Optimizing Performance: Common Bavet Integration Pitfalls
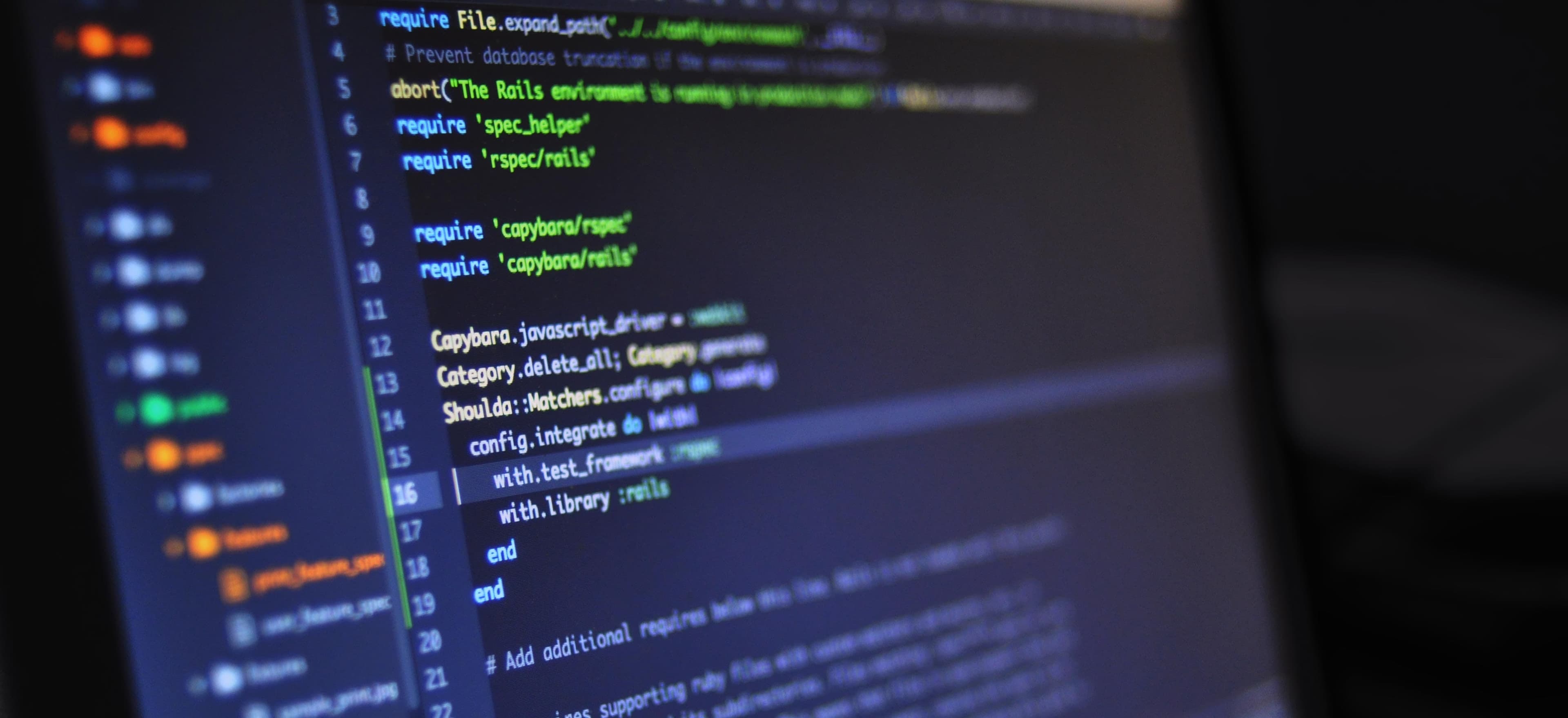
- Published on
Optimizing Performance: Common Bavet Integration Pitfalls in Java
In the realm of Java development, performance optimization is a critical component for creating high-performance applications. One approach gaining traction is the integration of Bavet, a reactive and performant constraints solver for Java. While Bavet offers significant performance improvements, it also comes with its own set of challenges. In this article, we will explore common pitfalls encountered when integrating Bavet, providing strategies for effective use and performance optimization.
Understanding Bavet
Before diving into the pitfalls, it's essential to understand what Bavet is. Bavet is designed to optimize constraint satisfaction problems, leveraging incremental computation techniques. This allows developers to create applications with dynamic and reactive user interfaces, particularly beneficial for data-driven applications.
The core benefit of Bavet lies in its ability to handle constant changes efficiently. For instance, when dealing with user inputs that affect the layout of a GUI, Bavet recalculates only the necessary constraints rather than recalculating everything from scratch. However, as powerful as it is, improper integration can lead to inefficiencies.
Common Bavet Integration Pitfalls
1. Overusing Constraints
One of the most typical mistakes developers make is overusing constraints. While Bavet is capable of handling complex constraint graphs, adding too many constraints can lead to performance bottlenecks.
Solution:
Focus on the key constraints that materially affect your application's logic. Simplify your models where possible.
Example:
ConstraintSource sourceA = new ConstraintSource();
ConstraintSource sourceB = new ConstraintSource();
// Only add constraints that are necessary
sourceA.addConstraint(new Constraint() {
@Override
public void satisfy() {
// Your logic here
}
});
// Excessive constraints can lead to performance issues
2. Ignoring Constraint Dependencies
Another pitfall is neglecting the relationships between different constraints. Bavet performs optimally when it can trace dependencies effectively. When developers do not properly track these dependencies, it can lead to unnecessary recalculation of constraints.
Solution:
Make sure to define and document the relationships between your constraints clearly. Use refined variable bindings strategically.
Example:
Bindable<Integer> width = new Bindable<>(400);
Bindable<Integer> height = new Bindable<>(300);
// Define dependencies clearly
Constraint.bind(width, height, (w, h) -> w * h); // Example of maintaining relation
3. Not Profiling the Performance
Failing to profile performance while integrating Bavet is a missed opportunity. Profiling provides insights into the specific areas where performance lags, which is crucial for effective optimization.
Solution:
Leverage tools like JVisualVM or YourKit to identify bottlenecks and focus your optimization efforts effectively.
Example:
# Starting JVisualVM
jvisualvm
4. Setting Up Incorrect Contexts
Many developers mistakenly set up incorrect contexts for their constraints, leading to performance issues. For example, if you attach constraints to a context that is seldom used, Bavet performs unnecessary checks.
Solution:
Always match your constraints to the correct context based on usage scenarios.
Example:
Bindable<Point> point = new Bindable<>(new Point(0, 0));
// Ensure this constraint is only active when necessary
constraintSet.addConstraint(new VisibilityConstraint(point).context(myContext));
5. Skipping Testing for Dynamic Updates
Dynamic applications often experience a lot of changes. Skipping the necessary testing after making late adjustments can lead to performance pitfalls.
Solution:
Integrate testing into your development process. Use unit tests and performance tests to ensure that updates to constraints perform as expected.
Example:
@Test
public void testDynamicUpdate() {
Bindable<Integer> value = new Bindable<>(100);
// Observing performance under dynamic updates
assertTrue(performanceCheck(value));
}
Best Practices for Optimizing Bavet Integration
Having discussed the common pitfalls, let's shift our focus to best practices to ensure smooth Bavet integration and optimal performance.
Regularly Refactor Constraints
Refactoring your constraints often can aid in maintaining clarity and reducing redundant dependencies. Avoid bloated code that might lead to pitfalls.
Utilize Lighter Weight Bindings
Where possible, use lighter-weight bindings that consume fewer resources. Avoid overloading the system with heavy objects that have high memory footprints.
Conduct Impact Analysis
When introducing new constraints, always perform a performance impact analysis. This helps anticipate the performance implications of your changes upfront.
Keep Documentation Handy
Maintain solid external documentation outlining your constraints and their interdependencies. This is especially useful for teams that may not be familiar with your codebase.
Leverage Bavet Community Resources
Engage with the Bavet community through forums and repositories. Continuous learning and sharing of experiences can lead to discovering nuances that can dramatically improve your integration strategies.
For further in-depth information, check out Bavet on GitHub and the Bavet User Documentation.
Key Takeaways
Integrating Bavet can be a powerful toolkit in your Java development arsenal. However, without mindful consideration of its common pitfalls, developers may find themselves battling unnecessary performance issues. By understanding these pitfalls and applying best practices, you can harness Bavet’s strengths effectively, leading to better-performing applications. Regular assessment, testing, and community participation will keep your integration on the right track.
Remember, performance is not a one-time setup but an ongoing process of understanding, refining, and repeating. Happy coding!