Avoiding Memory Leaks: The Case for Listener Handles
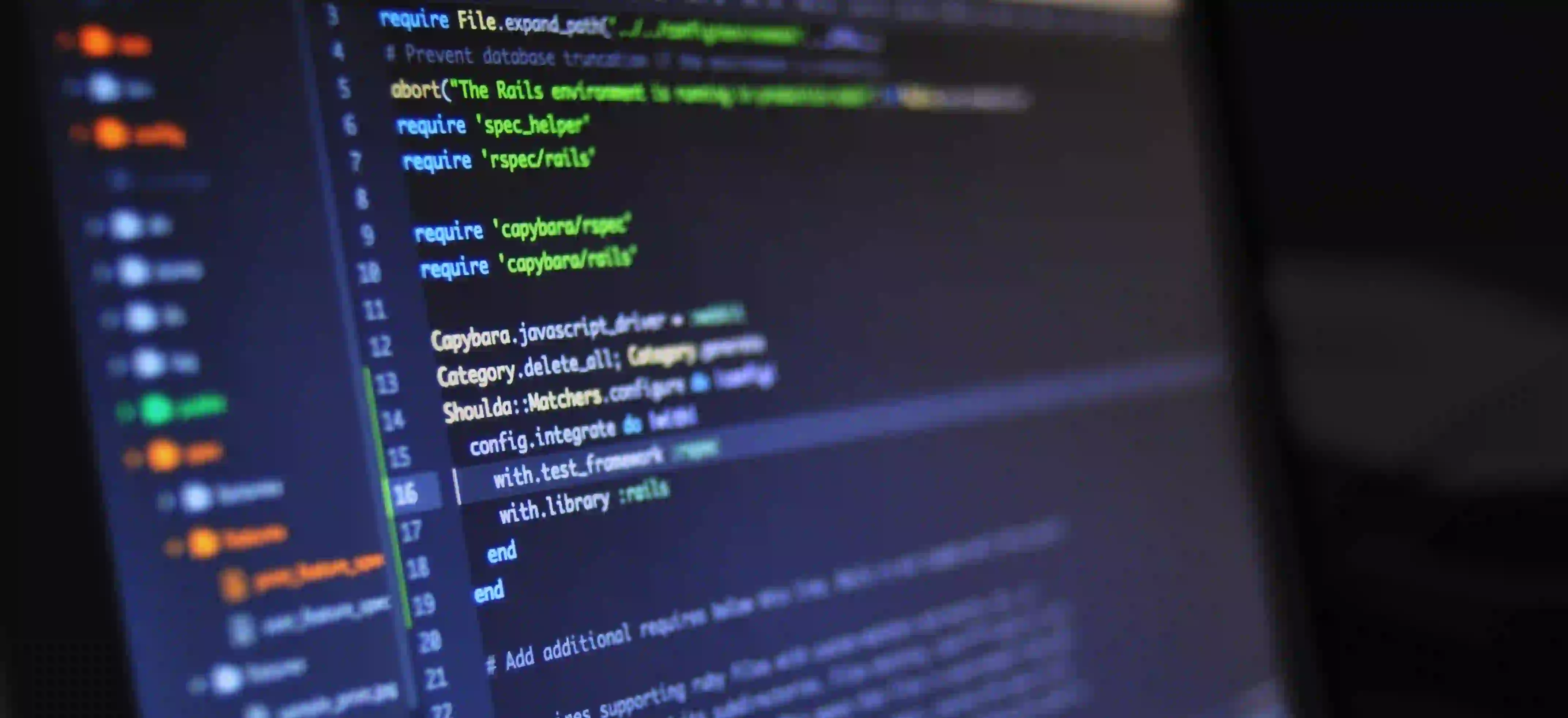
Avoiding Memory Leaks: The Case for Listener Handles in Java
Memory leaks can significantly degrade the performance of Java applications. They can result in increased memory consumption, application slowdowns, and eventual crashes. One prevalent cause of memory leaks in Java is the improper handling of event listeners. This blog post will focus on how to avoid memory leaks by effectively managing listener handles in Java applications.
Understanding the Problem
In Java, event-driven paradigms are prevalent, where components like GUI frameworks and server applications use listeners to handle events (such as clicks, key presses, etc.). A common mistake developers make is not properly unregistering these listeners when they are no longer needed. When this happens, the objects that should be garbage collected remain in memory, resulting in a memory leak.
For instance, consider an object that registers a listener for updates from another object. If the registering object is destroyed but the listener remains active, the garbage collection mechanism cannot reclaim the memory used by the listener's parent object.
Example Scenario
Let’s explore a trivial example where a GUI application listens for button clicks. Here’s a simplistic framework:
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class MemoryLeakExample {
private JButton button;
private ActionListener listener;
public MemoryLeakExample() {
button = new JButton("Click Me");
listener = new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("Button clicked!");
}
};
button.addActionListener(listener);
// GUI setup here...
}
// Simulate the destruction of the object
public void cleanup() {
// The listener is still attached, causing a memory leak
System.out.println("Cleaning up...");
}
}
In the code above, when the MemoryLeakExample
object is cleaned up, the listener
still holds a reference to the MemoryLeakExample
instance. Unless the listener is explicitly unregistered, the instance will remain in memory, leading to a potential memory leak.
The Case for Listener Handles
To tackle this problem, we can use listener handles that allow us to manage event listeners more efficiently. A listener handle encapsulates both the event listener and its association with the source of events. This approach clears up references, ensuring they get removed when the object is no longer needed.
Implementing Listener Handles
Let’s modify our previous example to implement listener handles correctly:
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class MemorySafeExample {
private JButton button;
private ActionListener listener;
public MemorySafeExample() {
button = new JButton("Click Me");
listener = new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("Button clicked!");
}
};
button.addActionListener(listener);
// GUI setup to add button to a JFrame etc.
}
public void cleanup() {
// Unregister the listener when cleaning up
button.removeActionListener(listener);
listener = null; // Help GC by clearing the reference
System.out.println("Cleanup completed, listener unregistered.");
}
}
Explanation
-
Unregistering the Listener: When the
cleanup
method is called, we explicitly remove the listener from the button usingbutton.removeActionListener(listener)
. By doing this, we eliminate the reference that prevents garbage collection of the listener’s enclosing class. -
Setting References to Null: After unregistering the listener, we set
listener
tonull
. While this is not strictly necessary for garbage collection, it signifies that we will not use this reference anymore and can help the garbage collector reclaim memory.
Best Practices for Managing Listener Handles
-
Use Weak References: For complex applications with a high number of listeners, consider using weak references. Java's
WeakReference
allows for listeners to be removed when they are no longer strongly reachable. -
Framework-Specific Patterns: Utilize frameworks that naturally manage listener lifecycles, such as JavaFX or certain libraries that implement event bus patterns. These frameworks handle listener registration and unregistration elegantly.
-
Self-Destructing Listeners: Incorporate inner classes or factory patterns that can automatically unregister themselves when the parent object gets collected.
-
Documentation and Code Reviews: Ensure your team is aware of memory leaks and consistently review code for proper listener management.
Engaging with the Community
Incorporating these practices helps reduce memory leaks significantly. It's also valuable to engage with the Java community through forums such as Stack Overflow and repositories on GitHub to share common pitfalls and solutions.
My Closing Thoughts on the Matter
By understanding the importance of listener handles in Java applications and utilizing the right strategies, developers can effectively avoid memory leaks and enhance application stability and performance. Emphasizing clean registration and unregistration of listeners not only optimizes memory usage but also establishes better coding practices.
For further reading on managing memory in Java, consider checking out Oracle's Garbage Collection Tuning Guide.
By consistently applying these principles, we can create more efficient Java applications, free from the clutches of memory leaks. Remember, good memory management is not just a technical choice; it’s a commitment to building robust software.