Troubleshooting Common Issues in Java EE 6 Galleria
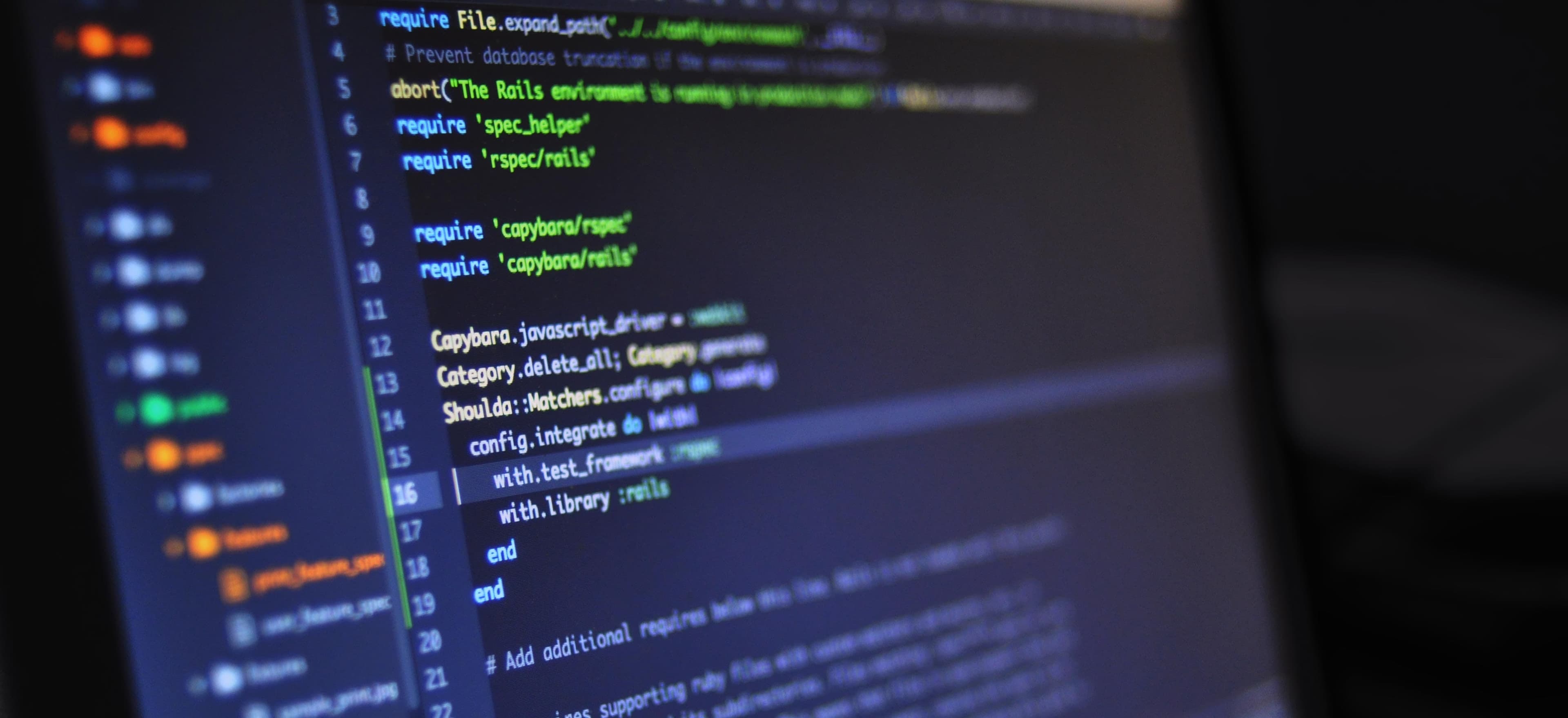
- Published on
Troubleshooting Common Issues in Java EE 6 Galleria
Java EE (Enterprise Edition) provides a robust platform for building large-scale, multi-tiered, scalable applications. The Galleria application, part of the Java EE tutorials, serves as an excellent example for developers interested in mastering the framework. However, encountering issues while working with Galleria or any Java EE application is common. In this blog post, we will address some of the most common issues faced in Java EE 6 Galleria and provide practical troubleshooting steps.
Understanding the Galleria Application Structure
Before diving into troubleshooting, it's essential to understand the architecture of the Galleria application. Galleria typically consists of:
- Frontend: This includes JSP (JavaServer Pages) files for presentation.
- Backend: Servlets, EJB (Enterprise JavaBeans), and beans that handle business logic.
- Persistence: Database interactions managed through JPA (Java Persistence API).
Each layer interacts with the others through well-defined interfaces, making the overall application robust yet complex.
Common Issues & Their Solutions
1. Dependency Injection Failures
Symptoms: Errors indicating failures in injecting beans or services.
Solution: Ensure that your beans are correctly annotated. For instance, if you're using an EJB, it should be properly annotated with @Stateless
or @Stateful
. Here's a simple example of a stateless EJB:
import javax.ejb.Stateless;
@Stateless
public class PhotoService {
public List<Photo> getAllPhotos() {
// fetch photos from the database
}
}
Why: Annotations are crucial in Java EE for dependency injection. If misconfigured, the container will not recognize the beans, leading to injection failures.
2. JSP Compilation Errors
Symptoms: The server throws errors related to the JSP files during deployment.
Solution: Check for syntactical errors in your JSP files. For example, ensure that all opened HTML tags are properly closed and that JSP directives are clearly defined. Here’s an example of a basic JSP file:
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<html>
<head>
<title>Photo Gallery</title>
</head>
<body>
<h1>Welcome to the Gallery</h1>
<c:forEach var="photo" items="${photos}">
<img src="${photo.url}" alt="${photo.description}" />
</c:forEach>
</body>
</html>
Why: JSP is prone to compile-time issues due to its dynamic nature; it’s essential to ensure that there are no typos or missing dependencies.
3. Database Connection Issues
Symptoms: NullPointerExceptions or database-related exceptions when attempting to access data.
Solution: Check the persistence.xml
configuration file for proper settings. Ensure that the database URL, username, and password are correctly specified. Here’s an example persistence configuration:
<persistence xmlns="http://xmlns.jcp.org/xml/ns/persistence"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/persistence
http://xmlns.jcp.org/xml/ns/persistence/persistence_2_0.xsd"
version="2.0">
<persistence-unit name="galleriaPU">
<provider>org.hibernate.jpa.HibernatePersistenceProvider</provider>
<class>com.example.Photo</class>
<properties>
<property name="javax.persistence.jdbc.url" value="jdbc:mysql://localhost:3306/galleria"/>
<property name="javax.persistence.jdbc.user" value="username"/>
<property name="javax.persistence.jdbc.password" value="password"/>
<property name="javax.persistence.jdbc.driver" value="com.mysql.cj.jdbc.Driver"/>
<property name="hibernate.dialect" value="org.hibernate.dialect.MySQL5Dialect"/>
</properties>
</persistence-unit>
</persistence>
Why: The configuration file serves as the connection point for JPA to interact with the database. Incorrect settings can lead to failures in establishing a connection.
4. ClassNotFoundException for Managed Beans
Symptoms: ClassNotFoundException errors during the deployment of WAR/EAR files.
Solution: Verify that your beans are located in the correct packages and are included in your build configuration. Adding your beans to the WEB-INF/classes
directory ensures that they are accessible to the container. For example:
package com.example.beans; // Correct package declaration
import javax.enterprise.context.RequestScoped;
@RequestScoped
public class UserBean {
private String username;
// getters and setters
}
Why: The application server needs access to all classes referenced in your application. If it cannot find them, it will throw a ClassNotFoundException.
5. JNDI Lookup Failures
Symptoms: Errors occur during JNDI (Java Naming and Directory Interface) lookups.
Solution: Make sure your resource reference in the web.xml
is consistent with what is defined in the application server’s configuration. Example configuration in web.xml
:
<resource-ref>
<description>DataSource for Galleria</description>
<res-ref-name>jdbc/GalleriaDB</res-ref-name>
<res-type>javax.sql.DataSource</res-type>
<res-link>jdbc/GalleriaDB</res-link>
</resource-ref>
Why: JNDI lookups rely on defined names to connect services, and a mismatch will result in lookup failures.
6. Client-Side Issues in AJAX Calls
Symptoms: Unresponsive UI or errors in the browser console during AJAX calls.
Solution: Ensure that your client-side JavaScript is error-free and that it's correctly configured to communicate with your backend. For example:
$.ajax({
url: "api/photos",
type: "GET",
success: function(data) {
populateGallery(data);
},
error: function(xhr, status, error) {
console.log("Error fetching photos: " + error);
}
});
Why: Client-side errors can significantly hinder user experience. Debugging JavaScript is equally important as server-side troubleshooting.
Additional Tips for Effective Troubleshooting
-
Logs are Your Friends: Always monitor your server logs. They provide vital insights into what might be going wrong.
-
Version Compatibility: Ensure that your libraries and the Java EE version are compatible. Running old libraries with new configurations may cause issues.
-
Use Testing Frameworks: Leverage testing frameworks like JUnit or Mockito to isolate and test components independently.
-
Refer to Documentation: Never hesitate to consult the official documentation. It is frequently updated and a valuable resource for troubleshooting.
For further reading on Java EE best practices, you may refer to the official Java EE documentation or visit Baeldung for Java tips.
Key Takeaways
Troubleshooting Java EE applications requires patience and a systematic approach. The common issues highlighted in this post are just the tip of the iceberg, but with practice, troubleshooting these errors can become second nature. By understanding the fundamentals of your Galleria application and maintaining solid coding practices, you can effectively minimize and resolve potential issues.
Feel free to share your experiences and solutions to troubleshooting challenges in Java EE Galleria or any other related queries in the comments below! Happy coding!