Debugging Kivakit with AWS Lambda: Common Pitfalls
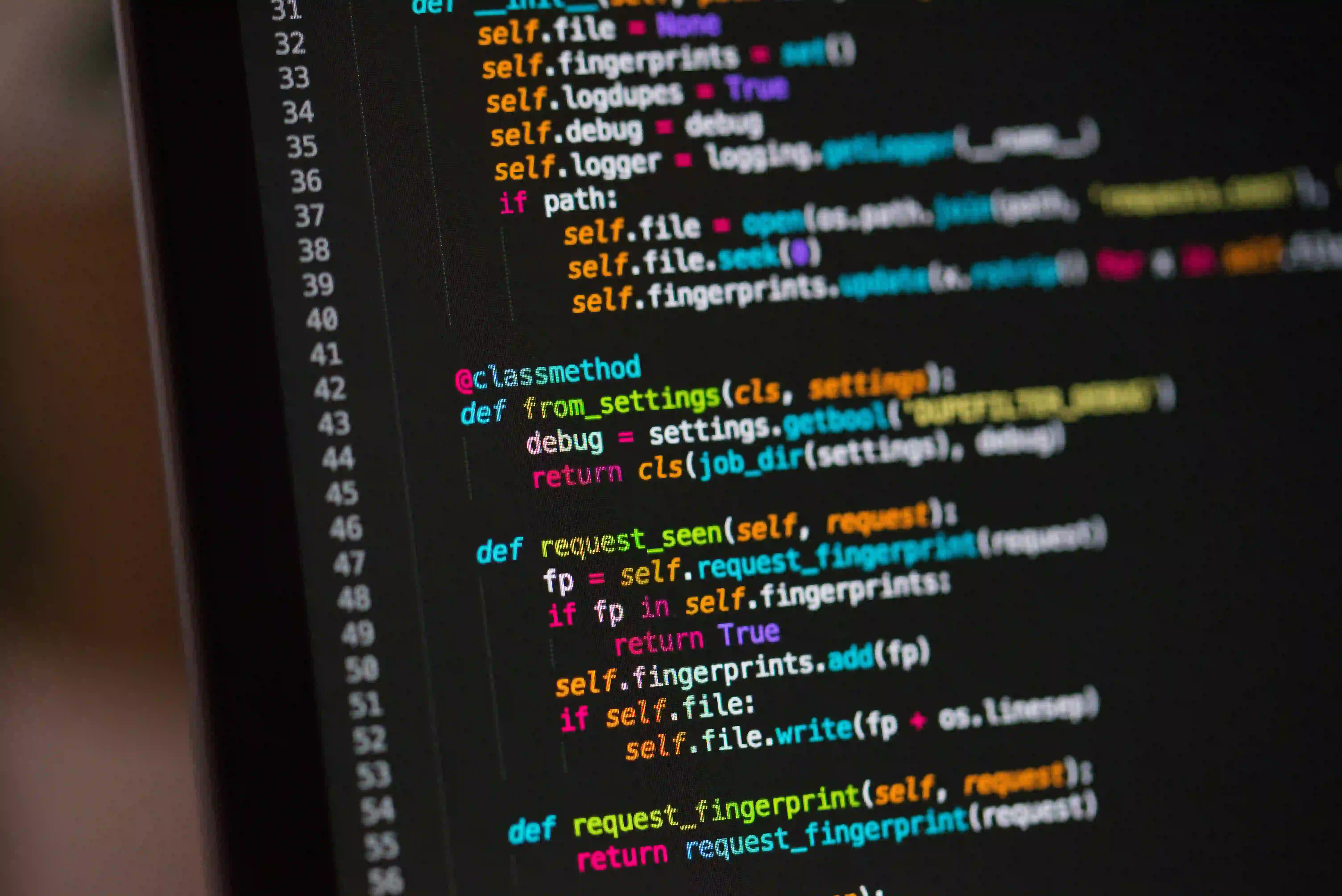
Debugging Kivakit with AWS Lambda: Common Pitfalls
Debugging applications can often be a daunting task. In the world of cloud computing, integrating services like AWS Lambda with frameworks such as Kivakit adds another layer of complexity. This blog post aims to pinpoint common pitfalls encountered during this process and provide practical solutions to tackle them effectively.
Understanding Kivakit and AWS Lambda
Kivakit is a versatile framework for developing and managing Java applications and has become quite popular due to its clean and powerful design. AWS Lambda, on the other hand, allows developers to execute code without provisioning servers, making it an attractive option for serverless architecture. Together, they create an efficient platform for developing cloud-native applications.
However, when integrating Kivakit with AWS Lambda, developers often face specific challenges. This article will highlight these common pitfalls and provide solutions.
Common Pitfall 1: Cold Starts
What Are Cold Starts?
Cold starts occur when an AWS Lambda function is invoked after not being used for some time. AWS triggers a new instance of the function, causing a delay in the response time, which can be problematic for real-time applications.
Solutions
- Keep the Function Warm: To mitigate latency due to cold starts, consider using scheduled events to invoke the Lambda regularly. This approach ensures that the function stays "warm."
// Example of setting a scheduled event in AWS CloudWatch
import com.amazonaws.services.cloudwatch.AmazonCloudWatch;
import com.amazonaws.services.cloudwatch.AmazonCloudWatchClientBuilder;
import com.amazonaws.services.cloudwatch.model.PutRuleRequest;
import com.amazonaws.services.cloudwatch.model.PutRuleResult;
public class LambdaScheduler {
public static void main(String[] args) {
AmazonCloudWatch cloudWatch = AmazonCloudWatchClientBuilder.defaultClient();
PutRuleRequest request = new PutRuleRequest()
.withName("KeepLambdaWarm")
.withScheduleExpression("rate(5 minutes)"); // Invokes the function every 5 minutes
PutRuleResult response = cloudWatch.putRule(request); // Set the schedule
System.out.println("Scheduled Lambda - Rule ARN: " + response.getRuleArn());
}
}
Keeping your Lambda function “warm” can help improve its response times, especially during peak usage.
Common Pitfall 2: Logging and Monitoring
The Need for Effective Logging
Without proper logging mechanisms, debugging can be a frustrating endeavor. AWS Lambda functions can produce logs in various ways but often require configuration to meet specific needs.
Solutions
- Utilize AWS CloudWatch: Every Lambda function can be connected to AWS CloudWatch for monitoring. Ensure you write appropriate logs to capture method entry and exit points, variable states, and error messages.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class LambdaFunction {
private static final Logger logger = LoggerFactory.getLogger(LambdaFunction.class);
public String handleRequest(String input, Context context) {
logger.info("Input: " + input); // Log input data
try {
// Business logic
String result = performOperation(input);
logger.info("Result: " + result);
return result;
} catch (Exception e) {
logger.error("Error occurred: " + e.getMessage(), e);
throw new RuntimeException(e); // Rethrow exception for Lambda to log
}
}
}
By maintaining detailed logs, you can track function performance and diagnose issues efficiently.
Common Pitfall 3: Resource Limitations
Understanding Resource Constraints
AWS Lambda imposes certain limits on resources such as memory and execution time. A common mistake is to overlook these limits, causing functions to timeout or fail unexpectedly.
Solutions
- Adjust Memory and Timeout Settings: Analyze execution time and optimize memory settings according to your usage patterns. Use AWS Lambda's metrics to find the right configuration.
// AWS CLI to update function configuration
aws lambda update-function-configuration --function-name YourFunctionName --memory-size 1024 --timeout 30
This command increases memory allocation to 1024 MB and sets a timeout to 30 seconds. Adjust these values as needed.
Common Pitfall 4: Local Development and Testing
Challenges of Local Development
Developers often find it challenging to replicate the AWS environment locally, leading to deployment issues. Misconfigured environments can cause failures that are hard to reproduce.
Solutions
- Utilize AWS SAM CLI: AWS Serverless Application Model (SAM) allows you to develop and test Lambda functions locally. Running your code in an environment similar to AWS can help identify issues before deployment.
# Start AWS SAM CLI in your terminal
sam local invoke YourFunctionName -e event.json
This command runs your Lambda function locally with a sample event, allowing for easier debugging.
Common Pitfall 5: Dependency Management
The Problem with Dependencies
When deploying to AWS Lambda, issues often arise depending on external libraries. A common mistake is failing to include all necessary dependencies in the deployment package.
Solutions
- Package Dependencies Properly: Always package your application using tools like Maven or Gradle to manage your dependencies effectively.
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>your-library</artifactId>
<version>1.0.0</version>
</dependency>
</dependencies>
Ensure the built JAR or ZIP file includes all dependencies required by your Kivakit application.
Bringing It All Together
Debugging a Kivakit application running on AWS Lambda can present several challenges, ranging from cold starts to dependency management issues. However, by understanding these common pitfalls and implementing the suggested solutions, you can streamline the debugging process and improve the performance and reliability of your applications.
For more resources on integrating Kivakit with AWS Lambda, consider referring to the AWS Documentation and the Kivakit Documentation.
Remember, debugging is not just about fixing issues—it's an opportunity to learn and grow as a developer. Happy coding!