Common Mistakes When Creating AAR Files with Gradle
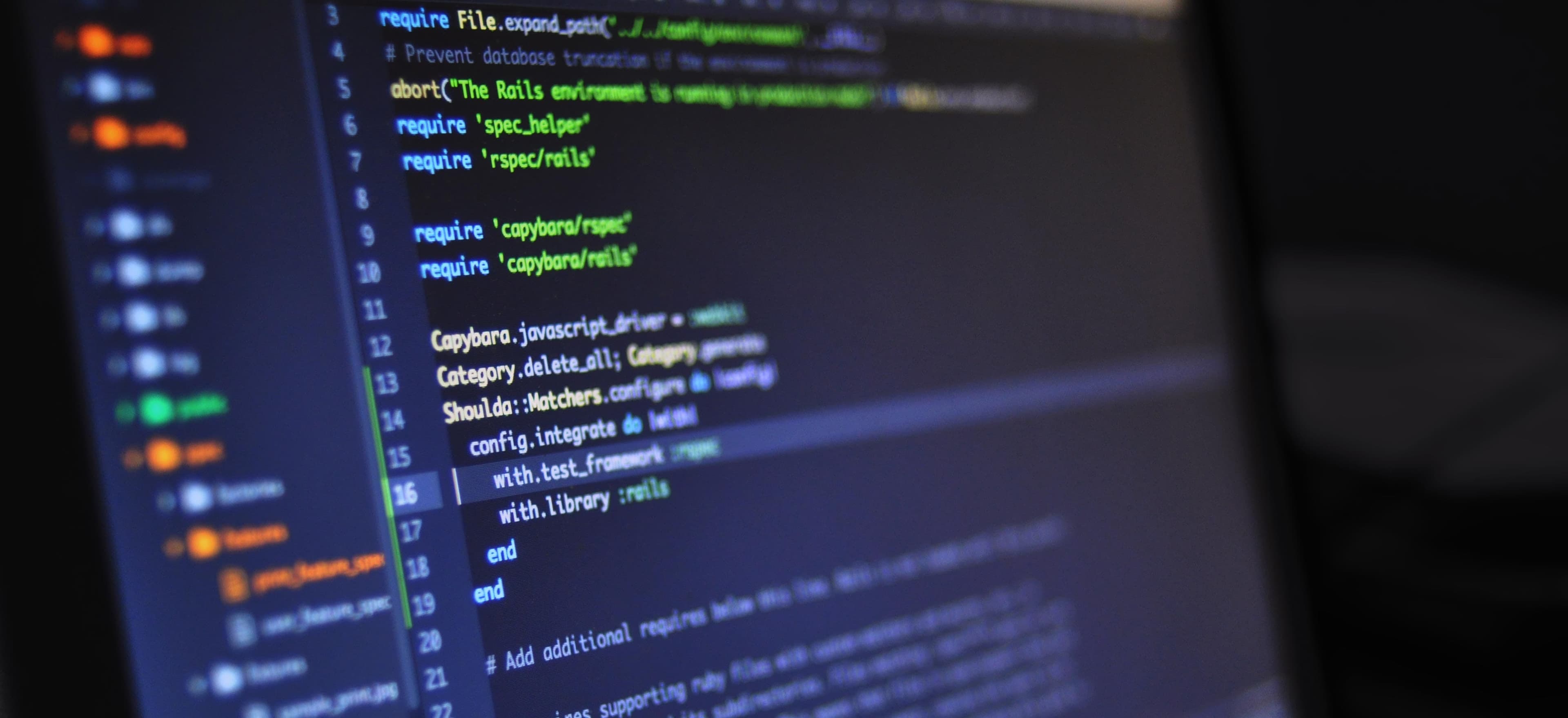
- Published on
Common Mistakes When Creating AAR Files with Gradle
When developing Android applications, creating a library to encapsulate reusable code can greatly improve maintainability and organization. One common way to package an Android library is through an Android Archive (AAR) file. The AAR file format allows the distribution of your libraries with all necessary resources and Manifest files bundled together. Using Gradle to build AAR files is standard; however, several common mistakes can lead to issues. In this blog post, we will discuss these common pitfalls, explain how to avoid them, and provide best practices for creating AAR files effectively.
What is an AAR File?
Before diving into the mistakes, let’s briefly summarize what an AAR file is. An AAR (Android Archive) is a format used for packaging Android libraries. It contains:
- Compiled code (in the form of
.class
files). - Resources (layouts, drawables, etc.).
- Manifest files.
- Proguard configuration.
This packaging allows developers to include such libraries in their apps seamlessly.
Common Mistakes
Let’s explore the common mistakes developers often encounter when creating AAR files with Gradle.
1. Missing Dependency Declaration
One of the primary mistakes is failing to declare dependencies used by the library in the build.gradle
file.
Example:
dependencies {
implementation 'com.squareup.retrofit2:retrofit:2.9.0' // Missing dependency declaration
}
Why it Matters:
If the dependencies are not declared, any application using your AAR file will crash with a NoClassDefFoundError
indicating that required libraries are not present.
Solution:
Always declare all external dependencies in your build.gradle
file to ensure that they are packaged with your library.
dependencies {
implementation 'com.squareup.retrofit2:retrofit:2.9.0' // Ensure proper dependency declaration
}
2. Not Including Resources
Another common pitfall is neglecting to include necessary resources such as images, layout files, or string resources with the AAR.
Why it Matters:
If resources are not included, any references in your library code will break, leading to NullPointerExceptions at runtime.
Solution:
Make sure to place all required resources inside the src/main/res
folder of your library module. Always check that these files are accessible and properly referenced in your code.
3. Incorrect Package Structure
Android libraries must adhere to a specific package structure. Mistakes in package naming conventions can lead to compile errors.
Why it Matters: Improper package structure can cause issues while working with your library in other projects, especially regarding naming conflicts.
Solution:
Follow Java package naming conventions, using lower case letters for package names and adhering to the reverse domain name system (e.g. com.yourcompany.yourlibrary
).
4. Not Using the aar
Plugin
A common error for beginners is not applying the AAR plugin in the build.gradle
file.
Example:
apply plugin: 'com.android.library' // This should be present
Why it Matters:
Failing to declare your module as a library will prevent it from packaging as an AAR.
Solution: Always apply the library plugin for the module intended to be built as an AAR:
apply plugin: 'com.android.library'
5. Ignoring Proguard Configuration
Proguard is a tool that shrinks and obfuscates your code. Not including the proper Proguard configuration can make your library unusable.
Why it Matters:
Without proper Proguard rules, users of your library may encounter issues with unavailable classes and methods after obfuscation.
Solution:
Provide a Proguard file with recommendations on rules that users should include when using your AAR. This file should be located in src/main/proguard/
.
Example proguard-rules.pro
:
-keep class com.yourcompany.yourlibrary.** { *; }
6. Not Updating API Documentation
Another overlooked aspect is the lack of generated documentation for the API exposed through the library.
Why it Matters:
Documentation is essential for users of your library. Without it, users may struggle to understand how to use your library.
Solution: Use tools like Javadoc to generate comprehensive API documentation. This can be done by running:
gradle javadoc
7. Failing to Test the AAR Locally
Skipping the testing phase is a critical error. Developers often assume that their AAR compilation is flawless.
Why it Matters:
Without testing, you risk releasing a defective library, leading to frustration for developers who will implement it.
Solution: Create a sample application that consumes your AAR and thoroughly test it. This can catch issues related to dependency conflicts and resource mismanagement early.
8. Lack of Version Control
Another mistake is neglecting proper versioning of your AAR files.
Why it Matters:
Without version control, users may face challenges when updates are made to the library. Different versions can lead to unexpected behavior and breaking changes.
Solution: Follow Semantic Versioning for your library releases. For example:
version = '1.0.0' // Update following semantic versioning guidelines
Packaging AAR With Gradle
Once you have taken care of the common issues, creating and packaging your AAR file can be performed simply by running the Gradle command:
./gradlew assembleRelease
This command compiles your code and packages everything into an AAR file located in:
<project-root>/your-library/build/outputs/aar/
Closing Remarks
Creating AAR files with Gradle in an Android development environment can be straightforward, provided you follow best practices to avoid common pitfalls. Proper dependency management, resource handling, package structure, Proguard configuration, documentation, testing, and version control are essential steps to ensure your library is functional.
For a deeper understanding of Gradle and its capabilities, consider checking out the official Gradle documentation. It offers extensive guidance on all aspects of building and packaging libraries.
By adhering to these rules and frameworks, you can create effective, reusable libraries that make development easier for everyone involved. Happy coding!