Overwhelmed by Annotations? Simplify Your Review Process!
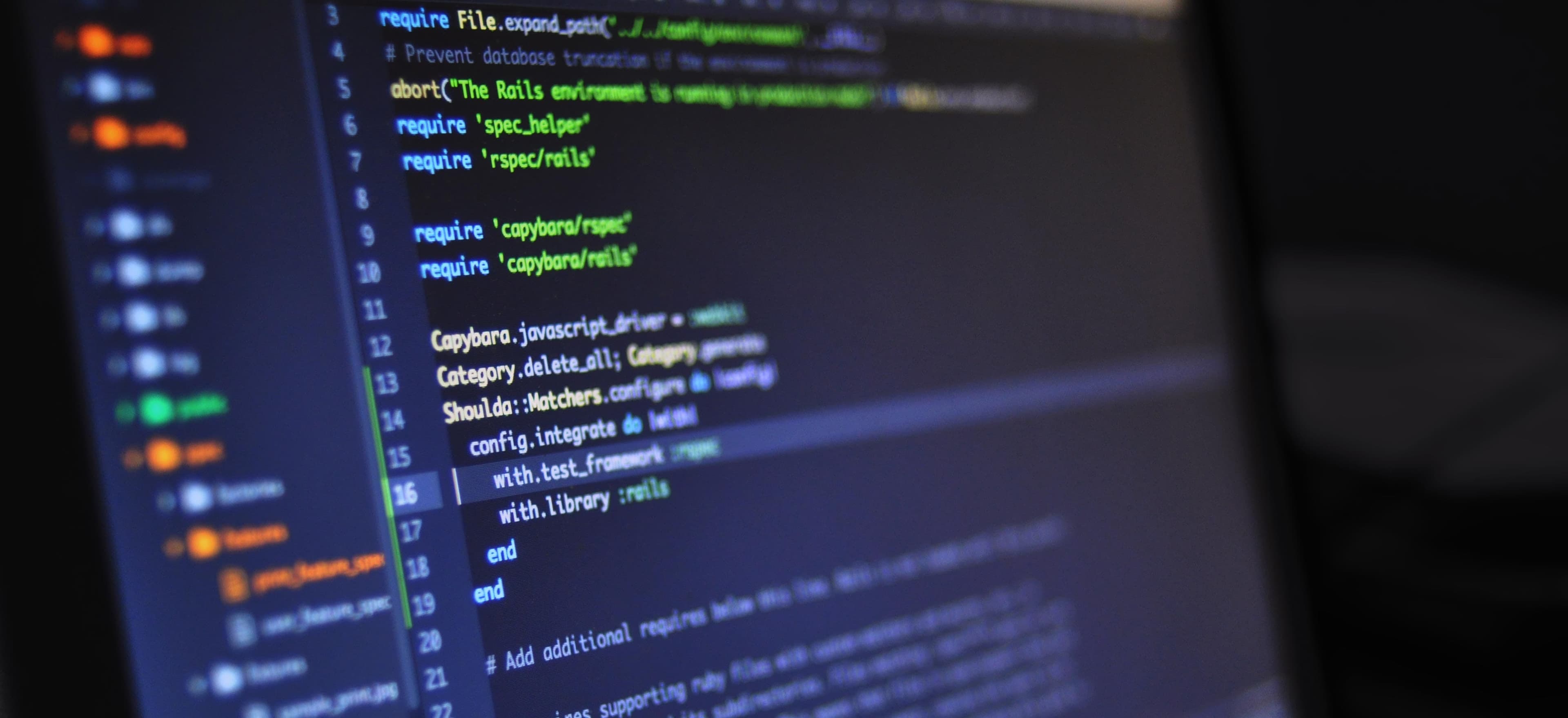
- Published on
Overwhelmed by Annotations? Simplify Your Review Process!
In the realm of software development, code annotations play a pivotal role in enhancing readability, learnability, and maintainability. However, when mismanaged or overused, they can quickly clutter your codebase, making it challenging to review and understand. In this blog post, we'll delve into the various types of annotations available in Java, their best practices, and tools to help simplify your review process.
Understanding Code Annotations in Java
Annotations in Java are metadata provided to your code to indicate information about it. They don't change the code's behavior but provide a way to convey additional information. Some of the most commonly used annotations include:
@Override
: Indicates that a method is overriding a method from a superclass.@Deprecated
: Marks a method or class as outdated and suggests that it should not be used.@SuppressWarnings
: Instructs the compiler to suppress specific warnings generated by the code.
Here's a brief code snippet demonstrating how to use the @Override
annotation effectively:
class Animal {
void makeSound() {
System.out.println("Some sound");
}
}
class Dog extends Animal {
@Override
void makeSound() {
System.out.println("Bark");
}
}
Why Use Annotations?
Annotations offer a declarative way to specify behavior in your code. They enhance the code's self-documenting capabilities and enable external tools and frameworks to process the metadata. Proper usage results in:
-
Improved Readability: Annotations clarify intentions and responsibilities, making it easier for others (or yourself in the future) to grasp the code.
-
Enhanced Tooling Support: Many IDEs provide specialized support for annotations, assisting with features like auto-completion and error-checking.
-
Seamless Integration with Frameworks: Popular frameworks such as Spring and Hibernate utilize annotations to simplify configuration and enhance functionality.
Best Practices for Using Annotations
While annotations are powerful, their overuse can lead to confusion. Here are some best practices to ensure clarity in your code:
1. Limit the Number of Annotations
Consider your code's maintainability. Avoid piling on multiple annotations. Instead, group related functionality wherever possible.
2. Prefer Standard Annotations
Opt for built-in annotations before creating custom ones. They enhance familiarity and reduce the learning curve for new developers.
3. Document Annotation Usage
Whenever a custom annotation is created, document its purpose, usage, and behavior. This information is crucial for your team and future maintainers.
4. Refine Code with Annotation Processing
Use tools for annotation processing to manage and generate necessary boilerplate code. Libraries like Lombok provide functionalities via annotations that significantly reduce repetitive coding.
Here’s a simple demonstration of using Lombok to reduce boilerplate code in a Java class:
import lombok.Getter;
import lombok.Setter;
@Getter
@Setter
public class User {
private String name;
private String email;
}
Why Lombok?
Instead of manually coding getter and setter methods, we can use annotations to automatically generate them during compile time. This not only reduces boilerplate but makes the code less prone to errors.
Tools for Simplifying the Review Process
Annotations can create clutter in your code, making reviews cumbersome. Here’s how to simplify your review process:
1. Code Linters
Linters help enforce coding standards, catching excessive or misplaced annotations. Tools like Checkstyle or PMD can be configured to flag unwanted annotations in your code.
2. Static Code Analyzers
Static analysis tools, such as SonarQube, can analyze your codebase for various quality issues, including correct annotation usage. They provide insightful metrics on how annotations affect readability and maintainability.
3. Using IDE Features
Modern IDEs like IntelliJ IDEA or Eclipse offer built-in features to analyze and manage annotations better. Utilize these tools to navigate quickly through annotations and comprehend their implications.
4. Review Annotations Separately
Create a convention within your team to review annotations separately from functional code changes. This not only clarifies intent but also focuses on the annotation's usefulness and correctness without cluttering the review with functional code.
Staying Alert with Version Control
When working on collaborative projects, version control is essential. Utilizing platforms like Git allows you to maintain a history of changes to your annotations. When reviewing pull requests, consider the following:
- Are the annotations meaningful for the team?
- Have potentially deprecated methods been marked correctly?
- Is there an alignment with the established guidelines on annotation usage?
Here’s an example of how to create a Git commit message regarding annotation changes:
fix: Update annotation usage in UserService
- Added @Deprecated on fetchUserById method, marking it for removal in future releases
- Replaced manual getter/setter methods with Lombok annotations
Closing the Chapter
While annotations are a powerful feature in Java, they can also become overwhelming if not managed properly. By adhering to best practices, utilizing appropriate tools, and fostering a disciplined review process, you can effectively streamline your code reviews. The benefits are significant—enhanced readability, fewer errors, and an overall better coding experience.
For a deeper dive into code annotations in Java, explore this comprehensive guide or check out Lombok's official documentation.
Remember, clarity is key. The goal is to ensure your annotations communicate effectively, simplifying the review process rather than complicating it. Happy coding!