Overcoming High-Scale Failure: Effective Isolation Strategies
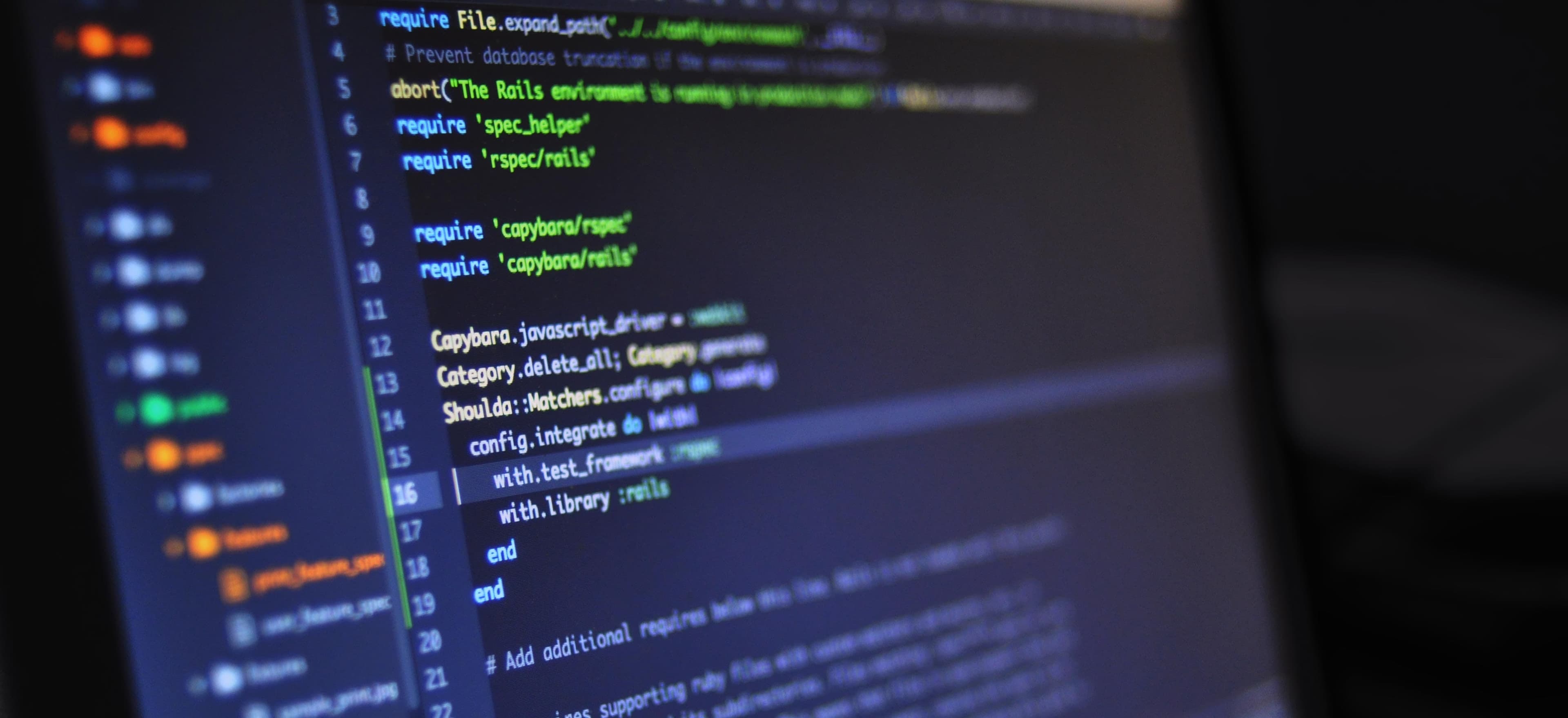
- Published on
Overcoming High-Scale Failure: Effective Isolation Strategies
In an era where applications serve millions of users, the potential for failure increases dramatically. High-scale systems can face numerous challenges, from server overload to software bugs, that can lead to service disruptions. One of the most effective approaches to managing these challenges is through isolation strategies. This blog post delves into the essence of isolation in high-scale systems, offering insights and code snippets to illustrate effective implementations.
Understanding Isolation Strategies
Isolation, in the context of software architecture, refers to the practice of separating components to reduce the impact of failures. Different isolation strategies can be applied depending on the architecture of the application and its specific needs. Here are some common isolation strategies:
- Service Isolation
- Database Transaction Isolation
- Thread Isolation
- Environment Isolation
Each of these strategies serves a critical purpose; let's explore them in more depth.
1. Service Isolation
Service isolation advocates breaking down monolithic applications into smaller, independent services, often referred to as microservices. This not only aids in fault tolerance but also simplifies updates and scalability.
Why Microservices?
Microservices allow teams to deploy updates independently. If one service encounters a failure, it doesn’t bring down the entire application, leading to better overall performance.
Here is a simple example in Java using Spring Boot that illustrates the creation of a microservice:
@RestController
@RequestMapping("/api/v1/user")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/{id}")
public User getUser(@PathVariable Long id) {
return userService.findById(id);
}
}
In this code snippet, we define a RESTful endpoint for retrieving user information. Each service can be independently maintained, allowing for a modular architecture.
Key Takeaway
Service isolation minimizes the risk of cascading failures in large-scale applications. You can learn more about microservices here.
2. Database Transaction Isolation
Database transaction isolation is crucial in systems where multiple transactions might occur simultaneously. It ensures that one transaction does not affect another, maintaining data integrity.
Isolation Levels
In SQL databases, there are several isolation levels defined by the ACID properties:
- Read Uncommitted
- Read Committed
- Repeatable Read
- Serializable
Each level offers a trade-off between performance and consistency. For instance, to set the isolation level in Java using JDBC, you can do something like this:
Connection connection = dataSource.getConnection();
connection.setTransactionIsolation(Connection.TRANSACTION_READ_COMMITTED);
Why Choose Read Committed?
The Read Committed level is often chosen because it provides a balance where dirty reads are prevented while still allowing for concurrent transactions. This prevents anomalies that could affect application performance.
Key Takeaway
Choosing the appropriate transaction isolation level is critical for maintaining data consistency without sacrificing performance. More information can be found here.
3. Thread Isolation
In high-scale applications, especially those handling multiple requests, thread isolation plays a vital role in maintaining performance. Thread pooling and proper handling of concurrent requests can significantly minimize overhead and increase efficiency.
Implementing Thread Pooling
Java provides robust threading capabilities through the Executors framework, which can be utilized to manage thread execution wisely:
ExecutorService executorService = Executors.newFixedThreadPool(10);
executorService.submit(() -> {
// Task implementation
System.out.println("Task executed by: " + Thread.currentThread().getName());
});
Benefits of Thread Isolation
By isolating tasks in a controlled thread pool environment, you avoid performance bottlenecks caused by context switching. Moreover, dedicated thread pools can help manage resource allocation more effectively.
Key Takeaway
Thread isolation aids in improving the system's responsiveness and resource management. You can find more about Java's Concurrency API here.
4. Environment Isolation
Environment isolation is vital in minimizing issues that arise from different deployment environments: development, testing, and production. Utilizing containers can improve the consistency of application deployment across different environments.
Docker as an Isolation Strategy
Docker allows developers to package applications in containers, ensuring they run the same way regardless of where they are deployed. A simple Dockerfile for a Java application might look like this:
FROM openjdk:11-jre-slim
VOLUME /tmp
COPY target/myapp.jar myapp.jar
ENTRYPOINT ["java","-jar","/myapp.jar"]
Why Use Docker?
By using Docker containers, you isolate the application from underlying environments. This reduces the friction caused by environmental inconsistencies that can lead to unexpected failures in high-scale systems.
Key Takeaway
Containerization provides a practical way to achieve environment isolation, contributing significantly to application reliability. Learn more about Docker here.
To Wrap Things Up
High-scale applications face numerous challenges that can lead to failures. By implementing effective isolation strategies, you can mitigate these risks and ensure better stability, performance, and reliability.
- Service Isolation: Helps in maintaining individual service functionality.
- Database Transaction Isolation: Maintains database integrity across transactions.
- Thread Isolation: Optimizes resource management for concurrent tasks.
- Environment Isolation: Ensures consistent application behavior across different deployment environments.
By understanding and applying these strategies, developers can create robust applications capable of handling high-scale demands effectively. Emphasizing the importance of isolation in your architecture can lead to a more resilient system.
If you would like to dive deeper into the principles of isolation or explore related concepts, consider checking the resources linked throughout this post. Happy coding!
Checkout our other articles