Mastering Batch Deletes: Speed Up Your JPA and Hibernate Queries
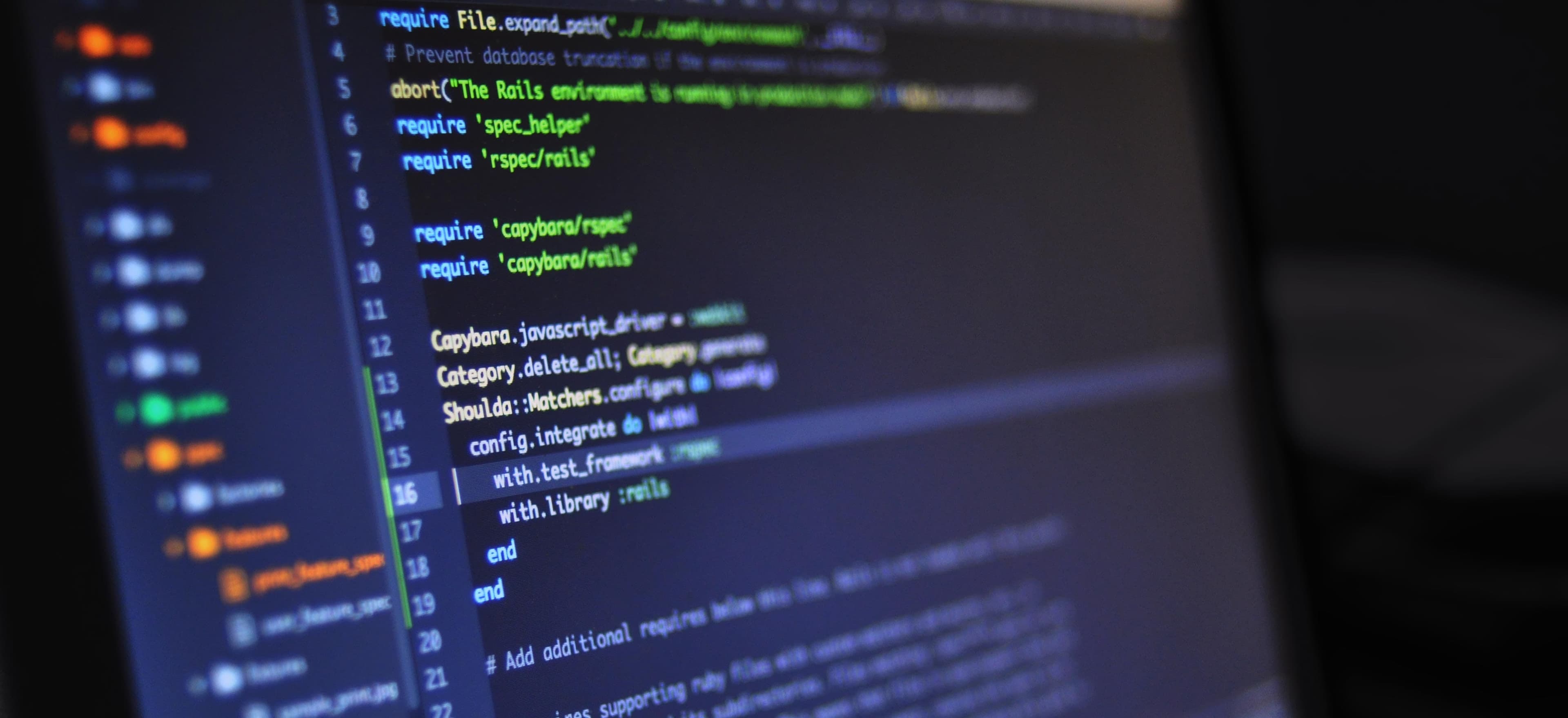
- Published on
Mastering Batch Deletes: Speed Up Your JPA and Hibernate Queries
Batch processing is an essential concept in data management, particularly when it comes to eliminating large datasets efficiently. This approach is invaluable in environments where performance and resource management are critical. In this blog post, we'll delve into the art of mastering batch deletes in JPA and Hibernate, offering insights, code examples, and optimization techniques that can enhance your applications.
Understanding JPA and Hibernate
Java Persistence API (JPA) is a specification for accessing, persisting, and managing data between Java objects and databases. Hibernate is one of the most popular implementations of JPA. By providing a robust framework for handling CRUD (Create, Read, Update, Delete) operations, Hibernate allows developers to manage database interactions with minimal boilerplate code.
The Importance of Batch Processing
When you need to delete a significant number of records from a database, executing multiple DELETE statements sequentially can lead to performance bottlenecks. Every DELETE call typically triggers a transaction, which adds overhead in terms of processing time and resources used.
Benefits of Batch Deletes
-
Reduced Network Round Trips: Instead of sending individual SQL statements for each delete operation, batching them reduces the number of calls made to the database.
-
Transaction Overhead Mitigation: By bundling multiple DELETE commands into a single transaction, you minimize the associated overhead.
-
Improved Performance: On large datasets, the performance gain can be significant, allowing operations to complete much faster.
Setting Up Your JPA Environment
To demonstrate batch deletes, ensure you have the following setup in your Java application:
- Hibernate ORM: This example is based on Hibernate, but the concepts apply to any JPA implementation.
- DataSource Configuration: Ensure your data source is set up correctly in your
persistence.xml
or through Spring configuration.
Here’s an example of what your persistence.xml
might look like:
<persistence xmlns="http://xmlns.jcp.org/xml/ns/persistence"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/persistence
http://xmlns.jcp.org/xml/ns/persistence/persistence_2_1.xsd"
version="2.1">
<persistence-unit name="myUnit">
<provider>org.hibernate.jpa.HibernatePersistenceProvider</provider>
<class>com.example.MyEntity</class>
<properties>
<property name="javax.persistence.jdbc.driver" value="org.h2.Driver"/>
<property name="javax.persistence.jdbc.url" value="jdbc:h2:mem:testdb"/>
<property name="javax.persistence.jdbc.user" value="sa"/>
<property name="javax.persistence.jdbc.password" value=""/>
<property name="hibernate.hbm2ddl.auto" value="update"/>
<property name="hibernate.dialect" value="org.hibernate.dialect.H2Dialect"/>
<property name="hibernate.jdbc.batch_size" value="50"/>
</properties>
</persistence-unit>
</persistence>
Batch Size Configuration
In the example above, we set hibernate.jdbc.batch_size
to 50
. This means that Hibernate will group up to 50 DELETE statements and execute them in a single batch. Adjust this number based on your database capabilities and application requirements for optimal performance.
Implementing Batch Deletes
Here’s how you can implement batch deletes in a straightforward manner:
import javax.persistence.EntityManager;
import javax.persistence.EntityTransaction;
import javax.persistence.Persistence;
public class BatchDeleteExample {
private EntityManager entityManager;
public BatchDeleteExample() {
this.entityManager = Persistence.createEntityManagerFactory("myUnit").createEntityManager();
}
public void deleteEntities(List<Long> idsToDelete) {
EntityTransaction transaction = entityManager.getTransaction();
transaction.begin();
int batchSize = 50;
for (int i = 0; i < idsToDelete.size(); i++) {
Long id = idsToDelete.get(i);
MyEntity entity = entityManager.find(MyEntity.class, id);
if (entity != null) {
entityManager.remove(entity);
}
if (i % batchSize == 0 && i > 0) {
entityManager.flush();
entityManager.clear();
}
}
transaction.commit();
}
}
Code Breakdown
- EntityManager: This component is responsible for managing the persistence context. We use it to find and remove entities.
- Transactions: JPA operations require transactions. We begin a transaction at the start of our delete operation and commit it at the end.
- Batching Logic: The key part of this code is the conditional flush and clear inside the loop:
- flush(): This method forces Hibernate to execute the changes to the database.
- clear(): This method detaches all entities from the persistence context, freeing up resources.
By invoking flush()
and clear()
, we mitigate the memory impact of potentially large collections of entities.
Handling Exceptions
When working with batch operations, handling exceptions is crucial. Ensure that you implement appropriate try-catch blocks and always roll back the transaction in case of errors.
public void deleteEntities(List<Long> idsToDelete) {
EntityTransaction transaction = entityManager.getTransaction();
transaction.begin();
try {
int batchSize = 50;
for (int i = 0; i < idsToDelete.size(); i++) {
Long id = idsToDelete.get(i);
MyEntity entity = entityManager.find(MyEntity.class, id);
if (entity != null) {
entityManager.remove(entity);
}
if (i % batchSize == 0 && i > 0) {
entityManager.flush();
entityManager.clear();
}
}
transaction.commit();
} catch (Exception e) {
if (transaction.isActive()) {
transaction.rollback(); // Ensure transaction rollback on failure
}
e.printStackTrace();
}
}
Key Points on Exception Handling
- Rollback: Always rollback your transaction to avoid partial deletes that can lead to data inconsistency.
- Logging: Logging your exceptions can significantly help in debugging potential issues that arise during batch processing.
Performance Considerations
- Batch Size Tuning: Experiment with different batch sizes to find the balance that best suits your database and use case. A size too small might not yield significant performance benefits, while a size too large can lead to memory issues.
- Entity State Management: Frequent flushing and clearing are essential, especially for large datasets, to prevent memory bloat.
- Database Settings: Some databases have specific optimizations for batch processing that may require additional configuration.
Bringing It All Together
Implementing batch deletes in your JPA and Hibernate applications is a surefire way to enhance performance and resource management. By reducing the frequency of database calls and handling units of work in batches, you will notice significant improvements, especially when dealing with large datasets.
For more advanced optimizations and to further improve your application's efficiency, consider exploring the official Hibernate Documentation.
Stay tuned for more insights and tips on mastering Java Persistence today! Investing time in learning these techniques will pay dividends in the long run as your applications scale.
This blog aims to provide a comprehensive understanding and guide for implementing batch deletes in concise, manageable syntax. By following these steps and considerations, you can optimize your JPA and Hibernate queries effectively.