Common Mistakes When Using URIBuilder for Quick URIs
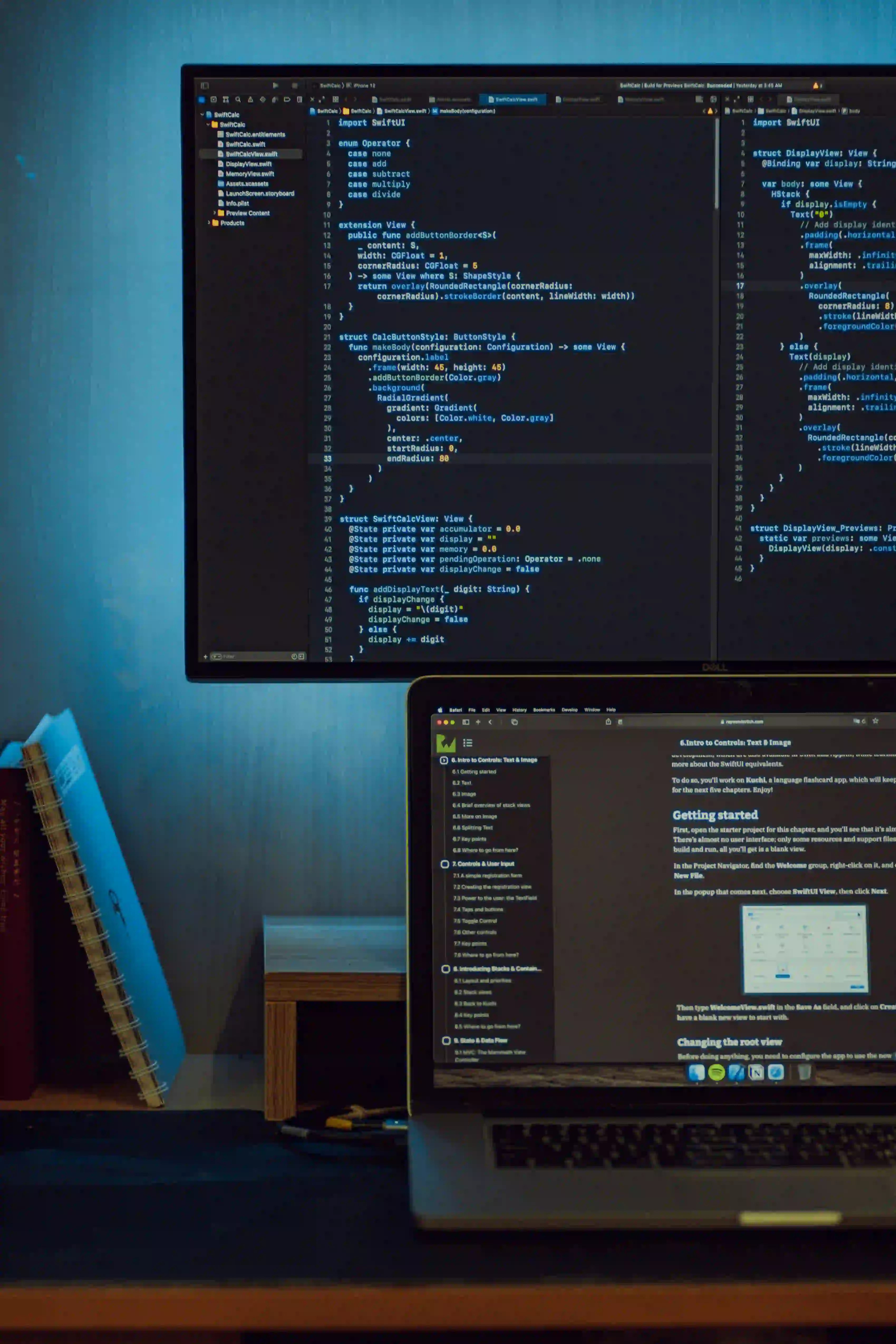
Common Mistakes When Using URIBuilder for Quick URIs in Java
When it comes to constructing URIs in Java, the URIBuilder
class can be a convenient tool, especially when you need to build URLs programmatically. This utility, however, isn't always straightforward. Developers often make mistakes that lead to unexpected results. In this blog post, we will explore common pitfalls when using URIBuilder
, provide insightful examples with code snippets, and highlight best practices.
Understanding URIBuilder
The URIBuilder
is part of Apache Commons and is designed to simplify creating URIs in Java. It provides an easy way to modify URL components, such as the scheme, host, path, and query parameters.
Replace:
import org.apache.http.client.utils.URIBuilder;
Common Mistakes
1. Not Encoding Query Parameters Properly
HTML and HTTP standards require certain characters to be encoded in URLs. When using URIBuilder
, it manages encoding automatically for you, but if you manipulate string parameters directly, you might forget this crucial step.
URIBuilder builder = new URIBuilder("http://example.com/search");
builder.addParameter("query", "Java & Spring Framework");
String uriString = builder.build().toString();
System.out.println(uriString);
Why This Matters: The query in the URL will be malformed if characters like &
are not correctly encoded. Always let URIBuilder
handle parameter values to avoid introducing errors.
2. Forgetting to Handle Exceptions
Building URIs can lead to URISyntaxException
. Proper exception handling is crucial, especially in production code. It's often overlooked by developers who anticipate the process to be always successful.
try {
URIBuilder builder = new URIBuilder("http://example.com");
builder.setPath("/api/resource");
System.out.println(builder.build().toString());
} catch (URISyntaxException e) {
e.printStackTrace();
// Take action, e.g., logging
}
Why This Matters: Failing to handle exceptions may crash your application. Always anticipate and handle potential exceptions.
3. Misusing Path Components
A frequent mistake occurs when developers mistakenly set the path in a way that results in unnecessary or duplicate slashes.
URIBuilder builder = new URIBuilder("http://example.com////api////resource");
builder.setPath("/api/resource"); // Intending to set path cleanly
System.out.println(builder.build().toString());
Output:
http://example.com/api/resource
Why This Matters: While URIBuilder
normalizes paths, assuming it will always do so can lead to unintended consequences. Always set the path properly.
4. Ignoring Default Port
When constructing URIs, developers often overlook the default port associated with a given scheme. For example, the default port for HTTP is 80, and for HTTPS, it's 443. If you specify a port but mean to use the default, errors could arise.
URIBuilder builder = new URIBuilder("http://example.com:80/api/resource");
System.out.println(builder.build().toString()); // Will treat 80 as a custom port
Output:
http://example.com:80/api/resource
Why This Matters: Only explicitly specify a port when it differs from the default. Otherwise, stick to the defaults to avoid confusion.
5. Confusing setParameter
With addParameter
When you are supposed to add multiple query parameters and mistakenly use setParameter
, the previous parameters will be overridden. This is a typical source of confusion among developers.
URIBuilder builder = new URIBuilder("http://example.com");
builder.addParameter("param1", "value1");
builder.setParameter("param1", "value2"); // This replaces the previous value
String uri = builder.build().toString();
System.out.println(uri);
Output:
http://example.com?param1=value2
Why This Matters: This behavior can lead to losing previously added parameters unintentionally. Use addParameter
to retain all parameters as required.
Best Practices
1. Use URIBuilder for Encoding
Always leverage URIBuilder
for adding query parameters to ensure they are properly encoded. Avoid manual string concatenation for URLs. For more details on HTTP URL encoding, check RFC 3986.
2. Consistent Exception Handling
Always wrap URI building logic in try-catch blocks to handle potential errors gracefully. Logging errors can help debug issues.
3. Verify Protocol and Port
Always confirm which protocol and port you intend to use. This can prevent a lot of headaches related to connectivity and server communication.
4. Validate URI
After building, you can validate the URI by testing it with an HTTP client. This ensures the URI is not just syntactically valid but also workable in practice.
// Example of sending a request to validate
try {
URIBuilder builder = new URIBuilder("http://example.com");
builder.setPath("/api/resource");
String uri = builder.build().toString();
// Sending a request to validate the URI would come here
// ...
} catch (URISyntaxException e) {
e.printStackTrace();
}
5. Read the Documentation
It might seem simple, but thoroughly understanding the libraries you are using, including their behavior and limitations, is always beneficial. Check the official documentation for Apache HttpClient for more insights.
To Wrap Things Up
Using URIBuilder
to construct URIs in Java can significantly simplify your networking code, but common mistakes can lead to frustrating debugging sessions. Avoiding these pitfalls—such as improper encoding of parameters, failing to handle exceptions, and mismanaging paths and parameters—can enhance your development experience. By adopting best practices, you can leverage this tool more effectively.
If you'd like to learn more about Java networking and URI-related topics, consider exploring additional resources. Happy coding!