Common Pitfalls of Java LocalDateTime with JPA
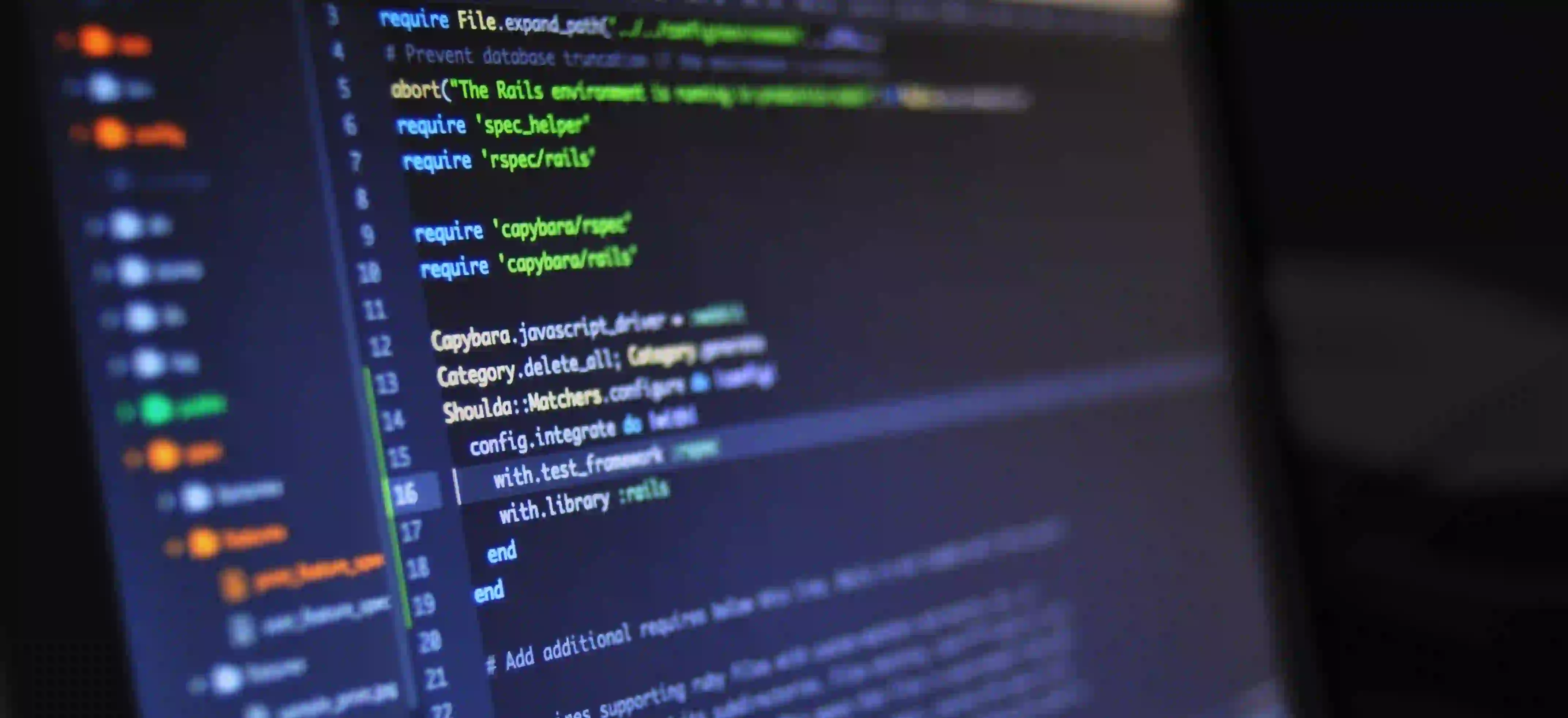
Common Pitfalls of Java LocalDateTime with JPA
Java's LocalDateTime
class is part of the Java 8 time API introduced to handle date and time more effectively. While it effectively represents a date-time without a time zone, using LocalDateTime
with Java Persistence API (JPA) brings its own set of challenges. This blog post will explore some common pitfalls when using LocalDateTime
with JPA, detailed explanations for each one, and how to overcome them.
Table of Contents
- Understanding LocalDateTime
- Pitfall 1: Storing in Database Without Time Zone
- Pitfall 2: Handling Serialization and Deserialization
- Pitfall 3: Default Values and Nullability
- Pitfall 4: Time Zone Awareness
- Strategies to Avoid Pitfalls
- Conclusion
Understanding LocalDateTime
Before diving into the pitfalls, let's clarify what LocalDateTime
is. The LocalDateTime
class represents a date-time with a precision down to nanoseconds. It is not tied to a specific time zone, making it ideal for applications where the concept of "local time" is relevant without ambiguity.
import java.time.LocalDateTime;
LocalDateTime dateTime = LocalDateTime.now();
System.out.println("Current DateTime: " + dateTime);
The above code snippet demonstrates how to acquire the current local date and time. Yet, it is crucial to recognize how this representation translates into database operations.
Pitfall 1: Storing in Database Without Time Zone
One of the critical issues when using LocalDateTime
in JPA is its behavior with respect to time zones. When you store LocalDateTime
in a database, it doesn't carry any time zone information. This can lead to severe complications if your application operates in multiple time zones or if you need to do timezone-based calculations.
Example
Imagine you are storing user activity timestamps in UTC but users are from different time zones:
@Entity
public class UserActivity {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private LocalDateTime actionTime; // No zone info, potential risks
// getters and setters
}
Suppose a user in New York performs an action at 3 PM EST. If you store this as a LocalDateTime
, it gets recorded as 2023-10-05T15:00:00
, without indicating that it happened in EST. Consequently, if you later query this timestamp from a user in another timezone, it could be misleading.
Solution
To avoid such pitfalls, consider using ZonedDateTime
instead, which includes time zone information.
import java.time.ZonedDateTime;
@Entity
public class UserActivity {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private ZonedDateTime actionTime; // Time zone included here
}
For more information on handling time zones effectively, refer to the Oracle documentation.
Pitfall 2: Handling Serialization and Deserialization
Serialization and deserialization issues treat LocalDateTime
poorly by default because JPA may not automatically convert it into the appropriate format when interfacing with a database. When sending or receiving JSON data, it’s common to use libraries like Jackson. By default, Jackson expects ISO-8601 format but the raw LocalDateTime
format can cause problems.
Example
Consider the following UserActivity
, where LocalDateTime is mapped incorrectly:
public class UserActivity {
private LocalDateTime actionTime; // May not serialize as expected
// getters and setters
}
Solution
You can customize the serialization and deserialization process by using Jackson annotations:
import com.fasterxml.jackson.annotation.JsonFormat;
public class UserActivity {
@JsonFormat(pattern = "yyyy-MM-dd'T'HH:mm:ss")
private LocalDateTime actionTime;
// getters and setters
}
With @JsonFormat
, you can specify how LocalDateTime
should be represented in JSON, avoiding common serialization issues.
Pitfall 3: Default Values and Nullability
Another issue arises regarding default values when using JPA. If the LocalDateTime
field in your entity does not have a default value, the database may initialize it to NULL. This behavior can lead to confusion, especially on retrieval.
Example
When querying UserActivity
without setting actionTime
, you might run into unexpected NULL values:
public void logActivity() {
UserActivity activity = new UserActivity();
// actionTime is not set and might return NULL
entityManager.persist(activity);
}
Solution
To ensure you always have a proper timestamp, initialize LocalDateTime
when creating entities.
public class UserActivity {
private LocalDateTime actionTime = LocalDateTime.now(); // Initialized
}
Pitfall 4: Time Zone Awareness
Java applications often deal with users across various time zones. Not being time zone-aware can lead to incorrect processing, misleading results, and ultimately, a poor user experience.
Example
Let's say you perform operations based on a LocalDateTime.valueOf("2023-10-05T15:00")
. You assume it's always in your local time zone, which may not apply to all users.
Solution
To manage time zone awareness gracefully, always convert LocalDateTime
to the relevant time zone when displaying or performing operations.
import java.time.ZoneId;
import java.time.ZonedDateTime;
public void displayLocalTime(LocalDateTime localDateTime) {
ZoneId zoneId = ZoneId.of("America/New_York");
ZonedDateTime zonedDateTime = localDateTime.atZone(zoneId);
System.out.println("Local DateTime: " + zonedDateTime);
}
By converting LocalDateTime
to ZonedDateTime
, you ensure the displayed time reflects the user’s time zone.
Strategies to Avoid Pitfalls
- Use ZonedDateTime: When storing or manipulating dates, prefer
ZonedDateTime
where time zone awareness is needed. - Define Serialization Routines: Use annotations or custom serializers to avoid serialization issues.
- Initialize Values: Ensure time-related fields have sensible defaults, minimizing the chance of NULL conflicts.
- Always Convert: Prioritize converting between
LocalDateTime
,ZonedDateTime
, and relevant time zones whenever displaying dates.
To Wrap Things Up
Though LocalDateTime
is a powerful and convenient way to handle date and time in Java, its pitfalls can lead to frustration and inaccuracies when interfaced with JPA. Awareness of these pitfalls—combined with the strategies suggested—will empower you to use LocalDateTime
effectively. With careful handling of time zones, serialization, and initialization, you can mitigate many common challenges and keep your application running smoothly.
For further reading on Java's date-time handling capabilities, check out the official Java Tutorials.
Remember, being proactive in the design phase can save you from potential headaches down the line!