Resolving Binding Issues with Java EE 8 MVC Bean Parameters
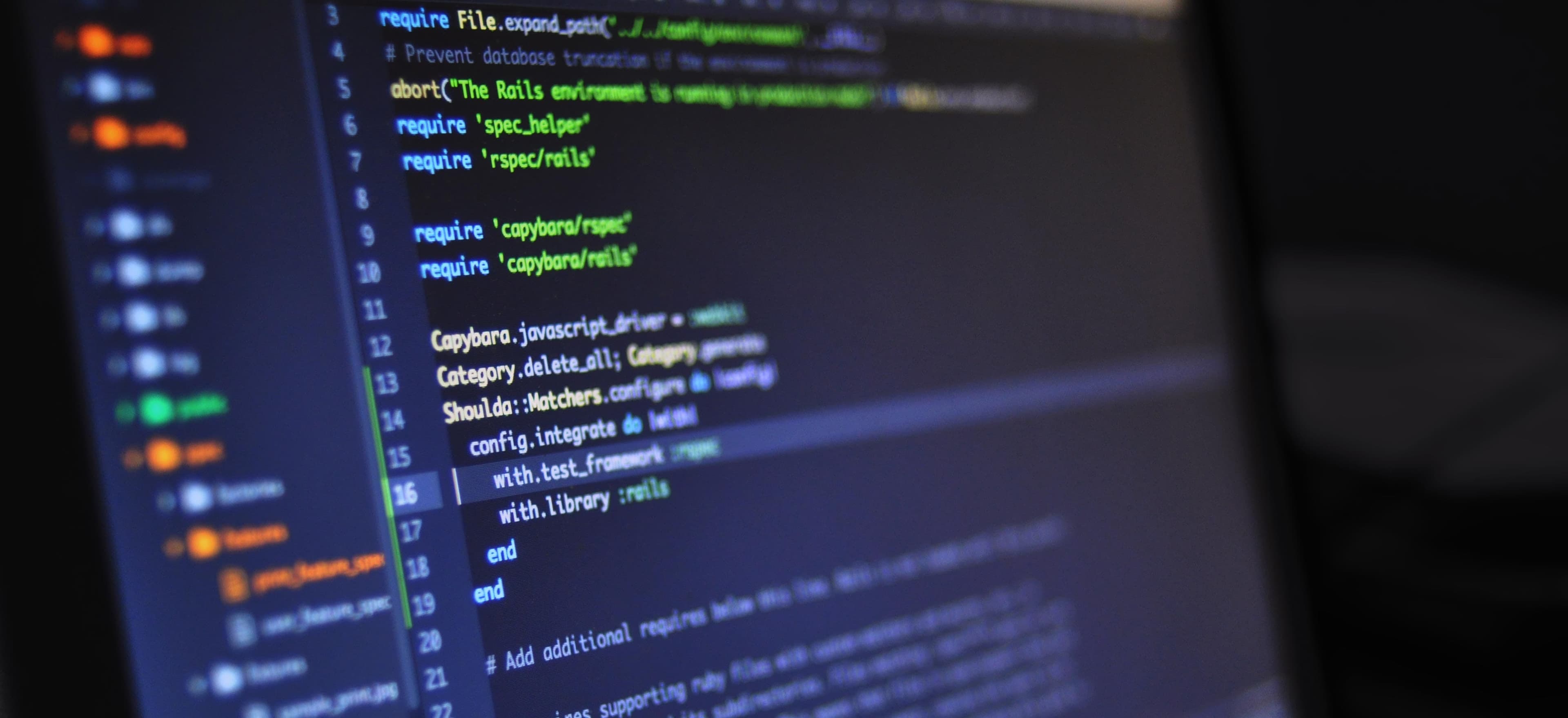
- Published on
Resolving Binding Issues with Java EE 8 MVC Bean Parameters
Java EE (Enterprise Edition), specifically version 8, introduces a robust framework for building scalable, secure, and flexible web applications. One of the essential components of Java EE is the MVC (Model-View-Controller) architecture, which aids in separating concerns, facilitating cleaner code, and easing maintenance efforts. However, developers can often encounter binding issues when dealing with MVC bean parameters. This blog post explores these binding challenges and provides effective strategies for resolving them.
Understanding Binding Issues in Java EE 8 MVC
Binding issues occur when data sent from the client to the server fails to match the expected data types or formats defined in your MVC bean. This can lead to issues such as null values, conversion errors, and exceptions during runtime.
Consider the following scenario: You are developing a simple web application where users can submit their profiles. The form input fields (like name, age, and email) are expected to bind directly to a Profile
bean. If a user's input does not conform to the expected type, such as entering text into an age field, binding fails, leading to runtime errors.
Example Profile Bean
Here’s a basic example of a Profile
bean representation:
package com.example.model;
import javax.validation.constraints.Email;
import javax.validation.constraints.Min;
import javax.validation.constraints.NotBlank;
public class Profile {
@NotBlank(message = "Name must not be blank")
private String name;
@Min(value = 1, message = "Age must be at least 1")
private int age;
@Email(message = "Invalid email format")
private String email;
// Getters and Setters
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
Why Binding Issues Matter
Correctly binding request parameters to your bean properties is crucial for several reasons:
- User Experience: When users fill out forms, they expect their input to be accepted and processed without errors.
- Data Integrity: Validating user input empowers you to maintain the integrity of the data being stored in your database.
- System Reliability: Binding issues can lead to application crashes. By addressing them, we ensure stability and a seamless experience.
Common Binding Errors
To address binding issues effectively, it's crucial to understand the common types of errors one might encounter:
- Null Values: When a form field is left empty and the corresponding bean property is required, you may face null pointer exceptions.
- Type Mismatch: When a user inputs data of an incorrect type (e.g., a string instead of an integer for the age), a conversion error occurs.
- Validation Failures: Constraints such as @NotBlank and @Email may be violated, leading to validation exceptions.
Strategies to Resolve Binding Issues
1. Validate Input Data
Utilize Java Validation API annotations (like those showcased in the Profile
bean). These annotations help enforce rules to validate incoming data.
import javax.validation.Valid;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.core.Response;
@Path("/profile")
public class ProfileController {
@POST
public Response createProfile(@Valid Profile profile) {
// If validation fails, a 400 error response will be generated automatically
return Response.ok("Profile created successfully").build();
}
}
Why: Using validation allows for preemptive error handling, reducing the likelihood of encountering binding issues downstream.
2. Specify Data Types Acutely
When developing forms, be meticulous about specifying data types. For example, using <input type="number">
for age in your HTML form can help prevent incorrect data entry.
<form action="/profile" method="post">
Name: <input type="text" name="name" required>
Age: <input type="number" name="age" required>
Email: <input type="email" name="email" required>
<button type="submit">Submit</button>
</form>
Why: Properly specifying input types in the HTML ensures that client-side validation occurs before sending data to the server.
3. Exception Handling
Implement global exception handling to catch binding-related exceptions. This consolidates your error management and makes it more maintainable.
import javax.ws.rs.core.Response;
import javax.ws.rs.ext.ExceptionMapper;
import javax.ws.rs.ext.Provider;
@Provider
public class BindingExceptionHandler implements ExceptionMapper<ConstraintViolationException> {
@Override
public Response toResponse(ConstraintViolationException exception) {
return Response.status(Response.Status.BAD_REQUEST)
.entity("Input data failed validation: " + exception.getMessage())
.build();
}
}
Why: Handling exceptions gracefully ensures users receive friendly feedback instead of generic error messages that discourage further interaction.
4. Testing Your Bindings
Regularly test your bindings using unit or integration tests. This practice allows you to identify potential issues early and protect your application from unexpected user input.
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
public class ProfileTest {
@Test
public void testValidProfile() {
Profile profile = new Profile();
profile.setName("John Doe");
profile.setAge(30);
profile.setEmail("john@example.com");
assertNotNull(profile.getName());
assertTrue(profile.getAge() > 0);
assertTrue(profile.getEmail().contains("@"));
}
}
Why: Testing is fundamental to ensuring reliability in your application. Catching binding issues before they reach production saves time and headaches.
5. Utilize REST Client Libraries
Adopting REST client libraries (like Apache HttpClient or Retrofit) can simplify and streamline data binding between the client and server.
For a comprehensive guide on choosing the right library, refer to REST Client Libraries.
Why: These libraries often have built-in validation mechanisms, facilitating easier data management.
The Last Word
Binding issues in Java EE 8 MVC frameworks can profoundly affect the user experience and the integrity of your application. By implementing the strategies discussed above—validations, efficient exception handling, and thorough testing—you can mitigate these challenges. A robust binding mechanism not only enhances data integrity but also fosters a more reliable application, allowing developers to focus on crafting features rather than troubleshooting errors.
For those looking to deepen their understanding of Java EE, consider exploring Java EE 8 MVC Specification for official documentation and additional resources.
Whether you're a seasoned developer or just starting your journey with Java EE, mastering binding issues is essential for creating dependable, user-friendly web applications.