Mitigating Spectre Vulnerabilities in JVM Environments
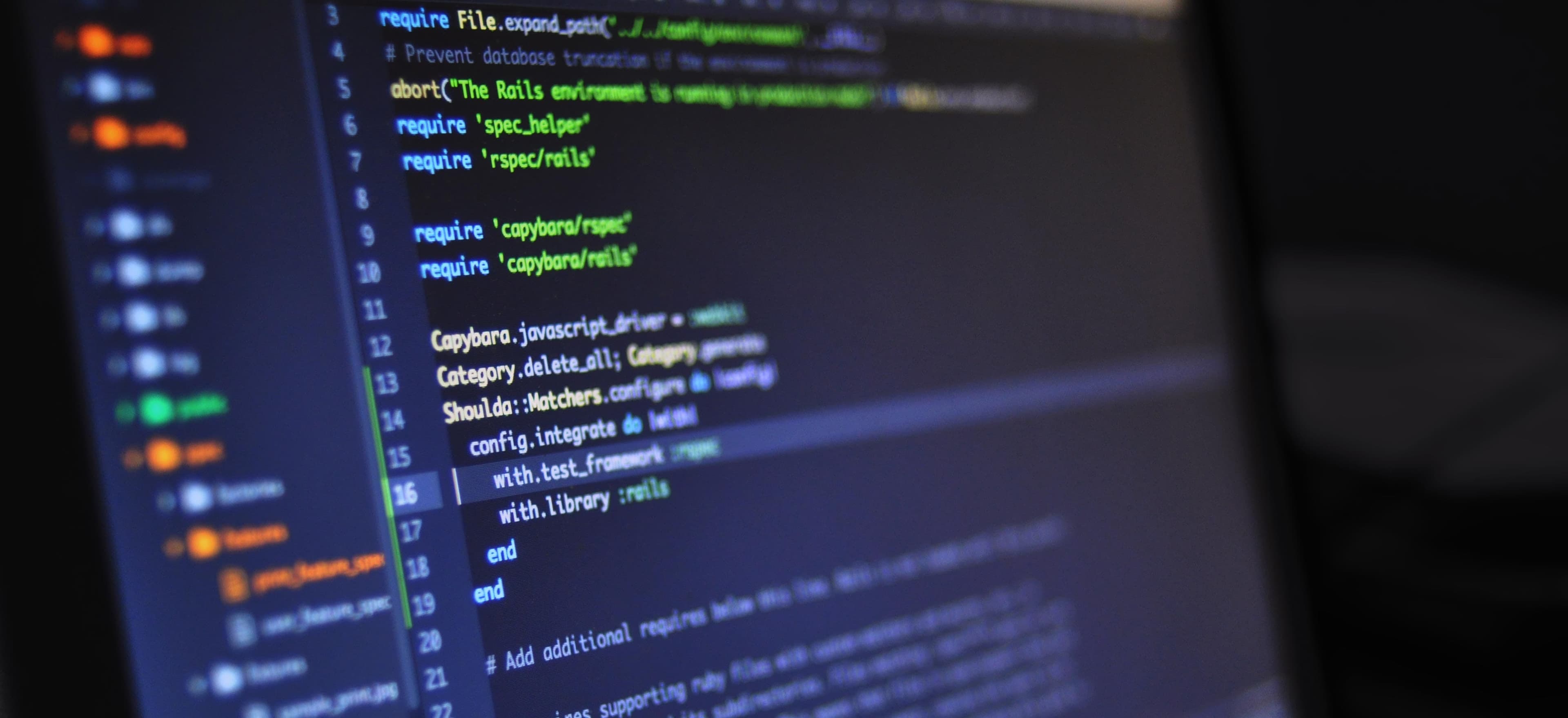
- Published on
Mitigating Spectre Vulnerabilities in JVM Environments
As the world becomes increasingly reliant on technology, vulnerabilities in software become a significant concern. One such issue is the Spectre vulnerability, which affects a range of processors and exploits speculative execution. While the Java Virtual Machine (JVM) abstracts many low-level details, understanding the implications of Spectre and how to mitigate its effects in JVM environments remains essential for developers and organizations alike.
Understanding Spectre Vulnerabilities
Spectre is a security vulnerability that exploits the modern CPU's speculative execution feature to gain access to sensitive information. This can result in sensitive data leaks, allowing an attacker to read memory contents that should not be accessible. Spectre is particularly dangerous because it is hardware-based and can potentially exploit any application, including those running on the JVM.
Key Concepts
- Speculative Execution: CPUs predict future instructions to speed up execution. While this improves performance, it can inadvertently expose sensitive data.
- Cache Timing: With Spectre, an attacker can measure access times to cached data, enabling them to infer sensitive information from memory.
To dive deeper into the details of Spectre and its implications, check out this insightful resource on Spectre and Meltdown.
Why JVM Environments Are Affected
While the JVM provides a level of abstraction, it runs on top of the host operating system and hardware. Consequently, applications running in the JVM can be vulnerable to Spectre attacks. This is especially evident in server-based applications and cloud environments where shared resources increase the risk of data being leaked.
The Importance of Mitigation
Proactively mitigating Spectre vulnerabilities is crucial for both application security and maintaining user trust. Organizations with sensitive data must ensure their applications are not vulnerable to exploitation.
Best Practices for Mitigating Spectre in JVM
Here are several best practices to consider when working in JVM environments:
1. Update JVM and Libraries
Updating your JVM to the latest version is one of the simplest ways to address security vulnerabilities, including Spectre. Developers of the JVM are continuously working to patch known vulnerabilities. Regularly updating all libraries used in your applications is essential for maintaining security.
// Check for the latest version from the official Oracle website
// Include a dependency management tool like Maven or Gradle to manage dependencies.
2. Use Safe Coding Practices
Implement safe coding practices by limiting the amount of sensitive data processed in any one function or method. By compartmentalizing sensitive information, you reduce the risk of exposure should a vulnerability be exploited.
class SensitiveDataHandler {
private String data;
public SensitiveDataHandler(String data) {
// Encapsulate sensitive data
this.data = data;
}
// Method that only exposes minimal necessary data
public String safeRetrieve() {
// Avoid leaking sensitive data through unnecessary methods
return data.substring(0, 4) + "****"; // Mask parts of the sensitive data
}
}
3. Avoid Untrusted Input
Applications should carefully validate all inputs, particularly if those inputs are used in Java Native Interface (JNI) calls or any functions that interact with lower-level system components.
String userInput = getUserInput();
if (!isValidInput(userInput)) {
throw new IllegalArgumentException("Invalid input!");
}
// Further process trusted input only
4. Leverage Security Features in the JVM
The JVM has several built-in security features that can enhance your application’s security posture:
- Security Manager: Use the SecurityManager to enforce access controls in Java applications. This ensures that even if there's a vulnerability, access to sensitive resources is restricted.
System.setSecurityManager(new SecurityManager());
- Java Modules: With the introduction of Java 9, the module system allows developers to better encapsulate packages, designing boundaries around sensitive data.
5. Monitor and Audit
Regularly verify the security posture of your applications through static and dynamic code analysis tools. These tools can help identify vulnerabilities, including potential Spectre-related issues.
// Use tools like SonarQube or Fortify to analyze code quality and security.
6. Reduce Memory Sharing
In cloud environments or microservices architecture, strive to avoid excessive memory sharing between applications. This configuration can help limit the attack surface.
// Design microservices to limit access to sensitive information when not necessary
// Use API Gateway patterns to handle traffic, minimizing direct access to internal services.
Final Thoughts
Mitigating Spectre vulnerabilities in JVM environments requires a combination of updating software regularly, adopting safe coding practices, and leveraging JVM security features. While no method can completely eliminate the risk, implementing these best practices can significantly reduce your application’s vulnerability to Spectre attacks.
For further exploration, refer to Oracle's Security Documentation to stay up-to-date on security practices.
By staying vigilant and proactive, you can create a safer environment for your Java applications and protect the sensitive data of your users against potential leaks due to vulnerabilities like Spectre.