensuring the correct implementation will lead to more robust code.
By following the practices discussed above, you will be in a solid position to utilize Gson effectively. For more in-depth functionalities and features, check out the Gson Documentation.
With a little care and attention, you can fully harness the power of Google Gson in your Java projects, avoiding potential pitfalls and ensuring seamless serialization and deserialization of your data.
Happy coding!
Checkout our other articles