Common Pitfalls for Java Developers in Elasticsearch Integration
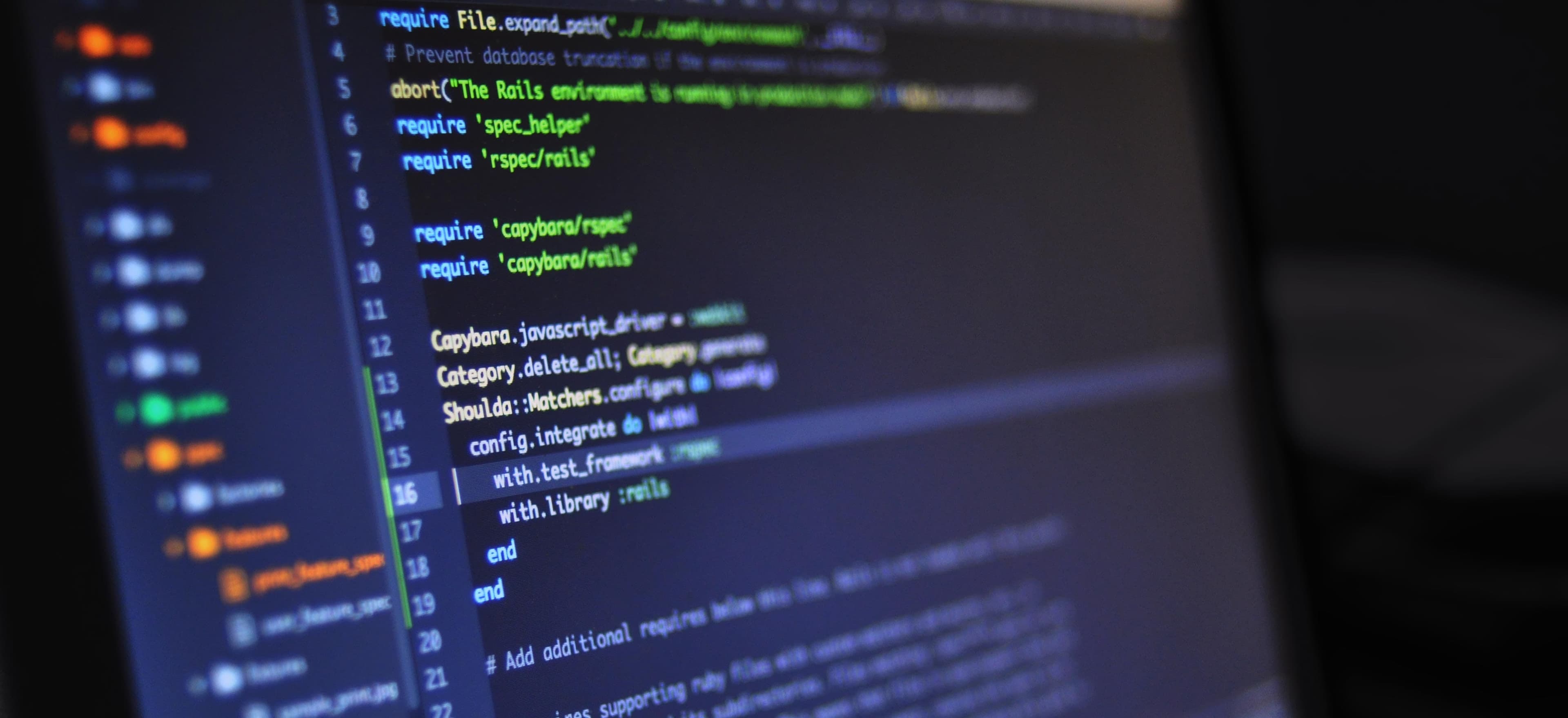
- Published on
Common Pitfalls for Java Developers in Elasticsearch Integration
Integrating Elasticsearch with Java applications can propel functionality and enhance the user experience. However, it is not without its challenges. This guide aims to illuminate common pitfalls Java developers encounter when working with Elasticsearch, helping you avoid mistakes and ensuring a seamless integration experience.
Understanding Elasticsearch
Elasticsearch is a powerful, distributed, and RESTful search engine built on top of Apache Lucene. It allows for scalable search and data handling, making it popular for applications that require full-text search capabilities. With its ability to handle huge amounts of data efficiently, integrating Elasticsearch in Java applications is a valuable skill.
However, improper integration can lead to performance issues and data inconsistencies. In this post, we will explore the common pitfalls and how you can avoid them.
Pitfall 1: Misunderstanding Indexing vs. Storing
One of the foundational concepts in Elasticsearch is the distinction between indexing and storing data.
- Indexing is the process of analyzing data and making it searchable.
- Storing refers to saving the original document.
Code Snippet
// Sample POJO for a document
@JsonInclude(JsonInclude.Include.NON_NULL)
public class Student {
private String id;
private String name;
// Constructors, getters, and setters
}
In the example above, we define a basic Student class. When you index this document in Elasticsearch, ensure you include all relevant fields for searching. Missing fields can lead to incomplete search results.
Why? Many developers overlook this distinction and try to store huge amounts of unnecessary data. This can lead to increased storage size and slower search performance.
Pitfall 2: Neglecting to Manage Versions
There is a version control mechanism built into Elasticsearch. However, many developers forget to manage versions effectively, leading to overwrites and data loss.
Code Snippet
UpdateRequest updateRequest = new UpdateRequest("students", "1")
.doc(jsonMap)
.upsert(jsonMap)
.setDocAsUpsert(true);
The above code snippet shows how to use the UpdateRequest
to efficiently manage document updates.
Why? If you fail to handle versions properly, you might overwrite data unintentionally. An effective strategy is to implement a versioning mechanism, including a proper handling of optimistic concurrency control.
Pitfall 3: Hardcoding Index Names
It's tempting to hardcode your Elasticsearch index names directly into your application. However, this practice can lead to issues when you need to change your index name or environment (development vs. production).
Code Snippet
public class ElasticConfig {
public static final String INDEX_NAME = "student_index";
// Other configurations
}
Instead of hardcoding, consider reading the index name from configuration files or environment variables.
Why? This practice allows you to change configurations without altering the codebase. It promotes maintainability and flexibility across environments.
Pitfall 4: Ignoring Connection Management
Establishing connections to Elasticsearch may seem trivial, but do not underestimate the importance of managing these connections effectively.
Code Snippet
RestHighLevelClient client = new RestHighLevelClient(
RestClient.builder(new HttpHost("localhost", 9200, "http")));
The sample above shows how to create a client connection. It is essential to properly close the client connection using a shutdown hook.
Why? Not managing connection lifecycles can lead to resource leaks and degraded performance, particularly in applications experiencing high traffic.
Pitfall 5: Not Leveraging Bulk Operations
Elasticsearch supports bulk operations, allowing you to send multiple requests in a single API call. Many developers fail to utilize this feature.
Code Snippet
BulkRequest bulkRequest = new BulkRequest();
bulkRequest.add(new IndexRequest("students").id("1").source(jsonMap));
bulkRequest.add(new IndexRequest("students").id("2").source(jsonMap2));
BulkResponse bulkResponse = client.bulk(bulkRequest, RequestOptions.DEFAULT);
In this code, we batch index operations, which can significantly improve performance.
Why? Not using bulk operations leads to increased latency and overhead in network traffic. Maximizing the efficiency of operations can vastly improve application performance.
Pitfall 6: Not Using the Right Data Types
Elasticsearch uses various data types for indexing, such as keyword
, text
, date
, and integer
. Improperly selecting data types can lead to unexpected behavior in searches.
Code Snippet
{
"mappings": {
"properties": {
"name": { "type": "text" },
"age": { "type": "integer" },
"registrationDate": { "type": "date" }
}
}
}
In this example, we define acceptable types for the name
, age
, and registrationDate
fields.
Why? Incorrect data types can affect queries and aggregations, leading to inaccurate results. Always experiment with mappings in a staging environment before applying changes to production.
Additional Resources
For interested developers looking to dive deeper, consider these resources:
- Elasticsearch Official Documentation: A comprehensive guide covering every aspect of Elasticsearch.
- Java High Level REST Client: Learn how to utilize the Java High Level REST Client for effective integration.
A Final Look
Integrating Elasticsearch within your Java applications can be a potent tool for enhancing search capabilities. However, acknowledging common pitfalls is crucial for leveraging its full potential. By understanding indexing, managing versions, avoiding hardcoded values, managing connections, using bulk operations, and selecting appropriate data types, you can ensure a more efficient and robust integration process.
Avoid these common mistakes to help your applications stand out. Mastering these principles will not only improve performance but also enhance your overall development process. Happy coding!
Checkout our other articles