Top Java EE 7 Features That Are Often Overlooked
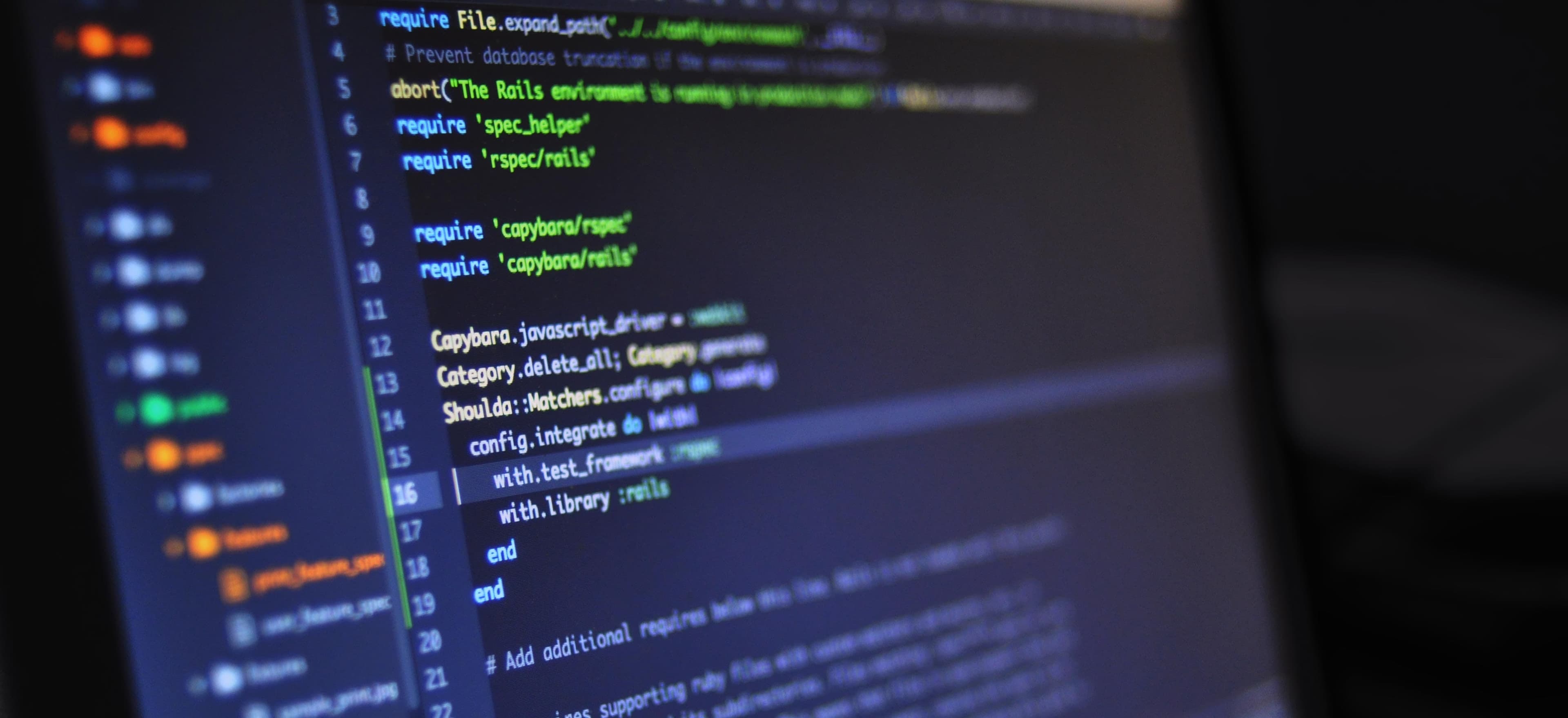
- Published on
Top Java EE 7 Features That Are Often Overlooked
Java EE 7, which debuted in 2013, is a powerful platform designed for enterprise applications. While many developers are familiar with popular features such as JSF, JPA, and EJB, there are several other features that provide substantial benefits yet remain underappreciated. In this blog post, we'll explore lesser-known features of Java EE 7 that can enhance your development experience and deliver robust applications.
1. Batch Processing
Overview
Java EE 7 introduced the Batch Processing API (JSR 352) to help manage large volumes of data with ease. The Batch Processing feature streamlines long-running tasks and can handle complex workflows through a simple set of annotations.
Why It Matters
In enterprise applications, batch processing is often vital for handling operations like processing payrolls, importing data, or generating reports. The Batch Processing API helps break tasks into manageable chunks, improving performance and resource consumption while providing fault-tolerance.
Example
Here's a simple example of how to define a batch job:
@Job
public class SampleJob {
@Step
public void processBatch() {
// Code for processing individual items
System.out.println("Processing batch item...");
}
}
Commentary
The code snippet above demonstrates the use of @Job
and @Step
annotations to create a simple batch job. The processBatch()
method contains the logic for processing items in bulk. The annotations help define the batch job in a clear manner, making it easier to manage and read.
2. WebSocket API
Overview
Java EE 7 also introduced the WebSocket API (JSR 356), enabling real-time, two-way communication between clients and servers. This is particularly beneficial for applications that require instant updates or live interactions, such as chat applications and online gaming.
Why It Matters
WebSockets reduce the overhead of traditional HTTP requests for real-time applications, making communication more fluid. Developers can create interactive apps with minimal latency, improving user experience.
Example
Here's a basic implementation of a WebSocket endpoint:
@ServerEndpoint("/chat")
public class ChatEndpoint {
@OnOpen
public void onOpen(Session session) {
System.out.println("Connected: " + session.getId());
}
@OnMessage
public void onMessage(String message, Session session) {
System.out.println("Message from client: " + message);
// Echo the message back
session.getAsyncRemote().sendText("Echo: " + message);
}
@OnClose
public void onClose(Session session) {
System.out.println("Disconnected: " + session.getId());
}
}
Commentary
In this example, we define a chat endpoint using the @ServerEndpoint
annotation, enabling real-time communication. The onMessage
method allows us to handle incoming messages and respond to clients. This feature can drastically improve user engagement in applications.
3. Java Message Service 2.0 (JMS 2.0)
Overview
JMS 2.0 simplifies messaging between distributed components, making it easier to create and manage message-driven applications. It offers a fluent API and added support for annotations to streamline message processing.
Why It Matters
In complex enterprise environments, messaging plays a crucial role in decoupling services and ensuring reliable communication. JMS 2.0 allows developers to focus on business logic rather than dealing with intricate messaging configurations.
Example
Here's how you can create and send a message using JMS 2.0:
@Resource
private ConnectionFactory connectionFactory;
@Resource
private Queue queue;
public void sendMessage(String text) {
try (JMSContext context = connectionFactory.createContext()) {
context.createProducer().send(queue, text);
System.out.println("Sent message: " + text);
}
}
Commentary
In this snippet, we utilize JMS 2.0's simpler API to create a message producer. The JMSContext
ensures that the connections are handled seamlessly, reducing boilerplate code and enhancing readability.
4. JSON-B (JSON Binding API)
Overview
With the growing adoption of RESTful services, Java EE 7 introduced JSON-B, a standard for binding JSON data to Java objects. This API simplifies the serialization and deserialization of Java objects to and from JSON format.
Why It Matters
JSON is ubiquitous in web applications, and effective handling of JSON data is critical. JSON-B creates a smooth conversion process, reducing the chances for error and boilerplate code.
Example
Here's a quick example of JSON-B usage:
Jsonb jsonb = JsonbBuilder.create();
String json = jsonb.toJson(new User("John", "Doe"));
System.out.println(json);
User user = jsonb.fromJson(json, User.class);
System.out.println(user);
Commentary
This code demonstrates how to convert a Java object into JSON format and back again using JSON-B. The use of JsonbBuilder
provides a fluid API that enhances usability and minimizes development effort.
5. Context and Dependency Injection (CDI 1.1)
Overview
Although CDI was introduced earlier, Java EE 7 solidified its presence. CDI 1.1 enhances the way developers manage dependencies and context lifecycle without using external frameworks, streamlining the code structure.
Why It Matters
Dependency injection facilitates better code organization and testing. By allowing easier management of component lifecycles, CDI makes applications more flexible and maintainable.
Example
Here's an example of a simple CDI bean:
@RequestScoped
public class UserService {
@Inject
private UserRepository userRepository;
public List<User> getAllUsers() {
return userRepository.findAll();
}
}
Commentary
This example showcases how CDI can simplify the management of service classes by injecting dependencies. The @RequestScoped
annotation ensures that the service is created once per HTTP request, keeping the resource usage efficient.
6. Concurrency Utilities
Overview
Java EE 7 provides built-in mechanisms for managing concurrency, which gives developers better control over concurrent tasks within their applications. This is crucial for performance and reliability in distributed systems.
Why It Matters
Concurrency is complex. By providing a straightforward API for managing threads and asynchronous tasks, Java EE 7 allows developers to focus on their application's logic rather than dealing with thread management intricacies.
Example
Here's an example of using the ManagedExecutorService:
@Resource
ManagedExecutorService executorService;
public void submitTask(Runnable task) {
executorService.submit(task);
}
Commentary
In this snippet, the ManagedExecutorService
facilitates submitting tasks without the hassle of managing thread pools manually. This feature empowers developers to execute background tasks effortlessly.
To Wrap Things Up
Java EE 7 is a feature-rich platform that extends well beyond the commonly known components. From Batch Processing and WebSockets to JSON-B and CDI, these overlooked features can significantly enhance your application's functionality and maintainability.
As a developer, understanding and utilizing these features can lead to cleaner, more efficient code and ultimately, a more robust enterprise application. If you're interested in more detailed documentation or guidelines, consider exploring the official Oracle documentation.
Incorporate these features into your projects and watch how they simplify your implementations while boosting performance. Happy coding!