Mastering App Widgets: Overcoming Common Development Hurdles
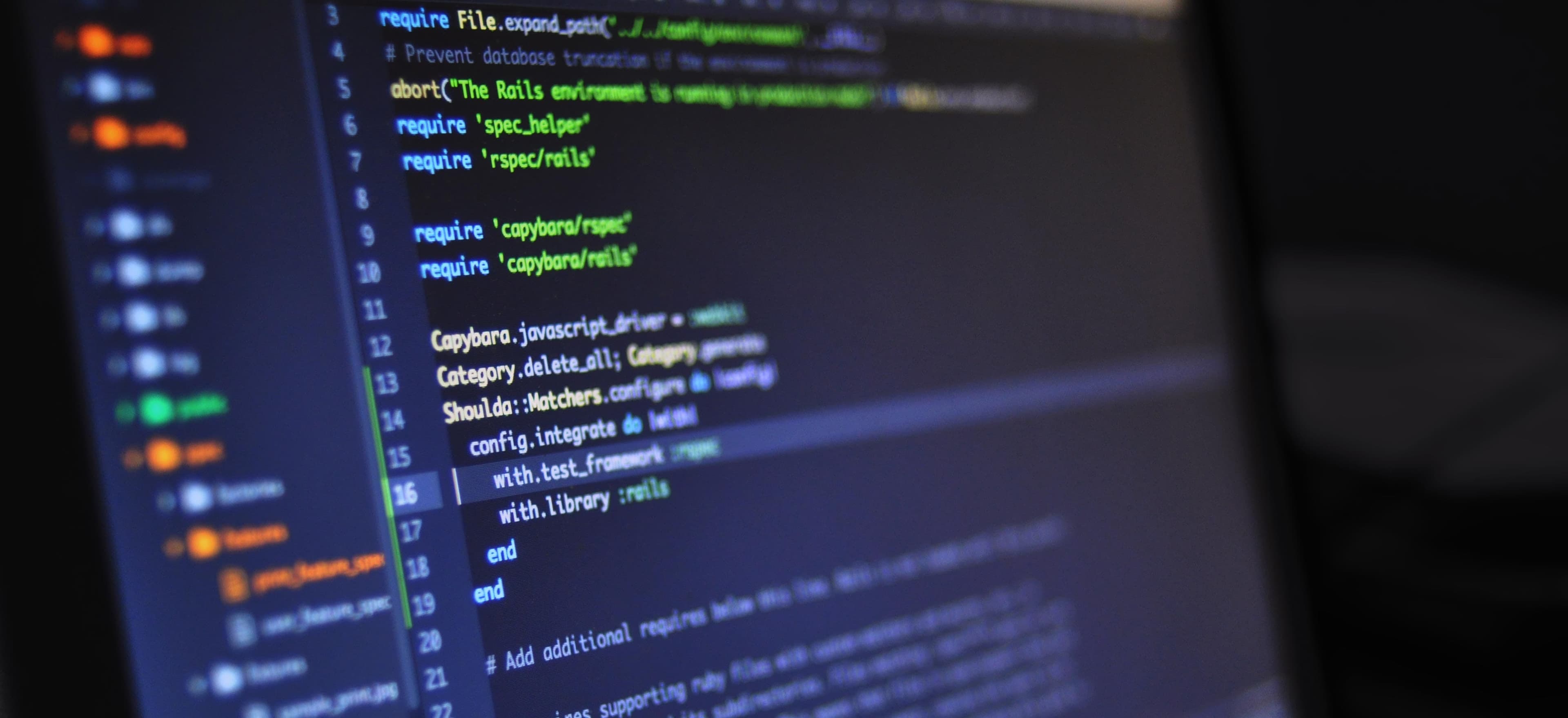
- Published on
Mastering App Widgets: Overcoming Common Development Hurdles
In the fast-evolving world of Android app development, app widgets stand out as powerful tools for user engagement. They provide the ability to create interactive and context-aware components that deliver important information right on the user's home screen. However, while widgets can significantly enhance user experience, they often come with their share of challenges. In this post, we will explore common app widget development hurdles and how to overcome them.
Understanding App Widgets
Before diving into the challenges of widget development, let’s clarify what app widgets are. Android app widgets are mini-apps that run on the home screen or the lock screen. These widgets allow users to access key functionalities of your app without needing to open it. They can display information, provide shortcuts, or support user interaction directly on the home screen.
Key Widget Features
- Interactivity: Users can interact with widgets by clicking to open the app or performing actions.
- Updates: Widgets can update their content dynamically based on user preferences or app data.
- Customization: Widgets can be resized and customized to fit user preferences.
Common Development Hurdles
While app widgets are a valuable addition to any Android application, developers face several hurdles when creating them:
- Layout Limitations
- Updating the Widget
- Handling User Interaction
- Memory Constraints
- Version Compatibility
Let’s dissect these challenges one by one.
1. Layout Limitations
Creating a visually appealing widget often comes with layout constraints. Unlike traditional activities, widgets have a much stricter layout system. Developers may find themselves struggling to fit all desired elements into the widget without it becoming cluttered.
Solution: Utilize RemoteViews
To manage layout constraints effectively, leveraging RemoteViews
is essential. RemoteViews
allows for the creation of a custom layout that can be applied to the widget. Here’s a simple example demonstrating how to implement a basic layout:
RemoteViews views = new RemoteViews(context.getPackageName(), R.layout.widget_layout);
views.setTextViewText(R.id.widget_title, "Hello, Widget!");
Why this works: By using RemoteViews
, you are able to define a consistent layout for your app widget, using the standard Android layout system for UI design, while working within the constraints set by the widget itself.
2. Updating the Widget
App widgets need to update regularly to provide users with fresh content. However, managing these updates can be tricky, particularly with system limitations on how frequently widgets can refresh.
Solution: Use AppWidgetManager
Using the AppWidgetManager
class allows you to efficiently update widget views. Here's how you can manage periodic updates:
public void updateWidget(Context context, AppWidgetManager appWidgetManager, int appWidgetId) {
RemoteViews views = new RemoteViews(context.getPackageName(), R.layout.widget_layout);
views.setTextViewText(R.id.widget_title, "Updated Content");
appWidgetManager.updateAppWidget(appWidgetId, views);
}
Why this works: By calling updateAppWidget
, you can send the new updates to the widget layout. Make sure to consider using AlarmManager
or JobScheduler
for periodic updates based on your requirements.
3. Handling User Interaction
Managing user interactivity is another common challenge when working with widgets. Developers need to ensure that touch events lead to the expected behavior, which can sometimes lead to complex logic.
Solution: Set Up PendingIntents
To handle interactions like button clicks, leverage PendingIntents
. Here’s a brief example:
Intent intent = new Intent(context, YourActivity.class);
PendingIntent pendingIntent = PendingIntent.getActivity(context, 0, intent, 0);
views.setOnClickPendingIntent(R.id.widget_button, pendingIntent);
Why this works: By tying user actions (like button clicks) to a PendingIntent
, you ensure that your widget interacts seamlessly with the rest of your app, directing users to appropriate activities or actions.
4. Memory Constraints
Widgets must operate with limited memory and processing power. This can result in difficulties when dealing with rich or dynamic content.
Solution: Optimize Resource Usage
Always ensure you're optimizing your widget by limiting the size and resolution of images and other resources. Use pay attention to how much data your widget is using. For instance:
BitmapFactory.Options options = new BitmapFactory.Options();
options.inSampleSize = 2; // Reduce the image size
Bitmap bitmap = BitmapFactory.decodeResource(getResources(), R.drawable.image, options);
views.setImageViewBitmap(R.id.widget_image, bitmap);
Why this works: By downsizing images before they are rendered in a widget, you minimize memory use without sacrificing quality.
5. Version Compatibility
With each Android version, there can be changes in library functions and UI capabilities. Ensure that your widgets maintain compatibility across varying versions of Android can be challenging.
Solution: Gradual Adoption of New APIs
Use Android’s compatibility libraries and check version compatibility before using specific features. Wrap newer API functionalities in conditional constructs to ensure the app runs smoothly.
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
// Use newer APIs available from Android Oreo onwards
}
Why this works: This ensures that your widget does not crash on earlier versions of Android while still embracing improvements available in newer ones.
Best Practices for Developing App Widgets
- Keep It Simple: The more complex the widget, the more prone it is to issues. Focus on delivering a few functionalities that enhance user experience.
- Test Extensively: Test the widget on various devices and screen sizes to ensure it looks and performs as expected.
- Monitor and Optimize: Use analytics to monitor widget interactions and optimize based on user feedback.
A Final Look
Developing Android app widgets can be a rewarding yet challenging task. By understanding the common hurdles, you can better prepare to tackle them head-on. Remember to optimize layouts, manage updates effectively, handle user interactions smoothly, conserve memory, and ensure version compatibility.
For more on mastering Android development, consider checking out the official Android developers' documentation and Android Developer Blog. These resources are continually updated with the latest best practices and tools to help developers succeed.
With these insights, tools, and strategies at your disposal, you are now well-equipped to create stunning, functional, and effective app widgets that enhance user engagement and satisfaction. Happy coding!
Checkout our other articles