Mastering Lucene: A Beginner's Guide to Common Pitfalls
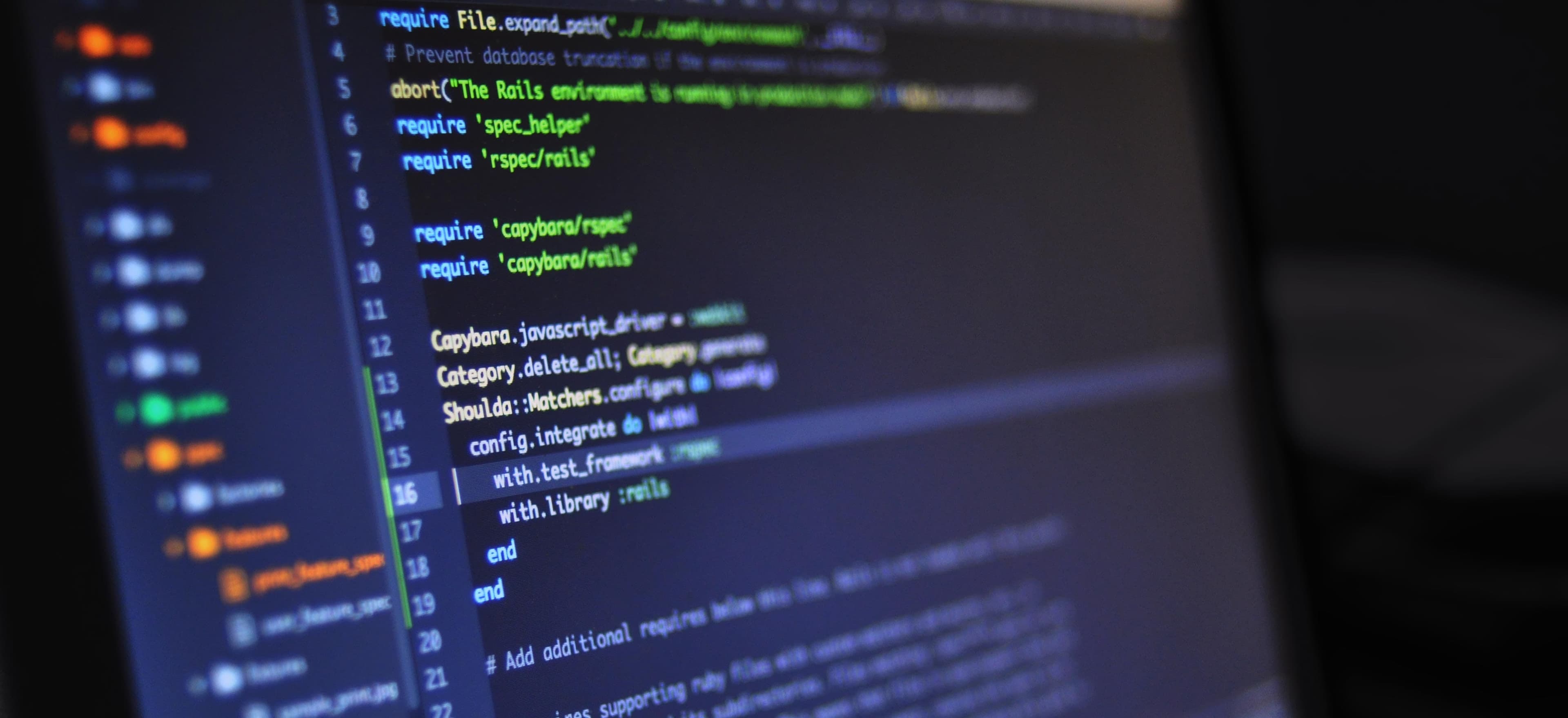
- Published on
Mastering Lucene: A Beginner's Guide to Common Pitfalls
Apache Lucene is a powerful search library widely used for full-text indexing and searching. However, as with any complex technology, beginners often face pitfalls that can hinder their understanding and implementation. In this guide, we aim to explore some of the common challenges faced when using Lucene and provide practical advice to navigate them effectively.
Table of Contents
- Understanding Lucene Basics
- Common Pitfalls
- Not Understanding Indexing
- Misconfigured Analyzers
- Ignoring Performance Optimization
- Effective Querying Strategies
- Best Practices
- Conclusion
Understanding Lucene Basics
Before diving into the common pitfalls, it is crucial to understand what Lucene is and how it fits into search applications. Lucene is a Java-based indexing and search library that provides the fundamental tools for searching large text datasets efficiently. The library employs various components, including indexes, documents, fields, and analyzers, to build an optimized search engine.
Important Components:
- Index: The core structure where your documents are stored.
- Document: Represents a collection of fields, much like a row in a database.
- Field: A single attribute of a document, which may be searchable or stored.
- Analyzer: Processes text and breaks it into tokens for indexing.
To get started with basic Lucene indexing, you might use the following code snippet:
import org.apache.lucene.analysis.standard.StandardAnalyzer;
import org.apache.lucene.document.Document;
import org.apache.lucene.document.Field;
import org.apache.lucene.document.StringField;
import org.apache.lucene.index.IndexWriter;
import org.apache.lucene.index.IndexWriterConfig;
import org.apache.lucene.store.Directory;
import org.apache.lucene.store.RAMDirectory;
public class SimpleIndexing {
public static void main(String[] args) throws Exception {
// Create a directory to store the index temporarily.
Directory directory = new RAMDirectory();
// Analyze text to tokenize it during indexing.
StandardAnalyzer analyzer = new StandardAnalyzer();
// Set up the index writer configuration.
IndexWriterConfig config = new IndexWriterConfig(analyzer);
IndexWriter writer = new IndexWriter(directory, config);
// Create a new document with fields.
Document doc = new Document();
doc.add(new StringField("title", "Lucene Introduction", Field.Store.YES));
doc.add(new StringField("author", "Author Name", Field.Store.YES));
// Add the document to the index.
writer.addDocument(doc);
// Commit and close the writer.
writer.close();
directory.close();
}
}
Why This Code Matters
This snippet simplifies the indexing process by demonstrating how to create a document, set fields, and write to an index. It's crucial for beginners to understand that without properly indexing data, querying it later will yield no results.
Common Pitfalls
While Lucene is immensely flexible, beginners often encounter several pitfalls. Below are key areas to be aware of.
Not Understanding Indexing
One major misconception is that simply adding documents to the index guarantees successful search queries. The reality is that you need to understand the anatomy of your documents and how they will be queried.
Solution:
- Always analyze your documents before indexing.
- Ensure that fields are correctly marked as searchable or stored based on your needs.
Misconfigured Analyzers
An analyzer’s job is to process text, so using the wrong one can drastically affect the search results. For instance, if an analyzer does not segment words correctly, your search will yield unexpected results.
import org.apache.lucene.analysis.Analyzer;
import org.apache.lucene.analysis.en.EnglishAnalyzer;
Analyzer analyzer = new EnglishAnalyzer(); // using EnglishAnalyzer for English text
Why It Matters
Using a specific analyzer like EnglishAnalyzer
ensures that your text is analyzed contextually. Failure to configure analyzers properly can lead to undesired queries.
Ignoring Performance Optimization
Lucene is built for speed, but neglecting its architectural principles can lead to poor performance. Beginners often create one large index without optimizing it for specific queries or using features like caching.
Solution:
- Use segment merging and commit strategies to keep your index performant.
Effective Querying Strategies
Understanding how Lucene queries work is equally vital. Common pitfalls include making overly complex queries or failing to consider query types.
Basic Query Example:
import org.apache.lucene.queryparser.classic.QueryParser;
import org.apache.lucene.search.IndexSearcher;
import org.apache.lucene.search.Query;
import org.apache.lucene.store.Directory;
import org.apache.lucene.store.RAMDirectory;
Directory directory = new RAMDirectory();
IndexSearcher searcher = new IndexSearcher(directory);
// Using QueryParser for user-friendly text queries
QueryParser parser = new QueryParser("title", analyzer);
Query query = parser.parse("Lucene");
Why understand Queries?
Lucene supports different query types, and knowing which to apply can significantly affect your results—be it a phrase query, boolean query, or range query.
Best Practices
To ensure success with Lucene, follow these best practices:
-
Regularly Review Analyzers: As your data changes, so should your approach to analysis.
-
Commit Frequently: Make sure to commit your index often to avoid losing data. Use a reasonable buffer size for queued documents.
-
Test Queries: Always test your queries with common data conditions to ensure they yield expected results.
-
Use Monitoring Tools: Employ monitoring tools to gather metrics on search performance and index usage.
In Conclusion, Here is What Matters
While Apache Lucene offers robust features for implementing search functionality, the journey can be filled with challenges for beginners. The key is to understand the structure you're working with, be cautious with analyzers, and always be mindful of performance impact.
For additional learning, consider the following resources:
By equipping yourself with this knowledge and avoiding the common pitfalls outlined above, you'll be better prepared to harness the full potential of Apache Lucene in your projects. Happy coding!
Checkout our other articles