Beware the Hidden Pitfalls of Hibernate Auto Flush!
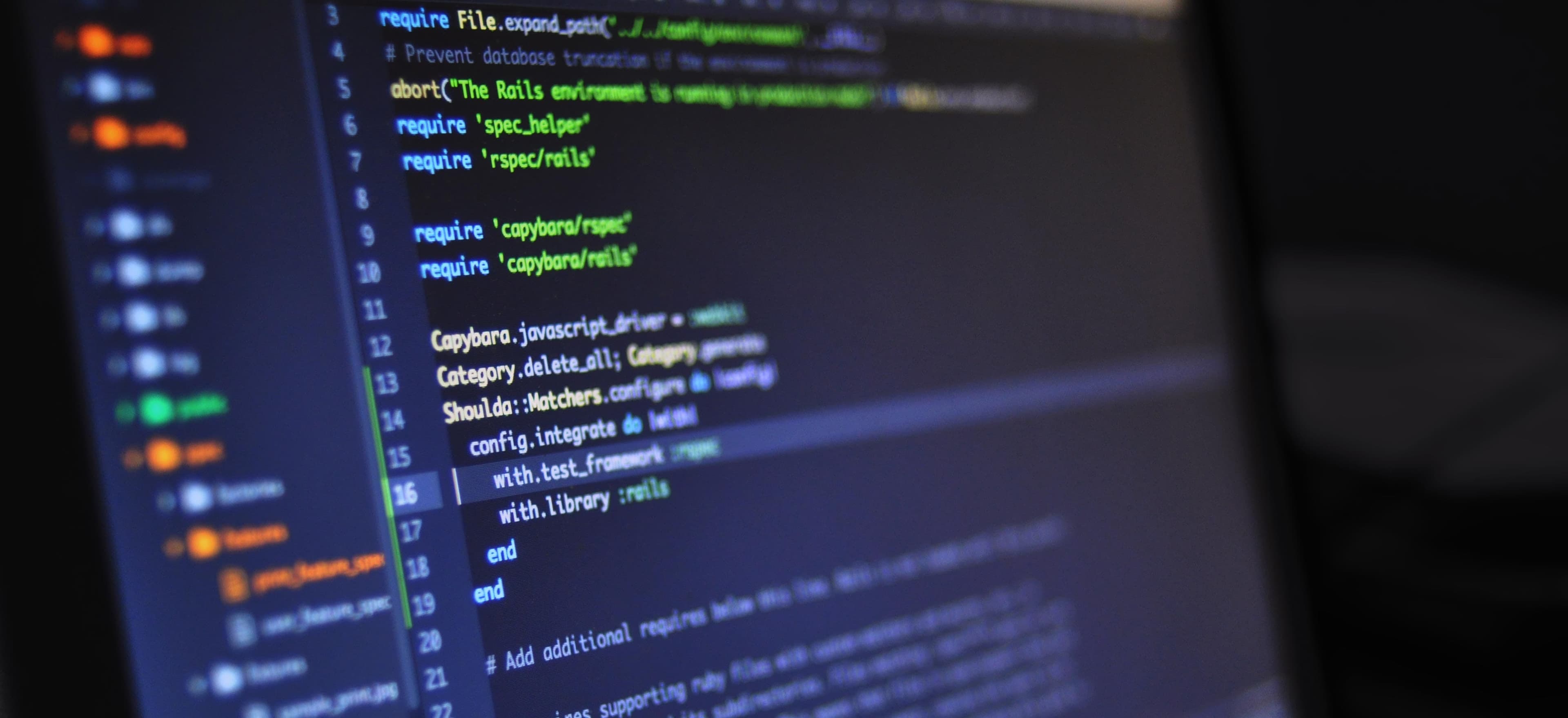
- Published on
Beware the Hidden Pitfalls of Hibernate Auto Flush!
Hibernate is a powerful Object-Relational Mapping (ORM) tool that makes it easy to manage database interactions in Java applications. However, while Hibernate simplifies database operations, it also introduces some complexities that developers must understand to avoid issues. One such complexity is auto flush. In this post, we'll explore what auto flush is, its potential pitfalls, and how to manage it effectively.
What is Hibernate Auto Flush?
Auto flush refers to Hibernate's behavior of synchronizing the state of the persistence context (first-level cache) with the database at specific points during the transaction lifecycle. This behavior can either be automatic or manual, depending on your configuration and the transaction boundaries defined in your code.
When certain actions occur—such as executing a query or committing a transaction—Hibernate checks if there are any pending changes in the persistence context that need to be flushed to the database. This ensures data consistency but can lead to performance issues or unexpected behavior if not handled properly.
How Hibernate Auto Flush Works
By default, Hibernate’s session is set to auto flush before:
- Executing a query
- Committing a transaction
- Calling
flush()
, and - At transaction boundaries
Example of Auto Flush Behavior
Consider the following code snippet demonstrating a simple use case of Hibernate session and entity management.
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
User user = new User();
user.setName("John Doe");
session.save(user); // Changes are pending in the persistence context
// At this point, Hibernate has not yet flushed the changes to the database
// Executing a query
List<User> users = session.createQuery("from User").getResultList(); // Auto flush occurs here
transaction.commit(); // Another auto flush may occur here
session.close();
In this code, when we execute the query to retrieve a list of users, Hibernate triggers an auto flush, synchronizing the user
entity's state with the database. But this behavior may introduce hidden pitfalls.
Hidden Pitfalls of Hibernate Auto Flush
1. Unexpected Database State
One common pitfall of auto flush is the unexpected state of the database due to the timing of flush calls. Suppose you save an entity and immediately query the database within the same transaction. You might not expect that the new entity would be visible in the query result, but due to the auto flush occurring just before the query execution, it will be present.
Advice: To avoid confusion, always be aware of the order of operations and consider separating the logic into different transaction boundaries if necessary.
2. Performance Issues
Frequent auto flush operations can cause performance degradation. Each flush operation writes changes to the database, and if your application has many modifications or queries within a single transaction, this can lead to excessive round trips to the database.
// A simple demonstration of multiple flushes can lead to performance issues
for (int i = 0; i < 1000; i++) {
User user = new User();
user.setName("User " + i);
session.save(user); // May cause multiple flushes on each save if not handled properly
}
Advice: Batch your operations or reduce the frequency of flush calls. You can do this by systematically calling flush()
at strategic points rather than relying solely on Hibernate’s automatic behavior.
3. Lazy Initialization Exceptions
When an auto flush occurs, and an entity is modified, the state of that entity may change unexpectedly. This can lead to cases where lazy-loaded associations may lead to LazyInitializationException
if accessed outside of an active session scope.
Example of Lazy Loading Issue
User user = session.get(User.class, userId);
// Assume user has a lazy loaded collection here
List<Post> posts = user.getPosts(); // Lazy loading occurs here
transaction.commit(); // Session closed
In this code, if we try to access the posts after committing the transaction, it throws a LazyInitializationException
because the session is closed.
Advice: Ensure that all necessary data is fetched while the session is open, or consider using the JOIN FETCH
in your queries to pre-fetch lazy collections to avoid such exceptions.
4. Transaction Management Complexity
Transactional boundaries can become confused when auto flush occurs. A developer might expect that the database state at the end of a transaction is determined by the last operation. However, due to auto flush, the actual state might differ based on prior operations.
Advice: Stick to a clear transactional model. Understand how and when flush calls occur, and break your operations into manageable transactions.
Managing Auto Flush Behavior
Fortunately, you can manage auto flush behavior in Hibernate to fit your needs. Here are some techniques to control this aspect effectively:
1. Disabling Auto Flush
You can disable auto flush on a specific session. This allows you to perform various operations without committing them immediately, only flushing the changes when you’re sure.
session.setFlushMode(FlushModeType.COMMIT); // Only flush on transaction commit
2. Manual Flush Control
Make use of manual flush control when needed. Instead of relying on auto flush, you can call session.flush()
only when you want to commit the changes. This gives you granular control.
session.flush(); // Manually trigger flush at required points
3. Setting Batch Size
To improve performance during a batch operation, set the Hibernate batch size property. This helps in reducing the number of flush calls.
hibernate.jdbc.batch_size=50 // Set the batch size according to your scenario
The Closing Argument
Hibernate's auto flush feature is both a blessing and a potential source of confusion. While it simplifies many database interactions, it also introduces pitfalls that can lead to unexpected behaviors and performance issues. By understanding how auto flush works and employing strategies for managing it, you can develop more efficient and effective Java applications.
For further reading, check out Hibernate documentation and learn more about transaction management in Spring. By keeping a vigilant eye on how Hibernate handles flushing, you can avoid the hidden pitfalls and build robust database interactions within your applications.
By being aware of these hidden pitfalls and how to navigate them, you can leverage the power of Hibernate while minimizing the risks of unexpected application behavior. Happy coding!