Struggling with Type Inference in Java 7? Here's Help!
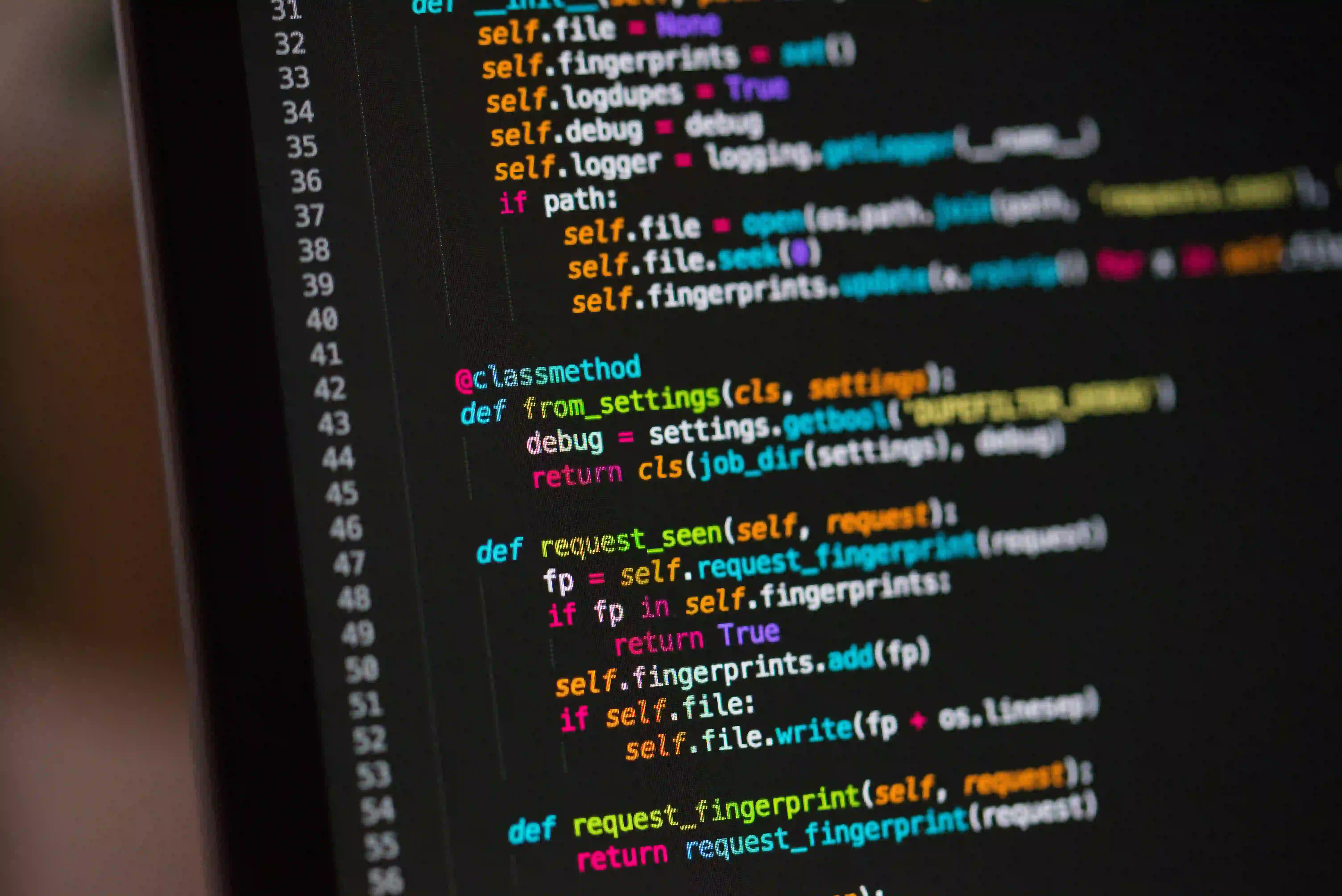
Struggling with Type Inference in Java 7? Here's Help!
Java has always been a strongly typed language, which means that you must explicitly declare the type of variables. However, with the introduction of Java 7, a more flexible feature came into play: Type Inference. This allows Java developers to write cleaner and more maintainable code by letting the compiler deduce the types of variables based on context. This blog post will guide you through the nuances of type inference in Java 7, helping you to harness its capabilities effectively.
What is Type Inference?
Type inference is a feature that enables the compiler to determine the type of a variable automatically. Instead of explicitly specifying the type, you can let Java's compiler make the decision for you in certain contexts. This is particularly useful in generics, which can often be verbose.
Here's an example to illustrate the basics of type inference:
List<String> stringList = new ArrayList<>();
As seen above, we explicitly declare the type of ArrayList
as String
. However, there's a cleaner way to declare this using type inference, as shown next:
var stringList = new ArrayList<String>();
Note that this syntax isn’t available in Java 7. The var
keyword made its debut in Java 10, but you'll want to remember that even without var
, type inference can significantly clean up your code.
The Benefits of Type Inference in Java 7
-
Reduced Boilerplate Code: When using collections or generics, you often have to repeat type arguments. With type inference, you can reduce the redundancy.
-
Improved Readability: Cleaner code is easier to read and maintain. Reducing the noise in variable declarations helps coders focus on the actual logic without the distraction of overly verbose type specifications.
-
Enhanced Expressiveness: It allows for more expressive coding styles, particularly when dealing with complex generic types.
Type Inference in Generics
The introduction of the diamond operator (<>
) in Java 7 was a game-changer. This operator allows you to simplify generic type declarations. Here’s how it works:
Example with the Diamond Operator
Map<String, List<String>> myMap = new HashMap<>();
In this case, you see how the type can be inferred without repeating it:
// Before Java 7
Map<String, List<String>> myMap = new HashMap<String, List<String>>();
Commentary on the Example
Using the diamond operator makes the code cleaner and reduces the clutter that comes from repeating types unnecessarily. This kind of inference can greatly simplify your code, especially when nested generics are involved.
Limitations of Type Inference
While type inference does provide advantages, it is essential to be aware of its limitations. Type inference does not apply in situations where the type cannot be unequivocally determined. For instance:
// Error: The compiler can't determine the type
var myList = new ArrayList<>(); // This will cause an error in Java 7
The appropriate declaration would be:
List<String> myList = new ArrayList<String>();
Here, the type must be explicitly specified.
Working with Collections and Streams
With Java 7 specifically, types are vital when dealing with collections and data streams. Although Java 8 introduced streams and lambda expressions, understanding how type inference contributes is crucial.
Example of Using Collections
Let's consider filtering with Collections through a simple example where we want to filter even numbers from a list:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
List<Integer> evenNumbers = new ArrayList<>();
for (Integer number : numbers) {
if (number % 2 == 0) {
evenNumbers.add(number);
}
}
Though valid, it involves some boilerplate. In scenarios with Java 8, we could use the Stream API which further reduces the verbosity.
Commentary
Java 7 provides the tools necessary for effectively managing types and collections. Code is more straightforward, focusing primarily on relevant business logic rather than declarations. However, most enhancement opportunities arise in Java 8 and beyond.
Best Practices for Using Type Inference
To maximize the advantages of type inference in Java 7 and beyond, consider the following best practices:
-
Maintain Clarity: While type inference simplifies code, ensure that your code remains clear. Type inference should not lead to confusion about what type a variable is.
-
Prefer the Diamond Operator: Always favor using the diamond operator when working with generics. This habit will not only make your code cleaner but will also prepare you for newer versions like Java 8 and later.
-
Check Compatibility with Other Types: When in doubt, be explicit about your types, especially when dealing with APIs or frameworks where inferring might not lead to clear understanding.
-
Use Integrated Development Environments (IDEs): Modern IDEs provide insights into inferred types as you type. Leverage these tools to enhance your coding efficiency.
Final Considerations
Type inference in Java 7 is a powerful feature that simplifies type declarations and improves code readability. The introduction of the diamond operator allows for cleaner code, although it's essential to remain clear about type contexts. While you may find limitations in Java 7, the principles laid out here will help you write efficient code.
As you grow more comfortable with Java's type inference—whether in Java 7 or later versions—you'll notice enriched productivity and happiness in development.
For further information, consider diving into Java's Official Documentation or exploring additional resources such as Java Generics.
Happy coding!