Common Pitfalls in Spring-JSF Integration and How to Avoid Them
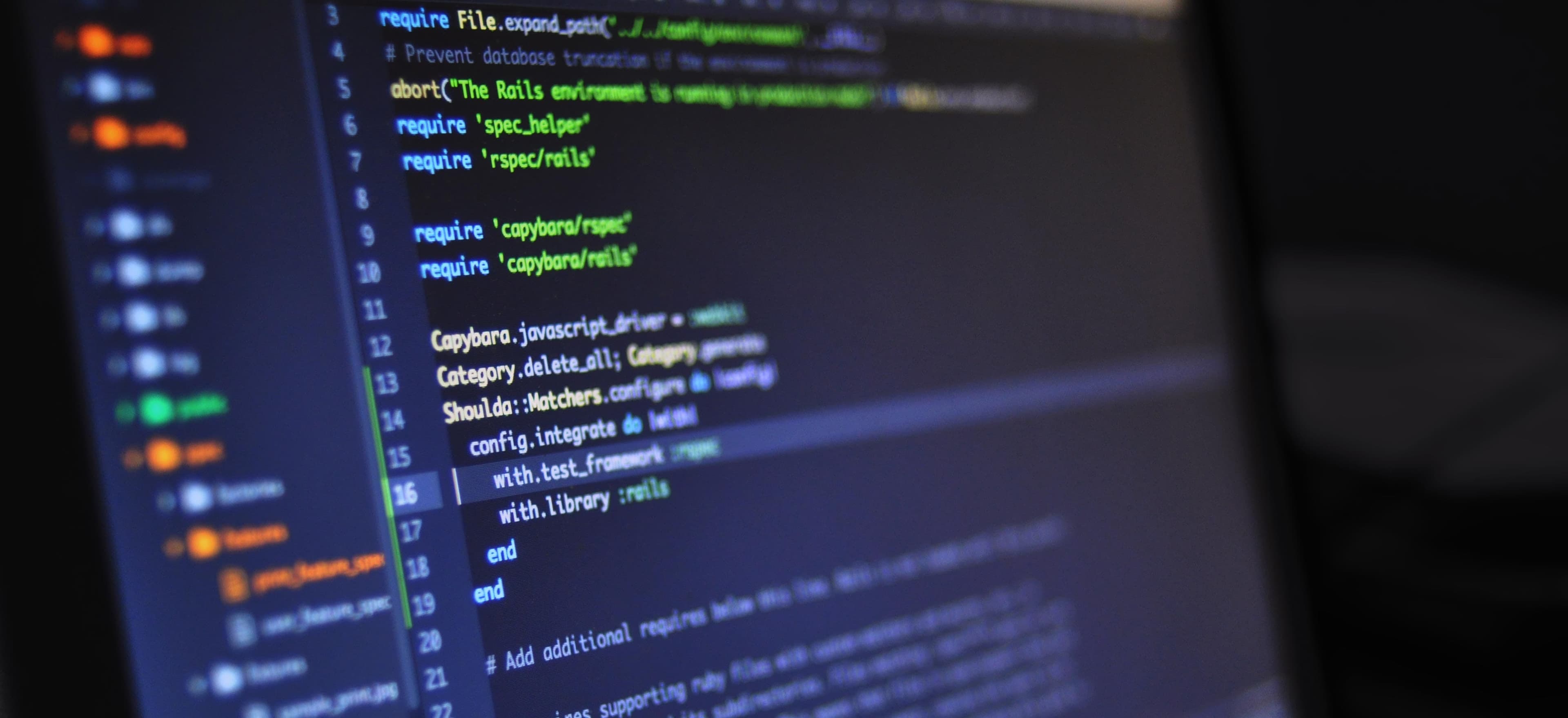
- Published on
Common Pitfalls in Spring-JSF Integration and How to Avoid Them
JavaServer Faces (JSF) is a popular framework for building web applications in Java, while Spring Framework is widely recognized for its powerful functionalities in developing enterprise-level applications. Combining these two can lead to a powerful and efficient application architecture. However, integrating Spring and JSF can come with its own set of challenges. In this blog post, we’ll explore some common pitfalls in Spring-JSF integration and how to avoid them.
Table of Contents
- Introduction to Spring and JSF
- Pitfall 1: Improper Configuration
- Pitfall 2: Bean Scope Confusion
- Pitfall 3: URL Handling Issues
- Pitfall 4: AJAX Compatibility Problems
- Conclusion
1. Introduction to Spring and JSF
Spring provides a comprehensive programming and configuration model for Java applications, while JSF is designed to simplify the development integration of web applications. When integrating the two, developers often seek the benefits of Spring’s dependency injection and transaction management alongside JSF’s component-based UI framework.
To facilitate their integration effectively, it's essential to understand both frameworks thoroughly and how they can complement each other.
2. Pitfall 1: Improper Configuration
One of the most prevalent pitfalls in Spring-JSF integration is improper or missing configuration. This can lead to a myriad of issues, including rendering failures and bean inconsistency.
Solution
Ensure that both Spring and JSF configurations are properly set up in the web.xml
and through Java configuration.
Example web.xml
configuration:
<servlet>
<servlet-name>FacesServlet</servlet-name>
<servlet-class>javax.faces.webapp.FacesServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>FacesServlet</servlet-name>
<url-pattern>*.xhtml</url-pattern>
</servlet-mapping>
Why This Matters
By specifying the FacesServlet
in your web.xml
, you're enabling JSF to handle the navigation of your JavaServer Pages. Configuring it correctly ensures that requests to your XHTML pages are correctly routed, preventing rendering errors and exceptions.
3. Pitfall 2: Bean Scope Confusion
Another common mistake is mismanagement of bean scopes between Spring and JSF. JSF beans can have different scopes such as @RequestScoped
, @ViewScoped
, and @SessionScoped
, while Spring's beans have their own lifecycle managed by the Spring IoC container. This can lead to confusion regarding the lifecycle of beans, especially when using them together.
Solution
When integrating, clearly define the scopes of your beans in both frameworks. Here’s an example:
import javax.faces.bean.ManagedBean;
import javax faces.bean.ViewScoped;
@ManagedBean
@ViewScoped
public class UserProfile {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Why This Matters
By defining the right scopes, you ensure that the beans live as long as needed and are disposed of properly, effectively managing memory and lifecycle, which is essential for a responsive application.
4. Pitfall 3: URL Handling Issues
A frequent issue developers face is URL handling, especially navigating between different views or handling JSF’s URL rewriting capabilities. In many cases, developers may expect that Spring’s routing and JSF’s view handling will seamlessly work together, but that's often not the case.
Solution
To address this, utilize Spring’s @Controller
and configure URLs carefully in tandem with JSF views.
For example, declaring a Spring controller:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class HomeController {
@RequestMapping("/home")
public String home() {
return "home"; // Returns home.xhtml
}
}
Why This Matters
By explicitly mapping your URLs in Spring, it makes handling navigation easier and provides a clearer route that avoids conflicts with JSF's navigation rules. This prevents routing errors which may cause a poor user experience.
5. Pitfall 4: AJAX Compatibility Problems
AJAX can greatly enhance user experience by allowing updates to parts of the web page without requiring a complete page refresh. However, JSF has its own set of complexities when dealing with AJAX requests, especially when combined with Spring-managed beans.
Solution
Make sure to use the @FacesConverter
and @FacesValidator
annotations properly, and configure AJAX calls correctly.
Example of an AJAX call in JSF:
<h:commandButton value="Submit" action="#{userProfile.submit}">
<f:ajax execute="@form" render="messages" />
</h:commandButton>
<h:messages id="messages" />
Why This Matters
With proper AJAX integration, you can greatly enhance the responsiveness of your application. Misconfigurations could lead to missed updates or incomplete requests, making AJAX less effective or outright failing to work.
6. Conclusion
Integrating Spring and JSF can yield a robust and dynamic web application if approached correctly. By being aware of the common pitfalls—improper configuration, bean scope confusion, URL handling issues, and AJAX compatibility problems—you can better navigate potential challenges.
By following best practices and leveraging both frameworks’ strengths, you can create highly effective and user-friendly applications.
For further reading on more advanced topics in Spring and JSF, check the following resources:
Integrate wisely, and enjoy the best of both worlds in your Java application development!