Speed Up Your Java 7 Code: Common Performance Pitfalls
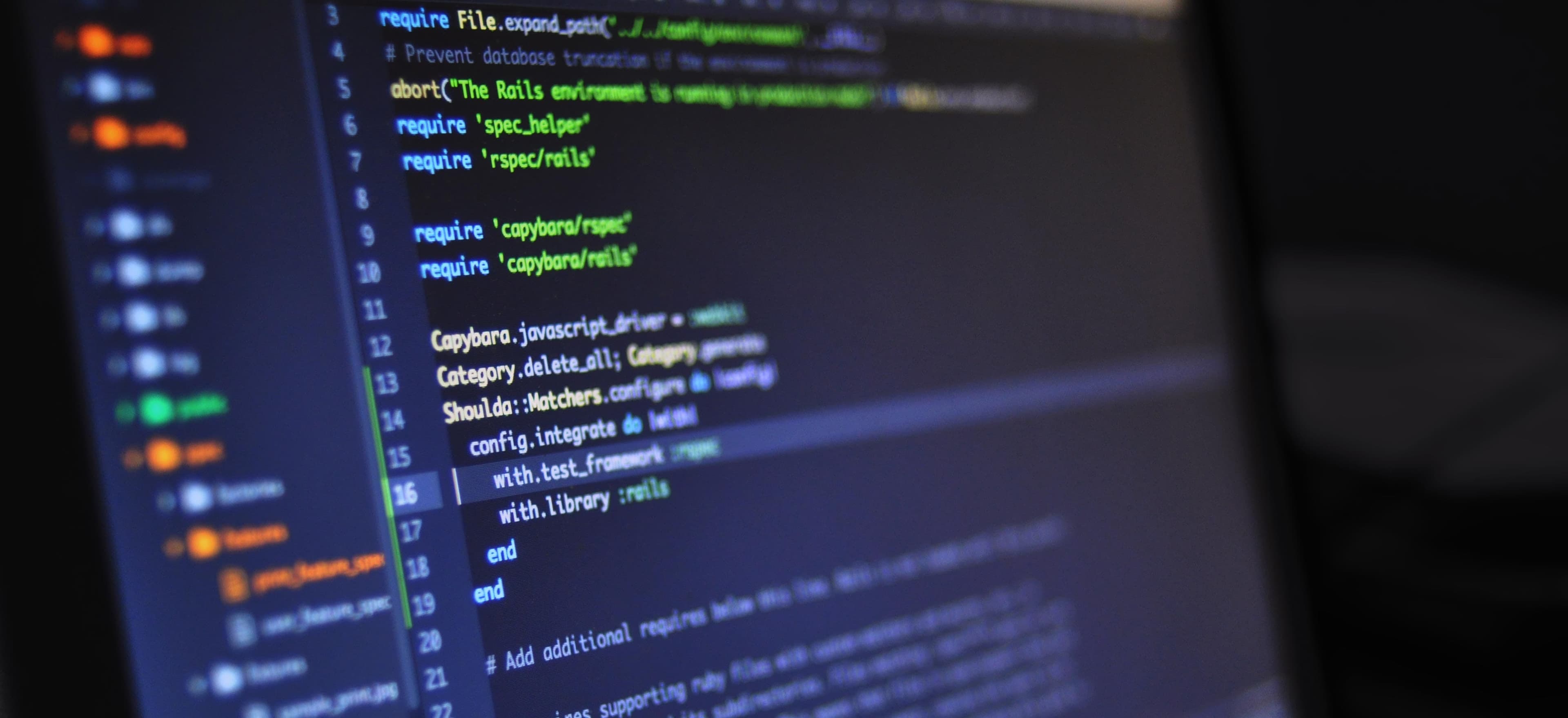
- Published on
Speed Up Your Java 7 Code: Common Performance Pitfalls
Java is a robust, high-performance programming language that powers billions of devices worldwide. However, despite its efficiency, developers often encounter performance issues, especially when working with older versions like Java 7. In this blog post, we will explore common performance pitfalls and practical strategies to help you speed up your Java 7 applications.
Understanding Java 7 Performance
Before diving into specific pitfalls, it’s essential to comprehend how Java executes code. Java uses a Just-In-Time (JIT) compiler that translates bytecode into machine code at runtime, optimizing execution based on actual usage. However, various coding practices and structures can disrupt this optimization, resulting in slower applications.
Common Performance Pitfalls
Here are the most prevalent performance pitfalls in Java 7, along with solutions and examples.
1. Excessive Object Creation
Java, being an object-oriented language, promotes the use of objects. However, creating too many objects can lead to memory overhead and garbage collection (GC) overhead, affecting performance.
Solution: Reuse objects whenever possible and utilize primitive types instead of their wrapper classes.
Example:
// Avoid this
Integer[] numbers = new Integer[1000];
for (int i = 0; i < numbers.length; i++) {
numbers[i] = Integer.valueOf(i);
}
// Prefer this
int[] numbers = new int[1000];
for (int i = 0; i < numbers.length; i++) {
numbers[i] = i; // Using primitive type
}
Why This Matters
Minimizing object creation reduces the burden on the garbage collector and enhances memory usage efficiency, positively impacting application performance.
2. Inefficient Looping
Loops are fundamental in programming; however, inefficient looping can result in performance bottlenecks. Consider the following example:
Example:
// Poor performance due to repeated calls
List<String> strings = ...;
for (int i = 0; i < strings.size(); i++) {
String str = strings.get(i); // This call is faster
// Process string
}
Improved Version:
// More efficient use of iterator
for (String str : strings) {
// Process string
}
Why This Matters
The enhanced loop syntax (for-each
) avoids the overhead associated with repeatedly calling methods (like get()
) and is generally more readable.
3. Unused Synchronization
While synchronization is essential for thread safety, excessive or unnecessary synchronization can lead to performance degradation.
Solution: Identify sections of code that do not require synchronization and remove it where possible.
Example:
// Unnecessarily synchronized block
public void addThreadSafe(String newElement) {
synchronized (this) {
this.list.add(newElement);
}
}
Improved Version:
// When no shared access is needed
public void add(String newElement) {
this.list.add(newElement);
}
Why This Matters
Reducing unnecessary synchronization can significantly improve throughput and application performance by allowing more concurrent access.
4. Inefficient String Manipulation
In Java, String
is immutable, meaning that each time you modify a string, a new object is created. This can lead to performance issues if string manipulation is frequent.
Solution: Use StringBuilder
or StringBuffer
for string concatenation in a loop.
Example:
// Inefficient string manipulation
String result = "";
for (String s : data) {
result += s; // Creates multiple String objects
}
Improved Version:
// Efficient string manipulation using StringBuilder
StringBuilder result = new StringBuilder();
for (String s : data) {
result.append(s); // Less overhead
}
Why This Matters
Using StringBuilder
minimizes memory usage and processing time, significantly enhancing application performance during string operations.
5. Overuse of Reflection
Reflection is a powerful feature in Java that allows you to inspect classes, methods, and fields at runtime. However, overusing reflection can lead to performance issues.
Solution: Limit reflection where possible. Consider alternatives such as interfaces or normal function calls.
Example:
// Reflection usage
Method method = obj.getClass().getMethod("someMethod");
method.invoke(obj);
Improved Version:
// Normal method call
obj.someMethod();
Why This Matters
Direct method calls are substantially faster than reflective calls, as they avoid the overhead associated with inspecting metadata at runtime.
6. Inefficient Use of Collections
Java's collection framework provides various data structures. Choosing the wrong type of collection or inefficiently utilizing them can lead to performance problems.
Solution: Choose the appropriate collection type based on use case. For example, if you only need to access elements by index, use an ArrayList
instead of a LinkedList
.
Example:
// Using LinkedList when not necessary
List<String> list = new LinkedList<>();
for (int i = 0; i < 1000; i++) {
list.add("Element " + i);
}
Improved Version:
// Using ArrayList for better performance
List<String> list = new ArrayList<>();
for (int i = 0; i < 1000; i++) {
list.add("Element " + i);
}
Why This Matters
Using the correct collection type can lead to significant performance gains, as each collection has unique strengths and weaknesses.
Final Thoughts
In summary, optimizing Java 7 code involves a thorough understanding of common performance pitfalls. Paying attention to object creation, loop efficiency, synchronization, string manipulation, reflection, and collections can greatly enhance your applications.
- Avoid unnecessary object creation: It reduces the memory footprint.
- Optimize looping structures: Use enhanced for-loops.
- Limit synchronization: Only use it when necessary for thread safety.
- Utilize
StringBuilder
for string manipulation: This minimizes overhead. - Reduce reflection usage: Direct calls are faster.
- Select appropriate collections: The right collection type saves time and memory.
By being aware of these issues, you can significantly enhance the performance of your Java applications. For further reading, check out Java Performance Tuning and Java Performance Best Practices.
Remember, performance optimization is a continuous process. Regularly profiling your code and understanding how it executes can make a world of difference in maintaining efficient applications. Happy coding!