Common JSON Parsing Errors and How to Fix Them
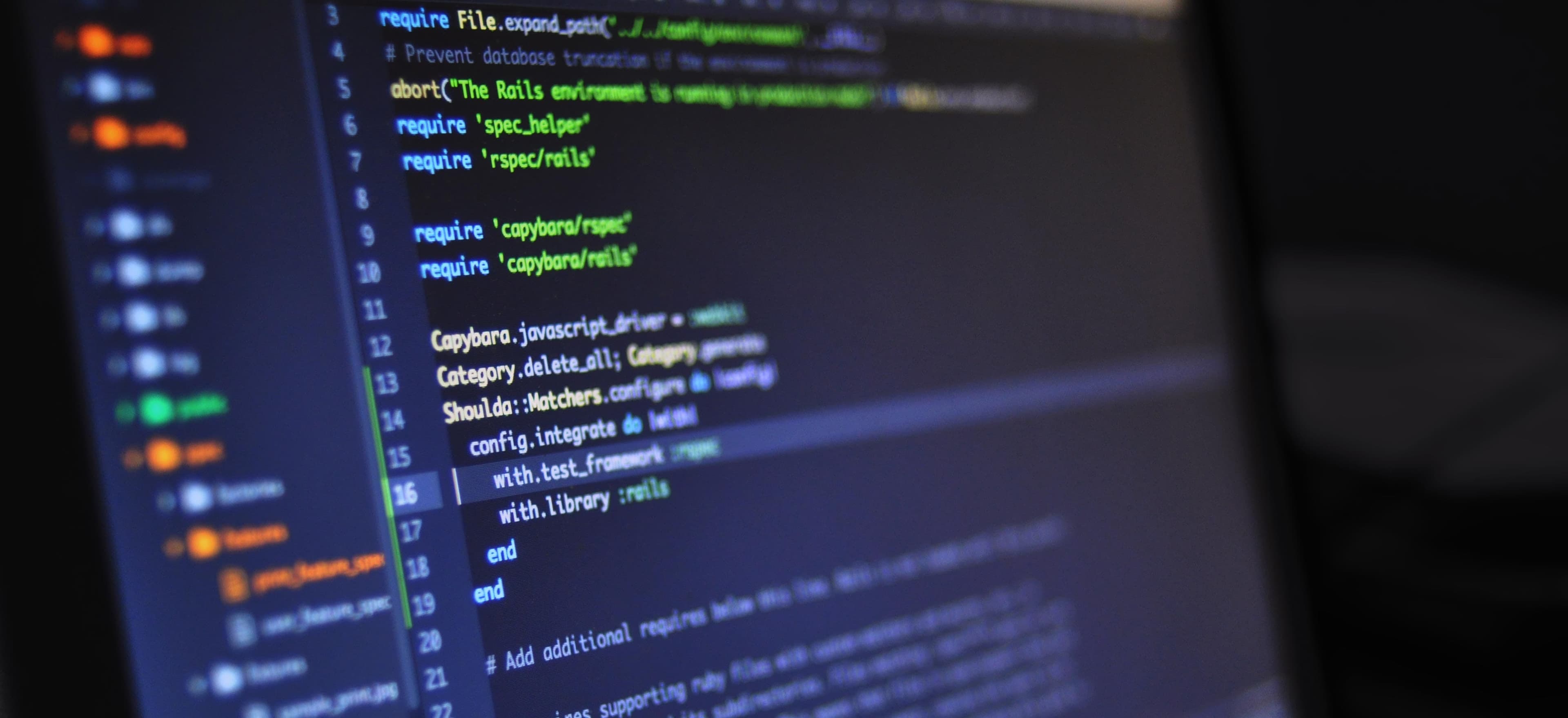
- Published on
Common JSON Parsing Errors and How to Fix Them
Java developers frequently work with JSON (JavaScript Object Notation) data, typically in web applications or APIs. JSON is popular due to its lightweight nature and ease of reading/writing. However, parsing JSON can lead to various errors that can disrupt applications. Understanding these errors, their causes, and how to fix them is vital for smooth development and deployment. In this blog post, we'll identify common JSON parsing errors in Java, provide code snippets, and discuss how to solve them effectively.
What is JSON?
Before diving into errors, let's briefly explore what JSON is. JSON is a data format that uses a text-based structure to represent data objects and arrays. Its syntax is quite simple and readable, using key-value pairs to store data.
Here's an example of a JSON object:
{
"name": "John Doe",
"age": 30,
"isDeveloper": true,
"skills": ["Java", "JavaScript", "Python"],
"address": {
"city": "New York",
"country": "USA"
}
}
Java JSON Libraries
In Java, common libraries for parsing JSON include:
- Jackson: A powerful data processing tool.
- Gson: Created by Google, it's easy to use.
- org.json: A straightforward library for handling JSON.
We'll focus mostly on Jackson, as it is widely used and offers robust features.
To include Jackson in your Maven project, add the following dependency:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.13.0</version>
</dependency>
Common JSON Parsing Errors
1. Malformed JSON Syntax
Error Summary: Malformed JSON is one of the most common issues when working with JSON data. Typical signs include missing commas, improper quotes, or unmatched brackets.
Example: Here's an example of malformed JSON:
{
"name": "John Doe"
"age": 30
}
Fix: Make sure your JSON data adheres to proper syntax. Correct the missing comma.
Correct JSON:
{
"name": "John Doe",
"age": 30
}
Java Code Snippet:
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonParsingExample {
public static void main(String[] args) {
String jsonString = "{\"name\": \"John Doe\" \"age\": 30}"; // Malformed JSON example
ObjectMapper objectMapper = new ObjectMapper();
try {
Person person = objectMapper.readValue(jsonString, Person.class);
} catch (Exception e) {
System.out.println("Failed to parse JSON: " + e.getMessage());
}
}
}
class Person {
public String name;
public int age;
}
2. Incorrect Data Type
Error Summary: JSON has specific data types (string, number, boolean, array, object, null). When you try to parse a number as a string or vice versa, it leads to errors.
Example:
{
"name": "John Doe",
"age": "30" // Age should be an integer
}
Fix: Ensure that the data types in your JSON match the expected Java class types.
Correct JSON:
{
"name": "John Doe",
"age": 30
}
Java Code Snippet:
String jsonString = "{\"name\": \"John Doe\", \"age\": \"30\"}"; // Incorrect data type for age
try {
Person person = objectMapper.readValue(jsonString, Person.class);
} catch (Exception e) {
// This will throw a formatting error because age is expected to be an int
System.out.println("Failed to parse JSON: " + e.getMessage());
}
3. Unrecognized Fields
Error Summary: When your JSON contains fields that do not match the fields of your Java class, you may run into inconsistencies.
Example:
{
"name": "John Doe",
"age": 30,
"height": 175 // Height field does not exist in the Person class
}
Fix: Ensure your Java class matches the JSON structure. You can ignore unknown fields using annotations.
Java Code Snippet:
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
@JsonIgnoreProperties(ignoreUnknown = true)
class Person {
public String name;
public int age;
}
String jsonString = "{\"name\": \"John Doe\", \"age\": 30, \"height\": 175}";
try {
Person person = objectMapper.readValue(jsonString, Person.class);
} catch (Exception e) {
System.out.println("Failed to parse JSON: " + e.getMessage());
}
4. Nested Objects and Arrays
Error Summary: Nested objects require careful handling. If you incorrectly define inner classes, it leads to parsing errors.
Example:
{
"name": "John Doe",
"contact": {
"email": "john@example.com"
// Missing comma for next property
"phone": "1234567890"
}
}
Fix: Always ensure proper syntax when defining nested objects. Additionally, create Java classes that accurately reflect the JSON structure.
Correct JSON:
{
"name": "John Doe",
"contact": {
"email": "john@example.com",
"phone": "1234567890"
}
}
Java Code Snippet:
class Contact {
public String email;
public String phone;
}
class Person {
public String name;
public Contact contact; // Nested Class
}
String jsonString = "{\"name\": \"John Doe\", \"contact\": {\"email\": \"john@example.com\", \"phone\": \"1234567890\"}}";
try {
Person person = objectMapper.readValue(jsonString, Person.class);
} catch (Exception e) {
System.out.println("Failed to parse JSON: " + e.getMessage());
}
5. Encoding Issues
Error Summary: JSON data should be properly encoded in UTF-8. If there are mismatches in character encoding, it can lead to parsing errors.
Fix: Make sure your JSON is always encoded in UTF-8 format.
Java Code Snippet:
import java.nio.charset.StandardCharsets;
String jsonString = ... // your JSON input
byte[] jsonData = jsonString.getBytes(StandardCharsets.UTF_8);
String utf8String = new String(jsonData, StandardCharsets.UTF_8);
try {
Person person = objectMapper.readValue(utf8String, Person.class);
} catch (Exception e) {
System.out.println("Failed to parse JSON: " + e.getMessage());
}
In Conclusion, Here is What Matters
Working with JSON in Java can lead to a variety of parsing errors, from syntax issues to unrecognized fields. By understanding these common problems and their solutions, developers can significantly reduce debugging time and improve the reliability of their applications. Always validate your JSON structure against your Java models, address any mismatched data types, and handle nested structures carefully.
Incorporating frameworks like Jackson facilitates efficient JSON handling, and utilizing annotations can simplify the parsing process. With these tips, your journey with JSON parsing in Java will be smoother and more efficient.
For further reading on JSON parsing in Java, consider checking out the official Jackson documentation. Happy coding!
Checkout our other articles