Understanding the Limitations of Duck Typing in Java
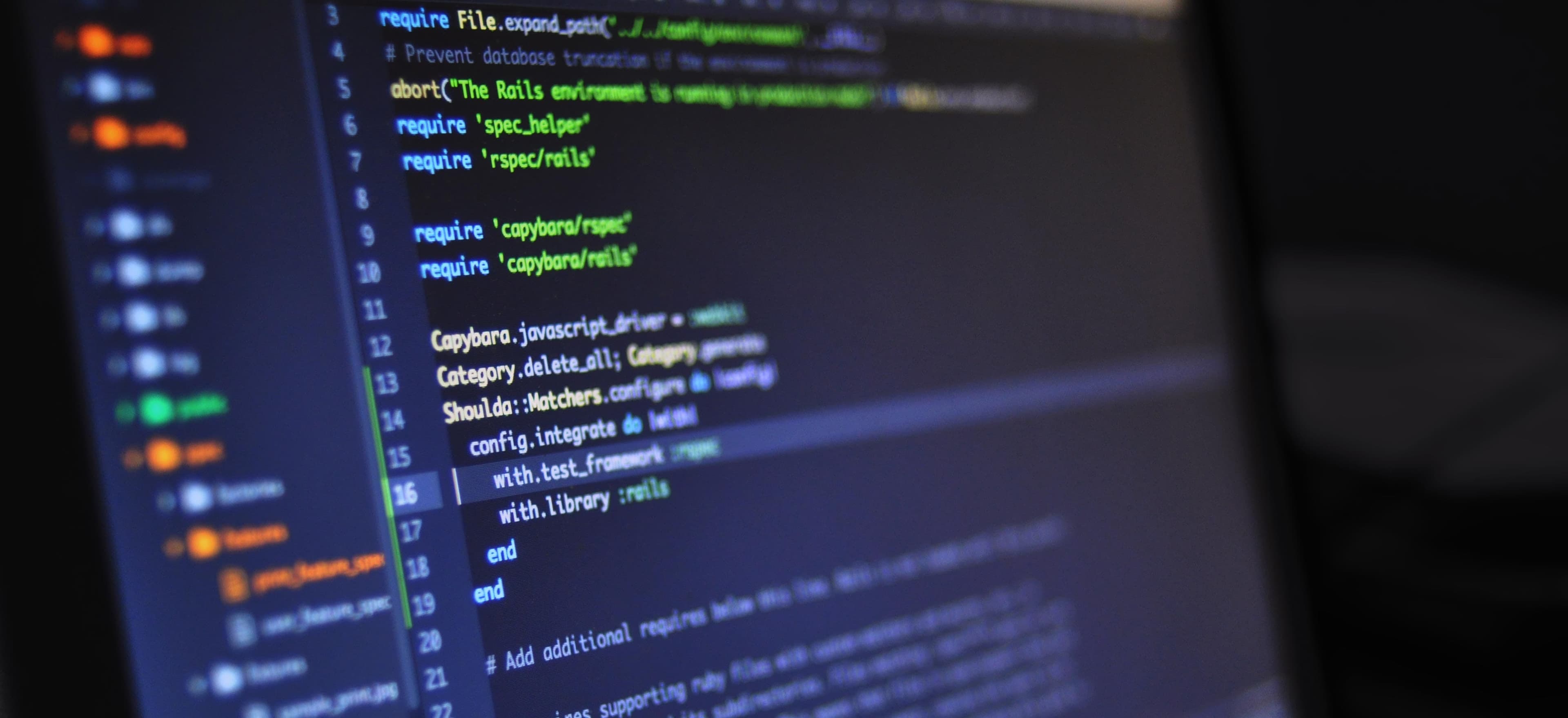
- Published on
Understanding the Limitations of Duck Typing in Java
Duck typing is a concept often associated with dynamic languages like Python or Ruby. In simple terms, it’s an approach where you do not necessarily rely on the explicit type of an object but rather on whether it has certain properties or methods. While this offers flexibility, it also introduces room for errors that can be challenging to debug. In this blog post, we’ll dive into the limitations of duck typing in Java, a statically typed language that does not inherently support this concept.
What is Duck Typing?
Duck typing derives its name from the saying, "If it looks like a duck, swims like a duck, and quacks like a duck, then it probably is a duck." This philosophy emphasizes that an object's suitability is determined by the presence of certain methods and properties, rather than the specific type of the object itself.
Example of Duck Typing in Dynamic Languages
Here’s a simple example in Python illustrating duck typing:
class Duck:
def quack(self):
print("Quack!")
class Dog:
def quack(self):
print("Woof! I mimic the duck!")
def sound_of_animal(animal):
animal.quack() # No type checking here
duck = Duck()
dog = Dog()
sound_of_animal(duck) # Outputs: Quack!
sound_of_animal(dog) # Outputs: Woof! I mimic the duck!
In this example, both Duck
and Dog
classes contain a quack()
method. The sound_of_animal()
function works without checking the actual type of the objects, which gives it the flexibility that duck typing is known for.
Duck Typing in Java: A Statically Typed Paradigm
Java, in contrast, is a statically typed language. This means that all variables must be declared with a type; the compiler checks these types during compile time. This leads Java to operate based on a strict type system, allowing for greater robustness and type safety, but it limits the flexibility akin to duck typing.
How Can Duck Typing Be Simulated in Java?
You can mimic duck typing in Java through interfaces and polymorphism. The interface allows for different classes to be treated similarly, based on the methods they implement.
interface Quackable {
void quack();
}
class Duck implements Quackable {
public void quack() {
System.out.println("Quack!");
}
}
class Dog implements Quackable {
public void quack() {
System.out.println("Woof! I mimic the duck!");
}
}
public class AnimalSounds {
public void soundOfAnimal(Quackable animal) {
animal.quack(); // Polymorphism at play
}
public static void main(String[] args) {
AnimalSounds animalSounds = new AnimalSounds();
Quackable duck = new Duck();
Quackable dog = new Dog();
animalSounds.soundOfAnimal(duck); // Outputs: Quack!
animalSounds.soundOfAnimal(dog); // Outputs: Woof! I mimic the duck!
}
}
Limitations of Duck Typing in Java
Even though we can simulate duck typing, it comes with certain limitations.
1. Verbose Syntax
The need to declare interfaces and classes becomes verbose. Whereas dynamic languages enable quick experimentation, Java's requirements for declaring types and interfaces can make it cumbersome.
2. Compile-Time Checks
Since Java checks types at compile-time, you miss the flexibility observed in dynamic languages. This ensures error handling is more structured but can prevent the rapid development cycles found in dynamic environments.
3. Inheritance Constraints
Java’s type system emphasizes inheritance which may not suit all scenarios. Duck typing allows individuals to write agile code without being constrained by the rigid structure of inheritance hierarchies.
4. Higher Learning Curve
For newcomers to Java, understanding how to implement interfaces and abstract classes may add to the learning curve. It can be particularly daunting for developers more accustomed to dynamically typed languages.
The Use Cases: When to Prefer Java's Strict Typing?
Java's strict typing offers undeniable benefits in various scenarios:
- Large-Scale Applications: The robustness provided by compile-time checks is invaluable in large, complex systems.
- Team Environments: With multiple contributors, clear interfaces prevent misunderstandings and mistakes — critical in collaborative environments.
- Performance Focused Applications: Static typing often leads to optimized performance, making Java suitable for performance-intensive applications.
Making the Most of Java's Type System
Concurrent to the exploration of duck typing limitations, it also helps to understand how we can harness the strengths of Java’s static typing:
-
Use Interfaces Judiciously: Interfaces could serve as the backbone for polymorphism without losing the advantages of type safety. Consider building a set of well-defined interfaces for your structures.
-
Generics: Java offers a way to achieve some level of flexibility through generics, allowing you to write more reusable code while maintaining strong type checks.
-
Functional Interfaces and Lambda Expressions: With Java 8, developers received the additional benefit of using functional programming constructs that can simplify code significantly.
import java.util.function.Consumer;
public class LambdaExample {
public static void main(String[] args) {
Consumer<String> quack = animal -> System.out.println(animal + " quacks!");
quack.accept("Duck"); // Outputs: Duck quacks!
quack.accept("Dog"); // Outputs: Dog quacks!
}
}
Utilizing lambda expressions can help bridge some gaps by allowing a lighter touch while still maintaining type safety.
Key Takeaways
While Java indeed does not support duck typing in the same ever-adaptive manner as dynamically typed languages, it offers mechanisms that allow for similar operational paradigms through interfaces and polymorphism. Understanding this contrast not only clarifies when and how to use Java effectively, but it reveals the strengths and weaknesses of each typing approach.
Developers must learn to appreciate these differences. Adapting to Java’s type system can yield benefits that outweigh the constraints presented by duck typing. Therefore, balancing type safety with the capabilities of polymorphism proves vital for proficient Java development.
For deeper insights into Java’s type system, you can explore Java Generics and Understanding Interfaces in Java.
Happy coding!
By understanding the limitations and solutions around duck typing in Java, developers can leverage the strengths of the Java language while navigating its challenges with clarity and purpose.
Checkout our other articles