Common Spring Cloud Stream Pitfalls and How to Avoid Them
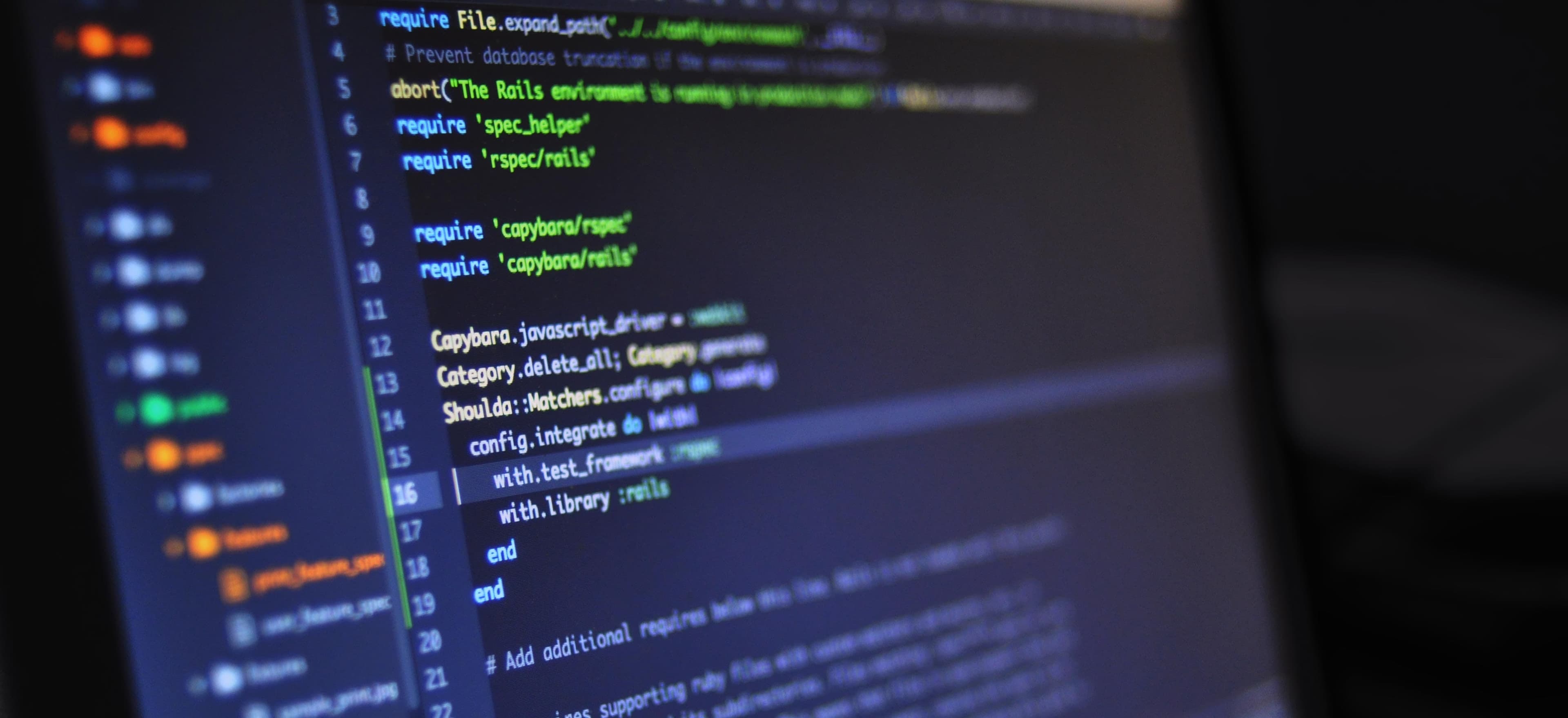
- Published on
Common Spring Cloud Stream Pitfalls and How to Avoid Them
Spring Cloud Stream offers a robust framework for building message-driven microservices. It simplifies the process of connecting to message brokers and managing messaging channels, but developers can encounter several pitfalls along the way. In this blog post, we will discuss some of the most common challenges and how to avoid them.
Understanding Spring Cloud Stream
Before diving into the pitfalls, it's essential to understand what Spring Cloud Stream is. It is a framework built on Spring Boot that enables the construction of event-driven microservices connected with shared messaging systems. With a programming model that abstracts the message broker, Spring Cloud Stream allows developers to focus on business logic rather than the complexities of messaging technology.
Key Features
- Binder Abstraction: Interacts with various messaging systems such as Kafka, RabbitMQ, etc.
- Declarative Programming Model: Simplifies messaging code through annotations and interfaces.
- Support for Partitioning: Handles scalability across multiple instances.
Common Pitfalls in Spring Cloud Stream
1. Misconfiguring Bindings
One of the most frequent mistakes developers make is misconfiguring their binding properties. Inadequate attention to the necessary configuration can lead to issues with message delivery and processing.
Example Configuration
spring:
cloud:
stream:
bindings:
input:
destination: inboundChannel
group: group1
output:
destination: outboundChannel
In the configuration above, we define bindings for both an input channel and an output channel. Ensure that the destination
and group
properties are correctly set up. Mismatched configurations can prevent consumers from receiving messages.
How to Avoid
- Double-check binding properties to ensure that they are correctly set up according to the documentation.
- Use integration tests to validate your configurations.
2. Ignoring Error Handling
Lack of proper error handling is another pitfall. By default, if exceptions are thrown during message processing, messages are lost unless you implement a strategy for error handling.
Example of Error Handling
@EnableBinding(Processor.class)
public class MessageProcessor {
@StreamListener(Processor.INPUT)
public void handle(String message) {
try {
// Process message
} catch (Exception e) {
// Log and handle error
throw new MessageProcessingException("Failed to process message", e);
}
}
}
In this snippet, we use a try-catch
block to catch any exceptions during message processing. If an error occurs, we throw a custom exception to signal that the message could not be processed.
How to Avoid
- Implement a dedicated error handler using the
@StreamListener
and handle exceptions gracefully. - Consider using a Dead Letter Queue (DLQ) to process unprocessable messages for later review.
3. Underestimating Message Serialization
Serialization can become a bottleneck if not handled properly. Understanding how messages are serialized and deserialized is crucial in keeping performance optimal.
Example of Custom Serializer
@EnableBinding(Processor.class)
public class CustomMessageSerialization {
@Bean
public MessageConverter messageConverter() {
return new GenericMessageConverter();
}
}
In this code snippet, we define a custom message converter that helps in converting messages to and from the desired format. Proper serialization can affect the size and speed of message processing.
How to Avoid
- Choose the right serialization format (e.g., JSON, Avro, Protocol Buffers).
- Regularly benchmark the serialization/deserialization performance.
4. Insufficient Logging
Without proper logging, debugging messaging issues can be a nightmare. Developers must ensure that they log important events and exceptions effectively.
Example of Logging
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@EnableBinding(Processor.class)
public class EnhancedLoggingProcessor {
private static final Logger logger = LoggerFactory.getLogger(EnhancedLoggingProcessor.class);
@StreamListener(Processor.INPUT)
public void handle(String message) {
logger.info("Received message: {}", message);
// Process message
}
}
In this case, we use SLF4J for logging purposes, which is widely accepted in Java applications. The logger captures every received message.
How to Avoid
- Implement comprehensive logging to trace the flow of messages.
- Use structured logging for easier search and analysis in log management tools.
5. Not Using the Functional Model
Spring Cloud Stream provides both an imperative and a functional style for processing messages. Ignoring the functional model can limit the flexibility and reactiveness of your code.
Example of Functional Model
@EnableBinding(Processor.class)
public class FunctionalProcessor {
@Bean
public Function<Message<String>, Message<String>> transform() {
return message -> {
// Transform message
return message;
};
}
}
By using the functional model, we can define functions for processing messages instead of using classes annotated with @StreamListener
.
How to Avoid
- Familiarize yourself with the functional programming model in Spring Cloud Stream.
- Consider using functions to encapsulate processing logic.
Final Considerations
Spring Cloud Stream offers powerful features to simplify building event-driven microservices, but knowing potential pitfalls is critical for successful implementation.
- Always double-check binding configuration to ensure proper setup.
- Implement robust error-handling strategies to avoid message loss.
- Optimize message serialization to enhance performance.
- Log significant events and exceptions for easier debugging.
- Embrace the functional programming model for more flexible code.
By being aware of these common pitfalls and how to avoid them, you will be better equipped to create reliable and maintainable microservices using Spring Cloud Stream. For more comprehensive information about Spring Cloud Stream, you can refer to the Spring official documentation and check out Guides on Spring Cloud.
Feel free to comment below with your experiences or any additional pitfalls you've encountered!