Mastering Consistent Logger Naming with Log4j2
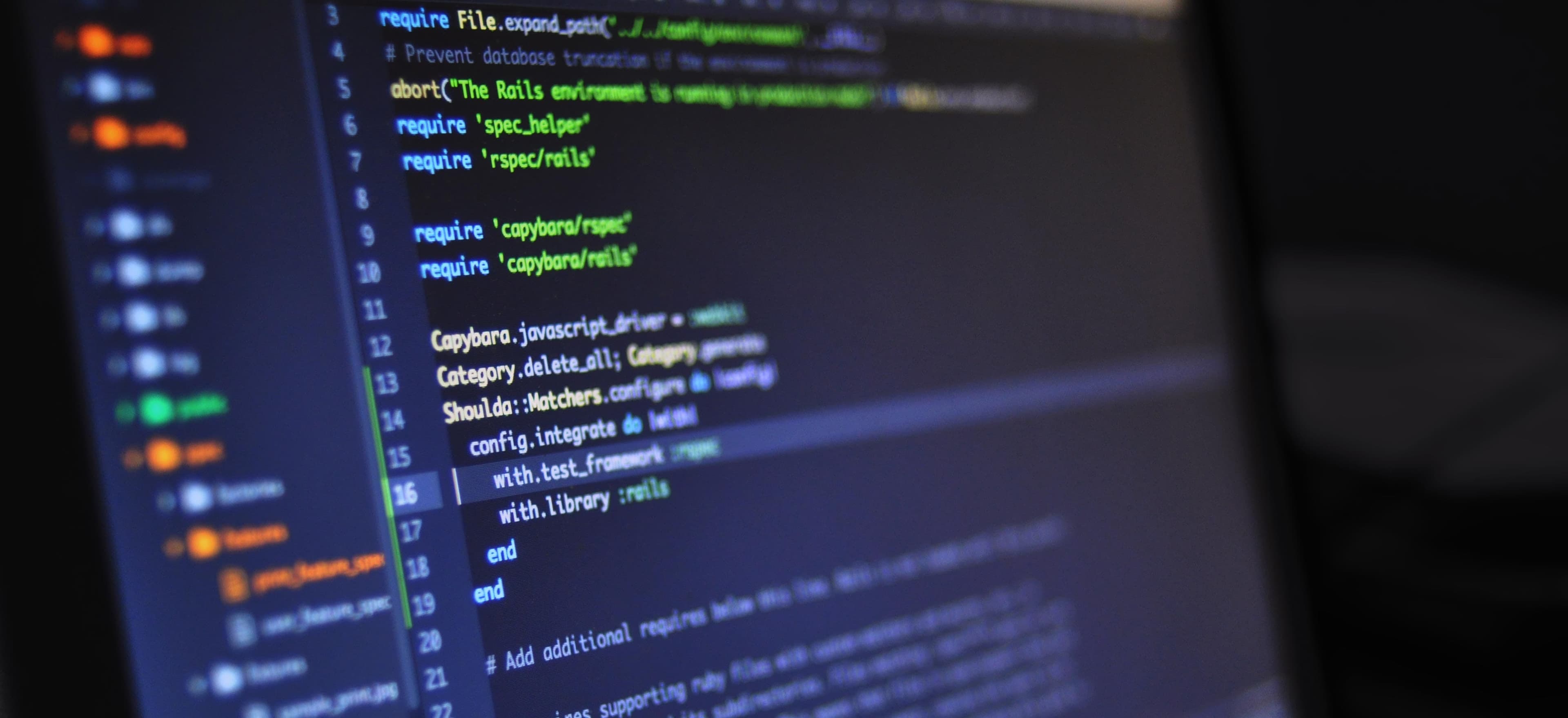
- Published on
Mastering Consistent Logger Naming with Log4j2
Logging is a crucial aspect of application development and management. With effective logging, developers and system administrators can diagnose issues, monitor performance, and maintain system reliability. One of the key components of successful logging is a consistent logger naming convention. In this blog post, we will discuss how to effectively use Log4j2, a popular logging framework, to establish and maintain consistent logger naming throughout your Java applications.
What is Log4j2?
Log4j2 is an open-source logging framework developed by the Apache Software Foundation. It is widely used for its flexibility, reliability, and scalability. The framework allows developers to create robust logging systems that can handle a high throughput of log messages. Log4j2 offers many features, including:
- Asynchronous logging
- Filters
- Custom logging levels
- Custom formatters
You can find more about Log4j2 in the official Apache Log4j documentation.
Why Logger Naming Matters
In a large Java application, there can be numerous classes and components. Each of these components might generate log messages to facilitate debugging and monitoring. A systematic approach to logger naming can contribute positively to:
- Readability: Consistent naming makes it easier for developers to identify where log messages originate. This improves the maintainability of the codebase.
- Filtering and Analyzing Logs: Log analysis tools can leverage consistent naming conventions for better filtering of log entries, thus improving problem identification.
- Navigation: Developers can quickly locate log statements of interest within large codebases.
Logger Naming Best Practices
1. Use Class-Based Naming
It is often recommended to name loggers using the fully qualified class name. This approach ensures that each logger is uniquely identifiable. For instance:
package com.example.application;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class UserService {
private static final Logger logger = LogManager.getLogger(UserService.class);
public void createUser(String username) {
logger.info("Creating user: {}", username);
// additional logic...
}
}
Why this is effective: By using UserService.class
for logger creation, it allows you to easily track logs specifically generated from the UserService
class.
2. Establish a Standard Logger Name Format
Consistency is key. Define a standard which might include information such as module name and functionality, if applicable. For instance:
package com.example.application.service;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class OrderService {
private static final Logger logger = LogManager.getLogger("com.example.application.service.OrderService");
public void processOrder(String orderId) {
logger.debug("Processing order with ID: {}", orderId);
// processing logic...
}
}
Why this is effective: By explicitly stating the logger name, you enhance clarity on which component of the application logs are being generated from.
3. Use Categories for High-Level Logging
In larger applications, you may want to categorize loggers based on functionality or module. You can create parent loggers for broader modules:
package com.example.application.service;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class PaymentService {
private static final Logger logger = LogManager.getLogger("com.example.application.service.PaymentService");
public void chargeCustomer(String customerId, double amount) {
logger.info("Charging customer {} an amount of {}", customerId, amount);
// charging logic...
}
}
Why this is effective: This allows for easier configuration of logger levels in the log4j2.xml
configuration file. You can decide to increase the verbosity of the entire service
category’s logs independently.
4. Avoid Logger Name Duplication
Always ensure that the logger names are unique across your application. If two classes unintentionally use the same logger name, it can cause confusion when filtering by logs.
5. Consistent Log Level Usage
Once you've established your loggers, it's equally essential to apply consistent logging levels (DEBUG, INFO, WARN, ERROR, FATAL) throughout your application. For example:
logger.error("An error occurred while processing order with ID: {}", orderId);
Why this is effective: Adhering to a structured logging level strategy helps in prioritizing logs. It also ensures that logs represent the operational status of the application accurately.
Example of Log4j2 Configuration
Log4j2 can be configured through XML or properties files. Here’s a sample log4j2.xml
configuration file to illustrate how you can set logging levels for the different modules in your application.
<Configuration status="WARN">
<Appenders>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{yyyy-MM-dd HH:mm:ss} %-5level %logger{36} - %msg%n"/>
</Console>
</Appenders>
<Loggers>
<Logger name="com.example.application.service" level="DEBUG" additivity="false">
<AppenderRef ref="Console"/>
</Logger>
<Root level="INFO">
<AppenderRef ref="Console"/>
</Root>
</Loggers>
</Configuration>
Why this is effective: This configuration sets the logging level for all classes within the service
package to DEBUG while keeping the root logger at INFO. As a result, debug logs from service classes will appear, while other logs will remain filtered.
Closing Remarks
Establishing a consistent logger naming convention with Log4j2 enhances your application's logging quality. It improves the readability, maintainability, and overall analysis of log messages. By embracing best practices such as utilizing class-based naming, integrating clear categorization, and understanding consistent log levels, your applications can become more manageable.
For further exploration into best practices, consider checking out this logging practices resource. Remember that good logging practices are vital to any software development lifecycle, as they can significantly improve troubleshooting efficiency.
Happy logging!